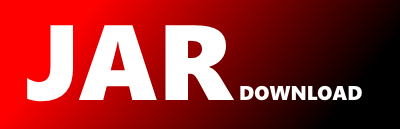
com.pulumi.gcp.gkehub.kotlin.inputs.FeatureMembershipConfigmanagementConfigSyncArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.gkehub.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.gkehub.inputs.FeatureMembershipConfigmanagementConfigSyncArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property git (Optional) Structure is documented below.
* @property metricsGcpServiceAccountEmail The Email of the Google Cloud Service Account (GSA) used for exporting Config Sync metrics to Cloud Monitoring. The GSA should have the Monitoring Metric Writer(roles/monitoring.metricWriter) IAM role. The Kubernetes ServiceAccount `default` in the namespace `config-management-monitoring` should be bound to the GSA.
* @property oci (Optional) Supported from ACM versions 1.12.0 onwards. Structure is documented below.
* Use either `git` or `oci` config option.
* @property preventDrift Supported from ACM versions 1.10.0 onwards. Set to true to enable the Config Sync admission webhook to prevent drifts. If set to "false", disables the Config Sync admission webhook and does not prevent drifts.
* @property sourceFormat Specifies whether the Config Sync Repo is in "hierarchical" or "unstructured" mode.
*/
public data class FeatureMembershipConfigmanagementConfigSyncArgs(
public val git: Output? = null,
public val metricsGcpServiceAccountEmail: Output? = null,
public val oci: Output? = null,
public val preventDrift: Output? = null,
public val sourceFormat: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.gkehub.inputs.FeatureMembershipConfigmanagementConfigSyncArgs =
com.pulumi.gcp.gkehub.inputs.FeatureMembershipConfigmanagementConfigSyncArgs.builder()
.git(git?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.metricsGcpServiceAccountEmail(metricsGcpServiceAccountEmail?.applyValue({ args0 -> args0 }))
.oci(oci?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.preventDrift(preventDrift?.applyValue({ args0 -> args0 }))
.sourceFormat(sourceFormat?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FeatureMembershipConfigmanagementConfigSyncArgs].
*/
@PulumiTagMarker
public class FeatureMembershipConfigmanagementConfigSyncArgsBuilder internal constructor() {
private var git: Output? = null
private var metricsGcpServiceAccountEmail: Output? = null
private var oci: Output? = null
private var preventDrift: Output? = null
private var sourceFormat: Output? = null
/**
* @param value (Optional) Structure is documented below.
*/
@JvmName("mivlmyrbpugouijk")
public suspend fun git(`value`: Output) {
this.git = value
}
/**
* @param value The Email of the Google Cloud Service Account (GSA) used for exporting Config Sync metrics to Cloud Monitoring. The GSA should have the Monitoring Metric Writer(roles/monitoring.metricWriter) IAM role. The Kubernetes ServiceAccount `default` in the namespace `config-management-monitoring` should be bound to the GSA.
*/
@JvmName("rirdlhcjqvnnilkf")
public suspend fun metricsGcpServiceAccountEmail(`value`: Output) {
this.metricsGcpServiceAccountEmail = value
}
/**
* @param value (Optional) Supported from ACM versions 1.12.0 onwards. Structure is documented below.
* Use either `git` or `oci` config option.
*/
@JvmName("itxlcylvcoyndsdy")
public suspend fun oci(`value`: Output) {
this.oci = value
}
/**
* @param value Supported from ACM versions 1.10.0 onwards. Set to true to enable the Config Sync admission webhook to prevent drifts. If set to "false", disables the Config Sync admission webhook and does not prevent drifts.
*/
@JvmName("raeutnbilovhoypm")
public suspend fun preventDrift(`value`: Output) {
this.preventDrift = value
}
/**
* @param value Specifies whether the Config Sync Repo is in "hierarchical" or "unstructured" mode.
*/
@JvmName("hlauiefapkwjlauu")
public suspend fun sourceFormat(`value`: Output) {
this.sourceFormat = value
}
/**
* @param value (Optional) Structure is documented below.
*/
@JvmName("powtdqnyggbcdqvl")
public suspend fun git(`value`: FeatureMembershipConfigmanagementConfigSyncGitArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.git = mapped
}
/**
* @param argument (Optional) Structure is documented below.
*/
@JvmName("fxnbkhaoyagufwbn")
public suspend fun git(argument: suspend FeatureMembershipConfigmanagementConfigSyncGitArgsBuilder.() -> Unit) {
val toBeMapped = FeatureMembershipConfigmanagementConfigSyncGitArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.git = mapped
}
/**
* @param value The Email of the Google Cloud Service Account (GSA) used for exporting Config Sync metrics to Cloud Monitoring. The GSA should have the Monitoring Metric Writer(roles/monitoring.metricWriter) IAM role. The Kubernetes ServiceAccount `default` in the namespace `config-management-monitoring` should be bound to the GSA.
*/
@JvmName("csldfmgppfgqukug")
public suspend fun metricsGcpServiceAccountEmail(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metricsGcpServiceAccountEmail = mapped
}
/**
* @param value (Optional) Supported from ACM versions 1.12.0 onwards. Structure is documented below.
* Use either `git` or `oci` config option.
*/
@JvmName("dsgtxhnjvdwyahmo")
public suspend fun oci(`value`: FeatureMembershipConfigmanagementConfigSyncOciArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.oci = mapped
}
/**
* @param argument (Optional) Supported from ACM versions 1.12.0 onwards. Structure is documented below.
* Use either `git` or `oci` config option.
*/
@JvmName("tjkooqvkuaktuefe")
public suspend fun oci(argument: suspend FeatureMembershipConfigmanagementConfigSyncOciArgsBuilder.() -> Unit) {
val toBeMapped = FeatureMembershipConfigmanagementConfigSyncOciArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.oci = mapped
}
/**
* @param value Supported from ACM versions 1.10.0 onwards. Set to true to enable the Config Sync admission webhook to prevent drifts. If set to "false", disables the Config Sync admission webhook and does not prevent drifts.
*/
@JvmName("scnvclevnhgxonbx")
public suspend fun preventDrift(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preventDrift = mapped
}
/**
* @param value Specifies whether the Config Sync Repo is in "hierarchical" or "unstructured" mode.
*/
@JvmName("hejavclmwnqnkhaq")
public suspend fun sourceFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceFormat = mapped
}
internal fun build(): FeatureMembershipConfigmanagementConfigSyncArgs =
FeatureMembershipConfigmanagementConfigSyncArgs(
git = git,
metricsGcpServiceAccountEmail = metricsGcpServiceAccountEmail,
oci = oci,
preventDrift = preventDrift,
sourceFormat = sourceFormat,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy