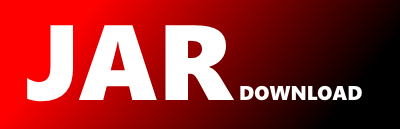
com.pulumi.gcp.diagflow.kotlin.inputs.CxTestCaseTestCaseConversationTurnUserInputInputArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.diagflow.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.diagflow.inputs.CxTestCaseTestCaseConversationTurnUserInputInputArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property dtmf The DTMF event to be handled.
* Structure is documented below.
* @property event The event to be triggered.
* Structure is documented below.
* @property languageCode The language of the input. See [Language Support](https://cloud.google.com/dialogflow/cx/docs/reference/language) for a list of the currently supported language codes.
* Note that queries in the same session do not necessarily need to specify the same language.
* @property text The natural language text to be processed.
* Structure is documented below.
*/
public data class CxTestCaseTestCaseConversationTurnUserInputInputArgs(
public val dtmf: Output? = null,
public val event: Output? = null,
public val languageCode: Output? = null,
public val text: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.diagflow.inputs.CxTestCaseTestCaseConversationTurnUserInputInputArgs =
com.pulumi.gcp.diagflow.inputs.CxTestCaseTestCaseConversationTurnUserInputInputArgs.builder()
.dtmf(dtmf?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.event(event?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.languageCode(languageCode?.applyValue({ args0 -> args0 }))
.text(text?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [CxTestCaseTestCaseConversationTurnUserInputInputArgs].
*/
@PulumiTagMarker
public class CxTestCaseTestCaseConversationTurnUserInputInputArgsBuilder internal constructor() {
private var dtmf: Output? = null
private var event: Output? = null
private var languageCode: Output? = null
private var text: Output? = null
/**
* @param value The DTMF event to be handled.
* Structure is documented below.
*/
@JvmName("ymgsflgrhruicnra")
public suspend fun dtmf(`value`: Output) {
this.dtmf = value
}
/**
* @param value The event to be triggered.
* Structure is documented below.
*/
@JvmName("dyefhrdusyjsclsn")
public suspend fun event(`value`: Output) {
this.event = value
}
/**
* @param value The language of the input. See [Language Support](https://cloud.google.com/dialogflow/cx/docs/reference/language) for a list of the currently supported language codes.
* Note that queries in the same session do not necessarily need to specify the same language.
*/
@JvmName("towyktlfykehvukr")
public suspend fun languageCode(`value`: Output) {
this.languageCode = value
}
/**
* @param value The natural language text to be processed.
* Structure is documented below.
*/
@JvmName("nxmpbvqfeffcvtlh")
public suspend fun text(`value`: Output) {
this.text = value
}
/**
* @param value The DTMF event to be handled.
* Structure is documented below.
*/
@JvmName("myxpqukwcqxxvesq")
public suspend fun dtmf(`value`: CxTestCaseTestCaseConversationTurnUserInputInputDtmfArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dtmf = mapped
}
/**
* @param argument The DTMF event to be handled.
* Structure is documented below.
*/
@JvmName("lctxwmwvvesqqaus")
public suspend fun dtmf(argument: suspend CxTestCaseTestCaseConversationTurnUserInputInputDtmfArgsBuilder.() -> Unit) {
val toBeMapped = CxTestCaseTestCaseConversationTurnUserInputInputDtmfArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dtmf = mapped
}
/**
* @param value The event to be triggered.
* Structure is documented below.
*/
@JvmName("lxxvoktcsqyfsgdh")
public suspend fun event(`value`: CxTestCaseTestCaseConversationTurnUserInputInputEventArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.event = mapped
}
/**
* @param argument The event to be triggered.
* Structure is documented below.
*/
@JvmName("mcupsfxnrjorfctr")
public suspend fun event(argument: suspend CxTestCaseTestCaseConversationTurnUserInputInputEventArgsBuilder.() -> Unit) {
val toBeMapped =
CxTestCaseTestCaseConversationTurnUserInputInputEventArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.event = mapped
}
/**
* @param value The language of the input. See [Language Support](https://cloud.google.com/dialogflow/cx/docs/reference/language) for a list of the currently supported language codes.
* Note that queries in the same session do not necessarily need to specify the same language.
*/
@JvmName("lctjfrbbaulrnlxn")
public suspend fun languageCode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.languageCode = mapped
}
/**
* @param value The natural language text to be processed.
* Structure is documented below.
*/
@JvmName("flnnculywlairfmo")
public suspend fun text(`value`: CxTestCaseTestCaseConversationTurnUserInputInputTextArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.text = mapped
}
/**
* @param argument The natural language text to be processed.
* Structure is documented below.
*/
@JvmName("iwlhmfijlavyjxtq")
public suspend fun text(argument: suspend CxTestCaseTestCaseConversationTurnUserInputInputTextArgsBuilder.() -> Unit) {
val toBeMapped = CxTestCaseTestCaseConversationTurnUserInputInputTextArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.text = mapped
}
internal fun build(): CxTestCaseTestCaseConversationTurnUserInputInputArgs =
CxTestCaseTestCaseConversationTurnUserInputInputArgs(
dtmf = dtmf,
event = event,
languageCode = languageCode,
text = text,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy