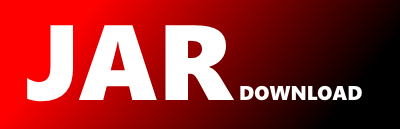
com.pulumi.gcp.gkebackup.kotlin.inputs.BackupPlanRetentionPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.gkebackup.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.gkebackup.inputs.BackupPlanRetentionPolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property backupDeleteLockDays Minimum age for a Backup created via this BackupPlan (in days).
* Must be an integer value between 0-90 (inclusive).
* A Backup created under this BackupPlan will not be deletable
* until it reaches Backup's (create time + backup_delete_lock_days).
* Updating this field of a BackupPlan does not affect existing Backups.
* Backups created after a successful update will inherit this new value.
* @property backupRetainDays The default maximum age of a Backup created via this BackupPlan.
* This field MUST be an integer value >= 0 and <= 365. If specified,
* a Backup created under this BackupPlan will be automatically deleted
* after its age reaches (createTime + backupRetainDays).
* If not specified, Backups created under this BackupPlan will NOT be
* subject to automatic deletion. Updating this field does NOT affect
* existing Backups under it. Backups created AFTER a successful update
* will automatically pick up the new value.
* NOTE: backupRetainDays must be >= backupDeleteLockDays.
* If cronSchedule is defined, then this must be <= 360 * the creation interval.
* If rpo_config is defined, then this must be
* <= 360 * targetRpoMinutes/(1440minutes/day)
* @property locked This flag denotes whether the retention policy of this BackupPlan is locked.
* If set to True, no further update is allowed on this policy, including
* the locked field itself.
*/
public data class BackupPlanRetentionPolicyArgs(
public val backupDeleteLockDays: Output? = null,
public val backupRetainDays: Output? = null,
public val locked: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.gkebackup.inputs.BackupPlanRetentionPolicyArgs =
com.pulumi.gcp.gkebackup.inputs.BackupPlanRetentionPolicyArgs.builder()
.backupDeleteLockDays(backupDeleteLockDays?.applyValue({ args0 -> args0 }))
.backupRetainDays(backupRetainDays?.applyValue({ args0 -> args0 }))
.locked(locked?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BackupPlanRetentionPolicyArgs].
*/
@PulumiTagMarker
public class BackupPlanRetentionPolicyArgsBuilder internal constructor() {
private var backupDeleteLockDays: Output? = null
private var backupRetainDays: Output? = null
private var locked: Output? = null
/**
* @param value Minimum age for a Backup created via this BackupPlan (in days).
* Must be an integer value between 0-90 (inclusive).
* A Backup created under this BackupPlan will not be deletable
* until it reaches Backup's (create time + backup_delete_lock_days).
* Updating this field of a BackupPlan does not affect existing Backups.
* Backups created after a successful update will inherit this new value.
*/
@JvmName("ckekkoblbdhrduyo")
public suspend fun backupDeleteLockDays(`value`: Output) {
this.backupDeleteLockDays = value
}
/**
* @param value The default maximum age of a Backup created via this BackupPlan.
* This field MUST be an integer value >= 0 and <= 365. If specified,
* a Backup created under this BackupPlan will be automatically deleted
* after its age reaches (createTime + backupRetainDays).
* If not specified, Backups created under this BackupPlan will NOT be
* subject to automatic deletion. Updating this field does NOT affect
* existing Backups under it. Backups created AFTER a successful update
* will automatically pick up the new value.
* NOTE: backupRetainDays must be >= backupDeleteLockDays.
* If cronSchedule is defined, then this must be <= 360 * the creation interval.
* If rpo_config is defined, then this must be
* <= 360 * targetRpoMinutes/(1440minutes/day)
*/
@JvmName("ylhucqwamuncptdn")
public suspend fun backupRetainDays(`value`: Output) {
this.backupRetainDays = value
}
/**
* @param value This flag denotes whether the retention policy of this BackupPlan is locked.
* If set to True, no further update is allowed on this policy, including
* the locked field itself.
*/
@JvmName("kjnslwjddyakekpk")
public suspend fun locked(`value`: Output) {
this.locked = value
}
/**
* @param value Minimum age for a Backup created via this BackupPlan (in days).
* Must be an integer value between 0-90 (inclusive).
* A Backup created under this BackupPlan will not be deletable
* until it reaches Backup's (create time + backup_delete_lock_days).
* Updating this field of a BackupPlan does not affect existing Backups.
* Backups created after a successful update will inherit this new value.
*/
@JvmName("bgyugivxwgvdodgn")
public suspend fun backupDeleteLockDays(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backupDeleteLockDays = mapped
}
/**
* @param value The default maximum age of a Backup created via this BackupPlan.
* This field MUST be an integer value >= 0 and <= 365. If specified,
* a Backup created under this BackupPlan will be automatically deleted
* after its age reaches (createTime + backupRetainDays).
* If not specified, Backups created under this BackupPlan will NOT be
* subject to automatic deletion. Updating this field does NOT affect
* existing Backups under it. Backups created AFTER a successful update
* will automatically pick up the new value.
* NOTE: backupRetainDays must be >= backupDeleteLockDays.
* If cronSchedule is defined, then this must be <= 360 * the creation interval.
* If rpo_config is defined, then this must be
* <= 360 * targetRpoMinutes/(1440minutes/day)
*/
@JvmName("ncdwfqmrqtxndlxs")
public suspend fun backupRetainDays(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backupRetainDays = mapped
}
/**
* @param value This flag denotes whether the retention policy of this BackupPlan is locked.
* If set to True, no further update is allowed on this policy, including
* the locked field itself.
*/
@JvmName("uugfqevtohrmqjnn")
public suspend fun locked(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.locked = mapped
}
internal fun build(): BackupPlanRetentionPolicyArgs = BackupPlanRetentionPolicyArgs(
backupDeleteLockDays = backupDeleteLockDays,
backupRetainDays = backupRetainDays,
locked = locked,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy