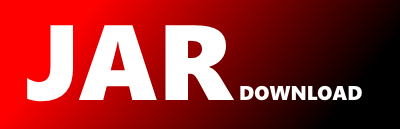
com.pulumi.gcp.eventarc.kotlin.Trigger.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.eventarc.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.eventarc.kotlin.outputs.TriggerDestination
import com.pulumi.gcp.eventarc.kotlin.outputs.TriggerMatchingCriteria
import com.pulumi.gcp.eventarc.kotlin.outputs.TriggerTransport
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Any
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.gcp.eventarc.kotlin.outputs.TriggerDestination.Companion.toKotlin as triggerDestinationToKotlin
import com.pulumi.gcp.eventarc.kotlin.outputs.TriggerMatchingCriteria.Companion.toKotlin as triggerMatchingCriteriaToKotlin
import com.pulumi.gcp.eventarc.kotlin.outputs.TriggerTransport.Companion.toKotlin as triggerTransportToKotlin
/**
* Builder for [Trigger].
*/
@PulumiTagMarker
public class TriggerResourceBuilder internal constructor() {
public var name: String? = null
public var args: TriggerArgs = TriggerArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend TriggerArgsBuilder.() -> Unit) {
val builder = TriggerArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Trigger {
val builtJavaResource = com.pulumi.gcp.eventarc.Trigger(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Trigger(builtJavaResource)
}
}
/**
* The Eventarc Trigger resource
* ## Example Usage
* ### Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.cloudrun.Service("default", {
* name: "eventarc-service",
* location: "europe-west1",
* metadata: {
* namespace: "my-project-name",
* },
* template: {
* spec: {
* containers: [{
* image: "gcr.io/cloudrun/hello",
* ports: [{
* containerPort: 8080,
* }],
* }],
* containerConcurrency: 50,
* timeoutSeconds: 100,
* },
* },
* traffics: [{
* percent: 100,
* latestRevision: true,
* }],
* });
* const primary = new gcp.eventarc.Trigger("primary", {
* name: "name",
* location: "europe-west1",
* matchingCriterias: [{
* attribute: "type",
* value: "google.cloud.pubsub.topic.v1.messagePublished",
* }],
* destination: {
* cloudRunService: {
* service: _default.name,
* region: "europe-west1",
* },
* },
* labels: {
* foo: "bar",
* },
* });
* const foo = new gcp.pubsub.Topic("foo", {name: "topic"});
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.cloudrun.Service("default",
* name="eventarc-service",
* location="europe-west1",
* metadata=gcp.cloudrun.ServiceMetadataArgs(
* namespace="my-project-name",
* ),
* template=gcp.cloudrun.ServiceTemplateArgs(
* spec=gcp.cloudrun.ServiceTemplateSpecArgs(
* containers=[gcp.cloudrun.ServiceTemplateSpecContainerArgs(
* image="gcr.io/cloudrun/hello",
* ports=[gcp.cloudrun.ServiceTemplateSpecContainerPortArgs(
* container_port=8080,
* )],
* )],
* container_concurrency=50,
* timeout_seconds=100,
* ),
* ),
* traffics=[gcp.cloudrun.ServiceTrafficArgs(
* percent=100,
* latest_revision=True,
* )])
* primary = gcp.eventarc.Trigger("primary",
* name="name",
* location="europe-west1",
* matching_criterias=[gcp.eventarc.TriggerMatchingCriteriaArgs(
* attribute="type",
* value="google.cloud.pubsub.topic.v1.messagePublished",
* )],
* destination=gcp.eventarc.TriggerDestinationArgs(
* cloud_run_service=gcp.eventarc.TriggerDestinationCloudRunServiceArgs(
* service=default.name,
* region="europe-west1",
* ),
* ),
* labels={
* "foo": "bar",
* })
* foo = gcp.pubsub.Topic("foo", name="topic")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.CloudRun.Service("default", new()
* {
* Name = "eventarc-service",
* Location = "europe-west1",
* Metadata = new Gcp.CloudRun.Inputs.ServiceMetadataArgs
* {
* Namespace = "my-project-name",
* },
* Template = new Gcp.CloudRun.Inputs.ServiceTemplateArgs
* {
* Spec = new Gcp.CloudRun.Inputs.ServiceTemplateSpecArgs
* {
* Containers = new[]
* {
* new Gcp.CloudRun.Inputs.ServiceTemplateSpecContainerArgs
* {
* Image = "gcr.io/cloudrun/hello",
* Ports = new[]
* {
* new Gcp.CloudRun.Inputs.ServiceTemplateSpecContainerPortArgs
* {
* ContainerPort = 8080,
* },
* },
* },
* },
* ContainerConcurrency = 50,
* TimeoutSeconds = 100,
* },
* },
* Traffics = new[]
* {
* new Gcp.CloudRun.Inputs.ServiceTrafficArgs
* {
* Percent = 100,
* LatestRevision = true,
* },
* },
* });
* var primary = new Gcp.Eventarc.Trigger("primary", new()
* {
* Name = "name",
* Location = "europe-west1",
* MatchingCriterias = new[]
* {
* new Gcp.Eventarc.Inputs.TriggerMatchingCriteriaArgs
* {
* Attribute = "type",
* Value = "google.cloud.pubsub.topic.v1.messagePublished",
* },
* },
* Destination = new Gcp.Eventarc.Inputs.TriggerDestinationArgs
* {
* CloudRunService = new Gcp.Eventarc.Inputs.TriggerDestinationCloudRunServiceArgs
* {
* Service = @default.Name,
* Region = "europe-west1",
* },
* },
* Labels =
* {
* { "foo", "bar" },
* },
* });
* var foo = new Gcp.PubSub.Topic("foo", new()
* {
* Name = "topic",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/cloudrun"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/eventarc"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/pubsub"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cloudrun.NewService(ctx, "default", &cloudrun.ServiceArgs{
* Name: pulumi.String("eventarc-service"),
* Location: pulumi.String("europe-west1"),
* Metadata: &cloudrun.ServiceMetadataArgs{
* Namespace: pulumi.String("my-project-name"),
* },
* Template: &cloudrun.ServiceTemplateArgs{
* Spec: &cloudrun.ServiceTemplateSpecArgs{
* Containers: cloudrun.ServiceTemplateSpecContainerArray{
* &cloudrun.ServiceTemplateSpecContainerArgs{
* Image: pulumi.String("gcr.io/cloudrun/hello"),
* Ports: cloudrun.ServiceTemplateSpecContainerPortArray{
* &cloudrun.ServiceTemplateSpecContainerPortArgs{
* ContainerPort: pulumi.Int(8080),
* },
* },
* },
* },
* ContainerConcurrency: pulumi.Int(50),
* TimeoutSeconds: pulumi.Int(100),
* },
* },
* Traffics: cloudrun.ServiceTrafficArray{
* &cloudrun.ServiceTrafficArgs{
* Percent: pulumi.Int(100),
* LatestRevision: pulumi.Bool(true),
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = eventarc.NewTrigger(ctx, "primary", &eventarc.TriggerArgs{
* Name: pulumi.String("name"),
* Location: pulumi.String("europe-west1"),
* MatchingCriterias: eventarc.TriggerMatchingCriteriaArray{
* &eventarc.TriggerMatchingCriteriaArgs{
* Attribute: pulumi.String("type"),
* Value: pulumi.String("google.cloud.pubsub.topic.v1.messagePublished"),
* },
* },
* Destination: &eventarc.TriggerDestinationArgs{
* CloudRunService: &eventarc.TriggerDestinationCloudRunServiceArgs{
* Service: _default.Name,
* Region: pulumi.String("europe-west1"),
* },
* },
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* })
* if err != nil {
* return err
* }
* _, err = pubsub.NewTopic(ctx, "foo", &pubsub.TopicArgs{
* Name: pulumi.String("topic"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.cloudrun.Service;
* import com.pulumi.gcp.cloudrun.ServiceArgs;
* import com.pulumi.gcp.cloudrun.inputs.ServiceMetadataArgs;
* import com.pulumi.gcp.cloudrun.inputs.ServiceTemplateArgs;
* import com.pulumi.gcp.cloudrun.inputs.ServiceTemplateSpecArgs;
* import com.pulumi.gcp.cloudrun.inputs.ServiceTrafficArgs;
* import com.pulumi.gcp.eventarc.Trigger;
* import com.pulumi.gcp.eventarc.TriggerArgs;
* import com.pulumi.gcp.eventarc.inputs.TriggerMatchingCriteriaArgs;
* import com.pulumi.gcp.eventarc.inputs.TriggerDestinationArgs;
* import com.pulumi.gcp.eventarc.inputs.TriggerDestinationCloudRunServiceArgs;
* import com.pulumi.gcp.pubsub.Topic;
* import com.pulumi.gcp.pubsub.TopicArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Service("default", ServiceArgs.builder()
* .name("eventarc-service")
* .location("europe-west1")
* .metadata(ServiceMetadataArgs.builder()
* .namespace("my-project-name")
* .build())
* .template(ServiceTemplateArgs.builder()
* .spec(ServiceTemplateSpecArgs.builder()
* .containers(ServiceTemplateSpecContainerArgs.builder()
* .image("gcr.io/cloudrun/hello")
* .ports(ServiceTemplateSpecContainerPortArgs.builder()
* .containerPort(8080)
* .build())
* .build())
* .containerConcurrency(50)
* .timeoutSeconds(100)
* .build())
* .build())
* .traffics(ServiceTrafficArgs.builder()
* .percent(100)
* .latestRevision(true)
* .build())
* .build());
* var primary = new Trigger("primary", TriggerArgs.builder()
* .name("name")
* .location("europe-west1")
* .matchingCriterias(TriggerMatchingCriteriaArgs.builder()
* .attribute("type")
* .value("google.cloud.pubsub.topic.v1.messagePublished")
* .build())
* .destination(TriggerDestinationArgs.builder()
* .cloudRunService(TriggerDestinationCloudRunServiceArgs.builder()
* .service(default_.name())
* .region("europe-west1")
* .build())
* .build())
* .labels(Map.of("foo", "bar"))
* .build());
* var foo = new Topic("foo", TopicArgs.builder()
* .name("topic")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* primary:
* type: gcp:eventarc:Trigger
* properties:
* name: name
* location: europe-west1
* matchingCriterias:
* - attribute: type
* value: google.cloud.pubsub.topic.v1.messagePublished
* destination:
* cloudRunService:
* service: ${default.name}
* region: europe-west1
* labels:
* foo: bar
* foo:
* type: gcp:pubsub:Topic
* properties:
* name: topic
* default:
* type: gcp:cloudrun:Service
* properties:
* name: eventarc-service
* location: europe-west1
* metadata:
* namespace: my-project-name
* template:
* spec:
* containers:
* - image: gcr.io/cloudrun/hello
* ports:
* - containerPort: 8080
* containerConcurrency: 50
* timeoutSeconds: 100
* traffics:
* - percent: 100
* latestRevision: true
* ```
*
* ## Import
* Trigger can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/triggers/{{name}}`
* * `{{project}}/{{location}}/{{name}}`
* * `{{location}}/{{name}}`
* When using the `pulumi import` command, Trigger can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:eventarc/trigger:Trigger default projects/{{project}}/locations/{{location}}/triggers/{{name}}
* ```
* ```sh
* $ pulumi import gcp:eventarc/trigger:Trigger default {{project}}/{{location}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:eventarc/trigger:Trigger default {{location}}/{{name}}
* ```
*/
public class Trigger internal constructor(
override val javaResource: com.pulumi.gcp.eventarc.Trigger,
) : KotlinCustomResource(javaResource, TriggerMapper) {
/**
* Optional. The name of the channel associated with the trigger in
* `projects/{project}/locations/{location}/channels/{channel}` format. You must provide a channel to receive events from
* Eventarc SaaS partners.
*/
public val channel: Output?
get() = javaResource.channel().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Output only. The reason(s) why a trigger is in FAILED state.
*/
public val conditions: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy