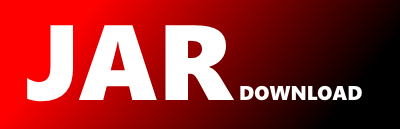
com.pulumi.gcp.gkebackup.kotlin.inputs.BackupPlanBackupScheduleRpoConfigExclusionWindowArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.gkebackup.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.gkebackup.inputs.BackupPlanBackupScheduleRpoConfigExclusionWindowArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property daily The exclusion window occurs every day if set to "True".
* Specifying this field to "False" is an error.
* Only one of singleOccurrenceDate, daily and daysOfWeek may be set.
* @property daysOfWeek The exclusion window occurs on these days of each week in UTC.
* Only one of singleOccurrenceDate, daily and daysOfWeek may be set.
* Structure is documented below.
* @property duration Specifies duration of the window in seconds with up to nine fractional digits,
* terminated by 's'. Example: "3.5s". Restrictions for duration based on the
* recurrence type to allow some time for backup to happen:
* - single_occurrence_date: no restriction
* - daily window: duration < 24 hours
* - weekly window:
* - days of week includes all seven days of a week: duration < 24 hours
* - all other weekly window: duration < 168 hours (i.e., 24 * 7 hours)
* @property singleOccurrenceDate No recurrence. The exclusion window occurs only once and on this date in UTC.
* Only one of singleOccurrenceDate, daily and daysOfWeek may be set.
* Structure is documented below.
* @property startTime Specifies the start time of the window using time of the day in UTC.
* Structure is documented below.
*/
public data class BackupPlanBackupScheduleRpoConfigExclusionWindowArgs(
public val daily: Output? = null,
public val daysOfWeek: Output? =
null,
public val duration: Output,
public val singleOccurrenceDate: Output? = null,
public val startTime: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.gkebackup.inputs.BackupPlanBackupScheduleRpoConfigExclusionWindowArgs =
com.pulumi.gcp.gkebackup.inputs.BackupPlanBackupScheduleRpoConfigExclusionWindowArgs.builder()
.daily(daily?.applyValue({ args0 -> args0 }))
.daysOfWeek(daysOfWeek?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.duration(duration.applyValue({ args0 -> args0 }))
.singleOccurrenceDate(
singleOccurrenceDate?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.startTime(startTime.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [BackupPlanBackupScheduleRpoConfigExclusionWindowArgs].
*/
@PulumiTagMarker
public class BackupPlanBackupScheduleRpoConfigExclusionWindowArgsBuilder internal constructor() {
private var daily: Output? = null
private var daysOfWeek: Output? =
null
private var duration: Output? = null
private var singleOccurrenceDate:
Output? = null
private var startTime: Output? =
null
/**
* @param value The exclusion window occurs every day if set to "True".
* Specifying this field to "False" is an error.
* Only one of singleOccurrenceDate, daily and daysOfWeek may be set.
*/
@JvmName("qfisahwtjtcsdvdq")
public suspend fun daily(`value`: Output) {
this.daily = value
}
/**
* @param value The exclusion window occurs on these days of each week in UTC.
* Only one of singleOccurrenceDate, daily and daysOfWeek may be set.
* Structure is documented below.
*/
@JvmName("hmjrhrauedvbdsev")
public suspend fun daysOfWeek(`value`: Output) {
this.daysOfWeek = value
}
/**
* @param value Specifies duration of the window in seconds with up to nine fractional digits,
* terminated by 's'. Example: "3.5s". Restrictions for duration based on the
* recurrence type to allow some time for backup to happen:
* - single_occurrence_date: no restriction
* - daily window: duration < 24 hours
* - weekly window:
* - days of week includes all seven days of a week: duration < 24 hours
* - all other weekly window: duration < 168 hours (i.e., 24 * 7 hours)
*/
@JvmName("ofjempexcdcikqjy")
public suspend fun duration(`value`: Output) {
this.duration = value
}
/**
* @param value No recurrence. The exclusion window occurs only once and on this date in UTC.
* Only one of singleOccurrenceDate, daily and daysOfWeek may be set.
* Structure is documented below.
*/
@JvmName("ufukieanfeeipimv")
public suspend fun singleOccurrenceDate(`value`: Output) {
this.singleOccurrenceDate = value
}
/**
* @param value Specifies the start time of the window using time of the day in UTC.
* Structure is documented below.
*/
@JvmName("vamnidrowiqihhnh")
public suspend fun startTime(`value`: Output) {
this.startTime = value
}
/**
* @param value The exclusion window occurs every day if set to "True".
* Specifying this field to "False" is an error.
* Only one of singleOccurrenceDate, daily and daysOfWeek may be set.
*/
@JvmName("qwqmdfoxgywjdhax")
public suspend fun daily(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.daily = mapped
}
/**
* @param value The exclusion window occurs on these days of each week in UTC.
* Only one of singleOccurrenceDate, daily and daysOfWeek may be set.
* Structure is documented below.
*/
@JvmName("roqsaonbwqsqlksn")
public suspend fun daysOfWeek(`value`: BackupPlanBackupScheduleRpoConfigExclusionWindowDaysOfWeekArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.daysOfWeek = mapped
}
/**
* @param argument The exclusion window occurs on these days of each week in UTC.
* Only one of singleOccurrenceDate, daily and daysOfWeek may be set.
* Structure is documented below.
*/
@JvmName("jtlnuggicpyiykxk")
public suspend fun daysOfWeek(argument: suspend BackupPlanBackupScheduleRpoConfigExclusionWindowDaysOfWeekArgsBuilder.() -> Unit) {
val toBeMapped =
BackupPlanBackupScheduleRpoConfigExclusionWindowDaysOfWeekArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.daysOfWeek = mapped
}
/**
* @param value Specifies duration of the window in seconds with up to nine fractional digits,
* terminated by 's'. Example: "3.5s". Restrictions for duration based on the
* recurrence type to allow some time for backup to happen:
* - single_occurrence_date: no restriction
* - daily window: duration < 24 hours
* - weekly window:
* - days of week includes all seven days of a week: duration < 24 hours
* - all other weekly window: duration < 168 hours (i.e., 24 * 7 hours)
*/
@JvmName("ecuvgdcordcvvyxn")
public suspend fun duration(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.duration = mapped
}
/**
* @param value No recurrence. The exclusion window occurs only once and on this date in UTC.
* Only one of singleOccurrenceDate, daily and daysOfWeek may be set.
* Structure is documented below.
*/
@JvmName("shblqmyyojswxvcp")
public suspend fun singleOccurrenceDate(`value`: BackupPlanBackupScheduleRpoConfigExclusionWindowSingleOccurrenceDateArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.singleOccurrenceDate = mapped
}
/**
* @param argument No recurrence. The exclusion window occurs only once and on this date in UTC.
* Only one of singleOccurrenceDate, daily and daysOfWeek may be set.
* Structure is documented below.
*/
@JvmName("nttuxiowlcthlasr")
public suspend fun singleOccurrenceDate(argument: suspend BackupPlanBackupScheduleRpoConfigExclusionWindowSingleOccurrenceDateArgsBuilder.() -> Unit) {
val toBeMapped =
BackupPlanBackupScheduleRpoConfigExclusionWindowSingleOccurrenceDateArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.singleOccurrenceDate = mapped
}
/**
* @param value Specifies the start time of the window using time of the day in UTC.
* Structure is documented below.
*/
@JvmName("qeqioqyqtifdkopu")
public suspend fun startTime(`value`: BackupPlanBackupScheduleRpoConfigExclusionWindowStartTimeArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.startTime = mapped
}
/**
* @param argument Specifies the start time of the window using time of the day in UTC.
* Structure is documented below.
*/
@JvmName("bvspbtxbreddvmyq")
public suspend fun startTime(argument: suspend BackupPlanBackupScheduleRpoConfigExclusionWindowStartTimeArgsBuilder.() -> Unit) {
val toBeMapped =
BackupPlanBackupScheduleRpoConfigExclusionWindowStartTimeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.startTime = mapped
}
internal fun build(): BackupPlanBackupScheduleRpoConfigExclusionWindowArgs =
BackupPlanBackupScheduleRpoConfigExclusionWindowArgs(
daily = daily,
daysOfWeek = daysOfWeek,
duration = duration ?: throw PulumiNullFieldException("duration"),
singleOccurrenceDate = singleOccurrenceDate,
startTime = startTime ?: throw PulumiNullFieldException("startTime"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy