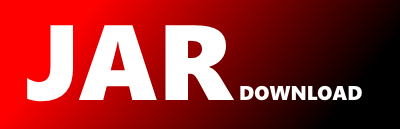
com.pulumi.gcp.diagflow.kotlin.inputs.CxPageFormParameterArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.diagflow.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.diagflow.inputs.CxPageFormParameterArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property advancedSettings Hierarchical advanced settings for this parameter. The settings exposed at the lower level overrides the settings exposed at the higher level.
* Hierarchy: Agent->Flow->Page->Fulfillment/Parameter.
* Structure is documented below.
* @property defaultValue The default value of an optional parameter. If the parameter is required, the default value will be ignored.
* @property displayName The human-readable name of the parameter, unique within the form.
* @property entityType The entity type of the parameter.
* Format: projects/-/locations/-/agents/-/entityTypes/ for system entity types (for example, projects/-/locations/-/agents/-/entityTypes/sys.date), or projects//locations//agents//entityTypes/ for developer entity types.
* @property fillBehavior Defines fill behavior for the parameter.
* Structure is documented below.
* @property isList Indicates whether the parameter represents a list of values.
* @property redact Indicates whether the parameter content should be redacted in log.
* If redaction is enabled, the parameter content will be replaced by parameter name during logging. Note: the parameter content is subject to redaction if either parameter level redaction or entity type level redaction is enabled.
* @property required Indicates whether the parameter is required. Optional parameters will not trigger prompts; however, they are filled if the user specifies them.
* Required parameters must be filled before form filling concludes.
*/
public data class CxPageFormParameterArgs(
public val advancedSettings: Output? = null,
public val defaultValue: Output? = null,
public val displayName: Output? = null,
public val entityType: Output? = null,
public val fillBehavior: Output? = null,
public val isList: Output? = null,
public val redact: Output? = null,
public val required: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.diagflow.inputs.CxPageFormParameterArgs =
com.pulumi.gcp.diagflow.inputs.CxPageFormParameterArgs.builder()
.advancedSettings(advancedSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.defaultValue(defaultValue?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.entityType(entityType?.applyValue({ args0 -> args0 }))
.fillBehavior(fillBehavior?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.isList(isList?.applyValue({ args0 -> args0 }))
.redact(redact?.applyValue({ args0 -> args0 }))
.required(required?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CxPageFormParameterArgs].
*/
@PulumiTagMarker
public class CxPageFormParameterArgsBuilder internal constructor() {
private var advancedSettings: Output? = null
private var defaultValue: Output? = null
private var displayName: Output? = null
private var entityType: Output? = null
private var fillBehavior: Output? = null
private var isList: Output? = null
private var redact: Output? = null
private var required: Output? = null
/**
* @param value Hierarchical advanced settings for this parameter. The settings exposed at the lower level overrides the settings exposed at the higher level.
* Hierarchy: Agent->Flow->Page->Fulfillment/Parameter.
* Structure is documented below.
*/
@JvmName("lgisbfcckypgsdyn")
public suspend fun advancedSettings(`value`: Output) {
this.advancedSettings = value
}
/**
* @param value The default value of an optional parameter. If the parameter is required, the default value will be ignored.
*/
@JvmName("rghjbkhdyqvtmbdu")
public suspend fun defaultValue(`value`: Output) {
this.defaultValue = value
}
/**
* @param value The human-readable name of the parameter, unique within the form.
*/
@JvmName("pvvlkfomyehhqtjo")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value The entity type of the parameter.
* Format: projects/-/locations/-/agents/-/entityTypes/ for system entity types (for example, projects/-/locations/-/agents/-/entityTypes/sys.date), or projects//locations//agents//entityTypes/ for developer entity types.
*/
@JvmName("cekpoutqmscmyqlh")
public suspend fun entityType(`value`: Output) {
this.entityType = value
}
/**
* @param value Defines fill behavior for the parameter.
* Structure is documented below.
*/
@JvmName("hbayblvwpdrphhor")
public suspend fun fillBehavior(`value`: Output) {
this.fillBehavior = value
}
/**
* @param value Indicates whether the parameter represents a list of values.
*/
@JvmName("usquuxlosdpfwbbr")
public suspend fun isList(`value`: Output) {
this.isList = value
}
/**
* @param value Indicates whether the parameter content should be redacted in log.
* If redaction is enabled, the parameter content will be replaced by parameter name during logging. Note: the parameter content is subject to redaction if either parameter level redaction or entity type level redaction is enabled.
*/
@JvmName("wmwsuvkkilexqgjj")
public suspend fun redact(`value`: Output) {
this.redact = value
}
/**
* @param value Indicates whether the parameter is required. Optional parameters will not trigger prompts; however, they are filled if the user specifies them.
* Required parameters must be filled before form filling concludes.
*/
@JvmName("owirxmgypxdqeyed")
public suspend fun required(`value`: Output) {
this.required = value
}
/**
* @param value Hierarchical advanced settings for this parameter. The settings exposed at the lower level overrides the settings exposed at the higher level.
* Hierarchy: Agent->Flow->Page->Fulfillment/Parameter.
* Structure is documented below.
*/
@JvmName("uksqdgopcvojanbp")
public suspend fun advancedSettings(`value`: CxPageFormParameterAdvancedSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.advancedSettings = mapped
}
/**
* @param argument Hierarchical advanced settings for this parameter. The settings exposed at the lower level overrides the settings exposed at the higher level.
* Hierarchy: Agent->Flow->Page->Fulfillment/Parameter.
* Structure is documented below.
*/
@JvmName("unvpdfenmtltjhfs")
public suspend fun advancedSettings(argument: suspend CxPageFormParameterAdvancedSettingsArgsBuilder.() -> Unit) {
val toBeMapped = CxPageFormParameterAdvancedSettingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.advancedSettings = mapped
}
/**
* @param value The default value of an optional parameter. If the parameter is required, the default value will be ignored.
*/
@JvmName("lvkmruvxuuvccftv")
public suspend fun defaultValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultValue = mapped
}
/**
* @param value The human-readable name of the parameter, unique within the form.
*/
@JvmName("tcitaspiocfqvcgw")
public suspend fun displayName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayName = mapped
}
/**
* @param value The entity type of the parameter.
* Format: projects/-/locations/-/agents/-/entityTypes/ for system entity types (for example, projects/-/locations/-/agents/-/entityTypes/sys.date), or projects//locations//agents//entityTypes/ for developer entity types.
*/
@JvmName("fjemugdadipfyrkm")
public suspend fun entityType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.entityType = mapped
}
/**
* @param value Defines fill behavior for the parameter.
* Structure is documented below.
*/
@JvmName("equwooyutrsbhuic")
public suspend fun fillBehavior(`value`: CxPageFormParameterFillBehaviorArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fillBehavior = mapped
}
/**
* @param argument Defines fill behavior for the parameter.
* Structure is documented below.
*/
@JvmName("glhqupsovfyjbcul")
public suspend fun fillBehavior(argument: suspend CxPageFormParameterFillBehaviorArgsBuilder.() -> Unit) {
val toBeMapped = CxPageFormParameterFillBehaviorArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.fillBehavior = mapped
}
/**
* @param value Indicates whether the parameter represents a list of values.
*/
@JvmName("hdtonkbqwiewheiq")
public suspend fun isList(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.isList = mapped
}
/**
* @param value Indicates whether the parameter content should be redacted in log.
* If redaction is enabled, the parameter content will be replaced by parameter name during logging. Note: the parameter content is subject to redaction if either parameter level redaction or entity type level redaction is enabled.
*/
@JvmName("oqtfogurhusnyfwq")
public suspend fun redact(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.redact = mapped
}
/**
* @param value Indicates whether the parameter is required. Optional parameters will not trigger prompts; however, they are filled if the user specifies them.
* Required parameters must be filled before form filling concludes.
*/
@JvmName("rfhqbknfcrnnvcat")
public suspend fun required(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.required = mapped
}
internal fun build(): CxPageFormParameterArgs = CxPageFormParameterArgs(
advancedSettings = advancedSettings,
defaultValue = defaultValue,
displayName = displayName,
entityType = entityType,
fillBehavior = fillBehavior,
isList = isList,
redact = redact,
required = required,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy