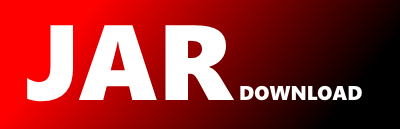
com.pulumi.gcp.integrationconnectors.kotlin.inputs.ConnectionEventingConfigAuthConfigAdditionalVariableArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.integrationconnectors.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.integrationconnectors.inputs.ConnectionEventingConfigAuthConfigAdditionalVariableArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property booleanValue Boolean Value of configVariable.
* @property encryptionKeyValue Encription key value of configVariable.
* Structure is documented below.
* @property integerValue Integer Value of configVariable.
* @property key Key for the configVariable
* @property secretValue Secret value of configVariable
* Structure is documented below.
* @property stringValue String Value of configVariabley.
*/
public data class ConnectionEventingConfigAuthConfigAdditionalVariableArgs(
public val booleanValue: Output? = null,
public val encryptionKeyValue: Output? = null,
public val integerValue: Output? = null,
public val key: Output,
public val secretValue: Output? = null,
public val stringValue: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.integrationconnectors.inputs.ConnectionEventingConfigAuthConfigAdditionalVariableArgs =
com.pulumi.gcp.integrationconnectors.inputs.ConnectionEventingConfigAuthConfigAdditionalVariableArgs.builder()
.booleanValue(booleanValue?.applyValue({ args0 -> args0 }))
.encryptionKeyValue(
encryptionKeyValue?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.integerValue(integerValue?.applyValue({ args0 -> args0 }))
.key(key.applyValue({ args0 -> args0 }))
.secretValue(secretValue?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.stringValue(stringValue?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConnectionEventingConfigAuthConfigAdditionalVariableArgs].
*/
@PulumiTagMarker
public class ConnectionEventingConfigAuthConfigAdditionalVariableArgsBuilder internal constructor() {
private var booleanValue: Output? = null
private var encryptionKeyValue:
Output? = null
private var integerValue: Output? = null
private var key: Output? = null
private var secretValue:
Output? = null
private var stringValue: Output? = null
/**
* @param value Boolean Value of configVariable.
*/
@JvmName("lyqqhfoyriivtwmm")
public suspend fun booleanValue(`value`: Output) {
this.booleanValue = value
}
/**
* @param value Encription key value of configVariable.
* Structure is documented below.
*/
@JvmName("bavlsrmfsvuqsjqs")
public suspend fun encryptionKeyValue(`value`: Output) {
this.encryptionKeyValue = value
}
/**
* @param value Integer Value of configVariable.
*/
@JvmName("ykkgpuojktytyyvw")
public suspend fun integerValue(`value`: Output) {
this.integerValue = value
}
/**
* @param value Key for the configVariable
*/
@JvmName("gjjwiyevbeggtgqc")
public suspend fun key(`value`: Output) {
this.key = value
}
/**
* @param value Secret value of configVariable
* Structure is documented below.
*/
@JvmName("sabkxhhvkowbgrpc")
public suspend fun secretValue(`value`: Output) {
this.secretValue = value
}
/**
* @param value String Value of configVariabley.
*/
@JvmName("vjlbbdqwhqggehdn")
public suspend fun stringValue(`value`: Output) {
this.stringValue = value
}
/**
* @param value Boolean Value of configVariable.
*/
@JvmName("ytqsdonayxmqvahf")
public suspend fun booleanValue(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.booleanValue = mapped
}
/**
* @param value Encription key value of configVariable.
* Structure is documented below.
*/
@JvmName("ajwiavubwoctxami")
public suspend fun encryptionKeyValue(`value`: ConnectionEventingConfigAuthConfigAdditionalVariableEncryptionKeyValueArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionKeyValue = mapped
}
/**
* @param argument Encription key value of configVariable.
* Structure is documented below.
*/
@JvmName("gyxpfqjbihxsveyo")
public suspend fun encryptionKeyValue(argument: suspend ConnectionEventingConfigAuthConfigAdditionalVariableEncryptionKeyValueArgsBuilder.() -> Unit) {
val toBeMapped =
ConnectionEventingConfigAuthConfigAdditionalVariableEncryptionKeyValueArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.encryptionKeyValue = mapped
}
/**
* @param value Integer Value of configVariable.
*/
@JvmName("wakufqcitwsoblmf")
public suspend fun integerValue(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.integerValue = mapped
}
/**
* @param value Key for the configVariable
*/
@JvmName("msbtdjephyhdchuw")
public suspend fun key(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.key = mapped
}
/**
* @param value Secret value of configVariable
* Structure is documented below.
*/
@JvmName("icitxjxamlgkwgjx")
public suspend fun secretValue(`value`: ConnectionEventingConfigAuthConfigAdditionalVariableSecretValueArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secretValue = mapped
}
/**
* @param argument Secret value of configVariable
* Structure is documented below.
*/
@JvmName("nulxyrrxcglaobrd")
public suspend fun secretValue(argument: suspend ConnectionEventingConfigAuthConfigAdditionalVariableSecretValueArgsBuilder.() -> Unit) {
val toBeMapped =
ConnectionEventingConfigAuthConfigAdditionalVariableSecretValueArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.secretValue = mapped
}
/**
* @param value String Value of configVariabley.
*/
@JvmName("ksjpmcnosovyqgij")
public suspend fun stringValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.stringValue = mapped
}
internal fun build(): ConnectionEventingConfigAuthConfigAdditionalVariableArgs =
ConnectionEventingConfigAuthConfigAdditionalVariableArgs(
booleanValue = booleanValue,
encryptionKeyValue = encryptionKeyValue,
integerValue = integerValue,
key = key ?: throw PulumiNullFieldException("key"),
secretValue = secretValue,
stringValue = stringValue,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy