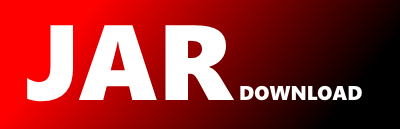
com.pulumi.gcp.diagflow.kotlin.AgentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.diagflow.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.diagflow.AgentArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A Dialogflow agent is a virtual agent that handles conversations with your end-users. It is a natural language
* understanding module that understands the nuances of human language. Dialogflow translates end-user text or audio
* during a conversation to structured data that your apps and services can understand. You design and build a Dialogflow
* agent to handle the types of conversations required for your system.
* To get more information about Agent, see:
* * [API documentation](https://cloud.google.com/dialogflow/docs/reference/rest/v2/projects/agent)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/dialogflow/docs/)
* ## Example Usage
* ### Dialogflow Agent Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const fullAgent = new gcp.diagflow.Agent("full_agent", {
* displayName: "dialogflow-agent",
* defaultLanguageCode: "en",
* supportedLanguageCodes: [
* "fr",
* "de",
* "es",
* ],
* timeZone: "America/New_York",
* description: "Example description.",
* avatarUri: "https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* enableLogging: true,
* matchMode: "MATCH_MODE_ML_ONLY",
* classificationThreshold: 0.3,
* apiVersion: "API_VERSION_V2_BETA_1",
* tier: "TIER_STANDARD",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* full_agent = gcp.diagflow.Agent("full_agent",
* display_name="dialogflow-agent",
* default_language_code="en",
* supported_language_codes=[
* "fr",
* "de",
* "es",
* ],
* time_zone="America/New_York",
* description="Example description.",
* avatar_uri="https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* enable_logging=True,
* match_mode="MATCH_MODE_ML_ONLY",
* classification_threshold=0.3,
* api_version="API_VERSION_V2_BETA_1",
* tier="TIER_STANDARD")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var fullAgent = new Gcp.Diagflow.Agent("full_agent", new()
* {
* DisplayName = "dialogflow-agent",
* DefaultLanguageCode = "en",
* SupportedLanguageCodes = new[]
* {
* "fr",
* "de",
* "es",
* },
* TimeZone = "America/New_York",
* Description = "Example description.",
* AvatarUri = "https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* EnableLogging = true,
* MatchMode = "MATCH_MODE_ML_ONLY",
* ClassificationThreshold = 0.3,
* ApiVersion = "API_VERSION_V2_BETA_1",
* Tier = "TIER_STANDARD",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/diagflow"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := diagflow.NewAgent(ctx, "full_agent", &diagflow.AgentArgs{
* DisplayName: pulumi.String("dialogflow-agent"),
* DefaultLanguageCode: pulumi.String("en"),
* SupportedLanguageCodes: pulumi.StringArray{
* pulumi.String("fr"),
* pulumi.String("de"),
* pulumi.String("es"),
* },
* TimeZone: pulumi.String("America/New_York"),
* Description: pulumi.String("Example description."),
* AvatarUri: pulumi.String("https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png"),
* EnableLogging: pulumi.Bool(true),
* MatchMode: pulumi.String("MATCH_MODE_ML_ONLY"),
* ClassificationThreshold: pulumi.Float64(0.3),
* ApiVersion: pulumi.String("API_VERSION_V2_BETA_1"),
* Tier: pulumi.String("TIER_STANDARD"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.diagflow.Agent;
* import com.pulumi.gcp.diagflow.AgentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var fullAgent = new Agent("fullAgent", AgentArgs.builder()
* .displayName("dialogflow-agent")
* .defaultLanguageCode("en")
* .supportedLanguageCodes(
* "fr",
* "de",
* "es")
* .timeZone("America/New_York")
* .description("Example description.")
* .avatarUri("https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png")
* .enableLogging(true)
* .matchMode("MATCH_MODE_ML_ONLY")
* .classificationThreshold(0.3)
* .apiVersion("API_VERSION_V2_BETA_1")
* .tier("TIER_STANDARD")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* fullAgent:
* type: gcp:diagflow:Agent
* name: full_agent
* properties:
* displayName: dialogflow-agent
* defaultLanguageCode: en
* supportedLanguageCodes:
* - fr
* - de
* - es
* timeZone: America/New_York
* description: Example description.
* avatarUri: https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png
* enableLogging: true
* matchMode: MATCH_MODE_ML_ONLY
* classificationThreshold: 0.3
* apiVersion: API_VERSION_V2_BETA_1
* tier: TIER_STANDARD
* ```
*
* ## Import
* Agent can be imported using any of these accepted formats:
* * `{{project}}`
* When using the `pulumi import` command, Agent can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:diagflow/agent:Agent default {{project}}
* ```
* @property apiVersion API version displayed in Dialogflow console. If not specified, V2 API is assumed. Clients are free to query
* different service endpoints for different API versions. However, bots connectors and webhook calls will follow
* the specified API version.
* * API_VERSION_V1: Legacy V1 API.
* * API_VERSION_V2: V2 API.
* * API_VERSION_V2_BETA_1: V2beta1 API.
* Possible values are: `API_VERSION_V1`, `API_VERSION_V2`, `API_VERSION_V2_BETA_1`.
* @property avatarUri The URI of the agent's avatar, which are used throughout the Dialogflow console. When an image URL is entered
* into this field, the Dialogflow will save the image in the backend. The address of the backend image returned
* from the API will be shown in the [avatarUriBackend] field.
* @property classificationThreshold To filter out false positive results and still get variety in matched natural language inputs for your agent,
* you can tune the machine learning classification threshold. If the returned score value is less than the threshold
* value, then a fallback intent will be triggered or, if there are no fallback intents defined, no intent will be
* triggered. The score values range from 0.0 (completely uncertain) to 1.0 (completely certain). If set to 0.0, the
* default of 0.3 is used.
* @property defaultLanguageCode The default language of the agent as a language tag. [See Language Support](https://cloud.google.com/dialogflow/docs/reference/language)
* for a list of the currently supported language codes. This field cannot be updated after creation.
* @property description The description of this agent. The maximum length is 500 characters. If exceeded, the request is rejected.
* @property displayName The name of this agent.
* @property enableLogging Determines whether this agent should log conversation queries.
* @property matchMode Determines how intents are detected from user queries.
* * MATCH_MODE_HYBRID: Best for agents with a small number of examples in intents and/or wide use of templates
* syntax and composite entities.
* * MATCH_MODE_ML_ONLY: Can be used for agents with a large number of examples in intents, especially the ones
* using @sys.any or very large developer entities.
* Possible values are: `MATCH_MODE_HYBRID`, `MATCH_MODE_ML_ONLY`.
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property supportedLanguageCodes The list of all languages supported by this agent (except for the defaultLanguageCode).
* @property tier The agent tier. If not specified, TIER_STANDARD is assumed.
* * TIER_STANDARD: Standard tier.
* * TIER_ENTERPRISE: Enterprise tier (Essentials).
* * TIER_ENTERPRISE_PLUS: Enterprise tier (Plus).
* NOTE: Due to consistency issues, the provider will not read this field from the API. Drift is possible between
* the the provider state and Dialogflow if the agent tier is changed outside of the provider.
* @property timeZone The time zone of this agent from the [time zone database](https://www.iana.org/time-zones), e.g., America/New_York,
* Europe/Paris.
* - - -
*/
public data class AgentArgs(
public val apiVersion: Output? = null,
public val avatarUri: Output? = null,
public val classificationThreshold: Output? = null,
public val defaultLanguageCode: Output? = null,
public val description: Output? = null,
public val displayName: Output? = null,
public val enableLogging: Output? = null,
public val matchMode: Output? = null,
public val project: Output? = null,
public val supportedLanguageCodes: Output>? = null,
public val tier: Output? = null,
public val timeZone: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.diagflow.AgentArgs =
com.pulumi.gcp.diagflow.AgentArgs.builder()
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.avatarUri(avatarUri?.applyValue({ args0 -> args0 }))
.classificationThreshold(classificationThreshold?.applyValue({ args0 -> args0 }))
.defaultLanguageCode(defaultLanguageCode?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.enableLogging(enableLogging?.applyValue({ args0 -> args0 }))
.matchMode(matchMode?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.supportedLanguageCodes(
supportedLanguageCodes?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
)
.tier(tier?.applyValue({ args0 -> args0 }))
.timeZone(timeZone?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AgentArgs].
*/
@PulumiTagMarker
public class AgentArgsBuilder internal constructor() {
private var apiVersion: Output? = null
private var avatarUri: Output? = null
private var classificationThreshold: Output? = null
private var defaultLanguageCode: Output? = null
private var description: Output? = null
private var displayName: Output? = null
private var enableLogging: Output? = null
private var matchMode: Output? = null
private var project: Output? = null
private var supportedLanguageCodes: Output>? = null
private var tier: Output? = null
private var timeZone: Output? = null
/**
* @param value API version displayed in Dialogflow console. If not specified, V2 API is assumed. Clients are free to query
* different service endpoints for different API versions. However, bots connectors and webhook calls will follow
* the specified API version.
* * API_VERSION_V1: Legacy V1 API.
* * API_VERSION_V2: V2 API.
* * API_VERSION_V2_BETA_1: V2beta1 API.
* Possible values are: `API_VERSION_V1`, `API_VERSION_V2`, `API_VERSION_V2_BETA_1`.
*/
@JvmName("irylgxfwqqyqvvsj")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value The URI of the agent's avatar, which are used throughout the Dialogflow console. When an image URL is entered
* into this field, the Dialogflow will save the image in the backend. The address of the backend image returned
* from the API will be shown in the [avatarUriBackend] field.
*/
@JvmName("kmoekmfhtpbkwdhs")
public suspend fun avatarUri(`value`: Output) {
this.avatarUri = value
}
/**
* @param value To filter out false positive results and still get variety in matched natural language inputs for your agent,
* you can tune the machine learning classification threshold. If the returned score value is less than the threshold
* value, then a fallback intent will be triggered or, if there are no fallback intents defined, no intent will be
* triggered. The score values range from 0.0 (completely uncertain) to 1.0 (completely certain). If set to 0.0, the
* default of 0.3 is used.
*/
@JvmName("eaqycuukjqvupvgp")
public suspend fun classificationThreshold(`value`: Output) {
this.classificationThreshold = value
}
/**
* @param value The default language of the agent as a language tag. [See Language Support](https://cloud.google.com/dialogflow/docs/reference/language)
* for a list of the currently supported language codes. This field cannot be updated after creation.
*/
@JvmName("pectbcfdddraaskk")
public suspend fun defaultLanguageCode(`value`: Output) {
this.defaultLanguageCode = value
}
/**
* @param value The description of this agent. The maximum length is 500 characters. If exceeded, the request is rejected.
*/
@JvmName("bihmdkdbrrgksafe")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The name of this agent.
*/
@JvmName("gxextwlyqeyqossi")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value Determines whether this agent should log conversation queries.
*/
@JvmName("xpwhywyujjpfotlb")
public suspend fun enableLogging(`value`: Output) {
this.enableLogging = value
}
/**
* @param value Determines how intents are detected from user queries.
* * MATCH_MODE_HYBRID: Best for agents with a small number of examples in intents and/or wide use of templates
* syntax and composite entities.
* * MATCH_MODE_ML_ONLY: Can be used for agents with a large number of examples in intents, especially the ones
* using @sys.any or very large developer entities.
* Possible values are: `MATCH_MODE_HYBRID`, `MATCH_MODE_ML_ONLY`.
*/
@JvmName("orskqmicaeeoqbfw")
public suspend fun matchMode(`value`: Output) {
this.matchMode = value
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("odgkjtduxhyfmgvk")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The list of all languages supported by this agent (except for the defaultLanguageCode).
*/
@JvmName("xcuayeuoyrpfwcjt")
public suspend fun supportedLanguageCodes(`value`: Output>) {
this.supportedLanguageCodes = value
}
@JvmName("lcwtxxtdkkimrwog")
public suspend fun supportedLanguageCodes(vararg values: Output) {
this.supportedLanguageCodes = Output.all(values.asList())
}
/**
* @param values The list of all languages supported by this agent (except for the defaultLanguageCode).
*/
@JvmName("aonsmelyivwtgxis")
public suspend fun supportedLanguageCodes(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy