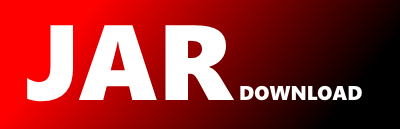
com.pulumi.gcp.gkebackup.kotlin.inputs.BackupPlanBackupConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.gkebackup.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.gkebackup.inputs.BackupPlanBackupConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property allNamespaces If True, include all namespaced resources.
* @property encryptionKey This defines a customer managed encryption key that will be used to encrypt the "config"
* portion (the Kubernetes resources) of Backups created via this plan.
* Structure is documented below.
* @property includeSecrets This flag specifies whether Kubernetes Secret resources should be included
* when they fall into the scope of Backups.
* @property includeVolumeData This flag specifies whether volume data should be backed up when PVCs are
* included in the scope of a Backup.
* @property selectedApplications A list of namespaced Kubernetes Resources.
* Structure is documented below.
* @property selectedNamespaces If set, include just the resources in the listed namespaces.
* Structure is documented below.
*/
public data class BackupPlanBackupConfigArgs(
public val allNamespaces: Output? = null,
public val encryptionKey: Output? = null,
public val includeSecrets: Output? = null,
public val includeVolumeData: Output? = null,
public val selectedApplications: Output? = null,
public val selectedNamespaces: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.gkebackup.inputs.BackupPlanBackupConfigArgs =
com.pulumi.gcp.gkebackup.inputs.BackupPlanBackupConfigArgs.builder()
.allNamespaces(allNamespaces?.applyValue({ args0 -> args0 }))
.encryptionKey(encryptionKey?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.includeSecrets(includeSecrets?.applyValue({ args0 -> args0 }))
.includeVolumeData(includeVolumeData?.applyValue({ args0 -> args0 }))
.selectedApplications(
selectedApplications?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.selectedNamespaces(
selectedNamespaces?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [BackupPlanBackupConfigArgs].
*/
@PulumiTagMarker
public class BackupPlanBackupConfigArgsBuilder internal constructor() {
private var allNamespaces: Output? = null
private var encryptionKey: Output? = null
private var includeSecrets: Output? = null
private var includeVolumeData: Output? = null
private var selectedApplications: Output? = null
private var selectedNamespaces: Output? = null
/**
* @param value If True, include all namespaced resources.
*/
@JvmName("rljfpgpkjsybsixw")
public suspend fun allNamespaces(`value`: Output) {
this.allNamespaces = value
}
/**
* @param value This defines a customer managed encryption key that will be used to encrypt the "config"
* portion (the Kubernetes resources) of Backups created via this plan.
* Structure is documented below.
*/
@JvmName("jrpxmjetxkruiter")
public suspend fun encryptionKey(`value`: Output) {
this.encryptionKey = value
}
/**
* @param value This flag specifies whether Kubernetes Secret resources should be included
* when they fall into the scope of Backups.
*/
@JvmName("ufrokiurnlbaewrv")
public suspend fun includeSecrets(`value`: Output) {
this.includeSecrets = value
}
/**
* @param value This flag specifies whether volume data should be backed up when PVCs are
* included in the scope of a Backup.
*/
@JvmName("sagppcpmqlqxrmqp")
public suspend fun includeVolumeData(`value`: Output) {
this.includeVolumeData = value
}
/**
* @param value A list of namespaced Kubernetes Resources.
* Structure is documented below.
*/
@JvmName("lxumoelplbhwyxeh")
public suspend fun selectedApplications(`value`: Output) {
this.selectedApplications = value
}
/**
* @param value If set, include just the resources in the listed namespaces.
* Structure is documented below.
*/
@JvmName("sqeumhqbsagcgscl")
public suspend fun selectedNamespaces(`value`: Output) {
this.selectedNamespaces = value
}
/**
* @param value If True, include all namespaced resources.
*/
@JvmName("mvctryqclttqnlsk")
public suspend fun allNamespaces(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allNamespaces = mapped
}
/**
* @param value This defines a customer managed encryption key that will be used to encrypt the "config"
* portion (the Kubernetes resources) of Backups created via this plan.
* Structure is documented below.
*/
@JvmName("banntjupfhyfdqqq")
public suspend fun encryptionKey(`value`: BackupPlanBackupConfigEncryptionKeyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionKey = mapped
}
/**
* @param argument This defines a customer managed encryption key that will be used to encrypt the "config"
* portion (the Kubernetes resources) of Backups created via this plan.
* Structure is documented below.
*/
@JvmName("ajmssojvgfajrguk")
public suspend fun encryptionKey(argument: suspend BackupPlanBackupConfigEncryptionKeyArgsBuilder.() -> Unit) {
val toBeMapped = BackupPlanBackupConfigEncryptionKeyArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.encryptionKey = mapped
}
/**
* @param value This flag specifies whether Kubernetes Secret resources should be included
* when they fall into the scope of Backups.
*/
@JvmName("dpwwfciyijxccejj")
public suspend fun includeSecrets(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includeSecrets = mapped
}
/**
* @param value This flag specifies whether volume data should be backed up when PVCs are
* included in the scope of a Backup.
*/
@JvmName("oeyktynwsstfxuwx")
public suspend fun includeVolumeData(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includeVolumeData = mapped
}
/**
* @param value A list of namespaced Kubernetes Resources.
* Structure is documented below.
*/
@JvmName("klaojsvforskveom")
public suspend fun selectedApplications(`value`: BackupPlanBackupConfigSelectedApplicationsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.selectedApplications = mapped
}
/**
* @param argument A list of namespaced Kubernetes Resources.
* Structure is documented below.
*/
@JvmName("jypxxlubnqghdnka")
public suspend fun selectedApplications(argument: suspend BackupPlanBackupConfigSelectedApplicationsArgsBuilder.() -> Unit) {
val toBeMapped = BackupPlanBackupConfigSelectedApplicationsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.selectedApplications = mapped
}
/**
* @param value If set, include just the resources in the listed namespaces.
* Structure is documented below.
*/
@JvmName("xcwckorqepbctryn")
public suspend fun selectedNamespaces(`value`: BackupPlanBackupConfigSelectedNamespacesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.selectedNamespaces = mapped
}
/**
* @param argument If set, include just the resources in the listed namespaces.
* Structure is documented below.
*/
@JvmName("sfegqcwtjnktikgq")
public suspend fun selectedNamespaces(argument: suspend BackupPlanBackupConfigSelectedNamespacesArgsBuilder.() -> Unit) {
val toBeMapped = BackupPlanBackupConfigSelectedNamespacesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.selectedNamespaces = mapped
}
internal fun build(): BackupPlanBackupConfigArgs = BackupPlanBackupConfigArgs(
allNamespaces = allNamespaces,
encryptionKey = encryptionKey,
includeSecrets = includeSecrets,
includeVolumeData = includeVolumeData,
selectedApplications = selectedApplications,
selectedNamespaces = selectedNamespaces,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy