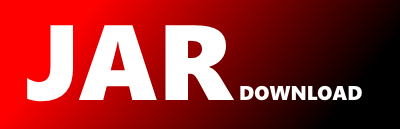
com.pulumi.gcp.folder.kotlin.AccessApprovalSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.folder.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.folder.AccessApprovalSettingsArgs.builder
import com.pulumi.gcp.folder.kotlin.inputs.AccessApprovalSettingsEnrolledServiceArgs
import com.pulumi.gcp.folder.kotlin.inputs.AccessApprovalSettingsEnrolledServiceArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Access Approval enables you to require your explicit approval whenever Google support and engineering need to access your customer content.
* To get more information about FolderSettings, see:
* * [API documentation](https://cloud.google.com/access-approval/docs/reference/rest/v1/folders)
* ## Example Usage
* ### Folder Access Approval Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const myFolder = new gcp.organizations.Folder("my_folder", {
* displayName: "my-folder",
* parent: "organizations/123456789",
* });
* const folderAccessApproval = new gcp.folder.AccessApprovalSettings("folder_access_approval", {
* folderId: myFolder.folderId,
* notificationEmails: [
* "[email protected]",
* "[email protected]",
* ],
* enrolledServices: [{
* cloudProduct: "all",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_folder = gcp.organizations.Folder("my_folder",
* display_name="my-folder",
* parent="organizations/123456789")
* folder_access_approval = gcp.folder.AccessApprovalSettings("folder_access_approval",
* folder_id=my_folder.folder_id,
* notification_emails=[
* "[email protected]",
* "[email protected]",
* ],
* enrolled_services=[gcp.folder.AccessApprovalSettingsEnrolledServiceArgs(
* cloud_product="all",
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var myFolder = new Gcp.Organizations.Folder("my_folder", new()
* {
* DisplayName = "my-folder",
* Parent = "organizations/123456789",
* });
* var folderAccessApproval = new Gcp.Folder.AccessApprovalSettings("folder_access_approval", new()
* {
* FolderId = myFolder.FolderId,
* NotificationEmails = new[]
* {
* "[email protected]",
* "[email protected]",
* },
* EnrolledServices = new[]
* {
* new Gcp.Folder.Inputs.AccessApprovalSettingsEnrolledServiceArgs
* {
* CloudProduct = "all",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/folder"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* myFolder, err := organizations.NewFolder(ctx, "my_folder", &organizations.FolderArgs{
* DisplayName: pulumi.String("my-folder"),
* Parent: pulumi.String("organizations/123456789"),
* })
* if err != nil {
* return err
* }
* _, err = folder.NewAccessApprovalSettings(ctx, "folder_access_approval", &folder.AccessApprovalSettingsArgs{
* FolderId: myFolder.FolderId,
* NotificationEmails: pulumi.StringArray{
* pulumi.String("[email protected]"),
* pulumi.String("[email protected]"),
* },
* EnrolledServices: folder.AccessApprovalSettingsEnrolledServiceArray{
* &folder.AccessApprovalSettingsEnrolledServiceArgs{
* CloudProduct: pulumi.String("all"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.Folder;
* import com.pulumi.gcp.organizations.FolderArgs;
* import com.pulumi.gcp.folder.AccessApprovalSettings;
* import com.pulumi.gcp.folder.AccessApprovalSettingsArgs;
* import com.pulumi.gcp.folder.inputs.AccessApprovalSettingsEnrolledServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var myFolder = new Folder("myFolder", FolderArgs.builder()
* .displayName("my-folder")
* .parent("organizations/123456789")
* .build());
* var folderAccessApproval = new AccessApprovalSettings("folderAccessApproval", AccessApprovalSettingsArgs.builder()
* .folderId(myFolder.folderId())
* .notificationEmails(
* "[email protected]",
* "[email protected]")
* .enrolledServices(AccessApprovalSettingsEnrolledServiceArgs.builder()
* .cloudProduct("all")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* myFolder:
* type: gcp:organizations:Folder
* name: my_folder
* properties:
* displayName: my-folder
* parent: organizations/123456789
* folderAccessApproval:
* type: gcp:folder:AccessApprovalSettings
* name: folder_access_approval
* properties:
* folderId: ${myFolder.folderId}
* notificationEmails:
* - [email protected]
* - [email protected]
* enrolledServices:
* - cloudProduct: all
* ```
*
* ### Folder Access Approval Active Key Version
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const myFolder = new gcp.organizations.Folder("my_folder", {
* displayName: "my-folder",
* parent: "organizations/123456789",
* });
* const myProject = new gcp.organizations.Project("my_project", {
* name: "My Project",
* projectId: "your-project-id",
* folderId: myFolder.name,
* });
* const keyRing = new gcp.kms.KeyRing("key_ring", {
* name: "key-ring",
* location: "global",
* project: myProject.projectId,
* });
* const cryptoKey = new gcp.kms.CryptoKey("crypto_key", {
* name: "crypto-key",
* keyRing: keyRing.id,
* purpose: "ASYMMETRIC_SIGN",
* versionTemplate: {
* algorithm: "EC_SIGN_P384_SHA384",
* },
* });
* const serviceAccount = gcp.accessapproval.getFolderServiceAccountOutput({
* folderId: myFolder.folderId,
* });
* const iam = new gcp.kms.CryptoKeyIAMMember("iam", {
* cryptoKeyId: cryptoKey.id,
* role: "roles/cloudkms.signerVerifier",
* member: serviceAccount.apply(serviceAccount => `serviceAccount:${serviceAccount.accountEmail}`),
* });
* const cryptoKeyVersion = gcp.kms.getKMSCryptoKeyVersionOutput({
* cryptoKey: cryptoKey.id,
* });
* const folderAccessApproval = new gcp.folder.AccessApprovalSettings("folder_access_approval", {
* folderId: myFolder.folderId,
* activeKeyVersion: cryptoKeyVersion.apply(cryptoKeyVersion => cryptoKeyVersion.name),
* enrolledServices: [{
* cloudProduct: "all",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_folder = gcp.organizations.Folder("my_folder",
* display_name="my-folder",
* parent="organizations/123456789")
* my_project = gcp.organizations.Project("my_project",
* name="My Project",
* project_id="your-project-id",
* folder_id=my_folder.name)
* key_ring = gcp.kms.KeyRing("key_ring",
* name="key-ring",
* location="global",
* project=my_project.project_id)
* crypto_key = gcp.kms.CryptoKey("crypto_key",
* name="crypto-key",
* key_ring=key_ring.id,
* purpose="ASYMMETRIC_SIGN",
* version_template=gcp.kms.CryptoKeyVersionTemplateArgs(
* algorithm="EC_SIGN_P384_SHA384",
* ))
* service_account = gcp.accessapproval.get_folder_service_account_output(folder_id=my_folder.folder_id)
* iam = gcp.kms.CryptoKeyIAMMember("iam",
* crypto_key_id=crypto_key.id,
* role="roles/cloudkms.signerVerifier",
* member=service_account.apply(lambda service_account: f"serviceAccount:{service_account.account_email}"))
* crypto_key_version = gcp.kms.get_kms_crypto_key_version_output(crypto_key=crypto_key.id)
* folder_access_approval = gcp.folder.AccessApprovalSettings("folder_access_approval",
* folder_id=my_folder.folder_id,
* active_key_version=crypto_key_version.name,
* enrolled_services=[gcp.folder.AccessApprovalSettingsEnrolledServiceArgs(
* cloud_product="all",
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var myFolder = new Gcp.Organizations.Folder("my_folder", new()
* {
* DisplayName = "my-folder",
* Parent = "organizations/123456789",
* });
* var myProject = new Gcp.Organizations.Project("my_project", new()
* {
* Name = "My Project",
* ProjectId = "your-project-id",
* FolderId = myFolder.Name,
* });
* var keyRing = new Gcp.Kms.KeyRing("key_ring", new()
* {
* Name = "key-ring",
* Location = "global",
* Project = myProject.ProjectId,
* });
* var cryptoKey = new Gcp.Kms.CryptoKey("crypto_key", new()
* {
* Name = "crypto-key",
* KeyRing = keyRing.Id,
* Purpose = "ASYMMETRIC_SIGN",
* VersionTemplate = new Gcp.Kms.Inputs.CryptoKeyVersionTemplateArgs
* {
* Algorithm = "EC_SIGN_P384_SHA384",
* },
* });
* var serviceAccount = Gcp.AccessApproval.GetFolderServiceAccount.Invoke(new()
* {
* FolderId = myFolder.FolderId,
* });
* var iam = new Gcp.Kms.CryptoKeyIAMMember("iam", new()
* {
* CryptoKeyId = cryptoKey.Id,
* Role = "roles/cloudkms.signerVerifier",
* Member = $"serviceAccount:{serviceAccount.Apply(getFolderServiceAccountResult => getFolderServiceAccountResult.AccountEmail)}",
* });
* var cryptoKeyVersion = Gcp.Kms.GetKMSCryptoKeyVersion.Invoke(new()
* {
* CryptoKey = cryptoKey.Id,
* });
* var folderAccessApproval = new Gcp.Folder.AccessApprovalSettings("folder_access_approval", new()
* {
* FolderId = myFolder.FolderId,
* ActiveKeyVersion = cryptoKeyVersion.Apply(getKMSCryptoKeyVersionResult => getKMSCryptoKeyVersionResult.Name),
* EnrolledServices = new[]
* {
* new Gcp.Folder.Inputs.AccessApprovalSettingsEnrolledServiceArgs
* {
* CloudProduct = "all",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/accessapproval"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/folder"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/kms"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* myFolder, err := organizations.NewFolder(ctx, "my_folder", &organizations.FolderArgs{
* DisplayName: pulumi.String("my-folder"),
* Parent: pulumi.String("organizations/123456789"),
* })
* if err != nil {
* return err
* }
* myProject, err := organizations.NewProject(ctx, "my_project", &organizations.ProjectArgs{
* Name: pulumi.String("My Project"),
* ProjectId: pulumi.String("your-project-id"),
* FolderId: myFolder.Name,
* })
* if err != nil {
* return err
* }
* keyRing, err := kms.NewKeyRing(ctx, "key_ring", &kms.KeyRingArgs{
* Name: pulumi.String("key-ring"),
* Location: pulumi.String("global"),
* Project: myProject.ProjectId,
* })
* if err != nil {
* return err
* }
* cryptoKey, err := kms.NewCryptoKey(ctx, "crypto_key", &kms.CryptoKeyArgs{
* Name: pulumi.String("crypto-key"),
* KeyRing: keyRing.ID(),
* Purpose: pulumi.String("ASYMMETRIC_SIGN"),
* VersionTemplate: &kms.CryptoKeyVersionTemplateArgs{
* Algorithm: pulumi.String("EC_SIGN_P384_SHA384"),
* },
* })
* if err != nil {
* return err
* }
* serviceAccount := accessapproval.GetFolderServiceAccountOutput(ctx, accessapproval.GetFolderServiceAccountOutputArgs{
* FolderId: myFolder.FolderId,
* }, nil)
* _, err = kms.NewCryptoKeyIAMMember(ctx, "iam", &kms.CryptoKeyIAMMemberArgs{
* CryptoKeyId: cryptoKey.ID(),
* Role: pulumi.String("roles/cloudkms.signerVerifier"),
* Member: serviceAccount.ApplyT(func(serviceAccount accessapproval.GetFolderServiceAccountResult) (string, error) {
* return fmt.Sprintf("serviceAccount:%v", serviceAccount.AccountEmail), nil
* }).(pulumi.StringOutput),
* })
* if err != nil {
* return err
* }
* cryptoKeyVersion := kms.GetKMSCryptoKeyVersionOutput(ctx, kms.GetKMSCryptoKeyVersionOutputArgs{
* CryptoKey: cryptoKey.ID(),
* }, nil)
* _, err = folder.NewAccessApprovalSettings(ctx, "folder_access_approval", &folder.AccessApprovalSettingsArgs{
* FolderId: myFolder.FolderId,
* ActiveKeyVersion: cryptoKeyVersion.ApplyT(func(cryptoKeyVersion kms.GetKMSCryptoKeyVersionResult) (*string, error) {
* return &cryptoKeyVersion.Name, nil
* }).(pulumi.StringPtrOutput),
* EnrolledServices: folder.AccessApprovalSettingsEnrolledServiceArray{
* &folder.AccessApprovalSettingsEnrolledServiceArgs{
* CloudProduct: pulumi.String("all"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.Folder;
* import com.pulumi.gcp.organizations.FolderArgs;
* import com.pulumi.gcp.organizations.Project;
* import com.pulumi.gcp.organizations.ProjectArgs;
* import com.pulumi.gcp.kms.KeyRing;
* import com.pulumi.gcp.kms.KeyRingArgs;
* import com.pulumi.gcp.kms.CryptoKey;
* import com.pulumi.gcp.kms.CryptoKeyArgs;
* import com.pulumi.gcp.kms.inputs.CryptoKeyVersionTemplateArgs;
* import com.pulumi.gcp.accessapproval.AccessapprovalFunctions;
* import com.pulumi.gcp.accessapproval.inputs.GetFolderServiceAccountArgs;
* import com.pulumi.gcp.kms.CryptoKeyIAMMember;
* import com.pulumi.gcp.kms.CryptoKeyIAMMemberArgs;
* import com.pulumi.gcp.kms.KmsFunctions;
* import com.pulumi.gcp.kms.inputs.GetKMSCryptoKeyVersionArgs;
* import com.pulumi.gcp.folder.AccessApprovalSettings;
* import com.pulumi.gcp.folder.AccessApprovalSettingsArgs;
* import com.pulumi.gcp.folder.inputs.AccessApprovalSettingsEnrolledServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var myFolder = new Folder("myFolder", FolderArgs.builder()
* .displayName("my-folder")
* .parent("organizations/123456789")
* .build());
* var myProject = new Project("myProject", ProjectArgs.builder()
* .name("My Project")
* .projectId("your-project-id")
* .folderId(myFolder.name())
* .build());
* var keyRing = new KeyRing("keyRing", KeyRingArgs.builder()
* .name("key-ring")
* .location("global")
* .project(myProject.projectId())
* .build());
* var cryptoKey = new CryptoKey("cryptoKey", CryptoKeyArgs.builder()
* .name("crypto-key")
* .keyRing(keyRing.id())
* .purpose("ASYMMETRIC_SIGN")
* .versionTemplate(CryptoKeyVersionTemplateArgs.builder()
* .algorithm("EC_SIGN_P384_SHA384")
* .build())
* .build());
* final var serviceAccount = AccessapprovalFunctions.getFolderServiceAccount(GetFolderServiceAccountArgs.builder()
* .folderId(myFolder.folderId())
* .build());
* var iam = new CryptoKeyIAMMember("iam", CryptoKeyIAMMemberArgs.builder()
* .cryptoKeyId(cryptoKey.id())
* .role("roles/cloudkms.signerVerifier")
* .member(serviceAccount.applyValue(getFolderServiceAccountResult -> getFolderServiceAccountResult).applyValue(serviceAccount -> String.format("serviceAccount:%s", serviceAccount.applyValue(getFolderServiceAccountResult -> getFolderServiceAccountResult.accountEmail()))))
* .build());
* final var cryptoKeyVersion = KmsFunctions.getKMSCryptoKeyVersion(GetKMSCryptoKeyVersionArgs.builder()
* .cryptoKey(cryptoKey.id())
* .build());
* var folderAccessApproval = new AccessApprovalSettings("folderAccessApproval", AccessApprovalSettingsArgs.builder()
* .folderId(myFolder.folderId())
* .activeKeyVersion(cryptoKeyVersion.applyValue(getKMSCryptoKeyVersionResult -> getKMSCryptoKeyVersionResult).applyValue(cryptoKeyVersion -> cryptoKeyVersion.applyValue(getKMSCryptoKeyVersionResult -> getKMSCryptoKeyVersionResult.name())))
* .enrolledServices(AccessApprovalSettingsEnrolledServiceArgs.builder()
* .cloudProduct("all")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* myFolder:
* type: gcp:organizations:Folder
* name: my_folder
* properties:
* displayName: my-folder
* parent: organizations/123456789
* myProject:
* type: gcp:organizations:Project
* name: my_project
* properties:
* name: My Project
* projectId: your-project-id
* folderId: ${myFolder.name}
* keyRing:
* type: gcp:kms:KeyRing
* name: key_ring
* properties:
* name: key-ring
* location: global
* project: ${myProject.projectId}
* cryptoKey:
* type: gcp:kms:CryptoKey
* name: crypto_key
* properties:
* name: crypto-key
* keyRing: ${keyRing.id}
* purpose: ASYMMETRIC_SIGN
* versionTemplate:
* algorithm: EC_SIGN_P384_SHA384
* iam:
* type: gcp:kms:CryptoKeyIAMMember
* properties:
* cryptoKeyId: ${cryptoKey.id}
* role: roles/cloudkms.signerVerifier
* member: serviceAccount:${serviceAccount.accountEmail}
* folderAccessApproval:
* type: gcp:folder:AccessApprovalSettings
* name: folder_access_approval
* properties:
* folderId: ${myFolder.folderId}
* activeKeyVersion: ${cryptoKeyVersion.name}
* enrolledServices:
* - cloudProduct: all
* variables:
* serviceAccount:
* fn::invoke:
* Function: gcp:accessapproval:getFolderServiceAccount
* Arguments:
* folderId: ${myFolder.folderId}
* cryptoKeyVersion:
* fn::invoke:
* Function: gcp:kms:getKMSCryptoKeyVersion
* Arguments:
* cryptoKey: ${cryptoKey.id}
* ```
*
* ## Import
* FolderSettings can be imported using any of these accepted formats:
* * `folders/{{folder_id}}/accessApprovalSettings`
* * `{{folder_id}}`
* When using the `pulumi import` command, FolderSettings can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:folder/accessApprovalSettings:AccessApprovalSettings default folders/{{folder_id}}/accessApprovalSettings
* ```
* ```sh
* $ pulumi import gcp:folder/accessApprovalSettings:AccessApprovalSettings default {{folder_id}}
* ```
* @property activeKeyVersion The asymmetric crypto key version to use for signing approval requests. Empty active_key_version indicates that a
* Google-managed key should be used for signing. This property will be ignored if set by an ancestor of the resource, and
* new non-empty values may not be set.
* @property enrolledServices A list of Google Cloud Services for which the given resource has Access Approval enrolled.
* Access requests for the resource given by name against any of these services contained here will be required
* to have explicit approval. Enrollment can only be done on an all or nothing basis.
* A maximum of 10 enrolled services will be enforced, to be expanded as the set of supported services is expanded.
* Structure is documented below.
* @property folderId ID of the folder of the access approval settings.
* @property notificationEmails A list of email addresses to which notifications relating to approval requests should be sent. Notifications relating to
* a resource will be sent to all emails in the settings of ancestor resources of that resource. A maximum of 50 email
* addresses are allowed.
*/
public data class AccessApprovalSettingsArgs(
public val activeKeyVersion: Output? = null,
public val enrolledServices: Output>? = null,
public val folderId: Output? = null,
public val notificationEmails: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.folder.AccessApprovalSettingsArgs =
com.pulumi.gcp.folder.AccessApprovalSettingsArgs.builder()
.activeKeyVersion(activeKeyVersion?.applyValue({ args0 -> args0 }))
.enrolledServices(
enrolledServices?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.folderId(folderId?.applyValue({ args0 -> args0 }))
.notificationEmails(
notificationEmails?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
).build()
}
/**
* Builder for [AccessApprovalSettingsArgs].
*/
@PulumiTagMarker
public class AccessApprovalSettingsArgsBuilder internal constructor() {
private var activeKeyVersion: Output? = null
private var enrolledServices: Output>? = null
private var folderId: Output? = null
private var notificationEmails: Output>? = null
/**
* @param value The asymmetric crypto key version to use for signing approval requests. Empty active_key_version indicates that a
* Google-managed key should be used for signing. This property will be ignored if set by an ancestor of the resource, and
* new non-empty values may not be set.
*/
@JvmName("ebnvwlslkyyggtrs")
public suspend fun activeKeyVersion(`value`: Output) {
this.activeKeyVersion = value
}
/**
* @param value A list of Google Cloud Services for which the given resource has Access Approval enrolled.
* Access requests for the resource given by name against any of these services contained here will be required
* to have explicit approval. Enrollment can only be done on an all or nothing basis.
* A maximum of 10 enrolled services will be enforced, to be expanded as the set of supported services is expanded.
* Structure is documented below.
*/
@JvmName("uuyxrrriqqlujkoq")
public suspend fun enrolledServices(`value`: Output>) {
this.enrolledServices = value
}
@JvmName("ybplnwlyxuvwptng")
public suspend fun enrolledServices(vararg values: Output) {
this.enrolledServices = Output.all(values.asList())
}
/**
* @param values A list of Google Cloud Services for which the given resource has Access Approval enrolled.
* Access requests for the resource given by name against any of these services contained here will be required
* to have explicit approval. Enrollment can only be done on an all or nothing basis.
* A maximum of 10 enrolled services will be enforced, to be expanded as the set of supported services is expanded.
* Structure is documented below.
*/
@JvmName("sbbfahhybnqwsobk")
public suspend fun enrolledServices(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy