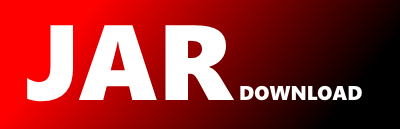
com.pulumi.gcp.diagflow.kotlin.Fulfillment.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.diagflow.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.diagflow.kotlin.outputs.FulfillmentFeature
import com.pulumi.gcp.diagflow.kotlin.outputs.FulfillmentGenericWebService
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.gcp.diagflow.kotlin.outputs.FulfillmentFeature.Companion.toKotlin as fulfillmentFeatureToKotlin
import com.pulumi.gcp.diagflow.kotlin.outputs.FulfillmentGenericWebService.Companion.toKotlin as fulfillmentGenericWebServiceToKotlin
/**
* Builder for [Fulfillment].
*/
@PulumiTagMarker
public class FulfillmentResourceBuilder internal constructor() {
public var name: String? = null
public var args: FulfillmentArgs = FulfillmentArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FulfillmentArgsBuilder.() -> Unit) {
val builder = FulfillmentArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Fulfillment {
val builtJavaResource = com.pulumi.gcp.diagflow.Fulfillment(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Fulfillment(builtJavaResource)
}
}
/**
* By default, your agent responds to a matched intent with a static response. If you're using one of the integration options, you can provide a more dynamic response by using fulfillment. When you enable fulfillment for an intent, Dialogflow responds to that intent by calling a service that you define. For example, if an end-user wants to schedule a haircut on Friday, your service can check your database and respond to the end-user with availability information for Friday.
* To get more information about Fulfillment, see:
* * [API documentation](https://cloud.google.com/dialogflow/es/docs/reference/rest/v2/projects.agent/getFulfillment)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/dialogflow/es/docs/fulfillment-overview)
* ## Example Usage
* ### Dialogflow Fulfillment Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const basicAgent = new gcp.diagflow.Agent("basic_agent", {
* displayName: "example_agent",
* defaultLanguageCode: "en",
* timeZone: "America/New_York",
* });
* const basicFulfillment = new gcp.diagflow.Fulfillment("basic_fulfillment", {
* displayName: "basic-fulfillment",
* enabled: true,
* genericWebService: {
* uri: "https://google.com",
* username: "admin",
* password: "password",
* requestHeaders: {
* name: "wrench",
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* basic_agent = gcp.diagflow.Agent("basic_agent",
* display_name="example_agent",
* default_language_code="en",
* time_zone="America/New_York")
* basic_fulfillment = gcp.diagflow.Fulfillment("basic_fulfillment",
* display_name="basic-fulfillment",
* enabled=True,
* generic_web_service=gcp.diagflow.FulfillmentGenericWebServiceArgs(
* uri="https://google.com",
* username="admin",
* password="password",
* request_headers={
* "name": "wrench",
* },
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var basicAgent = new Gcp.Diagflow.Agent("basic_agent", new()
* {
* DisplayName = "example_agent",
* DefaultLanguageCode = "en",
* TimeZone = "America/New_York",
* });
* var basicFulfillment = new Gcp.Diagflow.Fulfillment("basic_fulfillment", new()
* {
* DisplayName = "basic-fulfillment",
* Enabled = true,
* GenericWebService = new Gcp.Diagflow.Inputs.FulfillmentGenericWebServiceArgs
* {
* Uri = "https://google.com",
* Username = "admin",
* Password = "password",
* RequestHeaders =
* {
* { "name", "wrench" },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/diagflow"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := diagflow.NewAgent(ctx, "basic_agent", &diagflow.AgentArgs{
* DisplayName: pulumi.String("example_agent"),
* DefaultLanguageCode: pulumi.String("en"),
* TimeZone: pulumi.String("America/New_York"),
* })
* if err != nil {
* return err
* }
* _, err = diagflow.NewFulfillment(ctx, "basic_fulfillment", &diagflow.FulfillmentArgs{
* DisplayName: pulumi.String("basic-fulfillment"),
* Enabled: pulumi.Bool(true),
* GenericWebService: &diagflow.FulfillmentGenericWebServiceArgs{
* Uri: pulumi.String("https://google.com"),
* Username: pulumi.String("admin"),
* Password: pulumi.String("password"),
* RequestHeaders: pulumi.StringMap{
* "name": pulumi.String("wrench"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.diagflow.Agent;
* import com.pulumi.gcp.diagflow.AgentArgs;
* import com.pulumi.gcp.diagflow.Fulfillment;
* import com.pulumi.gcp.diagflow.FulfillmentArgs;
* import com.pulumi.gcp.diagflow.inputs.FulfillmentGenericWebServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var basicAgent = new Agent("basicAgent", AgentArgs.builder()
* .displayName("example_agent")
* .defaultLanguageCode("en")
* .timeZone("America/New_York")
* .build());
* var basicFulfillment = new Fulfillment("basicFulfillment", FulfillmentArgs.builder()
* .displayName("basic-fulfillment")
* .enabled(true)
* .genericWebService(FulfillmentGenericWebServiceArgs.builder()
* .uri("https://google.com")
* .username("admin")
* .password("password")
* .requestHeaders(Map.of("name", "wrench"))
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* basicAgent:
* type: gcp:diagflow:Agent
* name: basic_agent
* properties:
* displayName: example_agent
* defaultLanguageCode: en
* timeZone: America/New_York
* basicFulfillment:
* type: gcp:diagflow:Fulfillment
* name: basic_fulfillment
* properties:
* displayName: basic-fulfillment
* enabled: true
* genericWebService:
* uri: https://google.com
* username: admin
* password: password
* requestHeaders:
* name: wrench
* ```
*
* ## Import
* Fulfillment can be imported using any of these accepted formats:
* * `{{name}}`
* When using the `pulumi import` command, Fulfillment can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:diagflow/fulfillment:Fulfillment default {{name}}
* ```
*/
public class Fulfillment internal constructor(
override val javaResource: com.pulumi.gcp.diagflow.Fulfillment,
) : KotlinCustomResource(javaResource, FulfillmentMapper) {
/**
* The human-readable name of the fulfillment, unique within the agent.
* - - -
*/
public val displayName: Output
get() = javaResource.displayName().applyValue({ args0 -> args0 })
/**
* Whether fulfillment is enabled.
*/
public val enabled: Output?
get() = javaResource.enabled().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The field defines whether the fulfillment is enabled for certain features.
* Structure is documented below.
*/
public val features: Output>?
get() = javaResource.features().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> fulfillmentFeatureToKotlin(args0) })
})
}).orElse(null)
})
/**
* Represents configuration for a generic web service. Dialogflow supports two mechanisms for authentications: - Basic authentication with username and password. - Authentication with additional authentication headers.
* Structure is documented below.
*/
public val genericWebService: Output?
get() = javaResource.genericWebService().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> fulfillmentGenericWebServiceToKotlin(args0) })
}).orElse(null)
})
/**
* The unique identifier of the fulfillment.
* Format: projects//agent/fulfillment - projects//locations//agent/fulfillment
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
}
public object FulfillmentMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.diagflow.Fulfillment::class == javaResource::class
override fun map(javaResource: Resource): Fulfillment = Fulfillment(
javaResource as
com.pulumi.gcp.diagflow.Fulfillment,
)
}
/**
* @see [Fulfillment].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Fulfillment].
*/
public suspend fun fulfillment(name: String, block: suspend FulfillmentResourceBuilder.() -> Unit): Fulfillment {
val builder = FulfillmentResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Fulfillment].
* @param name The _unique_ name of the resulting resource.
*/
public fun fulfillment(name: String): Fulfillment {
val builder = FulfillmentResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy