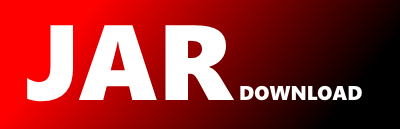
com.pulumi.gcp.firebase.kotlin.FirebaseFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.firebase.kotlin
import com.pulumi.gcp.firebase.FirebaseFunctions.getAndroidAppConfigPlain
import com.pulumi.gcp.firebase.FirebaseFunctions.getAndroidAppPlain
import com.pulumi.gcp.firebase.FirebaseFunctions.getAppleAppConfigPlain
import com.pulumi.gcp.firebase.FirebaseFunctions.getAppleAppPlain
import com.pulumi.gcp.firebase.FirebaseFunctions.getHostingChannelPlain
import com.pulumi.gcp.firebase.FirebaseFunctions.getWebAppConfigPlain
import com.pulumi.gcp.firebase.FirebaseFunctions.getWebAppPlain
import com.pulumi.gcp.firebase.kotlin.inputs.GetAndroidAppConfigPlainArgs
import com.pulumi.gcp.firebase.kotlin.inputs.GetAndroidAppConfigPlainArgsBuilder
import com.pulumi.gcp.firebase.kotlin.inputs.GetAndroidAppPlainArgs
import com.pulumi.gcp.firebase.kotlin.inputs.GetAndroidAppPlainArgsBuilder
import com.pulumi.gcp.firebase.kotlin.inputs.GetAppleAppConfigPlainArgs
import com.pulumi.gcp.firebase.kotlin.inputs.GetAppleAppConfigPlainArgsBuilder
import com.pulumi.gcp.firebase.kotlin.inputs.GetAppleAppPlainArgs
import com.pulumi.gcp.firebase.kotlin.inputs.GetAppleAppPlainArgsBuilder
import com.pulumi.gcp.firebase.kotlin.inputs.GetHostingChannelPlainArgs
import com.pulumi.gcp.firebase.kotlin.inputs.GetHostingChannelPlainArgsBuilder
import com.pulumi.gcp.firebase.kotlin.inputs.GetWebAppConfigPlainArgs
import com.pulumi.gcp.firebase.kotlin.inputs.GetWebAppConfigPlainArgsBuilder
import com.pulumi.gcp.firebase.kotlin.inputs.GetWebAppPlainArgs
import com.pulumi.gcp.firebase.kotlin.inputs.GetWebAppPlainArgsBuilder
import com.pulumi.gcp.firebase.kotlin.outputs.GetAndroidAppConfigResult
import com.pulumi.gcp.firebase.kotlin.outputs.GetAndroidAppResult
import com.pulumi.gcp.firebase.kotlin.outputs.GetAppleAppConfigResult
import com.pulumi.gcp.firebase.kotlin.outputs.GetAppleAppResult
import com.pulumi.gcp.firebase.kotlin.outputs.GetHostingChannelResult
import com.pulumi.gcp.firebase.kotlin.outputs.GetWebAppConfigResult
import com.pulumi.gcp.firebase.kotlin.outputs.GetWebAppResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.gcp.firebase.kotlin.outputs.GetAndroidAppConfigResult.Companion.toKotlin as getAndroidAppConfigResultToKotlin
import com.pulumi.gcp.firebase.kotlin.outputs.GetAndroidAppResult.Companion.toKotlin as getAndroidAppResultToKotlin
import com.pulumi.gcp.firebase.kotlin.outputs.GetAppleAppConfigResult.Companion.toKotlin as getAppleAppConfigResultToKotlin
import com.pulumi.gcp.firebase.kotlin.outputs.GetAppleAppResult.Companion.toKotlin as getAppleAppResultToKotlin
import com.pulumi.gcp.firebase.kotlin.outputs.GetHostingChannelResult.Companion.toKotlin as getHostingChannelResultToKotlin
import com.pulumi.gcp.firebase.kotlin.outputs.GetWebAppConfigResult.Companion.toKotlin as getWebAppConfigResultToKotlin
import com.pulumi.gcp.firebase.kotlin.outputs.GetWebAppResult.Companion.toKotlin as getWebAppResultToKotlin
public object FirebaseFunctions {
/**
*
* @param argument A collection of arguments for invoking getAndroidApp.
* @return A collection of values returned by getAndroidApp.
*/
public suspend fun getAndroidApp(argument: GetAndroidAppPlainArgs): GetAndroidAppResult =
getAndroidAppResultToKotlin(getAndroidAppPlain(argument.toJava()).await())
/**
* @see [getAndroidApp].
* @param appId The app_id of name of the Firebase androidApp.
* - - -
* @param project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @return A collection of values returned by getAndroidApp.
*/
public suspend fun getAndroidApp(appId: String, project: String? = null): GetAndroidAppResult {
val argument = GetAndroidAppPlainArgs(
appId = appId,
project = project,
)
return getAndroidAppResultToKotlin(getAndroidAppPlain(argument.toJava()).await())
}
/**
* @see [getAndroidApp].
* @param argument Builder for [com.pulumi.gcp.firebase.kotlin.inputs.GetAndroidAppPlainArgs].
* @return A collection of values returned by getAndroidApp.
*/
public suspend fun getAndroidApp(argument: suspend GetAndroidAppPlainArgsBuilder.() -> Unit): GetAndroidAppResult {
val builder = GetAndroidAppPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAndroidAppResultToKotlin(getAndroidAppPlain(builtArgument.toJava()).await())
}
/**
*
* @param argument A collection of arguments for invoking getAndroidAppConfig.
* @return A collection of values returned by getAndroidAppConfig.
*/
public suspend fun getAndroidAppConfig(argument: GetAndroidAppConfigPlainArgs): GetAndroidAppConfigResult =
getAndroidAppConfigResultToKotlin(getAndroidAppConfigPlain(argument.toJava()).await())
/**
* @see [getAndroidAppConfig].
* @param appId
* @param project
* @return A collection of values returned by getAndroidAppConfig.
*/
public suspend fun getAndroidAppConfig(appId: String, project: String? = null): GetAndroidAppConfigResult {
val argument = GetAndroidAppConfigPlainArgs(
appId = appId,
project = project,
)
return getAndroidAppConfigResultToKotlin(getAndroidAppConfigPlain(argument.toJava()).await())
}
/**
* @see [getAndroidAppConfig].
* @param argument Builder for [com.pulumi.gcp.firebase.kotlin.inputs.GetAndroidAppConfigPlainArgs].
* @return A collection of values returned by getAndroidAppConfig.
*/
public suspend fun getAndroidAppConfig(argument: suspend GetAndroidAppConfigPlainArgsBuilder.() -> Unit): GetAndroidAppConfigResult {
val builder = GetAndroidAppConfigPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAndroidAppConfigResultToKotlin(getAndroidAppConfigPlain(builtArgument.toJava()).await())
}
/**
*
* @param argument A collection of arguments for invoking getAppleApp.
* @return A collection of values returned by getAppleApp.
*/
public suspend fun getAppleApp(argument: GetAppleAppPlainArgs): GetAppleAppResult =
getAppleAppResultToKotlin(getAppleAppPlain(argument.toJava()).await())
/**
* @see [getAppleApp].
* @param appId The app_id of name of the Firebase iosApp.
* - - -
* @param project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @return A collection of values returned by getAppleApp.
*/
public suspend fun getAppleApp(appId: String, project: String? = null): GetAppleAppResult {
val argument = GetAppleAppPlainArgs(
appId = appId,
project = project,
)
return getAppleAppResultToKotlin(getAppleAppPlain(argument.toJava()).await())
}
/**
* @see [getAppleApp].
* @param argument Builder for [com.pulumi.gcp.firebase.kotlin.inputs.GetAppleAppPlainArgs].
* @return A collection of values returned by getAppleApp.
*/
public suspend fun getAppleApp(argument: suspend GetAppleAppPlainArgsBuilder.() -> Unit): GetAppleAppResult {
val builder = GetAppleAppPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAppleAppResultToKotlin(getAppleAppPlain(builtArgument.toJava()).await())
}
/**
*
* @param argument A collection of arguments for invoking getAppleAppConfig.
* @return A collection of values returned by getAppleAppConfig.
*/
public suspend fun getAppleAppConfig(argument: GetAppleAppConfigPlainArgs): GetAppleAppConfigResult =
getAppleAppConfigResultToKotlin(getAppleAppConfigPlain(argument.toJava()).await())
/**
* @see [getAppleAppConfig].
* @param appId The id of the Firebase iOS App.
* - - -
* @param project The ID of the project in which the resource belongs. If it
* is not provided, the provider project is used.
* @return A collection of values returned by getAppleAppConfig.
*/
public suspend fun getAppleAppConfig(appId: String, project: String? = null): GetAppleAppConfigResult {
val argument = GetAppleAppConfigPlainArgs(
appId = appId,
project = project,
)
return getAppleAppConfigResultToKotlin(getAppleAppConfigPlain(argument.toJava()).await())
}
/**
* @see [getAppleAppConfig].
* @param argument Builder for [com.pulumi.gcp.firebase.kotlin.inputs.GetAppleAppConfigPlainArgs].
* @return A collection of values returned by getAppleAppConfig.
*/
public suspend fun getAppleAppConfig(argument: suspend GetAppleAppConfigPlainArgsBuilder.() -> Unit): GetAppleAppConfigResult {
val builder = GetAppleAppConfigPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAppleAppConfigResultToKotlin(getAppleAppConfigPlain(builtArgument.toJava()).await())
}
/**
*
* @param argument A collection of arguments for invoking getHostingChannel.
* @return A collection of values returned by getHostingChannel.
*/
public suspend fun getHostingChannel(argument: GetHostingChannelPlainArgs): GetHostingChannelResult =
getHostingChannelResultToKotlin(getHostingChannelPlain(argument.toJava()).await())
/**
* @see [getHostingChannel].
* @param channelId The ID of the channel. Use `channel_id = "live"` for the default channel of a site.
* @param siteId The ID of the site this channel belongs to.
* @return A collection of values returned by getHostingChannel.
*/
public suspend fun getHostingChannel(channelId: String, siteId: String): GetHostingChannelResult {
val argument = GetHostingChannelPlainArgs(
channelId = channelId,
siteId = siteId,
)
return getHostingChannelResultToKotlin(getHostingChannelPlain(argument.toJava()).await())
}
/**
* @see [getHostingChannel].
* @param argument Builder for [com.pulumi.gcp.firebase.kotlin.inputs.GetHostingChannelPlainArgs].
* @return A collection of values returned by getHostingChannel.
*/
public suspend fun getHostingChannel(argument: suspend GetHostingChannelPlainArgsBuilder.() -> Unit): GetHostingChannelResult {
val builder = GetHostingChannelPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getHostingChannelResultToKotlin(getHostingChannelPlain(builtArgument.toJava()).await())
}
/**
* A Google Cloud Firebase web application instance
* @param argument A collection of arguments for invoking getWebApp.
* @return A collection of values returned by getWebApp.
*/
public suspend fun getWebApp(argument: GetWebAppPlainArgs): GetWebAppResult =
getWebAppResultToKotlin(getWebAppPlain(argument.toJava()).await())
/**
* @see [getWebApp].
* @param appId The app_ip of name of the Firebase webApp.
* - - -
* @param project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @return A collection of values returned by getWebApp.
*/
public suspend fun getWebApp(appId: String, project: String? = null): GetWebAppResult {
val argument = GetWebAppPlainArgs(
appId = appId,
project = project,
)
return getWebAppResultToKotlin(getWebAppPlain(argument.toJava()).await())
}
/**
* @see [getWebApp].
* @param argument Builder for [com.pulumi.gcp.firebase.kotlin.inputs.GetWebAppPlainArgs].
* @return A collection of values returned by getWebApp.
*/
public suspend fun getWebApp(argument: suspend GetWebAppPlainArgsBuilder.() -> Unit): GetWebAppResult {
val builder = GetWebAppPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getWebAppResultToKotlin(getWebAppPlain(builtArgument.toJava()).await())
}
/**
* A Google Cloud Firebase web application configuration
* To get more information about WebApp, see:
* * [API documentation](https://firebase.google.com/docs/projects/api/reference/rest/v1beta1/projects.webApps)
* * How-to Guides
* * [Official Documentation](https://firebase.google.com/)
* @param argument A collection of arguments for invoking getWebAppConfig.
* @return A collection of values returned by getWebAppConfig.
*/
public suspend fun getWebAppConfig(argument: GetWebAppConfigPlainArgs): GetWebAppConfigResult =
getWebAppConfigResultToKotlin(getWebAppConfigPlain(argument.toJava()).await())
/**
* @see [getWebAppConfig].
* @param project The ID of the project in which the resource belongs. If it
* is not provided, the provider project is used.
* @param webAppId the id of the firebase web app
* - - -
* @return A collection of values returned by getWebAppConfig.
*/
public suspend fun getWebAppConfig(project: String? = null, webAppId: String): GetWebAppConfigResult {
val argument = GetWebAppConfigPlainArgs(
project = project,
webAppId = webAppId,
)
return getWebAppConfigResultToKotlin(getWebAppConfigPlain(argument.toJava()).await())
}
/**
* @see [getWebAppConfig].
* @param argument Builder for [com.pulumi.gcp.firebase.kotlin.inputs.GetWebAppConfigPlainArgs].
* @return A collection of values returned by getWebAppConfig.
*/
public suspend fun getWebAppConfig(argument: suspend GetWebAppConfigPlainArgsBuilder.() -> Unit): GetWebAppConfigResult {
val builder = GetWebAppConfigPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getWebAppConfigResultToKotlin(getWebAppConfigPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy