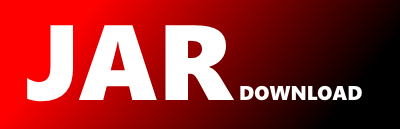
com.pulumi.gcp.integrationconnectors.kotlin.inputs.ConnectionAuthConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.integrationconnectors.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.integrationconnectors.inputs.ConnectionAuthConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property additionalVariables List containing additional auth configs.
* Structure is documented below.
* @property authKey The type of authentication configured.
* @property authType authType of the Connection
* Possible values are: `USER_PASSWORD`.
* @property oauth2AuthCodeFlow Parameters to support Oauth 2.0 Auth Code Grant Authentication.
* Structure is documented below.
* @property oauth2ClientCredentials OAuth3 Client Credentials for Authentication.
* Structure is documented below.
* @property oauth2JwtBearer OAuth2 JWT Bearer for Authentication.
* Structure is documented below.
* @property sshPublicKey SSH Public Key for Authentication.
* Structure is documented below.
* @property userPassword User password for Authentication.
* Structure is documented below.
*/
public data class ConnectionAuthConfigArgs(
public val additionalVariables: Output>? = null,
public val authKey: Output? = null,
public val authType: Output,
public val oauth2AuthCodeFlow: Output? = null,
public val oauth2ClientCredentials: Output? =
null,
public val oauth2JwtBearer: Output? = null,
public val sshPublicKey: Output? = null,
public val userPassword: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.integrationconnectors.inputs.ConnectionAuthConfigArgs =
com.pulumi.gcp.integrationconnectors.inputs.ConnectionAuthConfigArgs.builder()
.additionalVariables(
additionalVariables?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.authKey(authKey?.applyValue({ args0 -> args0 }))
.authType(authType.applyValue({ args0 -> args0 }))
.oauth2AuthCodeFlow(
oauth2AuthCodeFlow?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.oauth2ClientCredentials(
oauth2ClientCredentials?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.oauth2JwtBearer(oauth2JwtBearer?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.sshPublicKey(sshPublicKey?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.userPassword(userPassword?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ConnectionAuthConfigArgs].
*/
@PulumiTagMarker
public class ConnectionAuthConfigArgsBuilder internal constructor() {
private var additionalVariables: Output>? = null
private var authKey: Output? = null
private var authType: Output? = null
private var oauth2AuthCodeFlow: Output? = null
private var oauth2ClientCredentials: Output? =
null
private var oauth2JwtBearer: Output? = null
private var sshPublicKey: Output? = null
private var userPassword: Output? = null
/**
* @param value List containing additional auth configs.
* Structure is documented below.
*/
@JvmName("aslicjyrgdotmffn")
public suspend fun additionalVariables(`value`: Output>) {
this.additionalVariables = value
}
@JvmName("shmhmobtwddqgpyg")
public suspend fun additionalVariables(vararg values: Output) {
this.additionalVariables = Output.all(values.asList())
}
/**
* @param values List containing additional auth configs.
* Structure is documented below.
*/
@JvmName("svrgtnqtuhmoufqg")
public suspend fun additionalVariables(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy