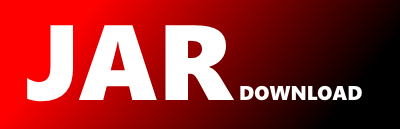
com.pulumi.gcp.essentialcontacts.kotlin.outputs.DocumentAiWarehouseDocumentSchemaPropertyDefinition.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.essentialcontacts.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property dateTimeTypeOptions Date time property. Not supported by CMEK compliant deployment.
* @property displayName The display-name for the property, used for front-end.
* @property enumTypeOptions Enum/categorical property.
* Structure is documented below.
* @property floatTypeOptions Float property.
* @property integerTypeOptions Integer property.
* @property isFilterable Whether the property can be filtered. If this is a sub-property, all the parent properties must be marked filterable.
* @property isMetadata Whether the property is user supplied metadata.
* @property isRepeatable Whether the property can have multiple values.
* @property isRequired Whether the property is mandatory.
* @property isSearchable Indicates that the property should be included in a global search.
* @property mapTypeOptions Map property.
* @property name The name of the metadata property.
* @property propertyTypeOptions Nested structured data property.
* Structure is documented below.
* @property retrievalImportance Stores the retrieval importance.
* Possible values are: `HIGHEST`, `HIGHER`, `HIGH`, `MEDIUM`, `LOW`, `LOWEST`.
* @property schemaSources The schema source information.
* Structure is documented below.
* @property textTypeOptions Text property.
* @property timestampTypeOptions Timestamp property. Not supported by CMEK compliant deployment.
*/
public data class DocumentAiWarehouseDocumentSchemaPropertyDefinition(
public val dateTimeTypeOptions: DocumentAiWarehouseDocumentSchemaPropertyDefinitionDateTimeTypeOptions? = null,
public val displayName: String? = null,
public val enumTypeOptions: DocumentAiWarehouseDocumentSchemaPropertyDefinitionEnumTypeOptions? =
null,
public val floatTypeOptions: DocumentAiWarehouseDocumentSchemaPropertyDefinitionFloatTypeOptions? =
null,
public val integerTypeOptions: DocumentAiWarehouseDocumentSchemaPropertyDefinitionIntegerTypeOptions? = null,
public val isFilterable: Boolean? = null,
public val isMetadata: Boolean? = null,
public val isRepeatable: Boolean? = null,
public val isRequired: Boolean? = null,
public val isSearchable: Boolean? = null,
public val mapTypeOptions: DocumentAiWarehouseDocumentSchemaPropertyDefinitionMapTypeOptions? =
null,
public val name: String,
public val propertyTypeOptions: DocumentAiWarehouseDocumentSchemaPropertyDefinitionPropertyTypeOptions? = null,
public val retrievalImportance: String? = null,
public val schemaSources: List? =
null,
public val textTypeOptions: DocumentAiWarehouseDocumentSchemaPropertyDefinitionTextTypeOptions? =
null,
public val timestampTypeOptions: DocumentAiWarehouseDocumentSchemaPropertyDefinitionTimestampTypeOptions? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.essentialcontacts.outputs.DocumentAiWarehouseDocumentSchemaPropertyDefinition): DocumentAiWarehouseDocumentSchemaPropertyDefinition =
DocumentAiWarehouseDocumentSchemaPropertyDefinition(
dateTimeTypeOptions = javaType.dateTimeTypeOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.essentialcontacts.kotlin.outputs.DocumentAiWarehouseDocumentSchemaPropertyDefinitionDateTimeTypeOptions.Companion.toKotlin(args0)
})
}).orElse(null),
displayName = javaType.displayName().map({ args0 -> args0 }).orElse(null),
enumTypeOptions = javaType.enumTypeOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.essentialcontacts.kotlin.outputs.DocumentAiWarehouseDocumentSchemaPropertyDefinitionEnumTypeOptions.Companion.toKotlin(args0)
})
}).orElse(null),
floatTypeOptions = javaType.floatTypeOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.essentialcontacts.kotlin.outputs.DocumentAiWarehouseDocumentSchemaPropertyDefinitionFloatTypeOptions.Companion.toKotlin(args0)
})
}).orElse(null),
integerTypeOptions = javaType.integerTypeOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.essentialcontacts.kotlin.outputs.DocumentAiWarehouseDocumentSchemaPropertyDefinitionIntegerTypeOptions.Companion.toKotlin(args0)
})
}).orElse(null),
isFilterable = javaType.isFilterable().map({ args0 -> args0 }).orElse(null),
isMetadata = javaType.isMetadata().map({ args0 -> args0 }).orElse(null),
isRepeatable = javaType.isRepeatable().map({ args0 -> args0 }).orElse(null),
isRequired = javaType.isRequired().map({ args0 -> args0 }).orElse(null),
isSearchable = javaType.isSearchable().map({ args0 -> args0 }).orElse(null),
mapTypeOptions = javaType.mapTypeOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.essentialcontacts.kotlin.outputs.DocumentAiWarehouseDocumentSchemaPropertyDefinitionMapTypeOptions.Companion.toKotlin(args0)
})
}).orElse(null),
name = javaType.name(),
propertyTypeOptions = javaType.propertyTypeOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.essentialcontacts.kotlin.outputs.DocumentAiWarehouseDocumentSchemaPropertyDefinitionPropertyTypeOptions.Companion.toKotlin(args0)
})
}).orElse(null),
retrievalImportance = javaType.retrievalImportance().map({ args0 -> args0 }).orElse(null),
schemaSources = javaType.schemaSources().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.essentialcontacts.kotlin.outputs.DocumentAiWarehouseDocumentSchemaPropertyDefinitionSchemaSource.Companion.toKotlin(args0)
})
}),
textTypeOptions = javaType.textTypeOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.essentialcontacts.kotlin.outputs.DocumentAiWarehouseDocumentSchemaPropertyDefinitionTextTypeOptions.Companion.toKotlin(args0)
})
}).orElse(null),
timestampTypeOptions = javaType.timestampTypeOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.essentialcontacts.kotlin.outputs.DocumentAiWarehouseDocumentSchemaPropertyDefinitionTimestampTypeOptions.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy