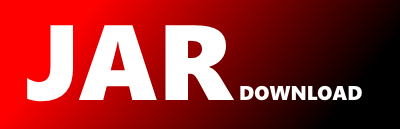
com.pulumi.gcp.kms.kotlin.CryptoKeyVersionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.kms.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.kms.CryptoKeyVersionArgs.builder
import com.pulumi.gcp.kms.kotlin.inputs.CryptoKeyVersionExternalProtectionLevelOptionsArgs
import com.pulumi.gcp.kms.kotlin.inputs.CryptoKeyVersionExternalProtectionLevelOptionsArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* A `CryptoKeyVersion` represents an individual cryptographic key, and the associated key material.
* Destroying a cryptoKeyVersion will not delete the resource from the project.
* To get more information about CryptoKeyVersion, see:
* * [API documentation](https://cloud.google.com/kms/docs/reference/rest/v1/projects.locations.keyRings.cryptoKeys.cryptoKeyVersions)
* * How-to Guides
* * [Creating a key Version](https://cloud.google.com/kms/docs/reference/rest/v1/projects.locations.keyRings.cryptoKeys.cryptoKeyVersions/create)
* ## Example Usage
* ### Kms Crypto Key Version Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const keyring = new gcp.kms.KeyRing("keyring", {
* name: "keyring-example",
* location: "global",
* });
* const cryptokey = new gcp.kms.CryptoKey("cryptokey", {
* name: "crypto-key-example",
* keyRing: keyring.id,
* rotationPeriod: "7776000s",
* });
* const example_key = new gcp.kms.CryptoKeyVersion("example-key", {cryptoKey: cryptokey.id});
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* keyring = gcp.kms.KeyRing("keyring",
* name="keyring-example",
* location="global")
* cryptokey = gcp.kms.CryptoKey("cryptokey",
* name="crypto-key-example",
* key_ring=keyring.id,
* rotation_period="7776000s")
* example_key = gcp.kms.CryptoKeyVersion("example-key", crypto_key=cryptokey.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var keyring = new Gcp.Kms.KeyRing("keyring", new()
* {
* Name = "keyring-example",
* Location = "global",
* });
* var cryptokey = new Gcp.Kms.CryptoKey("cryptokey", new()
* {
* Name = "crypto-key-example",
* KeyRing = keyring.Id,
* RotationPeriod = "7776000s",
* });
* var example_key = new Gcp.Kms.CryptoKeyVersion("example-key", new()
* {
* CryptoKey = cryptokey.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/kms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* keyring, err := kms.NewKeyRing(ctx, "keyring", &kms.KeyRingArgs{
* Name: pulumi.String("keyring-example"),
* Location: pulumi.String("global"),
* })
* if err != nil {
* return err
* }
* cryptokey, err := kms.NewCryptoKey(ctx, "cryptokey", &kms.CryptoKeyArgs{
* Name: pulumi.String("crypto-key-example"),
* KeyRing: keyring.ID(),
* RotationPeriod: pulumi.String("7776000s"),
* })
* if err != nil {
* return err
* }
* _, err = kms.NewCryptoKeyVersion(ctx, "example-key", &kms.CryptoKeyVersionArgs{
* CryptoKey: cryptokey.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.kms.KeyRing;
* import com.pulumi.gcp.kms.KeyRingArgs;
* import com.pulumi.gcp.kms.CryptoKey;
* import com.pulumi.gcp.kms.CryptoKeyArgs;
* import com.pulumi.gcp.kms.CryptoKeyVersion;
* import com.pulumi.gcp.kms.CryptoKeyVersionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var keyring = new KeyRing("keyring", KeyRingArgs.builder()
* .name("keyring-example")
* .location("global")
* .build());
* var cryptokey = new CryptoKey("cryptokey", CryptoKeyArgs.builder()
* .name("crypto-key-example")
* .keyRing(keyring.id())
* .rotationPeriod("7776000s")
* .build());
* var example_key = new CryptoKeyVersion("example-key", CryptoKeyVersionArgs.builder()
* .cryptoKey(cryptokey.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* keyring:
* type: gcp:kms:KeyRing
* properties:
* name: keyring-example
* location: global
* cryptokey:
* type: gcp:kms:CryptoKey
* properties:
* name: crypto-key-example
* keyRing: ${keyring.id}
* rotationPeriod: 7776000s
* example-key:
* type: gcp:kms:CryptoKeyVersion
* properties:
* cryptoKey: ${cryptokey.id}
* ```
*
* ## Import
* CryptoKeyVersion can be imported using any of these accepted formats:
* * `{{name}}`
* When using the `pulumi import` command, CryptoKeyVersion can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:kms/cryptoKeyVersion:CryptoKeyVersion default {{name}}
* ```
* @property cryptoKey The name of the cryptoKey associated with the CryptoKeyVersions.
* Format: `'projects/{{project}}/locations/{{location}}/keyRings/{{keyring}}/cryptoKeys/{{cryptoKey}}'`
* - - -
* @property externalProtectionLevelOptions ExternalProtectionLevelOptions stores a group of additional fields for configuring a CryptoKeyVersion that are specific to the EXTERNAL protection level and EXTERNAL_VPC protection levels.
* Structure is documented below.
* @property state The current state of the CryptoKeyVersion.
* Possible values are: `PENDING_GENERATION`, `ENABLED`, `DISABLED`, `DESTROYED`, `DESTROY_SCHEDULED`, `PENDING_IMPORT`, `IMPORT_FAILED`.
*/
public data class CryptoKeyVersionArgs(
public val cryptoKey: Output? = null,
public val externalProtectionLevelOptions: Output? = null,
public val state: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.kms.CryptoKeyVersionArgs =
com.pulumi.gcp.kms.CryptoKeyVersionArgs.builder()
.cryptoKey(cryptoKey?.applyValue({ args0 -> args0 }))
.externalProtectionLevelOptions(
externalProtectionLevelOptions?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.state(state?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CryptoKeyVersionArgs].
*/
@PulumiTagMarker
public class CryptoKeyVersionArgsBuilder internal constructor() {
private var cryptoKey: Output? = null
private var externalProtectionLevelOptions:
Output? = null
private var state: Output? = null
/**
* @param value The name of the cryptoKey associated with the CryptoKeyVersions.
* Format: `'projects/{{project}}/locations/{{location}}/keyRings/{{keyring}}/cryptoKeys/{{cryptoKey}}'`
* - - -
*/
@JvmName("pltogjdqwvvjbdcr")
public suspend fun cryptoKey(`value`: Output) {
this.cryptoKey = value
}
/**
* @param value ExternalProtectionLevelOptions stores a group of additional fields for configuring a CryptoKeyVersion that are specific to the EXTERNAL protection level and EXTERNAL_VPC protection levels.
* Structure is documented below.
*/
@JvmName("cgpiiloqlhroyfqr")
public suspend fun externalProtectionLevelOptions(`value`: Output) {
this.externalProtectionLevelOptions = value
}
/**
* @param value The current state of the CryptoKeyVersion.
* Possible values are: `PENDING_GENERATION`, `ENABLED`, `DISABLED`, `DESTROYED`, `DESTROY_SCHEDULED`, `PENDING_IMPORT`, `IMPORT_FAILED`.
*/
@JvmName("shdhpbfylwcfijid")
public suspend fun state(`value`: Output) {
this.state = value
}
/**
* @param value The name of the cryptoKey associated with the CryptoKeyVersions.
* Format: `'projects/{{project}}/locations/{{location}}/keyRings/{{keyring}}/cryptoKeys/{{cryptoKey}}'`
* - - -
*/
@JvmName("lykklgerbiultosh")
public suspend fun cryptoKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cryptoKey = mapped
}
/**
* @param value ExternalProtectionLevelOptions stores a group of additional fields for configuring a CryptoKeyVersion that are specific to the EXTERNAL protection level and EXTERNAL_VPC protection levels.
* Structure is documented below.
*/
@JvmName("gctharyngwhchkcr")
public suspend fun externalProtectionLevelOptions(`value`: CryptoKeyVersionExternalProtectionLevelOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.externalProtectionLevelOptions = mapped
}
/**
* @param argument ExternalProtectionLevelOptions stores a group of additional fields for configuring a CryptoKeyVersion that are specific to the EXTERNAL protection level and EXTERNAL_VPC protection levels.
* Structure is documented below.
*/
@JvmName("sukpyrfsbhyjxrdq")
public suspend fun externalProtectionLevelOptions(argument: suspend CryptoKeyVersionExternalProtectionLevelOptionsArgsBuilder.() -> Unit) {
val toBeMapped = CryptoKeyVersionExternalProtectionLevelOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.externalProtectionLevelOptions = mapped
}
/**
* @param value The current state of the CryptoKeyVersion.
* Possible values are: `PENDING_GENERATION`, `ENABLED`, `DISABLED`, `DESTROYED`, `DESTROY_SCHEDULED`, `PENDING_IMPORT`, `IMPORT_FAILED`.
*/
@JvmName("lrnkfgwusjjxwlrn")
public suspend fun state(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.state = mapped
}
internal fun build(): CryptoKeyVersionArgs = CryptoKeyVersionArgs(
cryptoKey = cryptoKey,
externalProtectionLevelOptions = externalProtectionLevelOptions,
state = state,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy