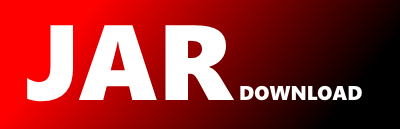
com.pulumi.gcp.gkeonprem.kotlin.inputs.BareMetalClusterLoadBalancerBgpLbConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.gkeonprem.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.gkeonprem.inputs.BareMetalClusterLoadBalancerBgpLbConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property addressPools AddressPools is a list of non-overlapping IP pools used by load balancer
* typed services. All addresses must be routable to load balancer nodes.
* IngressVIP must be included in the pools.
* Structure is documented below.
* @property asn BGP autonomous system number (ASN) of the cluster.
* This field can be updated after cluster creation.
* @property bgpPeerConfigs The list of BGP peers that the cluster will connect to.
* At least one peer must be configured for each control plane node.
* Control plane nodes will connect to these peers to advertise the control
* plane VIP. The Services load balancer also uses these peers by default.
* This field can be updated after cluster creation.
* Structure is documented below.
* @property loadBalancerNodePoolConfig Specifies the node pool running data plane load balancing. L2 connectivity
* is required among nodes in this pool. If missing, the control plane node
* pool is used for data plane load balancing.
* Structure is documented below.
*/
public data class BareMetalClusterLoadBalancerBgpLbConfigArgs(
public val addressPools: Output>,
public val asn: Output,
public val bgpPeerConfigs: Output>,
public val loadBalancerNodePoolConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.gkeonprem.inputs.BareMetalClusterLoadBalancerBgpLbConfigArgs = com.pulumi.gcp.gkeonprem.inputs.BareMetalClusterLoadBalancerBgpLbConfigArgs.builder()
.addressPools(
addressPools.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.asn(asn.applyValue({ args0 -> args0 }))
.bgpPeerConfigs(
bgpPeerConfigs.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.loadBalancerNodePoolConfig(
loadBalancerNodePoolConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [BareMetalClusterLoadBalancerBgpLbConfigArgs].
*/
@PulumiTagMarker
public class BareMetalClusterLoadBalancerBgpLbConfigArgsBuilder internal constructor() {
private var addressPools: Output>? =
null
private var asn: Output? = null
private var bgpPeerConfigs:
Output>? = null
private var loadBalancerNodePoolConfig:
Output? = null
/**
* @param value AddressPools is a list of non-overlapping IP pools used by load balancer
* typed services. All addresses must be routable to load balancer nodes.
* IngressVIP must be included in the pools.
* Structure is documented below.
*/
@JvmName("xeltpqgrbmhcnrkk")
public suspend fun addressPools(`value`: Output>) {
this.addressPools = value
}
@JvmName("tkjijnrlmwxxkobv")
public suspend fun addressPools(vararg values: Output) {
this.addressPools = Output.all(values.asList())
}
/**
* @param values AddressPools is a list of non-overlapping IP pools used by load balancer
* typed services. All addresses must be routable to load balancer nodes.
* IngressVIP must be included in the pools.
* Structure is documented below.
*/
@JvmName("dibdpakhijwdtphr")
public suspend fun addressPools(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy