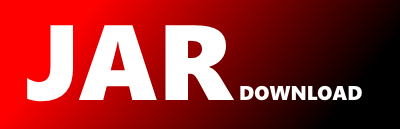
com.pulumi.gcp.essentialcontacts.kotlin.inputs.DocumentAiWarehouseDocumentSchemaPropertyDefinitionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.essentialcontacts.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.essentialcontacts.inputs.DocumentAiWarehouseDocumentSchemaPropertyDefinitionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property dateTimeTypeOptions Date time property. Not supported by CMEK compliant deployment.
* @property displayName The display-name for the property, used for front-end.
* @property enumTypeOptions Enum/categorical property.
* Structure is documented below.
* @property floatTypeOptions Float property.
* @property integerTypeOptions Integer property.
* @property isFilterable Whether the property can be filtered. If this is a sub-property, all the parent properties must be marked filterable.
* @property isMetadata Whether the property is user supplied metadata.
* @property isRepeatable Whether the property can have multiple values.
* @property isRequired Whether the property is mandatory.
* @property isSearchable Indicates that the property should be included in a global search.
* @property mapTypeOptions Map property.
* @property name The name of the metadata property.
* @property propertyTypeOptions Nested structured data property.
* Structure is documented below.
* @property retrievalImportance Stores the retrieval importance.
* Possible values are: `HIGHEST`, `HIGHER`, `HIGH`, `MEDIUM`, `LOW`, `LOWEST`.
* @property schemaSources The schema source information.
* Structure is documented below.
* @property textTypeOptions Text property.
* @property timestampTypeOptions Timestamp property. Not supported by CMEK compliant deployment.
*/
public data class DocumentAiWarehouseDocumentSchemaPropertyDefinitionArgs(
public val dateTimeTypeOptions: Output? = null,
public val displayName: Output? = null,
public val enumTypeOptions: Output? = null,
public val floatTypeOptions: Output? = null,
public val integerTypeOptions: Output? = null,
public val isFilterable: Output? = null,
public val isMetadata: Output? = null,
public val isRepeatable: Output? = null,
public val isRequired: Output? = null,
public val isSearchable: Output? = null,
public val mapTypeOptions: Output? = null,
public val name: Output,
public val propertyTypeOptions: Output? = null,
public val retrievalImportance: Output? = null,
public val schemaSources: Output>? = null,
public val textTypeOptions: Output? = null,
public val timestampTypeOptions: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.essentialcontacts.inputs.DocumentAiWarehouseDocumentSchemaPropertyDefinitionArgs =
com.pulumi.gcp.essentialcontacts.inputs.DocumentAiWarehouseDocumentSchemaPropertyDefinitionArgs.builder()
.dateTimeTypeOptions(
dateTimeTypeOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.displayName(displayName?.applyValue({ args0 -> args0 }))
.enumTypeOptions(enumTypeOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.floatTypeOptions(floatTypeOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.integerTypeOptions(
integerTypeOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.isFilterable(isFilterable?.applyValue({ args0 -> args0 }))
.isMetadata(isMetadata?.applyValue({ args0 -> args0 }))
.isRepeatable(isRepeatable?.applyValue({ args0 -> args0 }))
.isRequired(isRequired?.applyValue({ args0 -> args0 }))
.isSearchable(isSearchable?.applyValue({ args0 -> args0 }))
.mapTypeOptions(mapTypeOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name.applyValue({ args0 -> args0 }))
.propertyTypeOptions(
propertyTypeOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.retrievalImportance(retrievalImportance?.applyValue({ args0 -> args0 }))
.schemaSources(
schemaSources?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.textTypeOptions(textTypeOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.timestampTypeOptions(
timestampTypeOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [DocumentAiWarehouseDocumentSchemaPropertyDefinitionArgs].
*/
@PulumiTagMarker
public class DocumentAiWarehouseDocumentSchemaPropertyDefinitionArgsBuilder internal constructor() {
private var dateTimeTypeOptions:
Output? = null
private var displayName: Output? = null
private var enumTypeOptions:
Output? = null
private var floatTypeOptions:
Output? = null
private var integerTypeOptions:
Output? = null
private var isFilterable: Output? = null
private var isMetadata: Output? = null
private var isRepeatable: Output? = null
private var isRequired: Output? = null
private var isSearchable: Output? = null
private var mapTypeOptions:
Output? = null
private var name: Output? = null
private var propertyTypeOptions:
Output? = null
private var retrievalImportance: Output? = null
private var schemaSources:
Output>? = null
private var textTypeOptions:
Output? = null
private var timestampTypeOptions:
Output? = null
/**
* @param value Date time property. Not supported by CMEK compliant deployment.
*/
@JvmName("kjdwbfuyefxmsrch")
public suspend fun dateTimeTypeOptions(`value`: Output) {
this.dateTimeTypeOptions = value
}
/**
* @param value The display-name for the property, used for front-end.
*/
@JvmName("alhkxsrpysrdneym")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value Enum/categorical property.
* Structure is documented below.
*/
@JvmName("mpvobkwrcwtmjbiu")
public suspend fun enumTypeOptions(`value`: Output) {
this.enumTypeOptions = value
}
/**
* @param value Float property.
*/
@JvmName("hyyhgsynjtyjntyh")
public suspend fun floatTypeOptions(`value`: Output) {
this.floatTypeOptions = value
}
/**
* @param value Integer property.
*/
@JvmName("boavjpogjpgejlec")
public suspend fun integerTypeOptions(`value`: Output) {
this.integerTypeOptions = value
}
/**
* @param value Whether the property can be filtered. If this is a sub-property, all the parent properties must be marked filterable.
*/
@JvmName("ghtmjnfhwnaxkdek")
public suspend fun isFilterable(`value`: Output) {
this.isFilterable = value
}
/**
* @param value Whether the property is user supplied metadata.
*/
@JvmName("lutgyaqfprtbmqxu")
public suspend fun isMetadata(`value`: Output) {
this.isMetadata = value
}
/**
* @param value Whether the property can have multiple values.
*/
@JvmName("jwukiqufbyaqnppb")
public suspend fun isRepeatable(`value`: Output) {
this.isRepeatable = value
}
/**
* @param value Whether the property is mandatory.
*/
@JvmName("mggqiymfuiwjhrce")
public suspend fun isRequired(`value`: Output) {
this.isRequired = value
}
/**
* @param value Indicates that the property should be included in a global search.
*/
@JvmName("xsosmrfqagdyxlts")
public suspend fun isSearchable(`value`: Output) {
this.isSearchable = value
}
/**
* @param value Map property.
*/
@JvmName("bfpmcerbeyquhwjs")
public suspend fun mapTypeOptions(`value`: Output) {
this.mapTypeOptions = value
}
/**
* @param value The name of the metadata property.
*/
@JvmName("xlhvorgtuxrxfuvk")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Nested structured data property.
* Structure is documented below.
*/
@JvmName("ifcfdxrjmjckxijw")
public suspend fun propertyTypeOptions(`value`: Output) {
this.propertyTypeOptions = value
}
/**
* @param value Stores the retrieval importance.
* Possible values are: `HIGHEST`, `HIGHER`, `HIGH`, `MEDIUM`, `LOW`, `LOWEST`.
*/
@JvmName("pesagkhupmnhhhyw")
public suspend fun retrievalImportance(`value`: Output) {
this.retrievalImportance = value
}
/**
* @param value The schema source information.
* Structure is documented below.
*/
@JvmName("alvkalpgtsrfogbh")
public suspend fun schemaSources(`value`: Output>) {
this.schemaSources = value
}
@JvmName("mctejgpaavnsngqt")
public suspend fun schemaSources(vararg values: Output) {
this.schemaSources = Output.all(values.asList())
}
/**
* @param values The schema source information.
* Structure is documented below.
*/
@JvmName("qanuildxjibeloec")
public suspend fun schemaSources(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy