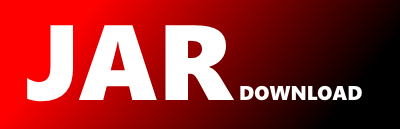
com.pulumi.gcp.diagflow.kotlin.inputs.CxPageTransitionRouteArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.diagflow.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.diagflow.inputs.CxPageTransitionRouteArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property condition The condition to evaluate against form parameters or session parameters.
* At least one of intent or condition must be specified. When both intent and condition are specified, the transition can only happen when both are fulfilled.
* @property intent The unique identifier of an Intent.
* Format: projects//locations//agents//intents/. Indicates that the transition can only happen when the given intent is matched. At least one of intent or condition must be specified. When both intent and condition are specified, the transition can only happen when both are fulfilled.
* @property name (Output)
* The unique identifier of this transition route.
* @property targetFlow The target flow to transition to.
* Format: projects//locations//agents//flows/.
* @property targetPage The target page to transition to.
* Format: projects//locations//agents//flows//pages/.
* @property triggerFulfillment The fulfillment to call when the condition is satisfied. At least one of triggerFulfillment and target must be specified. When both are defined, triggerFulfillment is executed first.
* Structure is documented below.
*/
public data class CxPageTransitionRouteArgs(
public val condition: Output? = null,
public val intent: Output? = null,
public val name: Output? = null,
public val targetFlow: Output? = null,
public val targetPage: Output? = null,
public val triggerFulfillment: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.diagflow.inputs.CxPageTransitionRouteArgs =
com.pulumi.gcp.diagflow.inputs.CxPageTransitionRouteArgs.builder()
.condition(condition?.applyValue({ args0 -> args0 }))
.intent(intent?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.targetFlow(targetFlow?.applyValue({ args0 -> args0 }))
.targetPage(targetPage?.applyValue({ args0 -> args0 }))
.triggerFulfillment(
triggerFulfillment?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [CxPageTransitionRouteArgs].
*/
@PulumiTagMarker
public class CxPageTransitionRouteArgsBuilder internal constructor() {
private var condition: Output? = null
private var intent: Output? = null
private var name: Output? = null
private var targetFlow: Output? = null
private var targetPage: Output? = null
private var triggerFulfillment: Output? = null
/**
* @param value The condition to evaluate against form parameters or session parameters.
* At least one of intent or condition must be specified. When both intent and condition are specified, the transition can only happen when both are fulfilled.
*/
@JvmName("mrmsyntygvievvnv")
public suspend fun condition(`value`: Output) {
this.condition = value
}
/**
* @param value The unique identifier of an Intent.
* Format: projects//locations//agents//intents/. Indicates that the transition can only happen when the given intent is matched. At least one of intent or condition must be specified. When both intent and condition are specified, the transition can only happen when both are fulfilled.
*/
@JvmName("nvqrhebafxlrahti")
public suspend fun intent(`value`: Output) {
this.intent = value
}
/**
* @param value (Output)
* The unique identifier of this transition route.
*/
@JvmName("uwgrhiskxfebwjvd")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The target flow to transition to.
* Format: projects//locations//agents//flows/.
*/
@JvmName("cqccbbwsrkchsdpv")
public suspend fun targetFlow(`value`: Output) {
this.targetFlow = value
}
/**
* @param value The target page to transition to.
* Format: projects//locations//agents//flows//pages/.
*/
@JvmName("axtkliwttdipdxly")
public suspend fun targetPage(`value`: Output) {
this.targetPage = value
}
/**
* @param value The fulfillment to call when the condition is satisfied. At least one of triggerFulfillment and target must be specified. When both are defined, triggerFulfillment is executed first.
* Structure is documented below.
*/
@JvmName("guujhobiwdwacbcy")
public suspend fun triggerFulfillment(`value`: Output) {
this.triggerFulfillment = value
}
/**
* @param value The condition to evaluate against form parameters or session parameters.
* At least one of intent or condition must be specified. When both intent and condition are specified, the transition can only happen when both are fulfilled.
*/
@JvmName("humrqcxbcmaihkfw")
public suspend fun condition(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.condition = mapped
}
/**
* @param value The unique identifier of an Intent.
* Format: projects//locations//agents//intents/. Indicates that the transition can only happen when the given intent is matched. At least one of intent or condition must be specified. When both intent and condition are specified, the transition can only happen when both are fulfilled.
*/
@JvmName("exaagtplecldoyxt")
public suspend fun intent(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.intent = mapped
}
/**
* @param value (Output)
* The unique identifier of this transition route.
*/
@JvmName("xqrpfpkelxfvfrpq")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The target flow to transition to.
* Format: projects//locations//agents//flows/.
*/
@JvmName("elaqijoelkymsjko")
public suspend fun targetFlow(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetFlow = mapped
}
/**
* @param value The target page to transition to.
* Format: projects//locations//agents//flows//pages/.
*/
@JvmName("ftealbovcogupqne")
public suspend fun targetPage(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetPage = mapped
}
/**
* @param value The fulfillment to call when the condition is satisfied. At least one of triggerFulfillment and target must be specified. When both are defined, triggerFulfillment is executed first.
* Structure is documented below.
*/
@JvmName("domtxepeevfvusyj")
public suspend fun triggerFulfillment(`value`: CxPageTransitionRouteTriggerFulfillmentArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.triggerFulfillment = mapped
}
/**
* @param argument The fulfillment to call when the condition is satisfied. At least one of triggerFulfillment and target must be specified. When both are defined, triggerFulfillment is executed first.
* Structure is documented below.
*/
@JvmName("qhfelofuotmavuju")
public suspend fun triggerFulfillment(argument: suspend CxPageTransitionRouteTriggerFulfillmentArgsBuilder.() -> Unit) {
val toBeMapped = CxPageTransitionRouteTriggerFulfillmentArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.triggerFulfillment = mapped
}
internal fun build(): CxPageTransitionRouteArgs = CxPageTransitionRouteArgs(
condition = condition,
intent = intent,
name = name,
targetFlow = targetFlow,
targetPage = targetPage,
triggerFulfillment = triggerFulfillment,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy