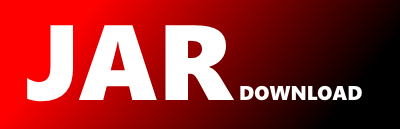
com.pulumi.gcp.edgecontainer.kotlin.inputs.ClusterControlPlaneEncryptionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.edgecontainer.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.edgecontainer.inputs.ClusterControlPlaneEncryptionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property kmsKey The Cloud KMS CryptoKey e.g.
* projects/{project}/locations/{location}/keyRings/{keyRing}/cryptoKeys/{cryptoKey}
* to use for protecting control plane disks. If not specified, a
* Google-managed key will be used instead.
* @property kmsKeyActiveVersion (Output)
* The Cloud KMS CryptoKeyVersion currently in use for protecting control
* plane disks. Only applicable if kms_key is set.
* @property kmsKeyState (Output)
* Availability of the Cloud KMS CryptoKey. If not `KEY_AVAILABLE`, then
* nodes may go offline as they cannot access their local data. This can be
* caused by a lack of permissions to use the key, or if the key is disabled
* or deleted.
* @property kmsStatuses (Output)
* Error status returned by Cloud KMS when using this key. This field may be
* populated only if `kms_key_state` is not `KMS_KEY_STATE_KEY_AVAILABLE`.
* If populated, this field contains the error status reported by Cloud KMS.
* Structure is documented below.
* The `kms_status` block contains:
*/
public data class ClusterControlPlaneEncryptionArgs(
public val kmsKey: Output? = null,
public val kmsKeyActiveVersion: Output? = null,
public val kmsKeyState: Output? = null,
public val kmsStatuses: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.edgecontainer.inputs.ClusterControlPlaneEncryptionArgs =
com.pulumi.gcp.edgecontainer.inputs.ClusterControlPlaneEncryptionArgs.builder()
.kmsKey(kmsKey?.applyValue({ args0 -> args0 }))
.kmsKeyActiveVersion(kmsKeyActiveVersion?.applyValue({ args0 -> args0 }))
.kmsKeyState(kmsKeyState?.applyValue({ args0 -> args0 }))
.kmsStatuses(
kmsStatuses?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [ClusterControlPlaneEncryptionArgs].
*/
@PulumiTagMarker
public class ClusterControlPlaneEncryptionArgsBuilder internal constructor() {
private var kmsKey: Output? = null
private var kmsKeyActiveVersion: Output? = null
private var kmsKeyState: Output? = null
private var kmsStatuses: Output>? = null
/**
* @param value The Cloud KMS CryptoKey e.g.
* projects/{project}/locations/{location}/keyRings/{keyRing}/cryptoKeys/{cryptoKey}
* to use for protecting control plane disks. If not specified, a
* Google-managed key will be used instead.
*/
@JvmName("qouqkrcatmxfwuii")
public suspend fun kmsKey(`value`: Output) {
this.kmsKey = value
}
/**
* @param value (Output)
* The Cloud KMS CryptoKeyVersion currently in use for protecting control
* plane disks. Only applicable if kms_key is set.
*/
@JvmName("uyymniugvtxvrbya")
public suspend fun kmsKeyActiveVersion(`value`: Output) {
this.kmsKeyActiveVersion = value
}
/**
* @param value (Output)
* Availability of the Cloud KMS CryptoKey. If not `KEY_AVAILABLE`, then
* nodes may go offline as they cannot access their local data. This can be
* caused by a lack of permissions to use the key, or if the key is disabled
* or deleted.
*/
@JvmName("htahlugeihbxgnop")
public suspend fun kmsKeyState(`value`: Output) {
this.kmsKeyState = value
}
/**
* @param value (Output)
* Error status returned by Cloud KMS when using this key. This field may be
* populated only if `kms_key_state` is not `KMS_KEY_STATE_KEY_AVAILABLE`.
* If populated, this field contains the error status reported by Cloud KMS.
* Structure is documented below.
* The `kms_status` block contains:
*/
@JvmName("ghervryitdlxuinr")
public suspend fun kmsStatuses(`value`: Output>) {
this.kmsStatuses = value
}
@JvmName("lqvtmdoiwdskmxvc")
public suspend fun kmsStatuses(vararg values: Output) {
this.kmsStatuses = Output.all(values.asList())
}
/**
* @param values (Output)
* Error status returned by Cloud KMS when using this key. This field may be
* populated only if `kms_key_state` is not `KMS_KEY_STATE_KEY_AVAILABLE`.
* If populated, this field contains the error status reported by Cloud KMS.
* Structure is documented below.
* The `kms_status` block contains:
*/
@JvmName("vmygaqngayhepulc")
public suspend fun kmsStatuses(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy