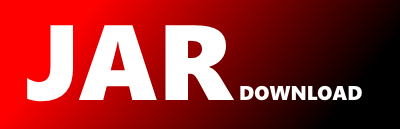
com.pulumi.gcp.firestore.kotlin.FieldArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.firestore.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.firestore.FieldArgs.builder
import com.pulumi.gcp.firestore.kotlin.inputs.FieldIndexConfigArgs
import com.pulumi.gcp.firestore.kotlin.inputs.FieldIndexConfigArgsBuilder
import com.pulumi.gcp.firestore.kotlin.inputs.FieldTtlConfigArgs
import com.pulumi.gcp.firestore.kotlin.inputs.FieldTtlConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Represents a single field in the database.
* Fields are grouped by their "Collection Group", which represent all collections
* in the database with the same id.
* To get more information about Field, see:
* * [API documentation](https://cloud.google.com/firestore/docs/reference/rest/v1/projects.databases.collectionGroups.fields)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/firestore/docs/query-data/indexing)
* > **Warning:** This resource creates a Firestore Single Field override on a project that
* already has a Firestore database. If you haven't already created it, you may
* create a `gcp.firestore.Database` resource with `location_id` set to your
* chosen location.
* ## Example Usage
* ### Firestore Field Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const database = new gcp.firestore.Database("database", {
* project: "my-project-name",
* name: "database-id",
* locationId: "nam5",
* type: "FIRESTORE_NATIVE",
* deleteProtectionState: "DELETE_PROTECTION_ENABLED",
* deletionPolicy: "DELETE",
* });
* const basic = new gcp.firestore.Field("basic", {
* project: "my-project-name",
* database: database.name,
* collection: "chatrooms__74000",
* field: "basic",
* indexConfig: {
* indexes: [
* {
* order: "ASCENDING",
* queryScope: "COLLECTION_GROUP",
* },
* {
* arrayConfig: "CONTAINS",
* },
* ],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* database = gcp.firestore.Database("database",
* project="my-project-name",
* name="database-id",
* location_id="nam5",
* type="FIRESTORE_NATIVE",
* delete_protection_state="DELETE_PROTECTION_ENABLED",
* deletion_policy="DELETE")
* basic = gcp.firestore.Field("basic",
* project="my-project-name",
* database=database.name,
* collection="chatrooms__74000",
* field="basic",
* index_config=gcp.firestore.FieldIndexConfigArgs(
* indexes=[
* gcp.firestore.FieldIndexConfigIndexArgs(
* order="ASCENDING",
* query_scope="COLLECTION_GROUP",
* ),
* gcp.firestore.FieldIndexConfigIndexArgs(
* array_config="CONTAINS",
* ),
* ],
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var database = new Gcp.Firestore.Database("database", new()
* {
* Project = "my-project-name",
* Name = "database-id",
* LocationId = "nam5",
* Type = "FIRESTORE_NATIVE",
* DeleteProtectionState = "DELETE_PROTECTION_ENABLED",
* DeletionPolicy = "DELETE",
* });
* var basic = new Gcp.Firestore.Field("basic", new()
* {
* Project = "my-project-name",
* Database = database.Name,
* Collection = "chatrooms__74000",
* FieldId = "basic",
* IndexConfig = new Gcp.Firestore.Inputs.FieldIndexConfigArgs
* {
* Indexes = new[]
* {
* new Gcp.Firestore.Inputs.FieldIndexConfigIndexArgs
* {
* Order = "ASCENDING",
* QueryScope = "COLLECTION_GROUP",
* },
* new Gcp.Firestore.Inputs.FieldIndexConfigIndexArgs
* {
* ArrayConfig = "CONTAINS",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/firestore"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* database, err := firestore.NewDatabase(ctx, "database", &firestore.DatabaseArgs{
* Project: pulumi.String("my-project-name"),
* Name: pulumi.String("database-id"),
* LocationId: pulumi.String("nam5"),
* Type: pulumi.String("FIRESTORE_NATIVE"),
* DeleteProtectionState: pulumi.String("DELETE_PROTECTION_ENABLED"),
* DeletionPolicy: pulumi.String("DELETE"),
* })
* if err != nil {
* return err
* }
* _, err = firestore.NewField(ctx, "basic", &firestore.FieldArgs{
* Project: pulumi.String("my-project-name"),
* Database: database.Name,
* Collection: pulumi.String("chatrooms__74000"),
* Field: pulumi.String("basic"),
* IndexConfig: &firestore.FieldIndexConfigArgs{
* Indexes: firestore.FieldIndexConfigIndexArray{
* &firestore.FieldIndexConfigIndexArgs{
* Order: pulumi.String("ASCENDING"),
* QueryScope: pulumi.String("COLLECTION_GROUP"),
* },
* &firestore.FieldIndexConfigIndexArgs{
* ArrayConfig: pulumi.String("CONTAINS"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.firestore.Database;
* import com.pulumi.gcp.firestore.DatabaseArgs;
* import com.pulumi.gcp.firestore.Field;
* import com.pulumi.gcp.firestore.FieldArgs;
* import com.pulumi.gcp.firestore.inputs.FieldIndexConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var database = new Database("database", DatabaseArgs.builder()
* .project("my-project-name")
* .name("database-id")
* .locationId("nam5")
* .type("FIRESTORE_NATIVE")
* .deleteProtectionState("DELETE_PROTECTION_ENABLED")
* .deletionPolicy("DELETE")
* .build());
* var basic = new Field("basic", FieldArgs.builder()
* .project("my-project-name")
* .database(database.name())
* .collection("chatrooms__74000")
* .field("basic")
* .indexConfig(FieldIndexConfigArgs.builder()
* .indexes(
* FieldIndexConfigIndexArgs.builder()
* .order("ASCENDING")
* .queryScope("COLLECTION_GROUP")
* .build(),
* FieldIndexConfigIndexArgs.builder()
* .arrayConfig("CONTAINS")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* database:
* type: gcp:firestore:Database
* properties:
* project: my-project-name
* name: database-id
* locationId: nam5
* type: FIRESTORE_NATIVE
* deleteProtectionState: DELETE_PROTECTION_ENABLED
* deletionPolicy: DELETE
* basic:
* type: gcp:firestore:Field
* properties:
* project: my-project-name
* database: ${database.name}
* collection: chatrooms__74000
* field: basic
* indexConfig:
* indexes:
* - order: ASCENDING
* queryScope: COLLECTION_GROUP
* - arrayConfig: CONTAINS
* ```
*
* ### Firestore Field Timestamp
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const database = new gcp.firestore.Database("database", {
* project: "my-project-name",
* name: "database-id",
* locationId: "nam5",
* type: "FIRESTORE_NATIVE",
* deleteProtectionState: "DELETE_PROTECTION_ENABLED",
* deletionPolicy: "DELETE",
* });
* const timestamp = new gcp.firestore.Field("timestamp", {
* project: "my-project-name",
* database: database.name,
* collection: "chatrooms",
* field: "timestamp",
* ttlConfig: {},
* indexConfig: {},
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* database = gcp.firestore.Database("database",
* project="my-project-name",
* name="database-id",
* location_id="nam5",
* type="FIRESTORE_NATIVE",
* delete_protection_state="DELETE_PROTECTION_ENABLED",
* deletion_policy="DELETE")
* timestamp = gcp.firestore.Field("timestamp",
* project="my-project-name",
* database=database.name,
* collection="chatrooms",
* field="timestamp",
* ttl_config=gcp.firestore.FieldTtlConfigArgs(),
* index_config=gcp.firestore.FieldIndexConfigArgs())
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var database = new Gcp.Firestore.Database("database", new()
* {
* Project = "my-project-name",
* Name = "database-id",
* LocationId = "nam5",
* Type = "FIRESTORE_NATIVE",
* DeleteProtectionState = "DELETE_PROTECTION_ENABLED",
* DeletionPolicy = "DELETE",
* });
* var timestamp = new Gcp.Firestore.Field("timestamp", new()
* {
* Project = "my-project-name",
* Database = database.Name,
* Collection = "chatrooms",
* FieldId = "timestamp",
* TtlConfig = null,
* IndexConfig = null,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/firestore"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* database, err := firestore.NewDatabase(ctx, "database", &firestore.DatabaseArgs{
* Project: pulumi.String("my-project-name"),
* Name: pulumi.String("database-id"),
* LocationId: pulumi.String("nam5"),
* Type: pulumi.String("FIRESTORE_NATIVE"),
* DeleteProtectionState: pulumi.String("DELETE_PROTECTION_ENABLED"),
* DeletionPolicy: pulumi.String("DELETE"),
* })
* if err != nil {
* return err
* }
* _, err = firestore.NewField(ctx, "timestamp", &firestore.FieldArgs{
* Project: pulumi.String("my-project-name"),
* Database: database.Name,
* Collection: pulumi.String("chatrooms"),
* Field: pulumi.String("timestamp"),
* TtlConfig: nil,
* IndexConfig: nil,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.firestore.Database;
* import com.pulumi.gcp.firestore.DatabaseArgs;
* import com.pulumi.gcp.firestore.Field;
* import com.pulumi.gcp.firestore.FieldArgs;
* import com.pulumi.gcp.firestore.inputs.FieldTtlConfigArgs;
* import com.pulumi.gcp.firestore.inputs.FieldIndexConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var database = new Database("database", DatabaseArgs.builder()
* .project("my-project-name")
* .name("database-id")
* .locationId("nam5")
* .type("FIRESTORE_NATIVE")
* .deleteProtectionState("DELETE_PROTECTION_ENABLED")
* .deletionPolicy("DELETE")
* .build());
* var timestamp = new Field("timestamp", FieldArgs.builder()
* .project("my-project-name")
* .database(database.name())
* .collection("chatrooms")
* .field("timestamp")
* .ttlConfig()
* .indexConfig()
* .build());
* }
* }
* ```
* ```yaml
* resources:
* database:
* type: gcp:firestore:Database
* properties:
* project: my-project-name
* name: database-id
* locationId: nam5
* type: FIRESTORE_NATIVE
* deleteProtectionState: DELETE_PROTECTION_ENABLED
* deletionPolicy: DELETE
* timestamp:
* type: gcp:firestore:Field
* properties:
* project: my-project-name
* database: ${database.name}
* collection: chatrooms
* field: timestamp
* ttlConfig: {}
* indexConfig: {}
* ```
*
* ### Firestore Field Match Override
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const database = new gcp.firestore.Database("database", {
* project: "my-project-name",
* name: "database-id",
* locationId: "nam5",
* type: "FIRESTORE_NATIVE",
* deleteProtectionState: "DELETE_PROTECTION_ENABLED",
* deletionPolicy: "DELETE",
* });
* const matchOverride = new gcp.firestore.Field("match_override", {
* project: "my-project-name",
* database: database.name,
* collection: "chatrooms__75125",
* field: "field_with_same_configuration_as_ancestor",
* indexConfig: {
* indexes: [
* {
* order: "ASCENDING",
* },
* {
* order: "DESCENDING",
* },
* {
* arrayConfig: "CONTAINS",
* },
* ],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* database = gcp.firestore.Database("database",
* project="my-project-name",
* name="database-id",
* location_id="nam5",
* type="FIRESTORE_NATIVE",
* delete_protection_state="DELETE_PROTECTION_ENABLED",
* deletion_policy="DELETE")
* match_override = gcp.firestore.Field("match_override",
* project="my-project-name",
* database=database.name,
* collection="chatrooms__75125",
* field="field_with_same_configuration_as_ancestor",
* index_config=gcp.firestore.FieldIndexConfigArgs(
* indexes=[
* gcp.firestore.FieldIndexConfigIndexArgs(
* order="ASCENDING",
* ),
* gcp.firestore.FieldIndexConfigIndexArgs(
* order="DESCENDING",
* ),
* gcp.firestore.FieldIndexConfigIndexArgs(
* array_config="CONTAINS",
* ),
* ],
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var database = new Gcp.Firestore.Database("database", new()
* {
* Project = "my-project-name",
* Name = "database-id",
* LocationId = "nam5",
* Type = "FIRESTORE_NATIVE",
* DeleteProtectionState = "DELETE_PROTECTION_ENABLED",
* DeletionPolicy = "DELETE",
* });
* var matchOverride = new Gcp.Firestore.Field("match_override", new()
* {
* Project = "my-project-name",
* Database = database.Name,
* Collection = "chatrooms__75125",
* FieldId = "field_with_same_configuration_as_ancestor",
* IndexConfig = new Gcp.Firestore.Inputs.FieldIndexConfigArgs
* {
* Indexes = new[]
* {
* new Gcp.Firestore.Inputs.FieldIndexConfigIndexArgs
* {
* Order = "ASCENDING",
* },
* new Gcp.Firestore.Inputs.FieldIndexConfigIndexArgs
* {
* Order = "DESCENDING",
* },
* new Gcp.Firestore.Inputs.FieldIndexConfigIndexArgs
* {
* ArrayConfig = "CONTAINS",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/firestore"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* database, err := firestore.NewDatabase(ctx, "database", &firestore.DatabaseArgs{
* Project: pulumi.String("my-project-name"),
* Name: pulumi.String("database-id"),
* LocationId: pulumi.String("nam5"),
* Type: pulumi.String("FIRESTORE_NATIVE"),
* DeleteProtectionState: pulumi.String("DELETE_PROTECTION_ENABLED"),
* DeletionPolicy: pulumi.String("DELETE"),
* })
* if err != nil {
* return err
* }
* _, err = firestore.NewField(ctx, "match_override", &firestore.FieldArgs{
* Project: pulumi.String("my-project-name"),
* Database: database.Name,
* Collection: pulumi.String("chatrooms__75125"),
* Field: pulumi.String("field_with_same_configuration_as_ancestor"),
* IndexConfig: &firestore.FieldIndexConfigArgs{
* Indexes: firestore.FieldIndexConfigIndexArray{
* &firestore.FieldIndexConfigIndexArgs{
* Order: pulumi.String("ASCENDING"),
* },
* &firestore.FieldIndexConfigIndexArgs{
* Order: pulumi.String("DESCENDING"),
* },
* &firestore.FieldIndexConfigIndexArgs{
* ArrayConfig: pulumi.String("CONTAINS"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.firestore.Database;
* import com.pulumi.gcp.firestore.DatabaseArgs;
* import com.pulumi.gcp.firestore.Field;
* import com.pulumi.gcp.firestore.FieldArgs;
* import com.pulumi.gcp.firestore.inputs.FieldIndexConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var database = new Database("database", DatabaseArgs.builder()
* .project("my-project-name")
* .name("database-id")
* .locationId("nam5")
* .type("FIRESTORE_NATIVE")
* .deleteProtectionState("DELETE_PROTECTION_ENABLED")
* .deletionPolicy("DELETE")
* .build());
* var matchOverride = new Field("matchOverride", FieldArgs.builder()
* .project("my-project-name")
* .database(database.name())
* .collection("chatrooms__75125")
* .field("field_with_same_configuration_as_ancestor")
* .indexConfig(FieldIndexConfigArgs.builder()
* .indexes(
* FieldIndexConfigIndexArgs.builder()
* .order("ASCENDING")
* .build(),
* FieldIndexConfigIndexArgs.builder()
* .order("DESCENDING")
* .build(),
* FieldIndexConfigIndexArgs.builder()
* .arrayConfig("CONTAINS")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* database:
* type: gcp:firestore:Database
* properties:
* project: my-project-name
* name: database-id
* locationId: nam5
* type: FIRESTORE_NATIVE
* deleteProtectionState: DELETE_PROTECTION_ENABLED
* deletionPolicy: DELETE
* matchOverride:
* type: gcp:firestore:Field
* name: match_override
* properties:
* project: my-project-name
* database: ${database.name}
* collection: chatrooms__75125
* field: field_with_same_configuration_as_ancestor
* indexConfig:
* indexes:
* - order: ASCENDING
* - order: DESCENDING
* - arrayConfig: CONTAINS
* ```
*
* ## Import
* Field can be imported using any of these accepted formats:
* * `{{name}}`
* When using the `pulumi import` command, Field can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:firestore/field:Field default {{name}}
* ```
* @property collection The id of the collection group to configure.
* @property database The Firestore database id. Defaults to `"(default)"`.
* @property field The id of the field to configure.
* - - -
* @property indexConfig The single field index configuration for this field.
* Creating an index configuration for this field will override any inherited configuration with the
* indexes specified. Configuring the index configuration with an empty block disables all indexes on
* the field.
* Structure is documented below.
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property ttlConfig The TTL configuration for this Field. If set to an empty block (i.e. `ttl_config {}`), a TTL policy is configured based on the field. If unset, a TTL policy is not configured (or will be disabled upon updating the resource).
* Structure is documented below.
*/
public data class FieldArgs(
public val collection: Output? = null,
public val database: Output? = null,
public val `field`: Output? = null,
public val indexConfig: Output? = null,
public val project: Output? = null,
public val ttlConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.firestore.FieldArgs =
com.pulumi.gcp.firestore.FieldArgs.builder()
.collection(collection?.applyValue({ args0 -> args0 }))
.database(database?.applyValue({ args0 -> args0 }))
.`field`(`field`?.applyValue({ args0 -> args0 }))
.indexConfig(indexConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.project(project?.applyValue({ args0 -> args0 }))
.ttlConfig(ttlConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [FieldArgs].
*/
@PulumiTagMarker
public class FieldArgsBuilder internal constructor() {
private var collection: Output? = null
private var database: Output? = null
private var `field`: Output? = null
private var indexConfig: Output? = null
private var project: Output? = null
private var ttlConfig: Output? = null
/**
* @param value The id of the collection group to configure.
*/
@JvmName("ugevlkuudkngpjbx")
public suspend fun collection(`value`: Output) {
this.collection = value
}
/**
* @param value The Firestore database id. Defaults to `"(default)"`.
*/
@JvmName("tonximutcukjfrwa")
public suspend fun database(`value`: Output) {
this.database = value
}
/**
* @param value The id of the field to configure.
* - - -
*/
@JvmName("fxyqugpeesyimxvo")
public suspend fun `field`(`value`: Output) {
this.`field` = value
}
/**
* @param value The single field index configuration for this field.
* Creating an index configuration for this field will override any inherited configuration with the
* indexes specified. Configuring the index configuration with an empty block disables all indexes on
* the field.
* Structure is documented below.
*/
@JvmName("gbntbwhjqlxknjkk")
public suspend fun indexConfig(`value`: Output) {
this.indexConfig = value
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("qmxpnslyuapqatba")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The TTL configuration for this Field. If set to an empty block (i.e. `ttl_config {}`), a TTL policy is configured based on the field. If unset, a TTL policy is not configured (or will be disabled upon updating the resource).
* Structure is documented below.
*/
@JvmName("xaiyeeeohbiswobn")
public suspend fun ttlConfig(`value`: Output) {
this.ttlConfig = value
}
/**
* @param value The id of the collection group to configure.
*/
@JvmName("mctcghjhvpyqolhq")
public suspend fun collection(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.collection = mapped
}
/**
* @param value The Firestore database id. Defaults to `"(default)"`.
*/
@JvmName("ntkdqrqbqlhpksca")
public suspend fun database(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.database = mapped
}
/**
* @param value The id of the field to configure.
* - - -
*/
@JvmName("tpgmardprnqtoyny")
public suspend fun `field`(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`field` = mapped
}
/**
* @param value The single field index configuration for this field.
* Creating an index configuration for this field will override any inherited configuration with the
* indexes specified. Configuring the index configuration with an empty block disables all indexes on
* the field.
* Structure is documented below.
*/
@JvmName("tltcokxwdhtcympo")
public suspend fun indexConfig(`value`: FieldIndexConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.indexConfig = mapped
}
/**
* @param argument The single field index configuration for this field.
* Creating an index configuration for this field will override any inherited configuration with the
* indexes specified. Configuring the index configuration with an empty block disables all indexes on
* the field.
* Structure is documented below.
*/
@JvmName("iwnkqcyhofmajpnk")
public suspend fun indexConfig(argument: suspend FieldIndexConfigArgsBuilder.() -> Unit) {
val toBeMapped = FieldIndexConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.indexConfig = mapped
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("mmhmomhlititwgkg")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value The TTL configuration for this Field. If set to an empty block (i.e. `ttl_config {}`), a TTL policy is configured based on the field. If unset, a TTL policy is not configured (or will be disabled upon updating the resource).
* Structure is documented below.
*/
@JvmName("rnhuexjigwnanffv")
public suspend fun ttlConfig(`value`: FieldTtlConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ttlConfig = mapped
}
/**
* @param argument The TTL configuration for this Field. If set to an empty block (i.e. `ttl_config {}`), a TTL policy is configured based on the field. If unset, a TTL policy is not configured (or will be disabled upon updating the resource).
* Structure is documented below.
*/
@JvmName("mxorpnibgptkivaf")
public suspend fun ttlConfig(argument: suspend FieldTtlConfigArgsBuilder.() -> Unit) {
val toBeMapped = FieldTtlConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.ttlConfig = mapped
}
internal fun build(): FieldArgs = FieldArgs(
collection = collection,
database = database,
`field` = `field`,
indexConfig = indexConfig,
project = project,
ttlConfig = ttlConfig,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy