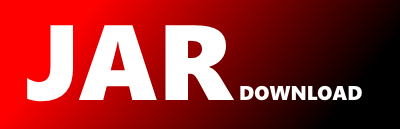
com.pulumi.gcp.diagflow.kotlin.inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.diagflow.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.diagflow.inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property channel The channel which the response is associated with. Clients can specify the channel via QueryParameters.channel, and only associated channel response will be returned.
* @property conversationSuccess Indicates that the conversation succeeded, i.e., the bot handled the issue that the customer talked to it about.
* Dialogflow only uses this to determine which conversations should be counted as successful and doesn't process the metadata in this message in any way. Note that Dialogflow also considers conversations that get to the conversation end page as successful even if they don't return ConversationSuccess.
* You may set this, for example:
* * In the entryFulfillment of a Page if entering the page indicates that the conversation succeeded.
* * In a webhook response when you determine that you handled the customer issue.
* Structure is documented below.
* @property liveAgentHandoff Indicates that the conversation should be handed off to a live agent.
* Dialogflow only uses this to determine which conversations were handed off to a human agent for measurement purposes. What else to do with this signal is up to you and your handoff procedures.
* You may set this, for example:
* * In the entryFulfillment of a Page if entering the page indicates something went extremely wrong in the conversation.
* * In a webhook response when you determine that the customer issue can only be handled by a human.
* Structure is documented below.
* @property outputAudioText A text or ssml response that is preferentially used for TTS output audio synthesis, as described in the comment on the ResponseMessage message.
* Structure is documented below.
* @property payload A custom, platform-specific payload.
* @property playAudio Specifies an audio clip to be played by the client as part of the response.
* Structure is documented below.
* @property telephonyTransferCall Represents the signal that telles the client to transfer the phone call connected to the agent to a third-party endpoint.
* Structure is documented below.
* @property text The text response message.
* Structure is documented below.
*/
public data class CxFlowEventHandlerTriggerFulfillmentMessageArgs(
public val channel: Output? = null,
public val conversationSuccess: Output? = null,
public val liveAgentHandoff: Output? = null,
public val outputAudioText: Output? = null,
public val payload: Output? = null,
public val playAudio: Output? = null,
public val telephonyTransferCall: Output? = null,
public val text: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.diagflow.inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs =
com.pulumi.gcp.diagflow.inputs.CxFlowEventHandlerTriggerFulfillmentMessageArgs.builder()
.channel(channel?.applyValue({ args0 -> args0 }))
.conversationSuccess(
conversationSuccess?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.liveAgentHandoff(liveAgentHandoff?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.outputAudioText(outputAudioText?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.payload(payload?.applyValue({ args0 -> args0 }))
.playAudio(playAudio?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.telephonyTransferCall(
telephonyTransferCall?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.text(text?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [CxFlowEventHandlerTriggerFulfillmentMessageArgs].
*/
@PulumiTagMarker
public class CxFlowEventHandlerTriggerFulfillmentMessageArgsBuilder internal constructor() {
private var channel: Output? = null
private var conversationSuccess:
Output? = null
private var liveAgentHandoff:
Output? = null
private var outputAudioText:
Output? = null
private var payload: Output? = null
private var playAudio: Output? = null
private var telephonyTransferCall:
Output? = null
private var text: Output? = null
/**
* @param value The channel which the response is associated with. Clients can specify the channel via QueryParameters.channel, and only associated channel response will be returned.
*/
@JvmName("dkxoviocdxfreahl")
public suspend fun channel(`value`: Output) {
this.channel = value
}
/**
* @param value Indicates that the conversation succeeded, i.e., the bot handled the issue that the customer talked to it about.
* Dialogflow only uses this to determine which conversations should be counted as successful and doesn't process the metadata in this message in any way. Note that Dialogflow also considers conversations that get to the conversation end page as successful even if they don't return ConversationSuccess.
* You may set this, for example:
* * In the entryFulfillment of a Page if entering the page indicates that the conversation succeeded.
* * In a webhook response when you determine that you handled the customer issue.
* Structure is documented below.
*/
@JvmName("doccfspsycmvwler")
public suspend fun conversationSuccess(`value`: Output) {
this.conversationSuccess = value
}
/**
* @param value Indicates that the conversation should be handed off to a live agent.
* Dialogflow only uses this to determine which conversations were handed off to a human agent for measurement purposes. What else to do with this signal is up to you and your handoff procedures.
* You may set this, for example:
* * In the entryFulfillment of a Page if entering the page indicates something went extremely wrong in the conversation.
* * In a webhook response when you determine that the customer issue can only be handled by a human.
* Structure is documented below.
*/
@JvmName("nmtmyyihtpxneist")
public suspend fun liveAgentHandoff(`value`: Output) {
this.liveAgentHandoff = value
}
/**
* @param value A text or ssml response that is preferentially used for TTS output audio synthesis, as described in the comment on the ResponseMessage message.
* Structure is documented below.
*/
@JvmName("ditovwbkarvetkix")
public suspend fun outputAudioText(`value`: Output) {
this.outputAudioText = value
}
/**
* @param value A custom, platform-specific payload.
*/
@JvmName("pvwofvnqnjbqxlpa")
public suspend fun payload(`value`: Output) {
this.payload = value
}
/**
* @param value Specifies an audio clip to be played by the client as part of the response.
* Structure is documented below.
*/
@JvmName("lhqvlwlqovxaxecs")
public suspend fun playAudio(`value`: Output) {
this.playAudio = value
}
/**
* @param value Represents the signal that telles the client to transfer the phone call connected to the agent to a third-party endpoint.
* Structure is documented below.
*/
@JvmName("nsbopfpkmtvtouim")
public suspend fun telephonyTransferCall(`value`: Output) {
this.telephonyTransferCall = value
}
/**
* @param value The text response message.
* Structure is documented below.
*/
@JvmName("gfjyntawjcuyyfmm")
public suspend fun text(`value`: Output) {
this.text = value
}
/**
* @param value The channel which the response is associated with. Clients can specify the channel via QueryParameters.channel, and only associated channel response will be returned.
*/
@JvmName("xfmtagsuifbrsphr")
public suspend fun channel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.channel = mapped
}
/**
* @param value Indicates that the conversation succeeded, i.e., the bot handled the issue that the customer talked to it about.
* Dialogflow only uses this to determine which conversations should be counted as successful and doesn't process the metadata in this message in any way. Note that Dialogflow also considers conversations that get to the conversation end page as successful even if they don't return ConversationSuccess.
* You may set this, for example:
* * In the entryFulfillment of a Page if entering the page indicates that the conversation succeeded.
* * In a webhook response when you determine that you handled the customer issue.
* Structure is documented below.
*/
@JvmName("qhgnrgcuebpohstb")
public suspend fun conversationSuccess(`value`: CxFlowEventHandlerTriggerFulfillmentMessageConversationSuccessArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.conversationSuccess = mapped
}
/**
* @param argument Indicates that the conversation succeeded, i.e., the bot handled the issue that the customer talked to it about.
* Dialogflow only uses this to determine which conversations should be counted as successful and doesn't process the metadata in this message in any way. Note that Dialogflow also considers conversations that get to the conversation end page as successful even if they don't return ConversationSuccess.
* You may set this, for example:
* * In the entryFulfillment of a Page if entering the page indicates that the conversation succeeded.
* * In a webhook response when you determine that you handled the customer issue.
* Structure is documented below.
*/
@JvmName("okttynylnoovgyah")
public suspend fun conversationSuccess(argument: suspend CxFlowEventHandlerTriggerFulfillmentMessageConversationSuccessArgsBuilder.() -> Unit) {
val toBeMapped =
CxFlowEventHandlerTriggerFulfillmentMessageConversationSuccessArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.conversationSuccess = mapped
}
/**
* @param value Indicates that the conversation should be handed off to a live agent.
* Dialogflow only uses this to determine which conversations were handed off to a human agent for measurement purposes. What else to do with this signal is up to you and your handoff procedures.
* You may set this, for example:
* * In the entryFulfillment of a Page if entering the page indicates something went extremely wrong in the conversation.
* * In a webhook response when you determine that the customer issue can only be handled by a human.
* Structure is documented below.
*/
@JvmName("fvryjcwornsphwnl")
public suspend fun liveAgentHandoff(`value`: CxFlowEventHandlerTriggerFulfillmentMessageLiveAgentHandoffArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.liveAgentHandoff = mapped
}
/**
* @param argument Indicates that the conversation should be handed off to a live agent.
* Dialogflow only uses this to determine which conversations were handed off to a human agent for measurement purposes. What else to do with this signal is up to you and your handoff procedures.
* You may set this, for example:
* * In the entryFulfillment of a Page if entering the page indicates something went extremely wrong in the conversation.
* * In a webhook response when you determine that the customer issue can only be handled by a human.
* Structure is documented below.
*/
@JvmName("tvisxyscycgotsul")
public suspend fun liveAgentHandoff(argument: suspend CxFlowEventHandlerTriggerFulfillmentMessageLiveAgentHandoffArgsBuilder.() -> Unit) {
val toBeMapped =
CxFlowEventHandlerTriggerFulfillmentMessageLiveAgentHandoffArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.liveAgentHandoff = mapped
}
/**
* @param value A text or ssml response that is preferentially used for TTS output audio synthesis, as described in the comment on the ResponseMessage message.
* Structure is documented below.
*/
@JvmName("xkflpprvoyrakmwo")
public suspend fun outputAudioText(`value`: CxFlowEventHandlerTriggerFulfillmentMessageOutputAudioTextArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.outputAudioText = mapped
}
/**
* @param argument A text or ssml response that is preferentially used for TTS output audio synthesis, as described in the comment on the ResponseMessage message.
* Structure is documented below.
*/
@JvmName("urnawhlyjvxymeqm")
public suspend fun outputAudioText(argument: suspend CxFlowEventHandlerTriggerFulfillmentMessageOutputAudioTextArgsBuilder.() -> Unit) {
val toBeMapped =
CxFlowEventHandlerTriggerFulfillmentMessageOutputAudioTextArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.outputAudioText = mapped
}
/**
* @param value A custom, platform-specific payload.
*/
@JvmName("oybijgqgxhgejsse")
public suspend fun payload(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.payload = mapped
}
/**
* @param value Specifies an audio clip to be played by the client as part of the response.
* Structure is documented below.
*/
@JvmName("vdpvdgvlegruvmjc")
public suspend fun playAudio(`value`: CxFlowEventHandlerTriggerFulfillmentMessagePlayAudioArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.playAudio = mapped
}
/**
* @param argument Specifies an audio clip to be played by the client as part of the response.
* Structure is documented below.
*/
@JvmName("bydhxpkmlgqaibai")
public suspend fun playAudio(argument: suspend CxFlowEventHandlerTriggerFulfillmentMessagePlayAudioArgsBuilder.() -> Unit) {
val toBeMapped = CxFlowEventHandlerTriggerFulfillmentMessagePlayAudioArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.playAudio = mapped
}
/**
* @param value Represents the signal that telles the client to transfer the phone call connected to the agent to a third-party endpoint.
* Structure is documented below.
*/
@JvmName("cumxaxlsapqeecpb")
public suspend fun telephonyTransferCall(`value`: CxFlowEventHandlerTriggerFulfillmentMessageTelephonyTransferCallArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.telephonyTransferCall = mapped
}
/**
* @param argument Represents the signal that telles the client to transfer the phone call connected to the agent to a third-party endpoint.
* Structure is documented below.
*/
@JvmName("qmikrlwhhumhcogk")
public suspend fun telephonyTransferCall(argument: suspend CxFlowEventHandlerTriggerFulfillmentMessageTelephonyTransferCallArgsBuilder.() -> Unit) {
val toBeMapped =
CxFlowEventHandlerTriggerFulfillmentMessageTelephonyTransferCallArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.telephonyTransferCall = mapped
}
/**
* @param value The text response message.
* Structure is documented below.
*/
@JvmName("hcrqqcvtvqxggaii")
public suspend fun text(`value`: CxFlowEventHandlerTriggerFulfillmentMessageTextArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.text = mapped
}
/**
* @param argument The text response message.
* Structure is documented below.
*/
@JvmName("tbsvqvlcrjmdqadl")
public suspend fun text(argument: suspend CxFlowEventHandlerTriggerFulfillmentMessageTextArgsBuilder.() -> Unit) {
val toBeMapped = CxFlowEventHandlerTriggerFulfillmentMessageTextArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.text = mapped
}
internal fun build(): CxFlowEventHandlerTriggerFulfillmentMessageArgs =
CxFlowEventHandlerTriggerFulfillmentMessageArgs(
channel = channel,
conversationSuccess = conversationSuccess,
liveAgentHandoff = liveAgentHandoff,
outputAudioText = outputAudioText,
payload = payload,
playAudio = playAudio,
telephonyTransferCall = telephonyTransferCall,
text = text,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy