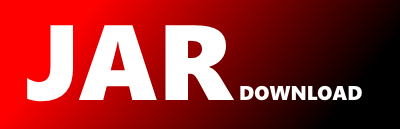
com.pulumi.gcp.gkebackup.kotlin.inputs.BackupPlanBackupScheduleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.gkebackup.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.gkebackup.inputs.BackupPlanBackupScheduleArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property cronSchedule A standard cron string that defines a repeating schedule for
* creating Backups via this BackupPlan.
* This is mutually exclusive with the rpoConfig field since at most one
* schedule can be defined for a BackupPlan.
* If this is defined, then backupRetainDays must also be defined.
* @property paused This flag denotes whether automatic Backup creation is paused for this BackupPlan.
* @property rpoConfig Defines the RPO schedule configuration for this BackupPlan. This is mutually
* exclusive with the cronSchedule field since at most one schedule can be defined
* for a BackupPLan. If this is defined, then backupRetainDays must also be defined.
* Structure is documented below.
*/
public data class BackupPlanBackupScheduleArgs(
public val cronSchedule: Output? = null,
public val paused: Output? = null,
public val rpoConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.gkebackup.inputs.BackupPlanBackupScheduleArgs =
com.pulumi.gcp.gkebackup.inputs.BackupPlanBackupScheduleArgs.builder()
.cronSchedule(cronSchedule?.applyValue({ args0 -> args0 }))
.paused(paused?.applyValue({ args0 -> args0 }))
.rpoConfig(rpoConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [BackupPlanBackupScheduleArgs].
*/
@PulumiTagMarker
public class BackupPlanBackupScheduleArgsBuilder internal constructor() {
private var cronSchedule: Output? = null
private var paused: Output? = null
private var rpoConfig: Output? = null
/**
* @param value A standard cron string that defines a repeating schedule for
* creating Backups via this BackupPlan.
* This is mutually exclusive with the rpoConfig field since at most one
* schedule can be defined for a BackupPlan.
* If this is defined, then backupRetainDays must also be defined.
*/
@JvmName("wradrbcpiydntotq")
public suspend fun cronSchedule(`value`: Output) {
this.cronSchedule = value
}
/**
* @param value This flag denotes whether automatic Backup creation is paused for this BackupPlan.
*/
@JvmName("gimergkvtbrtglln")
public suspend fun paused(`value`: Output) {
this.paused = value
}
/**
* @param value Defines the RPO schedule configuration for this BackupPlan. This is mutually
* exclusive with the cronSchedule field since at most one schedule can be defined
* for a BackupPLan. If this is defined, then backupRetainDays must also be defined.
* Structure is documented below.
*/
@JvmName("yiiyndtlltcvlxad")
public suspend fun rpoConfig(`value`: Output) {
this.rpoConfig = value
}
/**
* @param value A standard cron string that defines a repeating schedule for
* creating Backups via this BackupPlan.
* This is mutually exclusive with the rpoConfig field since at most one
* schedule can be defined for a BackupPlan.
* If this is defined, then backupRetainDays must also be defined.
*/
@JvmName("iygtjpyyqmsfkelt")
public suspend fun cronSchedule(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cronSchedule = mapped
}
/**
* @param value This flag denotes whether automatic Backup creation is paused for this BackupPlan.
*/
@JvmName("ldcwfbetigfpcruf")
public suspend fun paused(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.paused = mapped
}
/**
* @param value Defines the RPO schedule configuration for this BackupPlan. This is mutually
* exclusive with the cronSchedule field since at most one schedule can be defined
* for a BackupPLan. If this is defined, then backupRetainDays must also be defined.
* Structure is documented below.
*/
@JvmName("iywtubbhmjwgtyht")
public suspend fun rpoConfig(`value`: BackupPlanBackupScheduleRpoConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rpoConfig = mapped
}
/**
* @param argument Defines the RPO schedule configuration for this BackupPlan. This is mutually
* exclusive with the cronSchedule field since at most one schedule can be defined
* for a BackupPLan. If this is defined, then backupRetainDays must also be defined.
* Structure is documented below.
*/
@JvmName("kigwjcllvupogthq")
public suspend fun rpoConfig(argument: suspend BackupPlanBackupScheduleRpoConfigArgsBuilder.() -> Unit) {
val toBeMapped = BackupPlanBackupScheduleRpoConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.rpoConfig = mapped
}
internal fun build(): BackupPlanBackupScheduleArgs = BackupPlanBackupScheduleArgs(
cronSchedule = cronSchedule,
paused = paused,
rpoConfig = rpoConfig,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy