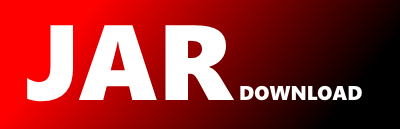
com.pulumi.gcp.dns.kotlin.inputs.ManagedZoneDnssecConfigDefaultKeySpecArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dns.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.dns.inputs.ManagedZoneDnssecConfigDefaultKeySpecArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property algorithm String mnemonic specifying the DNSSEC algorithm of this key
* Possible values are: `ecdsap256sha256`, `ecdsap384sha384`, `rsasha1`, `rsasha256`, `rsasha512`.
* @property keyLength Length of the keys in bits
* @property keyType Specifies whether this is a key signing key (KSK) or a zone
* signing key (ZSK). Key signing keys have the Secure Entry
* Point flag set and, when active, will only be used to sign
* resource record sets of type DNSKEY. Zone signing keys do
* not have the Secure Entry Point flag set and will be used
* to sign all other types of resource record sets.
* Possible values are: `keySigning`, `zoneSigning`.
* @property kind Identifies what kind of resource this is
*/
public data class ManagedZoneDnssecConfigDefaultKeySpecArgs(
public val algorithm: Output? = null,
public val keyLength: Output? = null,
public val keyType: Output? = null,
public val kind: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.dns.inputs.ManagedZoneDnssecConfigDefaultKeySpecArgs =
com.pulumi.gcp.dns.inputs.ManagedZoneDnssecConfigDefaultKeySpecArgs.builder()
.algorithm(algorithm?.applyValue({ args0 -> args0 }))
.keyLength(keyLength?.applyValue({ args0 -> args0 }))
.keyType(keyType?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ManagedZoneDnssecConfigDefaultKeySpecArgs].
*/
@PulumiTagMarker
public class ManagedZoneDnssecConfigDefaultKeySpecArgsBuilder internal constructor() {
private var algorithm: Output? = null
private var keyLength: Output? = null
private var keyType: Output? = null
private var kind: Output? = null
/**
* @param value String mnemonic specifying the DNSSEC algorithm of this key
* Possible values are: `ecdsap256sha256`, `ecdsap384sha384`, `rsasha1`, `rsasha256`, `rsasha512`.
*/
@JvmName("ojjlucvodoenvcpu")
public suspend fun algorithm(`value`: Output) {
this.algorithm = value
}
/**
* @param value Length of the keys in bits
*/
@JvmName("aecuuqsssrsjjxcc")
public suspend fun keyLength(`value`: Output) {
this.keyLength = value
}
/**
* @param value Specifies whether this is a key signing key (KSK) or a zone
* signing key (ZSK). Key signing keys have the Secure Entry
* Point flag set and, when active, will only be used to sign
* resource record sets of type DNSKEY. Zone signing keys do
* not have the Secure Entry Point flag set and will be used
* to sign all other types of resource record sets.
* Possible values are: `keySigning`, `zoneSigning`.
*/
@JvmName("qfojhkwiyxsbkpnr")
public suspend fun keyType(`value`: Output) {
this.keyType = value
}
/**
* @param value Identifies what kind of resource this is
*/
@JvmName("xfgghmuvmvhynbmt")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value String mnemonic specifying the DNSSEC algorithm of this key
* Possible values are: `ecdsap256sha256`, `ecdsap384sha384`, `rsasha1`, `rsasha256`, `rsasha512`.
*/
@JvmName("gyshgnndybbffadi")
public suspend fun algorithm(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.algorithm = mapped
}
/**
* @param value Length of the keys in bits
*/
@JvmName("dhhygymitmuwwqtm")
public suspend fun keyLength(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyLength = mapped
}
/**
* @param value Specifies whether this is a key signing key (KSK) or a zone
* signing key (ZSK). Key signing keys have the Secure Entry
* Point flag set and, when active, will only be used to sign
* resource record sets of type DNSKEY. Zone signing keys do
* not have the Secure Entry Point flag set and will be used
* to sign all other types of resource record sets.
* Possible values are: `keySigning`, `zoneSigning`.
*/
@JvmName("utcbcnqeumrrtpiv")
public suspend fun keyType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyType = mapped
}
/**
* @param value Identifies what kind of resource this is
*/
@JvmName("knpqhwqjbrdcakbi")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
internal fun build(): ManagedZoneDnssecConfigDefaultKeySpecArgs =
ManagedZoneDnssecConfigDefaultKeySpecArgs(
algorithm = algorithm,
keyLength = keyLength,
keyType = keyType,
kind = kind,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy