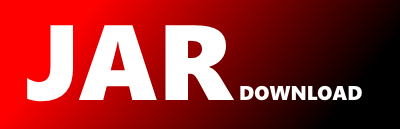
com.pulumi.gcp.diagflow.kotlin.CxIntent.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.diagflow.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.diagflow.kotlin.outputs.CxIntentParameter
import com.pulumi.gcp.diagflow.kotlin.outputs.CxIntentTrainingPhrase
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.gcp.diagflow.kotlin.outputs.CxIntentParameter.Companion.toKotlin as cxIntentParameterToKotlin
import com.pulumi.gcp.diagflow.kotlin.outputs.CxIntentTrainingPhrase.Companion.toKotlin as cxIntentTrainingPhraseToKotlin
/**
* Builder for [CxIntent].
*/
@PulumiTagMarker
public class CxIntentResourceBuilder internal constructor() {
public var name: String? = null
public var args: CxIntentArgs = CxIntentArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend CxIntentArgsBuilder.() -> Unit) {
val builder = CxIntentArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): CxIntent {
val builtJavaResource = com.pulumi.gcp.diagflow.CxIntent(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return CxIntent(builtJavaResource)
}
}
/**
* An intent represents a user's intent to interact with a conversational agent.
* To get more information about Intent, see:
* * [API documentation](https://cloud.google.com/dialogflow/cx/docs/reference/rest/v3/projects.locations.agents.intents)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/dialogflow/cx/docs)
* ## Example Usage
* ### Dialogflowcx Intent Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const agent = new gcp.diagflow.CxAgent("agent", {
* displayName: "dialogflowcx-agent",
* location: "global",
* defaultLanguageCode: "en",
* supportedLanguageCodes: [
* "fr",
* "de",
* "es",
* ],
* timeZone: "America/New_York",
* description: "Example description.",
* avatarUri: "https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* enableStackdriverLogging: true,
* enableSpellCorrection: true,
* speechToTextSettings: {
* enableSpeechAdaptation: true,
* },
* });
* const basicIntent = new gcp.diagflow.CxIntent("basic_intent", {
* parent: agent.id,
* displayName: "Example",
* priority: 1,
* description: "Intent example",
* trainingPhrases: [{
* parts: [
* {
* text: "training",
* },
* {
* text: "phrase",
* },
* {
* text: "example",
* },
* ],
* repeatCount: 1,
* }],
* parameters: [{
* id: "param1",
* entityType: "projects/-/locations/-/agents/-/entityTypes/sys.date",
* }],
* labels: {
* label1: "value1",
* label2: "value2",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* agent = gcp.diagflow.CxAgent("agent",
* display_name="dialogflowcx-agent",
* location="global",
* default_language_code="en",
* supported_language_codes=[
* "fr",
* "de",
* "es",
* ],
* time_zone="America/New_York",
* description="Example description.",
* avatar_uri="https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* enable_stackdriver_logging=True,
* enable_spell_correction=True,
* speech_to_text_settings=gcp.diagflow.CxAgentSpeechToTextSettingsArgs(
* enable_speech_adaptation=True,
* ))
* basic_intent = gcp.diagflow.CxIntent("basic_intent",
* parent=agent.id,
* display_name="Example",
* priority=1,
* description="Intent example",
* training_phrases=[gcp.diagflow.CxIntentTrainingPhraseArgs(
* parts=[
* gcp.diagflow.CxIntentTrainingPhrasePartArgs(
* text="training",
* ),
* gcp.diagflow.CxIntentTrainingPhrasePartArgs(
* text="phrase",
* ),
* gcp.diagflow.CxIntentTrainingPhrasePartArgs(
* text="example",
* ),
* ],
* repeat_count=1,
* )],
* parameters=[gcp.diagflow.CxIntentParameterArgs(
* id="param1",
* entity_type="projects/-/locations/-/agents/-/entityTypes/sys.date",
* )],
* labels={
* "label1": "value1",
* "label2": "value2",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var agent = new Gcp.Diagflow.CxAgent("agent", new()
* {
* DisplayName = "dialogflowcx-agent",
* Location = "global",
* DefaultLanguageCode = "en",
* SupportedLanguageCodes = new[]
* {
* "fr",
* "de",
* "es",
* },
* TimeZone = "America/New_York",
* Description = "Example description.",
* AvatarUri = "https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png",
* EnableStackdriverLogging = true,
* EnableSpellCorrection = true,
* SpeechToTextSettings = new Gcp.Diagflow.Inputs.CxAgentSpeechToTextSettingsArgs
* {
* EnableSpeechAdaptation = true,
* },
* });
* var basicIntent = new Gcp.Diagflow.CxIntent("basic_intent", new()
* {
* Parent = agent.Id,
* DisplayName = "Example",
* Priority = 1,
* Description = "Intent example",
* TrainingPhrases = new[]
* {
* new Gcp.Diagflow.Inputs.CxIntentTrainingPhraseArgs
* {
* Parts = new[]
* {
* new Gcp.Diagflow.Inputs.CxIntentTrainingPhrasePartArgs
* {
* Text = "training",
* },
* new Gcp.Diagflow.Inputs.CxIntentTrainingPhrasePartArgs
* {
* Text = "phrase",
* },
* new Gcp.Diagflow.Inputs.CxIntentTrainingPhrasePartArgs
* {
* Text = "example",
* },
* },
* RepeatCount = 1,
* },
* },
* Parameters = new[]
* {
* new Gcp.Diagflow.Inputs.CxIntentParameterArgs
* {
* Id = "param1",
* EntityType = "projects/-/locations/-/agents/-/entityTypes/sys.date",
* },
* },
* Labels =
* {
* { "label1", "value1" },
* { "label2", "value2" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/diagflow"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* agent, err := diagflow.NewCxAgent(ctx, "agent", &diagflow.CxAgentArgs{
* DisplayName: pulumi.String("dialogflowcx-agent"),
* Location: pulumi.String("global"),
* DefaultLanguageCode: pulumi.String("en"),
* SupportedLanguageCodes: pulumi.StringArray{
* pulumi.String("fr"),
* pulumi.String("de"),
* pulumi.String("es"),
* },
* TimeZone: pulumi.String("America/New_York"),
* Description: pulumi.String("Example description."),
* AvatarUri: pulumi.String("https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png"),
* EnableStackdriverLogging: pulumi.Bool(true),
* EnableSpellCorrection: pulumi.Bool(true),
* SpeechToTextSettings: &diagflow.CxAgentSpeechToTextSettingsArgs{
* EnableSpeechAdaptation: pulumi.Bool(true),
* },
* })
* if err != nil {
* return err
* }
* _, err = diagflow.NewCxIntent(ctx, "basic_intent", &diagflow.CxIntentArgs{
* Parent: agent.ID(),
* DisplayName: pulumi.String("Example"),
* Priority: pulumi.Int(1),
* Description: pulumi.String("Intent example"),
* TrainingPhrases: diagflow.CxIntentTrainingPhraseArray{
* &diagflow.CxIntentTrainingPhraseArgs{
* Parts: diagflow.CxIntentTrainingPhrasePartArray{
* &diagflow.CxIntentTrainingPhrasePartArgs{
* Text: pulumi.String("training"),
* },
* &diagflow.CxIntentTrainingPhrasePartArgs{
* Text: pulumi.String("phrase"),
* },
* &diagflow.CxIntentTrainingPhrasePartArgs{
* Text: pulumi.String("example"),
* },
* },
* RepeatCount: pulumi.Int(1),
* },
* },
* Parameters: diagflow.CxIntentParameterArray{
* &diagflow.CxIntentParameterArgs{
* Id: pulumi.String("param1"),
* EntityType: pulumi.String("projects/-/locations/-/agents/-/entityTypes/sys.date"),
* },
* },
* Labels: pulumi.StringMap{
* "label1": pulumi.String("value1"),
* "label2": pulumi.String("value2"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.diagflow.CxAgent;
* import com.pulumi.gcp.diagflow.CxAgentArgs;
* import com.pulumi.gcp.diagflow.inputs.CxAgentSpeechToTextSettingsArgs;
* import com.pulumi.gcp.diagflow.CxIntent;
* import com.pulumi.gcp.diagflow.CxIntentArgs;
* import com.pulumi.gcp.diagflow.inputs.CxIntentTrainingPhraseArgs;
* import com.pulumi.gcp.diagflow.inputs.CxIntentParameterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var agent = new CxAgent("agent", CxAgentArgs.builder()
* .displayName("dialogflowcx-agent")
* .location("global")
* .defaultLanguageCode("en")
* .supportedLanguageCodes(
* "fr",
* "de",
* "es")
* .timeZone("America/New_York")
* .description("Example description.")
* .avatarUri("https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png")
* .enableStackdriverLogging(true)
* .enableSpellCorrection(true)
* .speechToTextSettings(CxAgentSpeechToTextSettingsArgs.builder()
* .enableSpeechAdaptation(true)
* .build())
* .build());
* var basicIntent = new CxIntent("basicIntent", CxIntentArgs.builder()
* .parent(agent.id())
* .displayName("Example")
* .priority(1)
* .description("Intent example")
* .trainingPhrases(CxIntentTrainingPhraseArgs.builder()
* .parts(
* CxIntentTrainingPhrasePartArgs.builder()
* .text("training")
* .build(),
* CxIntentTrainingPhrasePartArgs.builder()
* .text("phrase")
* .build(),
* CxIntentTrainingPhrasePartArgs.builder()
* .text("example")
* .build())
* .repeatCount(1)
* .build())
* .parameters(CxIntentParameterArgs.builder()
* .id("param1")
* .entityType("projects/-/locations/-/agents/-/entityTypes/sys.date")
* .build())
* .labels(Map.ofEntries(
* Map.entry("label1", "value1"),
* Map.entry("label2", "value2")
* ))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* agent:
* type: gcp:diagflow:CxAgent
* properties:
* displayName: dialogflowcx-agent
* location: global
* defaultLanguageCode: en
* supportedLanguageCodes:
* - fr
* - de
* - es
* timeZone: America/New_York
* description: Example description.
* avatarUri: https://cloud.google.com/_static/images/cloud/icons/favicons/onecloud/super_cloud.png
* enableStackdriverLogging: true
* enableSpellCorrection: true
* speechToTextSettings:
* enableSpeechAdaptation: true
* basicIntent:
* type: gcp:diagflow:CxIntent
* name: basic_intent
* properties:
* parent: ${agent.id}
* displayName: Example
* priority: 1
* description: Intent example
* trainingPhrases:
* - parts:
* - text: training
* - text: phrase
* - text: example
* repeatCount: 1
* parameters:
* - id: param1
* entityType: projects/-/locations/-/agents/-/entityTypes/sys.date
* labels:
* label1: value1
* label2: value2
* ```
*
* ## Import
* Intent can be imported using any of these accepted formats:
* * `{{parent}}/intents/{{name}}`
* * `{{parent}}/{{name}}`
* When using the `pulumi import` command, Intent can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:diagflow/cxIntent:CxIntent default {{parent}}/intents/{{name}}
* ```
* ```sh
* $ pulumi import gcp:diagflow/cxIntent:CxIntent default {{parent}}/{{name}}
* ```
*/
public class CxIntent internal constructor(
override val javaResource: com.pulumi.gcp.diagflow.CxIntent,
) : KotlinCustomResource(javaResource, CxIntentMapper) {
/**
* Human readable description for better understanding an intent like its scope, content, result etc. Maximum character limit: 140 characters.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The human-readable name of the intent, unique within the agent.
* - - -
*/
public val displayName: Output
get() = javaResource.displayName().applyValue({ args0 -> args0 })
/**
* All of labels (key/value pairs) present on the resource in GCP, including the labels configured through Pulumi, other clients and services.
*/
public val effectiveLabels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy