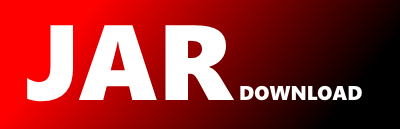
com.pulumi.gcp.gkehub.kotlin.inputs.FeatureFleetDefaultMemberConfigConfigmanagementConfigSyncOciArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.gkehub.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.gkehub.inputs.FeatureFleetDefaultMemberConfigConfigmanagementConfigSyncOciArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property gcpServiceAccountEmail The Google Cloud Service Account Email used for auth when secretType is gcpServiceAccount
* @property policyDir The absolute path of the directory that contains the local resources. Default: the root directory of the image
* @property secretType Type of secret configured for access to the Git repo
* @property syncRepo The OCI image repository URL for the package to sync from
* @property syncWaitSecs Period in seconds between consecutive syncs. Default: 15
* @property version (Optional, Deprecated)
* Version of ACM installed
* > **Warning:** The `configmanagement.config_sync.oci.version` field is deprecated and will be removed in a future major release. Please use `configmanagement.version` field to specify the version of ACM installed instead.
*/
public data class FeatureFleetDefaultMemberConfigConfigmanagementConfigSyncOciArgs(
public val gcpServiceAccountEmail: Output? = null,
public val policyDir: Output? = null,
public val secretType: Output,
public val syncRepo: Output? = null,
public val syncWaitSecs: Output? = null,
@Deprecated(
message = """
The `configmanagement.config_sync.oci.version` field is deprecated and will be removed in a future
major release. Please use `configmanagement.version` field to specify the version of ACM
installed instead.
""",
)
public val version: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.gkehub.inputs.FeatureFleetDefaultMemberConfigConfigmanagementConfigSyncOciArgs =
com.pulumi.gcp.gkehub.inputs.FeatureFleetDefaultMemberConfigConfigmanagementConfigSyncOciArgs.builder()
.gcpServiceAccountEmail(gcpServiceAccountEmail?.applyValue({ args0 -> args0 }))
.policyDir(policyDir?.applyValue({ args0 -> args0 }))
.secretType(secretType.applyValue({ args0 -> args0 }))
.syncRepo(syncRepo?.applyValue({ args0 -> args0 }))
.syncWaitSecs(syncWaitSecs?.applyValue({ args0 -> args0 }))
.version(version?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FeatureFleetDefaultMemberConfigConfigmanagementConfigSyncOciArgs].
*/
@PulumiTagMarker
public class FeatureFleetDefaultMemberConfigConfigmanagementConfigSyncOciArgsBuilder internal constructor() {
private var gcpServiceAccountEmail: Output? = null
private var policyDir: Output? = null
private var secretType: Output? = null
private var syncRepo: Output? = null
private var syncWaitSecs: Output? = null
private var version: Output? = null
/**
* @param value The Google Cloud Service Account Email used for auth when secretType is gcpServiceAccount
*/
@JvmName("itdlvrvxmvqrefbh")
public suspend fun gcpServiceAccountEmail(`value`: Output) {
this.gcpServiceAccountEmail = value
}
/**
* @param value The absolute path of the directory that contains the local resources. Default: the root directory of the image
*/
@JvmName("xfttnuhpcrpkskgg")
public suspend fun policyDir(`value`: Output) {
this.policyDir = value
}
/**
* @param value Type of secret configured for access to the Git repo
*/
@JvmName("vsoapiytdsxpnjwe")
public suspend fun secretType(`value`: Output) {
this.secretType = value
}
/**
* @param value The OCI image repository URL for the package to sync from
*/
@JvmName("cacwjqxqvroyloum")
public suspend fun syncRepo(`value`: Output) {
this.syncRepo = value
}
/**
* @param value Period in seconds between consecutive syncs. Default: 15
*/
@JvmName("fwnnwirtltofvlir")
public suspend fun syncWaitSecs(`value`: Output) {
this.syncWaitSecs = value
}
/**
* @param value (Optional, Deprecated)
* Version of ACM installed
* > **Warning:** The `configmanagement.config_sync.oci.version` field is deprecated and will be removed in a future major release. Please use `configmanagement.version` field to specify the version of ACM installed instead.
*/
@Deprecated(
message = """
The `configmanagement.config_sync.oci.version` field is deprecated and will be removed in a future
major release. Please use `configmanagement.version` field to specify the version of ACM
installed instead.
""",
)
@JvmName("fmckyhtahlxpwdpi")
public suspend fun version(`value`: Output) {
this.version = value
}
/**
* @param value The Google Cloud Service Account Email used for auth when secretType is gcpServiceAccount
*/
@JvmName("bjkyhgeaydnlfdxc")
public suspend fun gcpServiceAccountEmail(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gcpServiceAccountEmail = mapped
}
/**
* @param value The absolute path of the directory that contains the local resources. Default: the root directory of the image
*/
@JvmName("gdcolrcbkbkxbkhv")
public suspend fun policyDir(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.policyDir = mapped
}
/**
* @param value Type of secret configured for access to the Git repo
*/
@JvmName("kgsljpjwsmrfgevg")
public suspend fun secretType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.secretType = mapped
}
/**
* @param value The OCI image repository URL for the package to sync from
*/
@JvmName("qrgwvypxejuonafj")
public suspend fun syncRepo(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.syncRepo = mapped
}
/**
* @param value Period in seconds between consecutive syncs. Default: 15
*/
@JvmName("bhjjwhiwcdarglhj")
public suspend fun syncWaitSecs(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.syncWaitSecs = mapped
}
/**
* @param value (Optional, Deprecated)
* Version of ACM installed
* > **Warning:** The `configmanagement.config_sync.oci.version` field is deprecated and will be removed in a future major release. Please use `configmanagement.version` field to specify the version of ACM installed instead.
*/
@Deprecated(
message = """
The `configmanagement.config_sync.oci.version` field is deprecated and will be removed in a future
major release. Please use `configmanagement.version` field to specify the version of ACM
installed instead.
""",
)
@JvmName("lfxdhpmoblwkkuaa")
public suspend fun version(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.version = mapped
}
internal fun build(): FeatureFleetDefaultMemberConfigConfigmanagementConfigSyncOciArgs =
FeatureFleetDefaultMemberConfigConfigmanagementConfigSyncOciArgs(
gcpServiceAccountEmail = gcpServiceAccountEmail,
policyDir = policyDir,
secretType = secretType ?: throw PulumiNullFieldException("secretType"),
syncRepo = syncRepo,
syncWaitSecs = syncWaitSecs,
version = version,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy