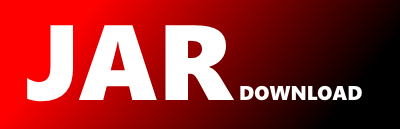
com.pulumi.gcp.endpoints.kotlin.ServiceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.endpoints.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.endpoints.ServiceArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* This resource creates and rolls out a Cloud Endpoints service using OpenAPI or gRPC. View the relevant docs for [OpenAPI](https://cloud.google.com/endpoints/docs/openapi/) and [gRPC](https://cloud.google.com/endpoints/docs/grpc/).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* import * as std from "@pulumi/std";
* const openapiService = new gcp.endpoints.Service("openapi_service", {
* serviceName: "api-name.endpoints.project-id.cloud.goog",
* project: "project-id",
* openapiConfig: std.file({
* input: "openapi_spec.yml",
* }).then(invoke => invoke.result),
* });
* const grpcService = new gcp.endpoints.Service("grpc_service", {
* serviceName: "api-name.endpoints.project-id.cloud.goog",
* project: "project-id",
* grpcConfig: std.file({
* input: "service_spec.yml",
* }).then(invoke => invoke.result),
* protocOutputBase64: std.filebase64({
* input: "compiled_descriptor_file.pb",
* }).then(invoke => invoke.result),
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* import pulumi_std as std
* openapi_service = gcp.endpoints.Service("openapi_service",
* service_name="api-name.endpoints.project-id.cloud.goog",
* project="project-id",
* openapi_config=std.file(input="openapi_spec.yml").result)
* grpc_service = gcp.endpoints.Service("grpc_service",
* service_name="api-name.endpoints.project-id.cloud.goog",
* project="project-id",
* grpc_config=std.file(input="service_spec.yml").result,
* protoc_output_base64=std.filebase64(input="compiled_descriptor_file.pb").result)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* using Std = Pulumi.Std;
* return await Deployment.RunAsync(() =>
* {
* var openapiService = new Gcp.Endpoints.Service("openapi_service", new()
* {
* ServiceName = "api-name.endpoints.project-id.cloud.goog",
* Project = "project-id",
* OpenapiConfig = Std.File.Invoke(new()
* {
* Input = "openapi_spec.yml",
* }).Apply(invoke => invoke.Result),
* });
* var grpcService = new Gcp.Endpoints.Service("grpc_service", new()
* {
* ServiceName = "api-name.endpoints.project-id.cloud.goog",
* Project = "project-id",
* GrpcConfig = Std.File.Invoke(new()
* {
* Input = "service_spec.yml",
* }).Apply(invoke => invoke.Result),
* ProtocOutputBase64 = Std.Filebase64.Invoke(new()
* {
* Input = "compiled_descriptor_file.pb",
* }).Apply(invoke => invoke.Result),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/endpoints"
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* invokeFile, err := std.File(ctx, &std.FileArgs{
* Input: "openapi_spec.yml",
* }, nil)
* if err != nil {
* return err
* }
* _, err = endpoints.NewService(ctx, "openapi_service", &endpoints.ServiceArgs{
* ServiceName: pulumi.String("api-name.endpoints.project-id.cloud.goog"),
* Project: pulumi.String("project-id"),
* OpenapiConfig: invokeFile.Result,
* })
* if err != nil {
* return err
* }
* invokeFile1, err := std.File(ctx, &std.FileArgs{
* Input: "service_spec.yml",
* }, nil)
* if err != nil {
* return err
* }
* invokeFilebase642, err := std.Filebase64(ctx, &std.Filebase64Args{
* Input: "compiled_descriptor_file.pb",
* }, nil)
* if err != nil {
* return err
* }
* _, err = endpoints.NewService(ctx, "grpc_service", &endpoints.ServiceArgs{
* ServiceName: pulumi.String("api-name.endpoints.project-id.cloud.goog"),
* Project: pulumi.String("project-id"),
* GrpcConfig: invokeFile1.Result,
* ProtocOutputBase64: invokeFilebase642.Result,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.endpoints.Service;
* import com.pulumi.gcp.endpoints.ServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var openapiService = new Service("openapiService", ServiceArgs.builder()
* .serviceName("api-name.endpoints.project-id.cloud.goog")
* .project("project-id")
* .openapiConfig(StdFunctions.file(FileArgs.builder()
* .input("openapi_spec.yml")
* .build()).result())
* .build());
* var grpcService = new Service("grpcService", ServiceArgs.builder()
* .serviceName("api-name.endpoints.project-id.cloud.goog")
* .project("project-id")
* .grpcConfig(StdFunctions.file(FileArgs.builder()
* .input("service_spec.yml")
* .build()).result())
* .protocOutputBase64(StdFunctions.filebase64(Filebase64Args.builder()
* .input("compiled_descriptor_file.pb")
* .build()).result())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* openapiService:
* type: gcp:endpoints:Service
* name: openapi_service
* properties:
* serviceName: api-name.endpoints.project-id.cloud.goog
* project: project-id
* openapiConfig:
* fn::invoke:
* Function: std:file
* Arguments:
* input: openapi_spec.yml
* Return: result
* grpcService:
* type: gcp:endpoints:Service
* name: grpc_service
* properties:
* serviceName: api-name.endpoints.project-id.cloud.goog
* project: project-id
* grpcConfig:
* fn::invoke:
* Function: std:file
* Arguments:
* input: service_spec.yml
* Return: result
* protocOutputBase64:
* fn::invoke:
* Function: std:filebase64
* Arguments:
* input: compiled_descriptor_file.pb
* Return: result
* ```
*
* The example in `examples/endpoints_on_compute_engine` shows the API from the quickstart running on a Compute Engine VM and reachable through Cloud Endpoints, which may also be useful.
* ## Import
* This resource does not support import.
* @property grpcConfig The full text of the Service Config YAML file (Example located [here](https://github.com/GoogleCloudPlatform/python-docs-samples/blob/main/endpoints/bookstore-grpc/api_config.yaml)).
* If provided, must also provide `protoc_output_base64`. `open_api` config must *not* be provided.
* @property openapiConfig The full text of the OpenAPI YAML configuration as described [here](https://github.com/OAI/OpenAPI-Specification/blob/main/versions/2.0.md).
* Either this, or *both* of `grpc_config` and `protoc_output_base64` must be specified.
* @property project The project ID that the service belongs to. If not provided, provider project is used.
* @property protocOutputBase64 The full contents of the Service Descriptor File generated by protoc. This should be a compiled .pb file, base64-encoded.
* @property serviceName The name of the service. Usually of the form `$apiname.endpoints.$projectid.cloud.goog`.
* - - -
*/
public data class ServiceArgs(
public val grpcConfig: Output? = null,
public val openapiConfig: Output? = null,
public val project: Output? = null,
public val protocOutputBase64: Output? = null,
public val serviceName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.endpoints.ServiceArgs =
com.pulumi.gcp.endpoints.ServiceArgs.builder()
.grpcConfig(grpcConfig?.applyValue({ args0 -> args0 }))
.openapiConfig(openapiConfig?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.protocOutputBase64(protocOutputBase64?.applyValue({ args0 -> args0 }))
.serviceName(serviceName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServiceArgs].
*/
@PulumiTagMarker
public class ServiceArgsBuilder internal constructor() {
private var grpcConfig: Output? = null
private var openapiConfig: Output? = null
private var project: Output? = null
private var protocOutputBase64: Output? = null
private var serviceName: Output? = null
/**
* @param value The full text of the Service Config YAML file (Example located [here](https://github.com/GoogleCloudPlatform/python-docs-samples/blob/main/endpoints/bookstore-grpc/api_config.yaml)).
* If provided, must also provide `protoc_output_base64`. `open_api` config must *not* be provided.
*/
@JvmName("lepjmhwtrvtebkwu")
public suspend fun grpcConfig(`value`: Output) {
this.grpcConfig = value
}
/**
* @param value The full text of the OpenAPI YAML configuration as described [here](https://github.com/OAI/OpenAPI-Specification/blob/main/versions/2.0.md).
* Either this, or *both* of `grpc_config` and `protoc_output_base64` must be specified.
*/
@JvmName("pdorsggletkpxduh")
public suspend fun openapiConfig(`value`: Output) {
this.openapiConfig = value
}
/**
* @param value The project ID that the service belongs to. If not provided, provider project is used.
*/
@JvmName("fwunkbtkfdbirrhs")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The full contents of the Service Descriptor File generated by protoc. This should be a compiled .pb file, base64-encoded.
*/
@JvmName("mqdopqfqhpvqciqs")
public suspend fun protocOutputBase64(`value`: Output) {
this.protocOutputBase64 = value
}
/**
* @param value The name of the service. Usually of the form `$apiname.endpoints.$projectid.cloud.goog`.
* - - -
*/
@JvmName("ahkjojwfcbojgbqx")
public suspend fun serviceName(`value`: Output) {
this.serviceName = value
}
/**
* @param value The full text of the Service Config YAML file (Example located [here](https://github.com/GoogleCloudPlatform/python-docs-samples/blob/main/endpoints/bookstore-grpc/api_config.yaml)).
* If provided, must also provide `protoc_output_base64`. `open_api` config must *not* be provided.
*/
@JvmName("ngufwjauknkdnmjn")
public suspend fun grpcConfig(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.grpcConfig = mapped
}
/**
* @param value The full text of the OpenAPI YAML configuration as described [here](https://github.com/OAI/OpenAPI-Specification/blob/main/versions/2.0.md).
* Either this, or *both* of `grpc_config` and `protoc_output_base64` must be specified.
*/
@JvmName("jhkhaevxqgnuhras")
public suspend fun openapiConfig(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.openapiConfig = mapped
}
/**
* @param value The project ID that the service belongs to. If not provided, provider project is used.
*/
@JvmName("srfaspbglwjkeway")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value The full contents of the Service Descriptor File generated by protoc. This should be a compiled .pb file, base64-encoded.
*/
@JvmName("oijffihscwuxlenb")
public suspend fun protocOutputBase64(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.protocOutputBase64 = mapped
}
/**
* @param value The name of the service. Usually of the form `$apiname.endpoints.$projectid.cloud.goog`.
* - - -
*/
@JvmName("ymtkmbnlavnrsecp")
public suspend fun serviceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceName = mapped
}
internal fun build(): ServiceArgs = ServiceArgs(
grpcConfig = grpcConfig,
openapiConfig = openapiConfig,
project = project,
protocOutputBase64 = protocOutputBase64,
serviceName = serviceName,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy