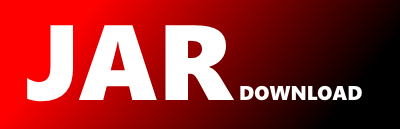
com.pulumi.gcp.gkehub.kotlin.ScopeRbacRoleBindingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.gkehub.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.gkehub.ScopeRbacRoleBindingArgs.builder
import com.pulumi.gcp.gkehub.kotlin.inputs.ScopeRbacRoleBindingRoleArgs
import com.pulumi.gcp.gkehub.kotlin.inputs.ScopeRbacRoleBindingRoleArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* RBACRoleBinding represents a rbacrolebinding across the Fleet.
* To get more information about ScopeRBACRoleBinding, see:
* * [API documentation](https://cloud.google.com/anthos/fleet-management/docs/reference/rest/v1/projects.locations.scopes.rbacrolebindings)
* * How-to Guides
* * [Registering a Cluster](https://cloud.google.com/anthos/multicluster-management/connect/registering-a-cluster#register_cluster)
* ## Example Usage
* ### Gkehub Scope Rbac Role Binding Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const scope = new gcp.gkehub.Scope("scope", {scopeId: "tf-test-scope_75223"});
* const scopeRbacRoleBinding = new gcp.gkehub.ScopeRbacRoleBinding("scope_rbac_role_binding", {
* scopeRbacRoleBindingId: "tf-test-scope-rbac-role-binding_41819",
* scopeId: scope.scopeId,
* user: "[email protected]",
* role: {
* predefinedRole: "ADMIN",
* },
* labels: {
* key: "value",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* scope = gcp.gkehub.Scope("scope", scope_id="tf-test-scope_75223")
* scope_rbac_role_binding = gcp.gkehub.ScopeRbacRoleBinding("scope_rbac_role_binding",
* scope_rbac_role_binding_id="tf-test-scope-rbac-role-binding_41819",
* scope_id=scope.scope_id,
* user="[email protected]",
* role=gcp.gkehub.ScopeRbacRoleBindingRoleArgs(
* predefined_role="ADMIN",
* ),
* labels={
* "key": "value",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var scope = new Gcp.GkeHub.Scope("scope", new()
* {
* ScopeId = "tf-test-scope_75223",
* });
* var scopeRbacRoleBinding = new Gcp.GkeHub.ScopeRbacRoleBinding("scope_rbac_role_binding", new()
* {
* ScopeRbacRoleBindingId = "tf-test-scope-rbac-role-binding_41819",
* ScopeId = scope.ScopeId,
* User = "[email protected]",
* Role = new Gcp.GkeHub.Inputs.ScopeRbacRoleBindingRoleArgs
* {
* PredefinedRole = "ADMIN",
* },
* Labels =
* {
* { "key", "value" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/gkehub"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* scope, err := gkehub.NewScope(ctx, "scope", &gkehub.ScopeArgs{
* ScopeId: pulumi.String("tf-test-scope_75223"),
* })
* if err != nil {
* return err
* }
* _, err = gkehub.NewScopeRbacRoleBinding(ctx, "scope_rbac_role_binding", &gkehub.ScopeRbacRoleBindingArgs{
* ScopeRbacRoleBindingId: pulumi.String("tf-test-scope-rbac-role-binding_41819"),
* ScopeId: scope.ScopeId,
* User: pulumi.String("[email protected]"),
* Role: &gkehub.ScopeRbacRoleBindingRoleArgs{
* PredefinedRole: pulumi.String("ADMIN"),
* },
* Labels: pulumi.StringMap{
* "key": pulumi.String("value"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.gkehub.Scope;
* import com.pulumi.gcp.gkehub.ScopeArgs;
* import com.pulumi.gcp.gkehub.ScopeRbacRoleBinding;
* import com.pulumi.gcp.gkehub.ScopeRbacRoleBindingArgs;
* import com.pulumi.gcp.gkehub.inputs.ScopeRbacRoleBindingRoleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var scope = new Scope("scope", ScopeArgs.builder()
* .scopeId("tf-test-scope_75223")
* .build());
* var scopeRbacRoleBinding = new ScopeRbacRoleBinding("scopeRbacRoleBinding", ScopeRbacRoleBindingArgs.builder()
* .scopeRbacRoleBindingId("tf-test-scope-rbac-role-binding_41819")
* .scopeId(scope.scopeId())
* .user("[email protected]")
* .role(ScopeRbacRoleBindingRoleArgs.builder()
* .predefinedRole("ADMIN")
* .build())
* .labels(Map.of("key", "value"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* scope:
* type: gcp:gkehub:Scope
* properties:
* scopeId: tf-test-scope_75223
* scopeRbacRoleBinding:
* type: gcp:gkehub:ScopeRbacRoleBinding
* name: scope_rbac_role_binding
* properties:
* scopeRbacRoleBindingId: tf-test-scope-rbac-role-binding_41819
* scopeId: ${scope.scopeId}
* user: [email protected]
* role:
* predefinedRole: ADMIN
* labels:
* key: value
* ```
*
* ## Import
* ScopeRBACRoleBinding can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/global/scopes/{{scope_id}}/rbacrolebindings/{{scope_rbac_role_binding_id}}`
* * `{{project}}/{{scope_id}}/{{scope_rbac_role_binding_id}}`
* * `{{scope_id}}/{{scope_rbac_role_binding_id}}`
* When using the `pulumi import` command, ScopeRBACRoleBinding can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:gkehub/scopeRbacRoleBinding:ScopeRbacRoleBinding default projects/{{project}}/locations/global/scopes/{{scope_id}}/rbacrolebindings/{{scope_rbac_role_binding_id}}
* ```
* ```sh
* $ pulumi import gcp:gkehub/scopeRbacRoleBinding:ScopeRbacRoleBinding default {{project}}/{{scope_id}}/{{scope_rbac_role_binding_id}}
* ```
* ```sh
* $ pulumi import gcp:gkehub/scopeRbacRoleBinding:ScopeRbacRoleBinding default {{scope_id}}/{{scope_rbac_role_binding_id}}
* ```
* @property group Principal that is be authorized in the cluster (at least of one the oneof is required). Updating one will unset the
* other automatically. group is the group, as seen by the kubernetes cluster.
* @property labels Labels for this ScopeRBACRoleBinding. **Note**: This field is non-authoritative, and will only manage the labels present
* in your configuration. Please refer to the field 'effective_labels' for all of the labels present on the resource.
* @property project
* @property role Role to bind to the principal.
* Structure is documented below.
* @property scopeId Id of the scope
* @property scopeRbacRoleBindingId The client-provided identifier of the RBAC Role Binding.
* @property user Principal that is be authorized in the cluster (at least of one the oneof is required). Updating one will unset the
* other automatically. user is the name of the user as seen by the kubernetes cluster, example "alice" or
* "[email protected]"
*/
public data class ScopeRbacRoleBindingArgs(
public val group: Output? = null,
public val labels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy