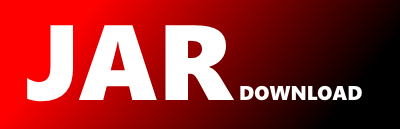
com.pulumi.gcp.gkeonprem.kotlin.inputs.VMwareClusterVcenterArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.gkeonprem.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.gkeonprem.inputs.VMwareClusterVcenterArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property address (Output)
* The vCenter IP address.
* @property caCertData Contains the vCenter CA certificate public key for SSL verification.
* @property cluster The name of the vCenter cluster for the user cluster.
* @property datacenter The name of the vCenter datacenter for the user cluster.
* @property datastore The name of the vCenter datastore for the user cluster.
* @property folder The name of the vCenter folder for the user cluster.
* @property resourcePool The name of the vCenter resource pool for the user cluster.
* @property storagePolicyName The name of the vCenter storage policy for the user cluster.
*/
public data class VMwareClusterVcenterArgs(
public val address: Output? = null,
public val caCertData: Output? = null,
public val cluster: Output? = null,
public val datacenter: Output? = null,
public val datastore: Output? = null,
public val folder: Output? = null,
public val resourcePool: Output? = null,
public val storagePolicyName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.gkeonprem.inputs.VMwareClusterVcenterArgs =
com.pulumi.gcp.gkeonprem.inputs.VMwareClusterVcenterArgs.builder()
.address(address?.applyValue({ args0 -> args0 }))
.caCertData(caCertData?.applyValue({ args0 -> args0 }))
.cluster(cluster?.applyValue({ args0 -> args0 }))
.datacenter(datacenter?.applyValue({ args0 -> args0 }))
.datastore(datastore?.applyValue({ args0 -> args0 }))
.folder(folder?.applyValue({ args0 -> args0 }))
.resourcePool(resourcePool?.applyValue({ args0 -> args0 }))
.storagePolicyName(storagePolicyName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VMwareClusterVcenterArgs].
*/
@PulumiTagMarker
public class VMwareClusterVcenterArgsBuilder internal constructor() {
private var address: Output? = null
private var caCertData: Output? = null
private var cluster: Output? = null
private var datacenter: Output? = null
private var datastore: Output? = null
private var folder: Output? = null
private var resourcePool: Output? = null
private var storagePolicyName: Output? = null
/**
* @param value (Output)
* The vCenter IP address.
*/
@JvmName("hmlquhygmfgkhotk")
public suspend fun address(`value`: Output) {
this.address = value
}
/**
* @param value Contains the vCenter CA certificate public key for SSL verification.
*/
@JvmName("gogofdlyjmemjsoy")
public suspend fun caCertData(`value`: Output) {
this.caCertData = value
}
/**
* @param value The name of the vCenter cluster for the user cluster.
*/
@JvmName("rehnugvwugscysil")
public suspend fun cluster(`value`: Output) {
this.cluster = value
}
/**
* @param value The name of the vCenter datacenter for the user cluster.
*/
@JvmName("hnkibeksindtnhdo")
public suspend fun datacenter(`value`: Output) {
this.datacenter = value
}
/**
* @param value The name of the vCenter datastore for the user cluster.
*/
@JvmName("xvndfldqasilbjgo")
public suspend fun datastore(`value`: Output) {
this.datastore = value
}
/**
* @param value The name of the vCenter folder for the user cluster.
*/
@JvmName("ljiqaxuupfbwnxft")
public suspend fun folder(`value`: Output) {
this.folder = value
}
/**
* @param value The name of the vCenter resource pool for the user cluster.
*/
@JvmName("rmutmghodsrfklme")
public suspend fun resourcePool(`value`: Output) {
this.resourcePool = value
}
/**
* @param value The name of the vCenter storage policy for the user cluster.
*/
@JvmName("ekipcyegqpfqeuxt")
public suspend fun storagePolicyName(`value`: Output) {
this.storagePolicyName = value
}
/**
* @param value (Output)
* The vCenter IP address.
*/
@JvmName("ogjcbsfetxljjdgx")
public suspend fun address(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.address = mapped
}
/**
* @param value Contains the vCenter CA certificate public key for SSL verification.
*/
@JvmName("dfdxejvbfvkdwqvy")
public suspend fun caCertData(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.caCertData = mapped
}
/**
* @param value The name of the vCenter cluster for the user cluster.
*/
@JvmName("sfbqgpqswqttqbnq")
public suspend fun cluster(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cluster = mapped
}
/**
* @param value The name of the vCenter datacenter for the user cluster.
*/
@JvmName("jujlfmsoxkqcwxru")
public suspend fun datacenter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.datacenter = mapped
}
/**
* @param value The name of the vCenter datastore for the user cluster.
*/
@JvmName("kvgrtirbljdjbpbv")
public suspend fun datastore(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.datastore = mapped
}
/**
* @param value The name of the vCenter folder for the user cluster.
*/
@JvmName("trhtaiboqlghddmw")
public suspend fun folder(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.folder = mapped
}
/**
* @param value The name of the vCenter resource pool for the user cluster.
*/
@JvmName("xquqdkeivxgvjnko")
public suspend fun resourcePool(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourcePool = mapped
}
/**
* @param value The name of the vCenter storage policy for the user cluster.
*/
@JvmName("jrneyoorjiorjtve")
public suspend fun storagePolicyName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storagePolicyName = mapped
}
internal fun build(): VMwareClusterVcenterArgs = VMwareClusterVcenterArgs(
address = address,
caCertData = caCertData,
cluster = cluster,
datacenter = datacenter,
datastore = datastore,
folder = folder,
resourcePool = resourcePool,
storagePolicyName = storagePolicyName,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy