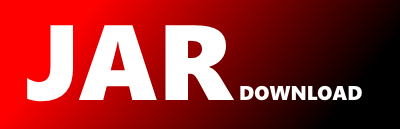
com.pulumi.gcp.firestore.kotlin.inputs.IndexFieldArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.firestore.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.firestore.inputs.IndexFieldArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property arrayConfig Indicates that this field supports operations on arrayValues. Only one of `order`, `arrayConfig`, and
* `vectorConfig` can be specified.
* Possible values are: `CONTAINS`.
* @property fieldPath Name of the field.
* @property order Indicates that this field supports ordering by the specified order or comparing using =, <, <=, >, >=.
* Only one of `order`, `arrayConfig`, and `vectorConfig` can be specified.
* Possible values are: `ASCENDING`, `DESCENDING`.
* @property vectorConfig Indicates that this field supports vector search operations. Only one of `order`, `arrayConfig`, and
* `vectorConfig` can be specified. Vector Fields should come after the field path `__name__`.
* Structure is documented below.
*/
public data class IndexFieldArgs(
public val arrayConfig: Output? = null,
public val fieldPath: Output? = null,
public val order: Output? = null,
public val vectorConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.firestore.inputs.IndexFieldArgs =
com.pulumi.gcp.firestore.inputs.IndexFieldArgs.builder()
.arrayConfig(arrayConfig?.applyValue({ args0 -> args0 }))
.fieldPath(fieldPath?.applyValue({ args0 -> args0 }))
.order(order?.applyValue({ args0 -> args0 }))
.vectorConfig(vectorConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [IndexFieldArgs].
*/
@PulumiTagMarker
public class IndexFieldArgsBuilder internal constructor() {
private var arrayConfig: Output? = null
private var fieldPath: Output? = null
private var order: Output? = null
private var vectorConfig: Output? = null
/**
* @param value Indicates that this field supports operations on arrayValues. Only one of `order`, `arrayConfig`, and
* `vectorConfig` can be specified.
* Possible values are: `CONTAINS`.
*/
@JvmName("onodshhhobqexmsx")
public suspend fun arrayConfig(`value`: Output) {
this.arrayConfig = value
}
/**
* @param value Name of the field.
*/
@JvmName("rhaphaajeisbdayp")
public suspend fun fieldPath(`value`: Output) {
this.fieldPath = value
}
/**
* @param value Indicates that this field supports ordering by the specified order or comparing using =, <, <=, >, >=.
* Only one of `order`, `arrayConfig`, and `vectorConfig` can be specified.
* Possible values are: `ASCENDING`, `DESCENDING`.
*/
@JvmName("hcclwobgooatdcbw")
public suspend fun order(`value`: Output) {
this.order = value
}
/**
* @param value Indicates that this field supports vector search operations. Only one of `order`, `arrayConfig`, and
* `vectorConfig` can be specified. Vector Fields should come after the field path `__name__`.
* Structure is documented below.
*/
@JvmName("ggitvlgyoqowqgdi")
public suspend fun vectorConfig(`value`: Output) {
this.vectorConfig = value
}
/**
* @param value Indicates that this field supports operations on arrayValues. Only one of `order`, `arrayConfig`, and
* `vectorConfig` can be specified.
* Possible values are: `CONTAINS`.
*/
@JvmName("abepqnlsunmomwod")
public suspend fun arrayConfig(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.arrayConfig = mapped
}
/**
* @param value Name of the field.
*/
@JvmName("dvtahuiherstykgy")
public suspend fun fieldPath(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fieldPath = mapped
}
/**
* @param value Indicates that this field supports ordering by the specified order or comparing using =, <, <=, >, >=.
* Only one of `order`, `arrayConfig`, and `vectorConfig` can be specified.
* Possible values are: `ASCENDING`, `DESCENDING`.
*/
@JvmName("vtqapmxoddmvrrbi")
public suspend fun order(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.order = mapped
}
/**
* @param value Indicates that this field supports vector search operations. Only one of `order`, `arrayConfig`, and
* `vectorConfig` can be specified. Vector Fields should come after the field path `__name__`.
* Structure is documented below.
*/
@JvmName("lwtrluucjsbxjjfj")
public suspend fun vectorConfig(`value`: IndexFieldVectorConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vectorConfig = mapped
}
/**
* @param argument Indicates that this field supports vector search operations. Only one of `order`, `arrayConfig`, and
* `vectorConfig` can be specified. Vector Fields should come after the field path `__name__`.
* Structure is documented below.
*/
@JvmName("fyvlplkksjsefhyq")
public suspend fun vectorConfig(argument: suspend IndexFieldVectorConfigArgsBuilder.() -> Unit) {
val toBeMapped = IndexFieldVectorConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.vectorConfig = mapped
}
internal fun build(): IndexFieldArgs = IndexFieldArgs(
arrayConfig = arrayConfig,
fieldPath = fieldPath,
order = order,
vectorConfig = vectorConfig,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy