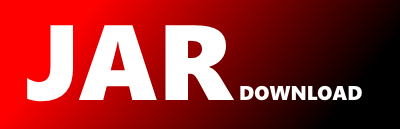
com.pulumi.gcp.diagflow.kotlin.CxFlowArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.diagflow.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.diagflow.CxFlowArgs.builder
import com.pulumi.gcp.diagflow.kotlin.inputs.CxFlowAdvancedSettingsArgs
import com.pulumi.gcp.diagflow.kotlin.inputs.CxFlowAdvancedSettingsArgsBuilder
import com.pulumi.gcp.diagflow.kotlin.inputs.CxFlowEventHandlerArgs
import com.pulumi.gcp.diagflow.kotlin.inputs.CxFlowEventHandlerArgsBuilder
import com.pulumi.gcp.diagflow.kotlin.inputs.CxFlowNluSettingsArgs
import com.pulumi.gcp.diagflow.kotlin.inputs.CxFlowNluSettingsArgsBuilder
import com.pulumi.gcp.diagflow.kotlin.inputs.CxFlowTransitionRouteArgs
import com.pulumi.gcp.diagflow.kotlin.inputs.CxFlowTransitionRouteArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Flows represents the conversation flows when you build your chatbot agent.
* To get more information about Flow, see:
* * [API documentation](https://cloud.google.com/dialogflow/cx/docs/reference/rest/v3/projects.locations.agents.flows)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/dialogflow/cx/docs)
* ## Example Usage
* ### Dialogflowcx Flow Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const agent = new gcp.diagflow.CxAgent("agent", {
* displayName: "dialogflowcx-agent",
* location: "global",
* defaultLanguageCode: "en",
* supportedLanguageCodes: [
* "fr",
* "de",
* "es",
* ],
* timeZone: "America/New_York",
* description: "Example description.",
* avatarUri: "https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* enableStackdriverLogging: true,
* enableSpellCorrection: true,
* speechToTextSettings: {
* enableSpeechAdaptation: true,
* },
* });
* const basicFlow = new gcp.diagflow.CxFlow("basic_flow", {
* parent: agent.id,
* displayName: "MyFlow",
* description: "Test Flow",
* nluSettings: {
* classificationThreshold: 0.3,
* modelType: "MODEL_TYPE_STANDARD",
* },
* eventHandlers: [
* {
* event: "custom-event",
* triggerFulfillment: {
* returnPartialResponses: false,
* messages: [{
* text: {
* texts: ["I didn't get that. Can you say it again?"],
* },
* }],
* },
* },
* {
* event: "sys.no-match-default",
* triggerFulfillment: {
* returnPartialResponses: false,
* messages: [{
* text: {
* texts: ["Sorry, could you say that again?"],
* },
* }],
* },
* },
* {
* event: "sys.no-input-default",
* triggerFulfillment: {
* returnPartialResponses: false,
* messages: [{
* text: {
* texts: ["One more time?"],
* },
* }],
* },
* },
* ],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* agent = gcp.diagflow.CxAgent("agent",
* display_name="dialogflowcx-agent",
* location="global",
* default_language_code="en",
* supported_language_codes=[
* "fr",
* "de",
* "es",
* ],
* time_zone="America/New_York",
* description="Example description.",
* avatar_uri="https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* enable_stackdriver_logging=True,
* enable_spell_correction=True,
* speech_to_text_settings=gcp.diagflow.CxAgentSpeechToTextSettingsArgs(
* enable_speech_adaptation=True,
* ))
* basic_flow = gcp.diagflow.CxFlow("basic_flow",
* parent=agent.id,
* display_name="MyFlow",
* description="Test Flow",
* nlu_settings=gcp.diagflow.CxFlowNluSettingsArgs(
* classification_threshold=0.3,
* model_type="MODEL_TYPE_STANDARD",
* ),
* event_handlers=[
* gcp.diagflow.CxFlowEventHandlerArgs(
* event="custom-event",
* trigger_fulfillment=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentArgs(
* return_partial_responses=False,
* messages=[gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* text=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs(
* texts=["I didn't get that. Can you say it again?"],
* ),
* )],
* ),
* ),
* gcp.diagflow.CxFlowEventHandlerArgs(
* event="sys.no-match-default",
* trigger_fulfillment=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentArgs(
* return_partial_responses=False,
* messages=[gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* text=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs(
* texts=["Sorry, could you say that again?"],
* ),
* )],
* ),
* ),
* gcp.diagflow.CxFlowEventHandlerArgs(
* event="sys.no-input-default",
* trigger_fulfillment=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentArgs(
* return_partial_responses=False,
* messages=[gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* text=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs(
* texts=["One more time?"],
* ),
* )],
* ),
* ),
* ])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var agent = new Gcp.Diagflow.CxAgent("agent", new()
* {
* DisplayName = "dialogflowcx-agent",
* Location = "global",
* DefaultLanguageCode = "en",
* SupportedLanguageCodes = new[]
* {
* "fr",
* "de",
* "es",
* },
* TimeZone = "America/New_York",
* Description = "Example description.",
* AvatarUri = "https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* EnableStackdriverLogging = true,
* EnableSpellCorrection = true,
* SpeechToTextSettings = new Gcp.Diagflow.Inputs.CxAgentSpeechToTextSettingsArgs
* {
* EnableSpeechAdaptation = true,
* },
* });
* var basicFlow = new Gcp.Diagflow.CxFlow("basic_flow", new()
* {
* Parent = agent.Id,
* DisplayName = "MyFlow",
* Description = "Test Flow",
* NluSettings = new Gcp.Diagflow.Inputs.CxFlowNluSettingsArgs
* {
* ClassificationThreshold = 0.3,
* ModelType = "MODEL_TYPE_STANDARD",
* },
* EventHandlers = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerArgs
* {
* Event = "custom-event",
* TriggerFulfillment = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentArgs
* {
* ReturnPartialResponses = false,
* Messages = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* Text = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs
* {
* Texts = new[]
* {
* "I didn't get that. Can you say it again?",
* },
* },
* },
* },
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerArgs
* {
* Event = "sys.no-match-default",
* TriggerFulfillment = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentArgs
* {
* ReturnPartialResponses = false,
* Messages = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* Text = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs
* {
* Texts = new[]
* {
* "Sorry, could you say that again?",
* },
* },
* },
* },
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerArgs
* {
* Event = "sys.no-input-default",
* TriggerFulfillment = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentArgs
* {
* ReturnPartialResponses = false,
* Messages = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* Text = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs
* {
* Texts = new[]
* {
* "One more time?",
* },
* },
* },
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/diagflow"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* agent, err := diagflow.NewCxAgent(ctx, "agent", &diagflow.CxAgentArgs{
* DisplayName: pulumi.String("dialogflowcx-agent"),
* Location: pulumi.String("global"),
* DefaultLanguageCode: pulumi.String("en"),
* SupportedLanguageCodes: pulumi.StringArray{
* pulumi.String("fr"),
* pulumi.String("de"),
* pulumi.String("es"),
* },
* TimeZone: pulumi.String("America/New_York"),
* Description: pulumi.String("Example description."),
* AvatarUri: pulumi.String("https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png"),
* EnableStackdriverLogging: pulumi.Bool(true),
* EnableSpellCorrection: pulumi.Bool(true),
* SpeechToTextSettings: &diagflow.CxAgentSpeechToTextSettingsArgs{
* EnableSpeechAdaptation: pulumi.Bool(true),
* },
* })
* if err != nil {
* return err
* }
* _, err = diagflow.NewCxFlow(ctx, "basic_flow", &diagflow.CxFlowArgs{
* Parent: agent.ID(),
* DisplayName: pulumi.String("MyFlow"),
* Description: pulumi.String("Test Flow"),
* NluSettings: &diagflow.CxFlowNluSettingsArgs{
* ClassificationThreshold: pulumi.Float64(0.3),
* ModelType: pulumi.String("MODEL_TYPE_STANDARD"),
* },
* EventHandlers: diagflow.CxFlowEventHandlerArray{
* &diagflow.CxFlowEventHandlerArgs{
* Event: pulumi.String("custom-event"),
* TriggerFulfillment: &diagflow.CxFlowEventHandlerTriggerFulfillmentArgs{
* ReturnPartialResponses: pulumi.Bool(false),
* Messages: diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArray{
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* Text: &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs{
* Texts: pulumi.StringArray{
* pulumi.String("I didn't get that. Can you say it again?"),
* },
* },
* },
* },
* },
* },
* &diagflow.CxFlowEventHandlerArgs{
* Event: pulumi.String("sys.no-match-default"),
* TriggerFulfillment: &diagflow.CxFlowEventHandlerTriggerFulfillmentArgs{
* ReturnPartialResponses: pulumi.Bool(false),
* Messages: diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArray{
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* Text: &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs{
* Texts: pulumi.StringArray{
* pulumi.String("Sorry, could you say that again?"),
* },
* },
* },
* },
* },
* },
* &diagflow.CxFlowEventHandlerArgs{
* Event: pulumi.String("sys.no-input-default"),
* TriggerFulfillment: &diagflow.CxFlowEventHandlerTriggerFulfillmentArgs{
* ReturnPartialResponses: pulumi.Bool(false),
* Messages: diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArray{
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* Text: &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs{
* Texts: pulumi.StringArray{
* pulumi.String("One more time?"),
* },
* },
* },
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.diagflow.CxAgent;
* import com.pulumi.gcp.diagflow.CxAgentArgs;
* import com.pulumi.gcp.diagflow.inputs.CxAgentSpeechToTextSettingsArgs;
* import com.pulumi.gcp.diagflow.CxFlow;
* import com.pulumi.gcp.diagflow.CxFlowArgs;
* import com.pulumi.gcp.diagflow.inputs.CxFlowNluSettingsArgs;
* import com.pulumi.gcp.diagflow.inputs.CxFlowEventHandlerArgs;
* import com.pulumi.gcp.diagflow.inputs.CxFlowEventHandlerTriggerFulfillmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var agent = new CxAgent("agent", CxAgentArgs.builder()
* .displayName("dialogflowcx-agent")
* .location("global")
* .defaultLanguageCode("en")
* .supportedLanguageCodes(
* "fr",
* "de",
* "es")
* .timeZone("America/New_York")
* .description("Example description.")
* .avatarUri("https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png")
* .enableStackdriverLogging(true)
* .enableSpellCorrection(true)
* .speechToTextSettings(CxAgentSpeechToTextSettingsArgs.builder()
* .enableSpeechAdaptation(true)
* .build())
* .build());
* var basicFlow = new CxFlow("basicFlow", CxFlowArgs.builder()
* .parent(agent.id())
* .displayName("MyFlow")
* .description("Test Flow")
* .nluSettings(CxFlowNluSettingsArgs.builder()
* .classificationThreshold(0.3)
* .modelType("MODEL_TYPE_STANDARD")
* .build())
* .eventHandlers(
* CxFlowEventHandlerArgs.builder()
* .event("custom-event")
* .triggerFulfillment(CxFlowEventHandlerTriggerFulfillmentArgs.builder()
* .returnPartialResponses(false)
* .messages(CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .text(CxFlowEventHandlerTriggerFulfillmentMessageTextArgs.builder()
* .texts("I didn't get that. Can you say it again?")
* .build())
* .build())
* .build())
* .build(),
* CxFlowEventHandlerArgs.builder()
* .event("sys.no-match-default")
* .triggerFulfillment(CxFlowEventHandlerTriggerFulfillmentArgs.builder()
* .returnPartialResponses(false)
* .messages(CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .text(CxFlowEventHandlerTriggerFulfillmentMessageTextArgs.builder()
* .texts("Sorry, could you say that again?")
* .build())
* .build())
* .build())
* .build(),
* CxFlowEventHandlerArgs.builder()
* .event("sys.no-input-default")
* .triggerFulfillment(CxFlowEventHandlerTriggerFulfillmentArgs.builder()
* .returnPartialResponses(false)
* .messages(CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .text(CxFlowEventHandlerTriggerFulfillmentMessageTextArgs.builder()
* .texts("One more time?")
* .build())
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* agent:
* type: gcp:diagflow:CxAgent
* properties:
* displayName: dialogflowcx-agent
* location: global
* defaultLanguageCode: en
* supportedLanguageCodes:
* - fr
* - de
* - es
* timeZone: America/New_York
* description: Example description.
* avatarUri: https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png
* enableStackdriverLogging: true
* enableSpellCorrection: true
* speechToTextSettings:
* enableSpeechAdaptation: true
* basicFlow:
* type: gcp:diagflow:CxFlow
* name: basic_flow
* properties:
* parent: ${agent.id}
* displayName: MyFlow
* description: Test Flow
* nluSettings:
* classificationThreshold: 0.3
* modelType: MODEL_TYPE_STANDARD
* eventHandlers:
* - event: custom-event
* triggerFulfillment:
* returnPartialResponses: false
* messages:
* - text:
* texts:
* - I didn't get that. Can you say it again?
* - event: sys.no-match-default
* triggerFulfillment:
* returnPartialResponses: false
* messages:
* - text:
* texts:
* - Sorry, could you say that again?
* - event: sys.no-input-default
* triggerFulfillment:
* returnPartialResponses: false
* messages:
* - text:
* texts:
* - One more time?
* ```
*
* ### Dialogflowcx Flow Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const agent = new gcp.diagflow.CxAgent("agent", {
* displayName: "dialogflowcx-agent",
* location: "global",
* defaultLanguageCode: "en",
* supportedLanguageCodes: [
* "fr",
* "de",
* "es",
* ],
* timeZone: "America/New_York",
* description: "Example description.",
* avatarUri: "https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* enableStackdriverLogging: true,
* enableSpellCorrection: true,
* speechToTextSettings: {
* enableSpeechAdaptation: true,
* },
* });
* const bucket = new gcp.storage.Bucket("bucket", {
* name: "dialogflowcx-bucket",
* location: "US",
* uniformBucketLevelAccess: true,
* });
* const basicFlow = new gcp.diagflow.CxFlow("basic_flow", {
* parent: agent.id,
* displayName: "MyFlow",
* description: "Test Flow",
* nluSettings: {
* classificationThreshold: 0.3,
* modelType: "MODEL_TYPE_STANDARD",
* },
* eventHandlers: [
* {
* event: "custom-event",
* triggerFulfillment: {
* returnPartialResponses: false,
* messages: [{
* text: {
* texts: ["I didn't get that. Can you say it again?"],
* },
* }],
* },
* },
* {
* event: "sys.no-match-default",
* triggerFulfillment: {
* returnPartialResponses: false,
* messages: [{
* text: {
* texts: ["Sorry, could you say that again?"],
* },
* }],
* },
* },
* {
* event: "sys.no-input-default",
* triggerFulfillment: {
* returnPartialResponses: false,
* messages: [{
* text: {
* texts: ["One more time?"],
* },
* }],
* },
* },
* {
* event: "another-event",
* triggerFulfillment: {
* returnPartialResponses: true,
* messages: [
* {
* channel: "some-channel",
* text: {
* texts: ["Some text"],
* },
* },
* {
* payload: " {\"some-key\": \"some-value\", \"other-key\": [\"other-value\"]}\n",
* },
* {
* conversationSuccess: {
* metadata: " {\"some-metadata-key\": \"some-value\", \"other-metadata-key\": 1234}\n",
* },
* },
* {
* outputAudioText: {
* text: "some output text",
* },
* },
* {
* outputAudioText: {
* ssml: " Some example SSML XML \n",
* },
* },
* {
* liveAgentHandoff: {
* metadata: " {\"some-metadata-key\": \"some-value\", \"other-metadata-key\": 1234}\n",
* },
* },
* {
* playAudio: {
* audioUri: "http://example.com/some-audio-file.mp3",
* },
* },
* {
* telephonyTransferCall: {
* phoneNumber: "1-234-567-8901",
* },
* },
* ],
* setParameterActions: [
* {
* parameter: "some-param",
* value: "123.45",
* },
* {
* parameter: "another-param",
* value: JSON.stringify("abc"),
* },
* {
* parameter: "other-param",
* value: JSON.stringify(["foo"]),
* },
* ],
* conditionalCases: [{
* cases: JSON.stringify([
* {
* condition: "$sys.func.RAND() < 0.5",
* caseContent: [
* {
* message: {
* text: {
* text: ["First case"],
* },
* },
* },
* {
* additionalCases: {
* cases: [{
* condition: "$sys.func.RAND() < 0.2",
* caseContent: [{
* message: {
* text: {
* text: ["Nested case"],
* },
* },
* }],
* }],
* },
* },
* ],
* },
* {
* caseContent: [{
* message: {
* text: {
* text: ["Final case"],
* },
* },
* }],
* },
* ]),
* }],
* },
* },
* ],
* transitionRoutes: [{
* condition: "true",
* triggerFulfillment: {
* returnPartialResponses: true,
* messages: [
* {
* channel: "some-channel",
* text: {
* texts: ["Some text"],
* },
* },
* {
* payload: " {\"some-key\": \"some-value\", \"other-key\": [\"other-value\"]}\n",
* },
* {
* conversationSuccess: {
* metadata: " {\"some-metadata-key\": \"some-value\", \"other-metadata-key\": 1234}\n",
* },
* },
* {
* outputAudioText: {
* text: "some output text",
* },
* },
* {
* outputAudioText: {
* ssml: " Some example SSML XML \n",
* },
* },
* {
* liveAgentHandoff: {
* metadata: " {\"some-metadata-key\": \"some-value\", \"other-metadata-key\": 1234}\n",
* },
* },
* {
* playAudio: {
* audioUri: "http://example.com/some-audio-file.mp3",
* },
* },
* {
* telephonyTransferCall: {
* phoneNumber: "1-234-567-8901",
* },
* },
* ],
* setParameterActions: [
* {
* parameter: "some-param",
* value: "123.45",
* },
* {
* parameter: "another-param",
* value: JSON.stringify("abc"),
* },
* {
* parameter: "other-param",
* value: JSON.stringify(["foo"]),
* },
* ],
* conditionalCases: [{
* cases: JSON.stringify([
* {
* condition: "$sys.func.RAND() < 0.5",
* caseContent: [
* {
* message: {
* text: {
* text: ["First case"],
* },
* },
* },
* {
* additionalCases: {
* cases: [{
* condition: "$sys.func.RAND() < 0.2",
* caseContent: [{
* message: {
* text: {
* text: ["Nested case"],
* },
* },
* }],
* }],
* },
* },
* ],
* },
* {
* caseContent: [{
* message: {
* text: {
* text: ["Final case"],
* },
* },
* }],
* },
* ]),
* }],
* },
* targetFlow: agent.startFlow,
* }],
* advancedSettings: {
* audioExportGcsDestination: {
* uri: pulumi.interpolate`${bucket.url}/prefix-`,
* },
* dtmfSettings: {
* enabled: true,
* maxDigits: 1,
* finishDigit: "#",
* },
* },
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_gcp as gcp
* agent = gcp.diagflow.CxAgent("agent",
* display_name="dialogflowcx-agent",
* location="global",
* default_language_code="en",
* supported_language_codes=[
* "fr",
* "de",
* "es",
* ],
* time_zone="America/New_York",
* description="Example description.",
* avatar_uri="https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* enable_stackdriver_logging=True,
* enable_spell_correction=True,
* speech_to_text_settings=gcp.diagflow.CxAgentSpeechToTextSettingsArgs(
* enable_speech_adaptation=True,
* ))
* bucket = gcp.storage.Bucket("bucket",
* name="dialogflowcx-bucket",
* location="US",
* uniform_bucket_level_access=True)
* basic_flow = gcp.diagflow.CxFlow("basic_flow",
* parent=agent.id,
* display_name="MyFlow",
* description="Test Flow",
* nlu_settings=gcp.diagflow.CxFlowNluSettingsArgs(
* classification_threshold=0.3,
* model_type="MODEL_TYPE_STANDARD",
* ),
* event_handlers=[
* gcp.diagflow.CxFlowEventHandlerArgs(
* event="custom-event",
* trigger_fulfillment=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentArgs(
* return_partial_responses=False,
* messages=[gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* text=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs(
* texts=["I didn't get that. Can you say it again?"],
* ),
* )],
* ),
* ),
* gcp.diagflow.CxFlowEventHandlerArgs(
* event="sys.no-match-default",
* trigger_fulfillment=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentArgs(
* return_partial_responses=False,
* messages=[gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* text=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs(
* texts=["Sorry, could you say that again?"],
* ),
* )],
* ),
* ),
* gcp.diagflow.CxFlowEventHandlerArgs(
* event="sys.no-input-default",
* trigger_fulfillment=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentArgs(
* return_partial_responses=False,
* messages=[gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* text=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs(
* texts=["One more time?"],
* ),
* )],
* ),
* ),
* gcp.diagflow.CxFlowEventHandlerArgs(
* event="another-event",
* trigger_fulfillment=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentArgs(
* return_partial_responses=True,
* messages=[
* gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* channel="some-channel",
* text=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs(
* texts=["Some text"],
* ),
* ),
* gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* payload=" {\"some-key\": \"some-value\", \"other-key\": [\"other-value\"]}\n",
* ),
* gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* conversation_success=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageConversationSuccessArgs(
* metadata=" {\"some-metadata-key\": \"some-value\", \"other-metadata-key\": 1234}\n",
* ),
* ),
* gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* output_audio_text=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageOutputAudioTextArgs(
* text="some output text",
* ),
* ),
* gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* output_audio_text=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageOutputAudioTextArgs(
* ssml=" Some example SSML XML \n",
* ),
* ),
* gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* live_agent_handoff=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageLiveAgentHandoffArgs(
* metadata=" {\"some-metadata-key\": \"some-value\", \"other-metadata-key\": 1234}\n",
* ),
* ),
* gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* play_audio=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessagePlayAudioArgs(
* audio_uri="http://example.com/some-audio-file.mp3",
* ),
* ),
* gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs(
* telephony_transfer_call=gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTelephonyTransferCallArgs(
* phone_number="1-234-567-8901",
* ),
* ),
* ],
* set_parameter_actions=[
* gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentSetParameterActionArgs(
* parameter="some-param",
* value="123.45",
* ),
* gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentSetParameterActionArgs(
* parameter="another-param",
* value=json.dumps("abc"),
* ),
* gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentSetParameterActionArgs(
* parameter="other-param",
* value=json.dumps(["foo"]),
* ),
* ],
* conditional_cases=[gcp.diagflow.CxFlowEventHandlerTriggerFulfillmentConditionalCaseArgs(
* cases=json.dumps([
* {
* "condition": "$sys.func.RAND() < 0.5",
* "caseContent": [
* {
* "message": {
* "text": {
* "text": ["First case"],
* },
* },
* },
* {
* "additionalCases": {
* "cases": [{
* "condition": "$sys.func.RAND() < 0.2",
* "caseContent": [{
* "message": {
* "text": {
* "text": ["Nested case"],
* },
* },
* }],
* }],
* },
* },
* ],
* },
* {
* "caseContent": [{
* "message": {
* "text": {
* "text": ["Final case"],
* },
* },
* }],
* },
* ]),
* )],
* ),
* ),
* ],
* transition_routes=[gcp.diagflow.CxFlowTransitionRouteArgs(
* condition="true",
* trigger_fulfillment=gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentArgs(
* return_partial_responses=True,
* messages=[
* gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs(
* channel="some-channel",
* text=gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageTextArgs(
* texts=["Some text"],
* ),
* ),
* gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs(
* payload=" {\"some-key\": \"some-value\", \"other-key\": [\"other-value\"]}\n",
* ),
* gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs(
* conversation_success=gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageConversationSuccessArgs(
* metadata=" {\"some-metadata-key\": \"some-value\", \"other-metadata-key\": 1234}\n",
* ),
* ),
* gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs(
* output_audio_text=gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageOutputAudioTextArgs(
* text="some output text",
* ),
* ),
* gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs(
* output_audio_text=gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageOutputAudioTextArgs(
* ssml=" Some example SSML XML \n",
* ),
* ),
* gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs(
* live_agent_handoff=gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageLiveAgentHandoffArgs(
* metadata=" {\"some-metadata-key\": \"some-value\", \"other-metadata-key\": 1234}\n",
* ),
* ),
* gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs(
* play_audio=gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessagePlayAudioArgs(
* audio_uri="http://example.com/some-audio-file.mp3",
* ),
* ),
* gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs(
* telephony_transfer_call=gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageTelephonyTransferCallArgs(
* phone_number="1-234-567-8901",
* ),
* ),
* ],
* set_parameter_actions=[
* gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentSetParameterActionArgs(
* parameter="some-param",
* value="123.45",
* ),
* gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentSetParameterActionArgs(
* parameter="another-param",
* value=json.dumps("abc"),
* ),
* gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentSetParameterActionArgs(
* parameter="other-param",
* value=json.dumps(["foo"]),
* ),
* ],
* conditional_cases=[gcp.diagflow.CxFlowTransitionRouteTriggerFulfillmentConditionalCaseArgs(
* cases=json.dumps([
* {
* "condition": "$sys.func.RAND() < 0.5",
* "caseContent": [
* {
* "message": {
* "text": {
* "text": ["First case"],
* },
* },
* },
* {
* "additionalCases": {
* "cases": [{
* "condition": "$sys.func.RAND() < 0.2",
* "caseContent": [{
* "message": {
* "text": {
* "text": ["Nested case"],
* },
* },
* }],
* }],
* },
* },
* ],
* },
* {
* "caseContent": [{
* "message": {
* "text": {
* "text": ["Final case"],
* },
* },
* }],
* },
* ]),
* )],
* ),
* target_flow=agent.start_flow,
* )],
* advanced_settings=gcp.diagflow.CxFlowAdvancedSettingsArgs(
* audio_export_gcs_destination=gcp.diagflow.CxFlowAdvancedSettingsAudioExportGcsDestinationArgs(
* uri=bucket.url.apply(lambda url: f"{url}/prefix-"),
* ),
* dtmf_settings=gcp.diagflow.CxFlowAdvancedSettingsDtmfSettingsArgs(
* enabled=True,
* max_digits=1,
* finish_digit="#",
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var agent = new Gcp.Diagflow.CxAgent("agent", new()
* {
* DisplayName = "dialogflowcx-agent",
* Location = "global",
* DefaultLanguageCode = "en",
* SupportedLanguageCodes = new[]
* {
* "fr",
* "de",
* "es",
* },
* TimeZone = "America/New_York",
* Description = "Example description.",
* AvatarUri = "https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* EnableStackdriverLogging = true,
* EnableSpellCorrection = true,
* SpeechToTextSettings = new Gcp.Diagflow.Inputs.CxAgentSpeechToTextSettingsArgs
* {
* EnableSpeechAdaptation = true,
* },
* });
* var bucket = new Gcp.Storage.Bucket("bucket", new()
* {
* Name = "dialogflowcx-bucket",
* Location = "US",
* UniformBucketLevelAccess = true,
* });
* var basicFlow = new Gcp.Diagflow.CxFlow("basic_flow", new()
* {
* Parent = agent.Id,
* DisplayName = "MyFlow",
* Description = "Test Flow",
* NluSettings = new Gcp.Diagflow.Inputs.CxFlowNluSettingsArgs
* {
* ClassificationThreshold = 0.3,
* ModelType = "MODEL_TYPE_STANDARD",
* },
* EventHandlers = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerArgs
* {
* Event = "custom-event",
* TriggerFulfillment = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentArgs
* {
* ReturnPartialResponses = false,
* Messages = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* Text = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs
* {
* Texts = new[]
* {
* "I didn't get that. Can you say it again?",
* },
* },
* },
* },
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerArgs
* {
* Event = "sys.no-match-default",
* TriggerFulfillment = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentArgs
* {
* ReturnPartialResponses = false,
* Messages = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* Text = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs
* {
* Texts = new[]
* {
* "Sorry, could you say that again?",
* },
* },
* },
* },
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerArgs
* {
* Event = "sys.no-input-default",
* TriggerFulfillment = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentArgs
* {
* ReturnPartialResponses = false,
* Messages = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* Text = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs
* {
* Texts = new[]
* {
* "One more time?",
* },
* },
* },
* },
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerArgs
* {
* Event = "another-event",
* TriggerFulfillment = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentArgs
* {
* ReturnPartialResponses = true,
* Messages = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* Channel = "some-channel",
* Text = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs
* {
* Texts = new[]
* {
* "Some text",
* },
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* Payload = @" {""some-key"": ""some-value"", ""other-key"": [""other-value""]}
* ",
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* ConversationSuccess = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageConversationSuccessArgs
* {
* Metadata = @" {""some-metadata-key"": ""some-value"", ""other-metadata-key"": 1234}
* ",
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* OutputAudioText = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageOutputAudioTextArgs
* {
* Text = "some output text",
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* OutputAudioText = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageOutputAudioTextArgs
* {
* Ssml = @" Some example SSML XML
* ",
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* LiveAgentHandoff = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageLiveAgentHandoffArgs
* {
* Metadata = @" {""some-metadata-key"": ""some-value"", ""other-metadata-key"": 1234}
* ",
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* PlayAudio = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessagePlayAudioArgs
* {
* AudioUri = "http://example.com/some-audio-file.mp3",
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs
* {
* TelephonyTransferCall = new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentMessageTelephonyTransferCallArgs
* {
* PhoneNumber = "1-234-567-8901",
* },
* },
* },
* SetParameterActions = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentSetParameterActionArgs
* {
* Parameter = "some-param",
* Value = "123.45",
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentSetParameterActionArgs
* {
* Parameter = "another-param",
* Value = JsonSerializer.Serialize("abc"),
* },
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentSetParameterActionArgs
* {
* Parameter = "other-param",
* Value = JsonSerializer.Serialize(new[]
* {
* "foo",
* }),
* },
* },
* ConditionalCases = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowEventHandlerTriggerFulfillmentConditionalCaseArgs
* {
* Cases = JsonSerializer.Serialize(new[]
* {
* new Dictionary
* {
* ["condition"] = "$sys.func.RAND() < 0.5",
* ["caseContent"] = new[]
* {
* new Dictionary
* {
* ["message"] = new Dictionary
* {
* ["text"] = new Dictionary
* {
* ["text"] = new[]
* {
* "First case",
* },
* },
* },
* },
* new Dictionary
* {
* ["additionalCases"] = new Dictionary
* {
* ["cases"] = new[]
* {
* new Dictionary
* {
* ["condition"] = "$sys.func.RAND() < 0.2",
* ["caseContent"] = new[]
* {
* new Dictionary
* {
* ["message"] = new Dictionary
* {
* ["text"] = new Dictionary
* {
* ["text"] = new[]
* {
* "Nested case",
* },
* },
* },
* },
* },
* },
* },
* },
* },
* },
* },
* new Dictionary
* {
* ["caseContent"] = new[]
* {
* new Dictionary
* {
* ["message"] = new Dictionary
* {
* ["text"] = new Dictionary
* {
* ["text"] = new[]
* {
* "Final case",
* },
* },
* },
* },
* },
* },
* }),
* },
* },
* },
* },
* },
* TransitionRoutes = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowTransitionRouteArgs
* {
* Condition = "true",
* TriggerFulfillment = new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentArgs
* {
* ReturnPartialResponses = true,
* Messages = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageArgs
* {
* Channel = "some-channel",
* Text = new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageTextArgs
* {
* Texts = new[]
* {
* "Some text",
* },
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageArgs
* {
* Payload = @" {""some-key"": ""some-value"", ""other-key"": [""other-value""]}
* ",
* },
* new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageArgs
* {
* ConversationSuccess = new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageConversationSuccessArgs
* {
* Metadata = @" {""some-metadata-key"": ""some-value"", ""other-metadata-key"": 1234}
* ",
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageArgs
* {
* OutputAudioText = new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageOutputAudioTextArgs
* {
* Text = "some output text",
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageArgs
* {
* OutputAudioText = new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageOutputAudioTextArgs
* {
* Ssml = @" Some example SSML XML
* ",
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageArgs
* {
* LiveAgentHandoff = new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageLiveAgentHandoffArgs
* {
* Metadata = @" {""some-metadata-key"": ""some-value"", ""other-metadata-key"": 1234}
* ",
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageArgs
* {
* PlayAudio = new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessagePlayAudioArgs
* {
* AudioUri = "http://example.com/some-audio-file.mp3",
* },
* },
* new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageArgs
* {
* TelephonyTransferCall = new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentMessageTelephonyTransferCallArgs
* {
* PhoneNumber = "1-234-567-8901",
* },
* },
* },
* SetParameterActions = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentSetParameterActionArgs
* {
* Parameter = "some-param",
* Value = "123.45",
* },
* new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentSetParameterActionArgs
* {
* Parameter = "another-param",
* Value = JsonSerializer.Serialize("abc"),
* },
* new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentSetParameterActionArgs
* {
* Parameter = "other-param",
* Value = JsonSerializer.Serialize(new[]
* {
* "foo",
* }),
* },
* },
* ConditionalCases = new[]
* {
* new Gcp.Diagflow.Inputs.CxFlowTransitionRouteTriggerFulfillmentConditionalCaseArgs
* {
* Cases = JsonSerializer.Serialize(new[]
* {
* new Dictionary
* {
* ["condition"] = "$sys.func.RAND() < 0.5",
* ["caseContent"] = new[]
* {
* new Dictionary
* {
* ["message"] = new Dictionary
* {
* ["text"] = new Dictionary
* {
* ["text"] = new[]
* {
* "First case",
* },
* },
* },
* },
* new Dictionary
* {
* ["additionalCases"] = new Dictionary
* {
* ["cases"] = new[]
* {
* new Dictionary
* {
* ["condition"] = "$sys.func.RAND() < 0.2",
* ["caseContent"] = new[]
* {
* new Dictionary
* {
* ["message"] = new Dictionary
* {
* ["text"] = new Dictionary
* {
* ["text"] = new[]
* {
* "Nested case",
* },
* },
* },
* },
* },
* },
* },
* },
* },
* },
* },
* new Dictionary
* {
* ["caseContent"] = new[]
* {
* new Dictionary
* {
* ["message"] = new Dictionary
* {
* ["text"] = new Dictionary
* {
* ["text"] = new[]
* {
* "Final case",
* },
* },
* },
* },
* },
* },
* }),
* },
* },
* },
* TargetFlow = agent.StartFlow,
* },
* },
* AdvancedSettings = new Gcp.Diagflow.Inputs.CxFlowAdvancedSettingsArgs
* {
* AudioExportGcsDestination = new Gcp.Diagflow.Inputs.CxFlowAdvancedSettingsAudioExportGcsDestinationArgs
* {
* Uri = bucket.Url.Apply(url => $"{url}/prefix-"),
* },
* DtmfSettings = new Gcp.Diagflow.Inputs.CxFlowAdvancedSettingsDtmfSettingsArgs
* {
* Enabled = true,
* MaxDigits = 1,
* FinishDigit = "#",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/diagflow"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* agent, err := diagflow.NewCxAgent(ctx, "agent", &diagflow.CxAgentArgs{
* DisplayName: pulumi.String("dialogflowcx-agent"),
* Location: pulumi.String("global"),
* DefaultLanguageCode: pulumi.String("en"),
* SupportedLanguageCodes: pulumi.StringArray{
* pulumi.String("fr"),
* pulumi.String("de"),
* pulumi.String("es"),
* },
* TimeZone: pulumi.String("America/New_York"),
* Description: pulumi.String("Example description."),
* AvatarUri: pulumi.String("https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png"),
* EnableStackdriverLogging: pulumi.Bool(true),
* EnableSpellCorrection: pulumi.Bool(true),
* SpeechToTextSettings: &diagflow.CxAgentSpeechToTextSettingsArgs{
* EnableSpeechAdaptation: pulumi.Bool(true),
* },
* })
* if err != nil {
* return err
* }
* bucket, err := storage.NewBucket(ctx, "bucket", &storage.BucketArgs{
* Name: pulumi.String("dialogflowcx-bucket"),
* Location: pulumi.String("US"),
* UniformBucketLevelAccess: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* tmpJSON0, err := json.Marshal("abc")
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* tmpJSON1, err := json.Marshal([]string{
* "foo",
* })
* if err != nil {
* return err
* }
* json1 := string(tmpJSON1)
* tmpJSON2, err := json.Marshal([]interface{}{
* map[string]interface{}{
* "condition": "$sys.func.RAND() < 0.5",
* "caseContent": []interface{}{
* map[string]interface{}{
* "message": map[string]interface{}{
* "text": map[string]interface{}{
* "text": []string{
* "First case",
* },
* },
* },
* },
* map[string]interface{}{
* "additionalCases": map[string]interface{}{
* "cases": []map[string]interface{}{
* map[string]interface{}{
* "condition": "$sys.func.RAND() < 0.2",
* "caseContent": []map[string]interface{}{
* map[string]interface{}{
* "message": map[string]interface{}{
* "text": map[string]interface{}{
* "text": []string{
* "Nested case",
* },
* },
* },
* },
* },
* },
* },
* },
* },
* },
* },
* map[string]interface{}{
* "caseContent": []map[string]interface{}{
* map[string]interface{}{
* "message": map[string]interface{}{
* "text": map[string]interface{}{
* "text": []string{
* "Final case",
* },
* },
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* json2 := string(tmpJSON2)
* tmpJSON3, err := json.Marshal("abc")
* if err != nil {
* return err
* }
* json3 := string(tmpJSON3)
* tmpJSON4, err := json.Marshal([]string{
* "foo",
* })
* if err != nil {
* return err
* }
* json4 := string(tmpJSON4)
* tmpJSON5, err := json.Marshal([]interface{}{
* map[string]interface{}{
* "condition": "$sys.func.RAND() < 0.5",
* "caseContent": []interface{}{
* map[string]interface{}{
* "message": map[string]interface{}{
* "text": map[string]interface{}{
* "text": []string{
* "First case",
* },
* },
* },
* },
* map[string]interface{}{
* "additionalCases": map[string]interface{}{
* "cases": []map[string]interface{}{
* map[string]interface{}{
* "condition": "$sys.func.RAND() < 0.2",
* "caseContent": []map[string]interface{}{
* map[string]interface{}{
* "message": map[string]interface{}{
* "text": map[string]interface{}{
* "text": []string{
* "Nested case",
* },
* },
* },
* },
* },
* },
* },
* },
* },
* },
* },
* map[string]interface{}{
* "caseContent": []map[string]interface{}{
* map[string]interface{}{
* "message": map[string]interface{}{
* "text": map[string]interface{}{
* "text": []string{
* "Final case",
* },
* },
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* json5 := string(tmpJSON5)
* _, err = diagflow.NewCxFlow(ctx, "basic_flow", &diagflow.CxFlowArgs{
* Parent: agent.ID(),
* DisplayName: pulumi.String("MyFlow"),
* Description: pulumi.String("Test Flow"),
* NluSettings: &diagflow.CxFlowNluSettingsArgs{
* ClassificationThreshold: pulumi.Float64(0.3),
* ModelType: pulumi.String("MODEL_TYPE_STANDARD"),
* },
* EventHandlers: diagflow.CxFlowEventHandlerArray{
* &diagflow.CxFlowEventHandlerArgs{
* Event: pulumi.String("custom-event"),
* TriggerFulfillment: &diagflow.CxFlowEventHandlerTriggerFulfillmentArgs{
* ReturnPartialResponses: pulumi.Bool(false),
* Messages: diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArray{
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* Text: &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs{
* Texts: pulumi.StringArray{
* pulumi.String("I didn't get that. Can you say it again?"),
* },
* },
* },
* },
* },
* },
* &diagflow.CxFlowEventHandlerArgs{
* Event: pulumi.String("sys.no-match-default"),
* TriggerFulfillment: &diagflow.CxFlowEventHandlerTriggerFulfillmentArgs{
* ReturnPartialResponses: pulumi.Bool(false),
* Messages: diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArray{
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* Text: &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs{
* Texts: pulumi.StringArray{
* pulumi.String("Sorry, could you say that again?"),
* },
* },
* },
* },
* },
* },
* &diagflow.CxFlowEventHandlerArgs{
* Event: pulumi.String("sys.no-input-default"),
* TriggerFulfillment: &diagflow.CxFlowEventHandlerTriggerFulfillmentArgs{
* ReturnPartialResponses: pulumi.Bool(false),
* Messages: diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArray{
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* Text: &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs{
* Texts: pulumi.StringArray{
* pulumi.String("One more time?"),
* },
* },
* },
* },
* },
* },
* &diagflow.CxFlowEventHandlerArgs{
* Event: pulumi.String("another-event"),
* TriggerFulfillment: &diagflow.CxFlowEventHandlerTriggerFulfillmentArgs{
* ReturnPartialResponses: pulumi.Bool(true),
* Messages: diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArray{
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* Channel: pulumi.String("some-channel"),
* Text: &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTextArgs{
* Texts: pulumi.StringArray{
* pulumi.String("Some text"),
* },
* },
* },
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* Payload: pulumi.String(" {\"some-key\": \"some-value\", \"other-key\": [\"other-value\"]}\n"),
* },
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* ConversationSuccess: &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageConversationSuccessArgs{
* Metadata: pulumi.String(" {\"some-metadata-key\": \"some-value\", \"other-metadata-key\": 1234}\n"),
* },
* },
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* OutputAudioText: &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageOutputAudioTextArgs{
* Text: pulumi.String("some output text"),
* },
* },
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* OutputAudioText: &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageOutputAudioTextArgs{
* Ssml: pulumi.String(" Some example SSML XML \n"),
* },
* },
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* LiveAgentHandoff: &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageLiveAgentHandoffArgs{
* Metadata: pulumi.String(" {\"some-metadata-key\": \"some-value\", \"other-metadata-key\": 1234}\n"),
* },
* },
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* PlayAudio: &diagflow.CxFlowEventHandlerTriggerFulfillmentMessagePlayAudioArgs{
* AudioUri: pulumi.String("http://example.com/some-audio-file.mp3"),
* },
* },
* &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageArgs{
* TelephonyTransferCall: &diagflow.CxFlowEventHandlerTriggerFulfillmentMessageTelephonyTransferCallArgs{
* PhoneNumber: pulumi.String("1-234-567-8901"),
* },
* },
* },
* SetParameterActions: diagflow.CxFlowEventHandlerTriggerFulfillmentSetParameterActionArray{
* &diagflow.CxFlowEventHandlerTriggerFulfillmentSetParameterActionArgs{
* Parameter: pulumi.String("some-param"),
* Value: pulumi.String("123.45"),
* },
* &diagflow.CxFlowEventHandlerTriggerFulfillmentSetParameterActionArgs{
* Parameter: pulumi.String("another-param"),
* Value: pulumi.String(json0),
* },
* &diagflow.CxFlowEventHandlerTriggerFulfillmentSetParameterActionArgs{
* Parameter: pulumi.String("other-param"),
* Value: pulumi.String(json1),
* },
* },
* ConditionalCases: diagflow.CxFlowEventHandlerTriggerFulfillmentConditionalCaseArray{
* &diagflow.CxFlowEventHandlerTriggerFulfillmentConditionalCaseArgs{
* Cases: pulumi.String(json2),
* },
* },
* },
* },
* },
* TransitionRoutes: diagflow.CxFlowTransitionRouteArray{
* &diagflow.CxFlowTransitionRouteArgs{
* Condition: pulumi.String("true"),
* TriggerFulfillment: &diagflow.CxFlowTransitionRouteTriggerFulfillmentArgs{
* ReturnPartialResponses: pulumi.Bool(true),
* Messages: diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArray{
* &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs{
* Channel: pulumi.String("some-channel"),
* Text: &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageTextArgs{
* Texts: pulumi.StringArray{
* pulumi.String("Some text"),
* },
* },
* },
* &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs{
* Payload: pulumi.String(" {\"some-key\": \"some-value\", \"other-key\": [\"other-value\"]}\n"),
* },
* &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs{
* ConversationSuccess: &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageConversationSuccessArgs{
* Metadata: pulumi.String(" {\"some-metadata-key\": \"some-value\", \"other-metadata-key\": 1234}\n"),
* },
* },
* &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs{
* OutputAudioText: &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageOutputAudioTextArgs{
* Text: pulumi.String("some output text"),
* },
* },
* &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs{
* OutputAudioText: &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageOutputAudioTextArgs{
* Ssml: pulumi.String(" Some example SSML XML \n"),
* },
* },
* &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs{
* LiveAgentHandoff: &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageLiveAgentHandoffArgs{
* Metadata: pulumi.String(" {\"some-metadata-key\": \"some-value\", \"other-metadata-key\": 1234}\n"),
* },
* },
* &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs{
* PlayAudio: &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessagePlayAudioArgs{
* AudioUri: pulumi.String("http://example.com/some-audio-file.mp3"),
* },
* },
* &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageArgs{
* TelephonyTransferCall: &diagflow.CxFlowTransitionRouteTriggerFulfillmentMessageTelephonyTransferCallArgs{
* PhoneNumber: pulumi.String("1-234-567-8901"),
* },
* },
* },
* SetParameterActions: diagflow.CxFlowTransitionRouteTriggerFulfillmentSetParameterActionArray{
* &diagflow.CxFlowTransitionRouteTriggerFulfillmentSetParameterActionArgs{
* Parameter: pulumi.String("some-param"),
* Value: pulumi.String("123.45"),
* },
* &diagflow.CxFlowTransitionRouteTriggerFulfillmentSetParameterActionArgs{
* Parameter: pulumi.String("another-param"),
* Value: pulumi.String(json3),
* },
* &diagflow.CxFlowTransitionRouteTriggerFulfillmentSetParameterActionArgs{
* Parameter: pulumi.String("other-param"),
* Value: pulumi.String(json4),
* },
* },
* ConditionalCases: diagflow.CxFlowTransitionRouteTriggerFulfillmentConditionalCaseArray{
* &diagflow.CxFlowTransitionRouteTriggerFulfillmentConditionalCaseArgs{
* Cases: pulumi.String(json5),
* },
* },
* },
* TargetFlow: agent.StartFlow,
* },
* },
* AdvancedSettings: &diagflow.CxFlowAdvancedSettingsArgs{
* AudioExportGcsDestination: &diagflow.CxFlowAdvancedSettingsAudioExportGcsDestinationArgs{
* Uri: bucket.Url.ApplyT(func(url string) (string, error) {
* return fmt.Sprintf("%v/prefix-", url), nil
* }).(pulumi.StringOutput),
* },
* DtmfSettings: &diagflow.CxFlowAdvancedSettingsDtmfSettingsArgs{
* Enabled: pulumi.Bool(true),
* MaxDigits: pulumi.Int(1),
* FinishDigit: pulumi.String("#"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.diagflow.CxAgent;
* import com.pulumi.gcp.diagflow.CxAgentArgs;
* import com.pulumi.gcp.diagflow.inputs.CxAgentSpeechToTextSettingsArgs;
* import com.pulumi.gcp.storage.Bucket;
* import com.pulumi.gcp.storage.BucketArgs;
* import com.pulumi.gcp.diagflow.CxFlow;
* import com.pulumi.gcp.diagflow.CxFlowArgs;
* import com.pulumi.gcp.diagflow.inputs.CxFlowNluSettingsArgs;
* import com.pulumi.gcp.diagflow.inputs.CxFlowEventHandlerArgs;
* import com.pulumi.gcp.diagflow.inputs.CxFlowEventHandlerTriggerFulfillmentArgs;
* import com.pulumi.gcp.diagflow.inputs.CxFlowTransitionRouteArgs;
* import com.pulumi.gcp.diagflow.inputs.CxFlowTransitionRouteTriggerFulfillmentArgs;
* import com.pulumi.gcp.diagflow.inputs.CxFlowAdvancedSettingsArgs;
* import com.pulumi.gcp.diagflow.inputs.CxFlowAdvancedSettingsAudioExportGcsDestinationArgs;
* import com.pulumi.gcp.diagflow.inputs.CxFlowAdvancedSettingsDtmfSettingsArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var agent = new CxAgent("agent", CxAgentArgs.builder()
* .displayName("dialogflowcx-agent")
* .location("global")
* .defaultLanguageCode("en")
* .supportedLanguageCodes(
* "fr",
* "de",
* "es")
* .timeZone("America/New_York")
* .description("Example description.")
* .avatarUri("https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png")
* .enableStackdriverLogging(true)
* .enableSpellCorrection(true)
* .speechToTextSettings(CxAgentSpeechToTextSettingsArgs.builder()
* .enableSpeechAdaptation(true)
* .build())
* .build());
* var bucket = new Bucket("bucket", BucketArgs.builder()
* .name("dialogflowcx-bucket")
* .location("US")
* .uniformBucketLevelAccess(true)
* .build());
* var basicFlow = new CxFlow("basicFlow", CxFlowArgs.builder()
* .parent(agent.id())
* .displayName("MyFlow")
* .description("Test Flow")
* .nluSettings(CxFlowNluSettingsArgs.builder()
* .classificationThreshold(0.3)
* .modelType("MODEL_TYPE_STANDARD")
* .build())
* .eventHandlers(
* CxFlowEventHandlerArgs.builder()
* .event("custom-event")
* .triggerFulfillment(CxFlowEventHandlerTriggerFulfillmentArgs.builder()
* .returnPartialResponses(false)
* .messages(CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .text(CxFlowEventHandlerTriggerFulfillmentMessageTextArgs.builder()
* .texts("I didn't get that. Can you say it again?")
* .build())
* .build())
* .build())
* .build(),
* CxFlowEventHandlerArgs.builder()
* .event("sys.no-match-default")
* .triggerFulfillment(CxFlowEventHandlerTriggerFulfillmentArgs.builder()
* .returnPartialResponses(false)
* .messages(CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .text(CxFlowEventHandlerTriggerFulfillmentMessageTextArgs.builder()
* .texts("Sorry, could you say that again?")
* .build())
* .build())
* .build())
* .build(),
* CxFlowEventHandlerArgs.builder()
* .event("sys.no-input-default")
* .triggerFulfillment(CxFlowEventHandlerTriggerFulfillmentArgs.builder()
* .returnPartialResponses(false)
* .messages(CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .text(CxFlowEventHandlerTriggerFulfillmentMessageTextArgs.builder()
* .texts("One more time?")
* .build())
* .build())
* .build())
* .build(),
* CxFlowEventHandlerArgs.builder()
* .event("another-event")
* .triggerFulfillment(CxFlowEventHandlerTriggerFulfillmentArgs.builder()
* .returnPartialResponses(true)
* .messages(
* CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .channel("some-channel")
* .text(CxFlowEventHandlerTriggerFulfillmentMessageTextArgs.builder()
* .texts("Some text")
* .build())
* .build(),
* CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .payload("""
* {"some-key": "some-value", "other-key": ["other-value"]}
* """)
* .build(),
* CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .conversationSuccess(CxFlowEventHandlerTriggerFulfillmentMessageConversationSuccessArgs.builder()
* .metadata("""
* {"some-metadata-key": "some-value", "other-metadata-key": 1234}
* """)
* .build())
* .build(),
* CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .outputAudioText(CxFlowEventHandlerTriggerFulfillmentMessageOutputAudioTextArgs.builder()
* .text("some output text")
* .build())
* .build(),
* CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .outputAudioText(CxFlowEventHandlerTriggerFulfillmentMessageOutputAudioTextArgs.builder()
* .ssml("""
* Some example SSML XML
* """)
* .build())
* .build(),
* CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .liveAgentHandoff(CxFlowEventHandlerTriggerFulfillmentMessageLiveAgentHandoffArgs.builder()
* .metadata("""
* {"some-metadata-key": "some-value", "other-metadata-key": 1234}
* """)
* .build())
* .build(),
* CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .playAudio(CxFlowEventHandlerTriggerFulfillmentMessagePlayAudioArgs.builder()
* .audioUri("http://example.com/some-audio-file.mp3")
* .build())
* .build(),
* CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
* .telephonyTransferCall(CxFlowEventHandlerTriggerFulfillmentMessageTelephonyTransferCallArgs.builder()
* .phoneNumber("1-234-567-8901")
* .build())
* .build())
* .setParameterActions(
* CxFlowEventHandlerTriggerFulfillmentSetParameterActionArgs.builder()
* .parameter("some-param")
* .value("123.45")
* .build(),
* CxFlowEventHandlerTriggerFulfillmentSetParameterActionArgs.builder()
* .parameter("another-param")
* .value(serializeJson(
* "abc"))
* .build(),
* CxFlowEventHandlerTriggerFulfillmentSetParameterActionArgs.builder()
* .parameter("other-param")
* .value(serializeJson(
* jsonArray("foo")))
* .build())
* .conditionalCases(CxFlowEventHandlerTriggerFulfillmentConditionalCaseArgs.builder()
* .cases(serializeJson(
* jsonArray(
* jsonObject(
* jsonProperty("condition", "$sys.func.RAND() < 0.5"),
* jsonProperty("caseContent", jsonArray(
* jsonObject(
* jsonProperty("message", jsonObject(
* jsonProperty("text", jsonObject(
* jsonProperty("text", jsonArray("First case"))
* ))
* ))
* ),
* jsonObject(
* jsonProperty("additionalCases", jsonObject(
* jsonProperty("cases", jsonArray(jsonObject(
* jsonProperty("condition", "$sys.func.RAND() < 0.2"),
* jsonProperty("caseContent", jsonArray(jsonObject(
* jsonProperty("message", jsonObject(
* jsonProperty("text", jsonObject(
* jsonProperty("text", jsonArray("Nested case"))
* ))
* ))
* )))
* )))
* ))
* )
* ))
* ),
* jsonObject(
* jsonProperty("caseContent", jsonArray(jsonObject(
* jsonProperty("message", jsonObject(
* jsonProperty("text", jsonObject(
* jsonProperty("text", jsonArray("Final case"))
* ))
* ))
* )))
* )
* )))
* .build())
* .build())
* .build())
* .transitionRoutes(CxFlowTransitionRouteArgs.builder()
* .condition("true")
* .triggerFulfillment(CxFlowTransitionRouteTriggerFulfillmentArgs.builder()
* .returnPartialResponses(true)
* .messages(
* CxFlowTransitionRouteTriggerFulfillmentMessageArgs.builder()
* .channel("some-channel")
* .text(CxFlowTransitionRouteTriggerFulfillmentMessageTextArgs.builder()
* .texts("Some text")
* .build())
* .build(),
* CxFlowTransitionRouteTriggerFulfillmentMessageArgs.builder()
* .payload("""
* {"some-key": "some-value", "other-key": ["other-value"]}
* """)
* .build(),
* CxFlowTransitionRouteTriggerFulfillmentMessageArgs.builder()
* .conversationSuccess(CxFlowTransitionRouteTriggerFulfillmentMessageConversationSuccessArgs.builder()
* .metadata("""
* {"some-metadata-key": "some-value", "other-metadata-key": 1234}
* """)
* .build())
* .build(),
* CxFlowTransitionRouteTriggerFulfillmentMessageArgs.builder()
* .outputAudioText(CxFlowTransitionRouteTriggerFulfillmentMessageOutputAudioTextArgs.builder()
* .text("some output text")
* .build())
* .build(),
* CxFlowTransitionRouteTriggerFulfillmentMessageArgs.builder()
* .outputAudioText(CxFlowTransitionRouteTriggerFulfillmentMessageOutputAudioTextArgs.builder()
* .ssml("""
* Some example SSML XML
* """)
* .build())
* .build(),
* CxFlowTransitionRouteTriggerFulfillmentMessageArgs.builder()
* .liveAgentHandoff(CxFlowTransitionRouteTriggerFulfillmentMessageLiveAgentHandoffArgs.builder()
* .metadata("""
* {"some-metadata-key": "some-value", "other-metadata-key": 1234}
* """)
* .build())
* .build(),
* CxFlowTransitionRouteTriggerFulfillmentMessageArgs.builder()
* .playAudio(CxFlowTransitionRouteTriggerFulfillmentMessagePlayAudioArgs.builder()
* .audioUri("http://example.com/some-audio-file.mp3")
* .build())
* .build(),
* CxFlowTransitionRouteTriggerFulfillmentMessageArgs.builder()
* .telephonyTransferCall(CxFlowTransitionRouteTriggerFulfillmentMessageTelephonyTransferCallArgs.builder()
* .phoneNumber("1-234-567-8901")
* .build())
* .build())
* .setParameterActions(
* CxFlowTransitionRouteTriggerFulfillmentSetParameterActionArgs.builder()
* .parameter("some-param")
* .value("123.45")
* .build(),
* CxFlowTransitionRouteTriggerFulfillmentSetParameterActionArgs.builder()
* .parameter("another-param")
* .value(serializeJson(
* "abc"))
* .build(),
* CxFlowTransitionRouteTriggerFulfillmentSetParameterActionArgs.builder()
* .parameter("other-param")
* .value(serializeJson(
* jsonArray("foo")))
* .build())
* .conditionalCases(CxFlowTransitionRouteTriggerFulfillmentConditionalCaseArgs.builder()
* .cases(serializeJson(
* jsonArray(
* jsonObject(
* jsonProperty("condition", "$sys.func.RAND() < 0.5"),
* jsonProperty("caseContent", jsonArray(
* jsonObject(
* jsonProperty("message", jsonObject(
* jsonProperty("text", jsonObject(
* jsonProperty("text", jsonArray("First case"))
* ))
* ))
* ),
* jsonObject(
* jsonProperty("additionalCases", jsonObject(
* jsonProperty("cases", jsonArray(jsonObject(
* jsonProperty("condition", "$sys.func.RAND() < 0.2"),
* jsonProperty("caseContent", jsonArray(jsonObject(
* jsonProperty("message", jsonObject(
* jsonProperty("text", jsonObject(
* jsonProperty("text", jsonArray("Nested case"))
* ))
* ))
* )))
* )))
* ))
* )
* ))
* ),
* jsonObject(
* jsonProperty("caseContent", jsonArray(jsonObject(
* jsonProperty("message", jsonObject(
* jsonProperty("text", jsonObject(
* jsonProperty("text", jsonArray("Final case"))
* ))
* ))
* )))
* )
* )))
* .build())
* .build())
* .targetFlow(agent.startFlow())
* .build())
* .advancedSettings(CxFlowAdvancedSettingsArgs.builder()
* .audioExportGcsDestination(CxFlowAdvancedSettingsAudioExportGcsDestinationArgs.builder()
* .uri(bucket.url().applyValue(url -> String.format("%s/prefix-", url)))
* .build())
* .dtmfSettings(CxFlowAdvancedSettingsDtmfSettingsArgs.builder()
* .enabled(true)
* .maxDigits(1)
* .finishDigit("#")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* agent:
* type: gcp:diagflow:CxAgent
* properties:
* displayName: dialogflowcx-agent
* location: global
* defaultLanguageCode: en
* supportedLanguageCodes:
* - fr
* - de
* - es
* timeZone: America/New_York
* description: Example description.
* avatarUri: https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png
* enableStackdriverLogging: true
* enableSpellCorrection: true
* speechToTextSettings:
* enableSpeechAdaptation: true
* bucket:
* type: gcp:storage:Bucket
* properties:
* name: dialogflowcx-bucket
* location: US
* uniformBucketLevelAccess: true
* basicFlow:
* type: gcp:diagflow:CxFlow
* name: basic_flow
* properties:
* parent: ${agent.id}
* displayName: MyFlow
* description: Test Flow
* nluSettings:
* classificationThreshold: 0.3
* modelType: MODEL_TYPE_STANDARD
* eventHandlers:
* - event: custom-event
* triggerFulfillment:
* returnPartialResponses: false
* messages:
* - text:
* texts:
* - I didn't get that. Can you say it again?
* - event: sys.no-match-default
* triggerFulfillment:
* returnPartialResponses: false
* messages:
* - text:
* texts:
* - Sorry, could you say that again?
* - event: sys.no-input-default
* triggerFulfillment:
* returnPartialResponses: false
* messages:
* - text:
* texts:
* - One more time?
* - event: another-event
* triggerFulfillment:
* returnPartialResponses: true
* messages:
* - channel: some-channel
* text:
* texts:
* - Some text
* - payload: |2
* {"some-key": "some-value", "other-key": ["other-value"]}
* - conversationSuccess:
* metadata: |2
* {"some-metadata-key": "some-value", "other-metadata-key": 1234}
* - outputAudioText:
* text: some output text
* - outputAudioText:
* ssml: |2
* Some example SSML XML
* - liveAgentHandoff:
* metadata: |2
* {"some-metadata-key": "some-value", "other-metadata-key": 1234}
* - playAudio:
* audioUri: http://example.com/some-audio-file.mp3
* - telephonyTransferCall:
* phoneNumber: 1-234-567-8901
* setParameterActions:
* - parameter: some-param
* value: '123.45'
* - parameter: another-param
* value:
* fn::toJSON: abc
* - parameter: other-param
* value:
* fn::toJSON:
* - foo
* conditionalCases:
* - cases:
* fn::toJSON:
* - condition: $sys.func.RAND() < 0.5
* caseContent:
* - message:
* text:
* text:
* - First case
* - additionalCases:
* cases:
* - condition: $sys.func.RAND() < 0.2
* caseContent:
* - message:
* text:
* text:
* - Nested case
* - caseContent:
* - message:
* text:
* text:
* - Final case
* transitionRoutes:
* - condition: 'true'
* triggerFulfillment:
* returnPartialResponses: true
* messages:
* - channel: some-channel
* text:
* texts:
* - Some text
* - payload: |2
* {"some-key": "some-value", "other-key": ["other-value"]}
* - conversationSuccess:
* metadata: |2
* {"some-metadata-key": "some-value", "other-metadata-key": 1234}
* - outputAudioText:
* text: some output text
* - outputAudioText:
* ssml: |2
* Some example SSML XML
* - liveAgentHandoff:
* metadata: |2
* {"some-metadata-key": "some-value", "other-metadata-key": 1234}
* - playAudio:
* audioUri: http://example.com/some-audio-file.mp3
* - telephonyTransferCall:
* phoneNumber: 1-234-567-8901
* setParameterActions:
* - parameter: some-param
* value: '123.45'
* - parameter: another-param
* value:
* fn::toJSON: abc
* - parameter: other-param
* value:
* fn::toJSON:
* - foo
* conditionalCases:
* - cases:
* fn::toJSON:
* - condition: $sys.func.RAND() < 0.5
* caseContent:
* - message:
* text:
* text:
* - First case
* - additionalCases:
* cases:
* - condition: $sys.func.RAND() < 0.2
* caseContent:
* - message:
* text:
* text:
* - Nested case
* - caseContent:
* - message:
* text:
* text:
* - Final case
* targetFlow: ${agent.startFlow}
* advancedSettings:
* audioExportGcsDestination:
* uri: ${bucket.url}/prefix-
* dtmfSettings:
* enabled: true
* maxDigits: 1
* finishDigit: '#'
* ```
*
* ## Import
* Flow can be imported using any of these accepted formats:
* * `{{parent}}/flows/{{name}}`
* * `{{parent}}/{{name}}`
* When using the `pulumi import` command, Flow can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:diagflow/cxFlow:CxFlow default {{parent}}/flows/{{name}}
* ```
* ```sh
* $ pulumi import gcp:diagflow/cxFlow:CxFlow default {{parent}}/{{name}}
* ```
* @property advancedSettings Hierarchical advanced settings for this flow. The settings exposed at the lower level overrides the settings exposed at the higher level.
* Hierarchy: Agent->Flow->Page->Fulfillment/Parameter.
* Structure is documented below.
* @property description The description of the flow. The maximum length is 500 characters. If exceeded, the request is rejected.
* @property displayName The human-readable name of the flow.
* - - -
* @property eventHandlers A flow's event handlers serve two purposes:
* They are responsible for handling events (e.g. no match, webhook errors) in the flow.
* They are inherited by every page's [event handlers][Page.event_handlers], which can be used to handle common events regardless of the current page. Event handlers defined in the page have higher priority than those defined in the flow.
* Unlike transitionRoutes, these handlers are evaluated on a first-match basis. The first one that matches the event get executed, with the rest being ignored.
* Structure is documented below.
* @property isDefaultStartFlow Marks this as the [Default Start Flow](https://cloud.google.com/dialogflow/cx/docs/concept/flow#start) for an agent. When you create an agent, the Default Start Flow is created automatically.
* The Default Start Flow cannot be deleted; deleting the `gcp.diagflow.CxFlow` resource does nothing to the underlying GCP resources.
* > Avoid having multiple `gcp.diagflow.CxFlow` resources linked to the same agent with `is_default_start_flow = true` because they will compete to control a single Default Start Flow resource in GCP.
* @property languageCode The language of the following fields in flow:
* Flow.event_handlers.trigger_fulfillment.messages
* Flow.event_handlers.trigger_fulfillment.conditional_cases
* Flow.transition_routes.trigger_fulfillment.messages
* Flow.transition_routes.trigger_fulfillment.conditional_cases
* If not specified, the agent's default language is used. Many languages are supported. Note: languages must be enabled in the agent before they can be used.
* @property nluSettings NLU related settings of the flow.
* Structure is documented below.
* @property parent The agent to create a flow for.
* Format: projects//locations//agents/.
* @property transitionRouteGroups A flow's transition route group serve two purposes:
* They are responsible for matching the user's first utterances in the flow.
* They are inherited by every page's [transition route groups][Page.transition_route_groups]. Transition route groups defined in the page have higher priority than those defined in the flow.
* Format:projects//locations//agents//flows//transitionRouteGroups/.
* @property transitionRoutes A flow's transition routes serve two purposes:
* They are responsible for matching the user's first utterances in the flow.
* They are inherited by every page's [transition routes][Page.transition_routes] and can support use cases such as the user saying "help" or "can I talk to a human?", which can be handled in a common way regardless of the current page. Transition routes defined in the page have higher priority than those defined in the flow.
* TransitionRoutes are evalauted in the following order:
* TransitionRoutes with intent specified.
* TransitionRoutes with only condition specified.
* TransitionRoutes with intent specified are inherited by pages in the flow.
* Structure is documented below.
*/
public data class CxFlowArgs(
public val advancedSettings: Output? = null,
public val description: Output? = null,
public val displayName: Output? = null,
public val eventHandlers: Output>? = null,
public val isDefaultStartFlow: Output? = null,
public val languageCode: Output? = null,
public val nluSettings: Output? = null,
public val parent: Output? = null,
public val transitionRouteGroups: Output>? = null,
public val transitionRoutes: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.diagflow.CxFlowArgs =
com.pulumi.gcp.diagflow.CxFlowArgs.builder()
.advancedSettings(advancedSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.eventHandlers(
eventHandlers?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.isDefaultStartFlow(isDefaultStartFlow?.applyValue({ args0 -> args0 }))
.languageCode(languageCode?.applyValue({ args0 -> args0 }))
.nluSettings(nluSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.parent(parent?.applyValue({ args0 -> args0 }))
.transitionRouteGroups(transitionRouteGroups?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.transitionRoutes(
transitionRoutes?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [CxFlowArgs].
*/
@PulumiTagMarker
public class CxFlowArgsBuilder internal constructor() {
private var advancedSettings: Output? = null
private var description: Output? = null
private var displayName: Output? = null
private var eventHandlers: Output>? = null
private var isDefaultStartFlow: Output? = null
private var languageCode: Output? = null
private var nluSettings: Output? = null
private var parent: Output? = null
private var transitionRouteGroups: Output>? = null
private var transitionRoutes: Output>? = null
/**
* @param value Hierarchical advanced settings for this flow. The settings exposed at the lower level overrides the settings exposed at the higher level.
* Hierarchy: Agent->Flow->Page->Fulfillment/Parameter.
* Structure is documented below.
*/
@JvmName("wlminaqbboftjyek")
public suspend fun advancedSettings(`value`: Output) {
this.advancedSettings = value
}
/**
* @param value The description of the flow. The maximum length is 500 characters. If exceeded, the request is rejected.
*/
@JvmName("fopcoqpsvuruhruo")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The human-readable name of the flow.
* - - -
*/
@JvmName("gycvuvokdnypidbf")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value A flow's event handlers serve two purposes:
* They are responsible for handling events (e.g. no match, webhook errors) in the flow.
* They are inherited by every page's [event handlers][Page.event_handlers], which can be used to handle common events regardless of the current page. Event handlers defined in the page have higher priority than those defined in the flow.
* Unlike transitionRoutes, these handlers are evaluated on a first-match basis. The first one that matches the event get executed, with the rest being ignored.
* Structure is documented below.
*/
@JvmName("ofxmpvyrsxdoosxj")
public suspend fun eventHandlers(`value`: Output>) {
this.eventHandlers = value
}
@JvmName("cuhcgyeyxcroaamg")
public suspend fun eventHandlers(vararg values: Output) {
this.eventHandlers = Output.all(values.asList())
}
/**
* @param values A flow's event handlers serve two purposes:
* They are responsible for handling events (e.g. no match, webhook errors) in the flow.
* They are inherited by every page's [event handlers][Page.event_handlers], which can be used to handle common events regardless of the current page. Event handlers defined in the page have higher priority than those defined in the flow.
* Unlike transitionRoutes, these handlers are evaluated on a first-match basis. The first one that matches the event get executed, with the rest being ignored.
* Structure is documented below.
*/
@JvmName("utugkydgurtuixov")
public suspend fun eventHandlers(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy