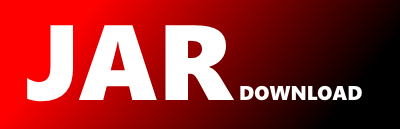
com.pulumi.gcp.essentialcontacts.kotlin.DocumentAiWarehouseLocationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.essentialcontacts.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.essentialcontacts.DocumentAiWarehouseLocationArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A location is used to initialize a project.
* To get more information about Location, see:
* * [API documentation](https://cloud.google.com/document-warehouse/docs/reference/rest/v1/projects.locations)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/document-warehouse/docs/overview)
* ## Example Usage
* ### Document Ai Warehouse Location
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const project = gcp.organizations.getProject({});
* const example = new gcp.essentialcontacts.DocumentAiWarehouseLocation("example", {
* location: "us",
* projectNumber: project.then(project => project.number),
* accessControlMode: "ACL_MODE_DOCUMENT_LEVEL_ACCESS_CONTROL_GCI",
* databaseType: "DB_INFRA_SPANNER",
* kmsKey: "dummy_key",
* documentCreatorDefaultRole: "DOCUMENT_ADMIN",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* project = gcp.organizations.get_project()
* example = gcp.essentialcontacts.DocumentAiWarehouseLocation("example",
* location="us",
* project_number=project.number,
* access_control_mode="ACL_MODE_DOCUMENT_LEVEL_ACCESS_CONTROL_GCI",
* database_type="DB_INFRA_SPANNER",
* kms_key="dummy_key",
* document_creator_default_role="DOCUMENT_ADMIN")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var project = Gcp.Organizations.GetProject.Invoke();
* var example = new Gcp.EssentialContacts.DocumentAiWarehouseLocation("example", new()
* {
* Location = "us",
* ProjectNumber = project.Apply(getProjectResult => getProjectResult.Number),
* AccessControlMode = "ACL_MODE_DOCUMENT_LEVEL_ACCESS_CONTROL_GCI",
* DatabaseType = "DB_INFRA_SPANNER",
* KmsKey = "dummy_key",
* DocumentCreatorDefaultRole = "DOCUMENT_ADMIN",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/essentialcontacts"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = essentialcontacts.NewDocumentAiWarehouseLocation(ctx, "example", &essentialcontacts.DocumentAiWarehouseLocationArgs{
* Location: pulumi.String("us"),
* ProjectNumber: pulumi.String(project.Number),
* AccessControlMode: pulumi.String("ACL_MODE_DOCUMENT_LEVEL_ACCESS_CONTROL_GCI"),
* DatabaseType: pulumi.String("DB_INFRA_SPANNER"),
* KmsKey: pulumi.String("dummy_key"),
* DocumentCreatorDefaultRole: pulumi.String("DOCUMENT_ADMIN"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.essentialcontacts.DocumentAiWarehouseLocation;
* import com.pulumi.gcp.essentialcontacts.DocumentAiWarehouseLocationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var project = OrganizationsFunctions.getProject();
* var example = new DocumentAiWarehouseLocation("example", DocumentAiWarehouseLocationArgs.builder()
* .location("us")
* .projectNumber(project.applyValue(getProjectResult -> getProjectResult.number()))
* .accessControlMode("ACL_MODE_DOCUMENT_LEVEL_ACCESS_CONTROL_GCI")
* .databaseType("DB_INFRA_SPANNER")
* .kmsKey("dummy_key")
* .documentCreatorDefaultRole("DOCUMENT_ADMIN")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gcp:essentialcontacts:DocumentAiWarehouseLocation
* properties:
* location: us
* projectNumber: ${project.number}
* accessControlMode: ACL_MODE_DOCUMENT_LEVEL_ACCESS_CONTROL_GCI
* databaseType: DB_INFRA_SPANNER
* kmsKey: dummy_key
* documentCreatorDefaultRole: DOCUMENT_ADMIN
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ## Import
* This resource does not support import.
* @property accessControlMode The access control mode for accessing the customer data.
* Possible values are: `ACL_MODE_DOCUMENT_LEVEL_ACCESS_CONTROL_GCI`, `ACL_MODE_DOCUMENT_LEVEL_ACCESS_CONTROL_BYOID`, `ACL_MODE_UNIVERSAL_ACCESS`.
* @property databaseType The type of database used to store customer data.
* Possible values are: `DB_INFRA_SPANNER`, `DB_CLOUD_SQL_POSTGRES`.
* @property documentCreatorDefaultRole The default role for the person who create a document.
* Possible values are: `DOCUMENT_ADMIN`, `DOCUMENT_EDITOR`, `DOCUMENT_VIEWER`.
* @property kmsKey The KMS key used for CMEK encryption. It is required that
* the kms key is in the same region as the endpoint. The
* same key will be used for all provisioned resources, if
* encryption is available. If the kmsKey is left empty, no
* encryption will be enforced.
* @property location The location in which the instance is to be provisioned. It takes the form projects/{projectNumber}/locations/{location}.
* - - -
* @property projectNumber The unique identifier of the project.
*/
public data class DocumentAiWarehouseLocationArgs(
public val accessControlMode: Output? = null,
public val databaseType: Output? = null,
public val documentCreatorDefaultRole: Output? = null,
public val kmsKey: Output? = null,
public val location: Output? = null,
public val projectNumber: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.essentialcontacts.DocumentAiWarehouseLocationArgs =
com.pulumi.gcp.essentialcontacts.DocumentAiWarehouseLocationArgs.builder()
.accessControlMode(accessControlMode?.applyValue({ args0 -> args0 }))
.databaseType(databaseType?.applyValue({ args0 -> args0 }))
.documentCreatorDefaultRole(documentCreatorDefaultRole?.applyValue({ args0 -> args0 }))
.kmsKey(kmsKey?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.projectNumber(projectNumber?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DocumentAiWarehouseLocationArgs].
*/
@PulumiTagMarker
public class DocumentAiWarehouseLocationArgsBuilder internal constructor() {
private var accessControlMode: Output? = null
private var databaseType: Output? = null
private var documentCreatorDefaultRole: Output? = null
private var kmsKey: Output? = null
private var location: Output? = null
private var projectNumber: Output? = null
/**
* @param value The access control mode for accessing the customer data.
* Possible values are: `ACL_MODE_DOCUMENT_LEVEL_ACCESS_CONTROL_GCI`, `ACL_MODE_DOCUMENT_LEVEL_ACCESS_CONTROL_BYOID`, `ACL_MODE_UNIVERSAL_ACCESS`.
*/
@JvmName("kxrixrenjotqvvle")
public suspend fun accessControlMode(`value`: Output) {
this.accessControlMode = value
}
/**
* @param value The type of database used to store customer data.
* Possible values are: `DB_INFRA_SPANNER`, `DB_CLOUD_SQL_POSTGRES`.
*/
@JvmName("lvrytxyfeqiagpat")
public suspend fun databaseType(`value`: Output) {
this.databaseType = value
}
/**
* @param value The default role for the person who create a document.
* Possible values are: `DOCUMENT_ADMIN`, `DOCUMENT_EDITOR`, `DOCUMENT_VIEWER`.
*/
@JvmName("kjgvacrgogeyhqsl")
public suspend fun documentCreatorDefaultRole(`value`: Output) {
this.documentCreatorDefaultRole = value
}
/**
* @param value The KMS key used for CMEK encryption. It is required that
* the kms key is in the same region as the endpoint. The
* same key will be used for all provisioned resources, if
* encryption is available. If the kmsKey is left empty, no
* encryption will be enforced.
*/
@JvmName("qolfpjsyffnrmvmv")
public suspend fun kmsKey(`value`: Output) {
this.kmsKey = value
}
/**
* @param value The location in which the instance is to be provisioned. It takes the form projects/{projectNumber}/locations/{location}.
* - - -
*/
@JvmName("tadoawaqphcbaisg")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value The unique identifier of the project.
*/
@JvmName("whyhbwgwtylpvpdv")
public suspend fun projectNumber(`value`: Output) {
this.projectNumber = value
}
/**
* @param value The access control mode for accessing the customer data.
* Possible values are: `ACL_MODE_DOCUMENT_LEVEL_ACCESS_CONTROL_GCI`, `ACL_MODE_DOCUMENT_LEVEL_ACCESS_CONTROL_BYOID`, `ACL_MODE_UNIVERSAL_ACCESS`.
*/
@JvmName("ssugnwonfhcccbso")
public suspend fun accessControlMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accessControlMode = mapped
}
/**
* @param value The type of database used to store customer data.
* Possible values are: `DB_INFRA_SPANNER`, `DB_CLOUD_SQL_POSTGRES`.
*/
@JvmName("afvvfbuxnodyoqxd")
public suspend fun databaseType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.databaseType = mapped
}
/**
* @param value The default role for the person who create a document.
* Possible values are: `DOCUMENT_ADMIN`, `DOCUMENT_EDITOR`, `DOCUMENT_VIEWER`.
*/
@JvmName("ajdvuxvouogqfegj")
public suspend fun documentCreatorDefaultRole(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.documentCreatorDefaultRole = mapped
}
/**
* @param value The KMS key used for CMEK encryption. It is required that
* the kms key is in the same region as the endpoint. The
* same key will be used for all provisioned resources, if
* encryption is available. If the kmsKey is left empty, no
* encryption will be enforced.
*/
@JvmName("ehauxurvpnoyoyqx")
public suspend fun kmsKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsKey = mapped
}
/**
* @param value The location in which the instance is to be provisioned. It takes the form projects/{projectNumber}/locations/{location}.
* - - -
*/
@JvmName("ihmrfdbgumpadfeq")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value The unique identifier of the project.
*/
@JvmName("npcpvdbcptfcumdt")
public suspend fun projectNumber(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.projectNumber = mapped
}
internal fun build(): DocumentAiWarehouseLocationArgs = DocumentAiWarehouseLocationArgs(
accessControlMode = accessControlMode,
databaseType = databaseType,
documentCreatorDefaultRole = documentCreatorDefaultRole,
kmsKey = kmsKey,
location = location,
projectNumber = projectNumber,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy