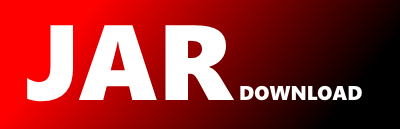
com.pulumi.gcp.firebase.kotlin.AppCheckAppAttestConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.firebase.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.firebase.AppCheckAppAttestConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* An app's App Attest configuration object. Note that the Team ID registered with your
* app is used as part of the validation process. Make sure your `gcp.firebase.AppleApp` has a team_id present.
* To get more information about AppAttestConfig, see:
* * [API documentation](https://firebase.google.com/docs/reference/appcheck/rest/v1/projects.apps.appAttestConfig)
* * How-to Guides
* * [Official Documentation](https://firebase.google.com/docs/app-check)
* ## Example Usage
* ### Firebase App Check App Attest Config Minimal
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* import * as time from "@pulumi/time";
* const _default = new gcp.firebase.AppleApp("default", {
* project: "my-project-name",
* displayName: "Apple app",
* bundleId: "bundle.id.appattest",
* teamId: "9987654321",
* });
* // It takes a while for App Check to recognize the new app
* // If your app already exists, you don't have to wait 30 seconds.
* const wait30s = new time.index.Sleep("wait_30s", {createDuration: "30s"});
* const defaultAppCheckAppAttestConfig = new gcp.firebase.AppCheckAppAttestConfig("default", {
* project: "my-project-name",
* appId: _default.appId,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* import pulumi_time as time
* default = gcp.firebase.AppleApp("default",
* project="my-project-name",
* display_name="Apple app",
* bundle_id="bundle.id.appattest",
* team_id="9987654321")
* # It takes a while for App Check to recognize the new app
* # If your app already exists, you don't have to wait 30 seconds.
* wait30s = time.index.Sleep("wait_30s", create_duration=30s)
* default_app_check_app_attest_config = gcp.firebase.AppCheckAppAttestConfig("default",
* project="my-project-name",
* app_id=default.app_id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* using Time = Pulumi.Time;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Firebase.AppleApp("default", new()
* {
* Project = "my-project-name",
* DisplayName = "Apple app",
* BundleId = "bundle.id.appattest",
* TeamId = "9987654321",
* });
* // It takes a while for App Check to recognize the new app
* // If your app already exists, you don't have to wait 30 seconds.
* var wait30s = new Time.Index.Sleep("wait_30s", new()
* {
* CreateDuration = "30s",
* });
* var defaultAppCheckAppAttestConfig = new Gcp.Firebase.AppCheckAppAttestConfig("default", new()
* {
* Project = "my-project-name",
* AppId = @default.AppId,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/firebase"
* "github.com/pulumi/pulumi-time/sdk/go/time"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := firebase.NewAppleApp(ctx, "default", &firebase.AppleAppArgs{
* Project: pulumi.String("my-project-name"),
* DisplayName: pulumi.String("Apple app"),
* BundleId: pulumi.String("bundle.id.appattest"),
* TeamId: pulumi.String("9987654321"),
* })
* if err != nil {
* return err
* }
* // It takes a while for App Check to recognize the new app
* // If your app already exists, you don't have to wait 30 seconds.
* _, err = time.NewSleep(ctx, "wait_30s", &time.SleepArgs{
* CreateDuration: "30s",
* })
* if err != nil {
* return err
* }
* _, err = firebase.NewAppCheckAppAttestConfig(ctx, "default", &firebase.AppCheckAppAttestConfigArgs{
* Project: pulumi.String("my-project-name"),
* AppId: _default.AppId,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.firebase.AppleApp;
* import com.pulumi.gcp.firebase.AppleAppArgs;
* import com.pulumi.time.sleep;
* import com.pulumi.time.SleepArgs;
* import com.pulumi.gcp.firebase.AppCheckAppAttestConfig;
* import com.pulumi.gcp.firebase.AppCheckAppAttestConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new AppleApp("default", AppleAppArgs.builder()
* .project("my-project-name")
* .displayName("Apple app")
* .bundleId("bundle.id.appattest")
* .teamId("9987654321")
* .build());
* // It takes a while for App Check to recognize the new app
* // If your app already exists, you don't have to wait 30 seconds.
* var wait30s = new Sleep("wait30s", SleepArgs.builder()
* .createDuration("30s")
* .build());
* var defaultAppCheckAppAttestConfig = new AppCheckAppAttestConfig("defaultAppCheckAppAttestConfig", AppCheckAppAttestConfigArgs.builder()
* .project("my-project-name")
* .appId(default_.appId())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:firebase:AppleApp
* properties:
* project: my-project-name
* displayName: Apple app
* bundleId: bundle.id.appattest
* teamId: '9987654321'
* # It takes a while for App Check to recognize the new app
* # If your app already exists, you don't have to wait 30 seconds.
* wait30s:
* type: time:sleep
* name: wait_30s
* properties:
* createDuration: 30s
* defaultAppCheckAppAttestConfig:
* type: gcp:firebase:AppCheckAppAttestConfig
* name: default
* properties:
* project: my-project-name
* appId: ${default.appId}
* ```
*
* ### Firebase App Check App Attest Config Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* import * as time from "@pulumi/time";
* const _default = new gcp.firebase.AppleApp("default", {
* project: "my-project-name",
* displayName: "Apple app",
* bundleId: "bundle.id.appattest",
* teamId: "9987654321",
* });
* // It takes a while for App Check to recognize the new app
* // If your app already exists, you don't have to wait 30 seconds.
* const wait30s = new time.index.Sleep("wait_30s", {createDuration: "30s"});
* const defaultAppCheckAppAttestConfig = new gcp.firebase.AppCheckAppAttestConfig("default", {
* project: "my-project-name",
* appId: _default.appId,
* tokenTtl: "7200s",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* import pulumi_time as time
* default = gcp.firebase.AppleApp("default",
* project="my-project-name",
* display_name="Apple app",
* bundle_id="bundle.id.appattest",
* team_id="9987654321")
* # It takes a while for App Check to recognize the new app
* # If your app already exists, you don't have to wait 30 seconds.
* wait30s = time.index.Sleep("wait_30s", create_duration=30s)
* default_app_check_app_attest_config = gcp.firebase.AppCheckAppAttestConfig("default",
* project="my-project-name",
* app_id=default.app_id,
* token_ttl="7200s")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* using Time = Pulumi.Time;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Firebase.AppleApp("default", new()
* {
* Project = "my-project-name",
* DisplayName = "Apple app",
* BundleId = "bundle.id.appattest",
* TeamId = "9987654321",
* });
* // It takes a while for App Check to recognize the new app
* // If your app already exists, you don't have to wait 30 seconds.
* var wait30s = new Time.Index.Sleep("wait_30s", new()
* {
* CreateDuration = "30s",
* });
* var defaultAppCheckAppAttestConfig = new Gcp.Firebase.AppCheckAppAttestConfig("default", new()
* {
* Project = "my-project-name",
* AppId = @default.AppId,
* TokenTtl = "7200s",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/firebase"
* "github.com/pulumi/pulumi-time/sdk/go/time"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := firebase.NewAppleApp(ctx, "default", &firebase.AppleAppArgs{
* Project: pulumi.String("my-project-name"),
* DisplayName: pulumi.String("Apple app"),
* BundleId: pulumi.String("bundle.id.appattest"),
* TeamId: pulumi.String("9987654321"),
* })
* if err != nil {
* return err
* }
* // It takes a while for App Check to recognize the new app
* // If your app already exists, you don't have to wait 30 seconds.
* _, err = time.NewSleep(ctx, "wait_30s", &time.SleepArgs{
* CreateDuration: "30s",
* })
* if err != nil {
* return err
* }
* _, err = firebase.NewAppCheckAppAttestConfig(ctx, "default", &firebase.AppCheckAppAttestConfigArgs{
* Project: pulumi.String("my-project-name"),
* AppId: _default.AppId,
* TokenTtl: pulumi.String("7200s"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.firebase.AppleApp;
* import com.pulumi.gcp.firebase.AppleAppArgs;
* import com.pulumi.time.sleep;
* import com.pulumi.time.SleepArgs;
* import com.pulumi.gcp.firebase.AppCheckAppAttestConfig;
* import com.pulumi.gcp.firebase.AppCheckAppAttestConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new AppleApp("default", AppleAppArgs.builder()
* .project("my-project-name")
* .displayName("Apple app")
* .bundleId("bundle.id.appattest")
* .teamId("9987654321")
* .build());
* // It takes a while for App Check to recognize the new app
* // If your app already exists, you don't have to wait 30 seconds.
* var wait30s = new Sleep("wait30s", SleepArgs.builder()
* .createDuration("30s")
* .build());
* var defaultAppCheckAppAttestConfig = new AppCheckAppAttestConfig("defaultAppCheckAppAttestConfig", AppCheckAppAttestConfigArgs.builder()
* .project("my-project-name")
* .appId(default_.appId())
* .tokenTtl("7200s")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:firebase:AppleApp
* properties:
* project: my-project-name
* displayName: Apple app
* bundleId: bundle.id.appattest
* teamId: '9987654321'
* # It takes a while for App Check to recognize the new app
* # If your app already exists, you don't have to wait 30 seconds.
* wait30s:
* type: time:sleep
* name: wait_30s
* properties:
* createDuration: 30s
* defaultAppCheckAppAttestConfig:
* type: gcp:firebase:AppCheckAppAttestConfig
* name: default
* properties:
* project: my-project-name
* appId: ${default.appId}
* tokenTtl: 7200s
* ```
*
* ## Import
* AppAttestConfig can be imported using any of these accepted formats:
* * `projects/{{project}}/apps/{{app_id}}/appAttestConfig`
* * `{{project}}/{{app_id}}`
* * `{{app_id}}`
* When using the `pulumi import` command, AppAttestConfig can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:firebase/appCheckAppAttestConfig:AppCheckAppAttestConfig default projects/{{project}}/apps/{{app_id}}/appAttestConfig
* ```
* ```sh
* $ pulumi import gcp:firebase/appCheckAppAttestConfig:AppCheckAppAttestConfig default {{project}}/{{app_id}}
* ```
* ```sh
* $ pulumi import gcp:firebase/appCheckAppAttestConfig:AppCheckAppAttestConfig default {{app_id}}
* ```
* @property appId The ID of an
* [Apple App](https://firebase.google.com/docs/reference/firebase-management/rest/v1beta1/projects.iosApps#IosApp.FIELDS.app_id).
* - - -
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property tokenTtl Specifies the duration for which App Check tokens exchanged from App Attest artifacts will be valid.
* If unset, a default value of 1 hour is assumed. Must be between 30 minutes and 7 days, inclusive.
* A duration in seconds with up to nine fractional digits, ending with 's'. Example: "3.5s".
*/
public data class AppCheckAppAttestConfigArgs(
public val appId: Output? = null,
public val project: Output? = null,
public val tokenTtl: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.firebase.AppCheckAppAttestConfigArgs =
com.pulumi.gcp.firebase.AppCheckAppAttestConfigArgs.builder()
.appId(appId?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.tokenTtl(tokenTtl?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AppCheckAppAttestConfigArgs].
*/
@PulumiTagMarker
public class AppCheckAppAttestConfigArgsBuilder internal constructor() {
private var appId: Output? = null
private var project: Output? = null
private var tokenTtl: Output? = null
/**
* @param value The ID of an
* [Apple App](https://firebase.google.com/docs/reference/firebase-management/rest/v1beta1/projects.iosApps#IosApp.FIELDS.app_id).
* - - -
*/
@JvmName("nepuupwshwyvtwtv")
public suspend fun appId(`value`: Output) {
this.appId = value
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("yihisntjjjejwpij")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value Specifies the duration for which App Check tokens exchanged from App Attest artifacts will be valid.
* If unset, a default value of 1 hour is assumed. Must be between 30 minutes and 7 days, inclusive.
* A duration in seconds with up to nine fractional digits, ending with 's'. Example: "3.5s".
*/
@JvmName("bvugwjrefhclurvw")
public suspend fun tokenTtl(`value`: Output) {
this.tokenTtl = value
}
/**
* @param value The ID of an
* [Apple App](https://firebase.google.com/docs/reference/firebase-management/rest/v1beta1/projects.iosApps#IosApp.FIELDS.app_id).
* - - -
*/
@JvmName("lrnxbmvvfebaexwa")
public suspend fun appId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.appId = mapped
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("xhqdisxfwnrdkndx")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value Specifies the duration for which App Check tokens exchanged from App Attest artifacts will be valid.
* If unset, a default value of 1 hour is assumed. Must be between 30 minutes and 7 days, inclusive.
* A duration in seconds with up to nine fractional digits, ending with 's'. Example: "3.5s".
*/
@JvmName("ndwfcdfcnabtfywo")
public suspend fun tokenTtl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tokenTtl = mapped
}
internal fun build(): AppCheckAppAttestConfigArgs = AppCheckAppAttestConfigArgs(
appId = appId,
project = project,
tokenTtl = tokenTtl,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy