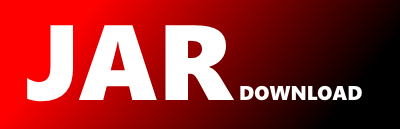
com.pulumi.gcp.kms.kotlin.EkmConnectionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.kms.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.kms.EkmConnectionArgs.builder
import com.pulumi.gcp.kms.kotlin.inputs.EkmConnectionServiceResolverArgs
import com.pulumi.gcp.kms.kotlin.inputs.EkmConnectionServiceResolverArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* `Ekm Connections` are used to control the connection settings for an `EXTERNAL_VPC` CryptoKey.
* It is used to connect customer's external key manager to Google Cloud EKM.
* > **Note:** Ekm Connections cannot be deleted from Google Cloud Platform.
* To get more information about EkmConnection, see:
* * [API documentation](https://cloud.google.com/kms/docs/reference/rest/v1/projects.locations.ekmConnections)
* * How-to Guides
* * [Creating a Ekm Connection](https://cloud.google.com/kms/docs/create-ekm-connection)
* ## Example Usage
* ### Kms Ekm Connection Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const example_ekmconnection = new gcp.kms.EkmConnection("example-ekmconnection", {
* name: "ekmconnection_example",
* location: "us-central1",
* keyManagementMode: "MANUAL",
* serviceResolvers: [{
* serviceDirectoryService: "projects/project_id/locations/us-central1/namespaces/namespace_name/services/service_name",
* hostname: "example-ekm.goog",
* serverCertificates: [{
* rawDer: "==HAwIBCCAr6gAwIBAgIUWR+EV4lqiV7Ql12VY==",
* }],
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* example_ekmconnection = gcp.kms.EkmConnection("example-ekmconnection",
* name="ekmconnection_example",
* location="us-central1",
* key_management_mode="MANUAL",
* service_resolvers=[gcp.kms.EkmConnectionServiceResolverArgs(
* service_directory_service="projects/project_id/locations/us-central1/namespaces/namespace_name/services/service_name",
* hostname="example-ekm.goog",
* server_certificates=[gcp.kms.EkmConnectionServiceResolverServerCertificateArgs(
* raw_der="==HAwIBCCAr6gAwIBAgIUWR+EV4lqiV7Ql12VY==",
* )],
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var example_ekmconnection = new Gcp.Kms.EkmConnection("example-ekmconnection", new()
* {
* Name = "ekmconnection_example",
* Location = "us-central1",
* KeyManagementMode = "MANUAL",
* ServiceResolvers = new[]
* {
* new Gcp.Kms.Inputs.EkmConnectionServiceResolverArgs
* {
* ServiceDirectoryService = "projects/project_id/locations/us-central1/namespaces/namespace_name/services/service_name",
* Hostname = "example-ekm.goog",
* ServerCertificates = new[]
* {
* new Gcp.Kms.Inputs.EkmConnectionServiceResolverServerCertificateArgs
* {
* RawDer = "==HAwIBCCAr6gAwIBAgIUWR+EV4lqiV7Ql12VY==",
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/kms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kms.NewEkmConnection(ctx, "example-ekmconnection", &kms.EkmConnectionArgs{
* Name: pulumi.String("ekmconnection_example"),
* Location: pulumi.String("us-central1"),
* KeyManagementMode: pulumi.String("MANUAL"),
* ServiceResolvers: kms.EkmConnectionServiceResolverArray{
* &kms.EkmConnectionServiceResolverArgs{
* ServiceDirectoryService: pulumi.String("projects/project_id/locations/us-central1/namespaces/namespace_name/services/service_name"),
* Hostname: pulumi.String("example-ekm.goog"),
* ServerCertificates: kms.EkmConnectionServiceResolverServerCertificateArray{
* &kms.EkmConnectionServiceResolverServerCertificateArgs{
* RawDer: pulumi.String("==HAwIBCCAr6gAwIBAgIUWR+EV4lqiV7Ql12VY=="),
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.kms.EkmConnection;
* import com.pulumi.gcp.kms.EkmConnectionArgs;
* import com.pulumi.gcp.kms.inputs.EkmConnectionServiceResolverArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example_ekmconnection = new EkmConnection("example-ekmconnection", EkmConnectionArgs.builder()
* .name("ekmconnection_example")
* .location("us-central1")
* .keyManagementMode("MANUAL")
* .serviceResolvers(EkmConnectionServiceResolverArgs.builder()
* .serviceDirectoryService("projects/project_id/locations/us-central1/namespaces/namespace_name/services/service_name")
* .hostname("example-ekm.goog")
* .serverCertificates(EkmConnectionServiceResolverServerCertificateArgs.builder()
* .rawDer("==HAwIBCCAr6gAwIBAgIUWR+EV4lqiV7Ql12VY==")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example-ekmconnection:
* type: gcp:kms:EkmConnection
* properties:
* name: ekmconnection_example
* location: us-central1
* keyManagementMode: MANUAL
* serviceResolvers:
* - serviceDirectoryService: projects/project_id/locations/us-central1/namespaces/namespace_name/services/service_name
* hostname: example-ekm.goog
* serverCertificates:
* - rawDer: ==HAwIBCCAr6gAwIBAgIUWR+EV4lqiV7Ql12VY==
* ```
*
* ## Import
* EkmConnection can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/ekmConnections/{{name}}`
* * `{{project}}/{{location}}/{{name}}`
* * `{{location}}/{{name}}`
* When using the `pulumi import` command, EkmConnection can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:kms/ekmConnection:EkmConnection default projects/{{project}}/locations/{{location}}/ekmConnections/{{name}}
* ```
* ```sh
* $ pulumi import gcp:kms/ekmConnection:EkmConnection default {{project}}/{{location}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:kms/ekmConnection:EkmConnection default {{location}}/{{name}}
* ```
* @property cryptoSpacePath Optional. Identifies the EKM Crypto Space that this EkmConnection maps to. Note: This field is required if
* KeyManagementMode is CLOUD_KMS.
* @property etag Optional. Etag of the currently stored EkmConnection.
* @property keyManagementMode Optional. Describes who can perform control plane operations on the EKM. If unset, this defaults to MANUAL Default
* value: "MANUAL" Possible values: ["MANUAL", "CLOUD_KMS"]
* @property location The location for the EkmConnection.
* A full list of valid locations can be found by running `gcloud kms locations list`.
* @property name The resource name for the EkmConnection.
* @property project
* @property serviceResolvers A list of ServiceResolvers where the EKM can be reached. There should be one ServiceResolver per EKM replica. Currently, only a single ServiceResolver is supported
* Structure is documented below.
*/
public data class EkmConnectionArgs(
public val cryptoSpacePath: Output? = null,
public val etag: Output? = null,
public val keyManagementMode: Output? = null,
public val location: Output? = null,
public val name: Output? = null,
public val project: Output? = null,
public val serviceResolvers: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.kms.EkmConnectionArgs =
com.pulumi.gcp.kms.EkmConnectionArgs.builder()
.cryptoSpacePath(cryptoSpacePath?.applyValue({ args0 -> args0 }))
.etag(etag?.applyValue({ args0 -> args0 }))
.keyManagementMode(keyManagementMode?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.serviceResolvers(
serviceResolvers?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [EkmConnectionArgs].
*/
@PulumiTagMarker
public class EkmConnectionArgsBuilder internal constructor() {
private var cryptoSpacePath: Output? = null
private var etag: Output? = null
private var keyManagementMode: Output? = null
private var location: Output? = null
private var name: Output? = null
private var project: Output? = null
private var serviceResolvers: Output>? = null
/**
* @param value Optional. Identifies the EKM Crypto Space that this EkmConnection maps to. Note: This field is required if
* KeyManagementMode is CLOUD_KMS.
*/
@JvmName("cubwvfpkaiqvuifl")
public suspend fun cryptoSpacePath(`value`: Output) {
this.cryptoSpacePath = value
}
/**
* @param value Optional. Etag of the currently stored EkmConnection.
*/
@JvmName("ijdaexxtkdxxisgo")
public suspend fun etag(`value`: Output) {
this.etag = value
}
/**
* @param value Optional. Describes who can perform control plane operations on the EKM. If unset, this defaults to MANUAL Default
* value: "MANUAL" Possible values: ["MANUAL", "CLOUD_KMS"]
*/
@JvmName("xuuxiakggaifyqmr")
public suspend fun keyManagementMode(`value`: Output) {
this.keyManagementMode = value
}
/**
* @param value The location for the EkmConnection.
* A full list of valid locations can be found by running `gcloud kms locations list`.
*/
@JvmName("revpqccyydgdpoou")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value The resource name for the EkmConnection.
*/
@JvmName("dvtkkndcbceftewq")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value
*/
@JvmName("fajthpeasqtpbfkb")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value A list of ServiceResolvers where the EKM can be reached. There should be one ServiceResolver per EKM replica. Currently, only a single ServiceResolver is supported
* Structure is documented below.
*/
@JvmName("eyotgbbiqbxolcew")
public suspend fun serviceResolvers(`value`: Output>) {
this.serviceResolvers = value
}
@JvmName("tnsfdfmicitemhth")
public suspend fun serviceResolvers(vararg values: Output) {
this.serviceResolvers = Output.all(values.asList())
}
/**
* @param values A list of ServiceResolvers where the EKM can be reached. There should be one ServiceResolver per EKM replica. Currently, only a single ServiceResolver is supported
* Structure is documented below.
*/
@JvmName("hchfqvmmhjmgbfif")
public suspend fun serviceResolvers(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy