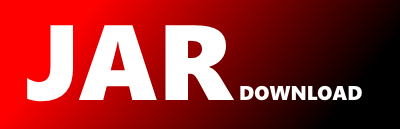
com.pulumi.gcp.kms.kotlin.KeyRingImportJobArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.kms.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.kms.KeyRingImportJobArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A `KeyRingImportJob` can be used to create `CryptoKeys` and `CryptoKeyVersions` using pre-existing
* key material, generated outside of Cloud KMS. A `KeyRingImportJob` expires 3 days after it is created.
* Once expired, Cloud KMS will no longer be able to import or unwrap any key material that
* was wrapped with the `KeyRingImportJob`'s public key.
* > **Note:** KeyRingImportJobs cannot be deleted from Google Cloud Platform.
* Destroying a provider-managed KeyRingImportJob will remove it from state but
* *will not delete the resource from the project.*
* To get more information about KeyRingImportJob, see:
* * [API documentation](https://cloud.google.com/kms/docs/reference/rest/v1/projects.locations.keyRings.importJobs)
* * How-to Guides
* * [Importing a key](https://cloud.google.com/kms/docs/importing-a-key)
* ## Example Usage
* ## Import
* KeyRingImportJob can be imported using any of these accepted formats:
* * `{{name}}`
* When using the `pulumi import` command, KeyRingImportJob can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:kms/keyRingImportJob:KeyRingImportJob default {{name}}
* ```
* @property importJobId It must be unique within a KeyRing and match the regular expression [a-zA-Z0-9_-]{1,63}
* - - -
* @property importMethod The wrapping method to be used for incoming key material.
* Possible values are: `RSA_OAEP_3072_SHA1_AES_256`, `RSA_OAEP_4096_SHA1_AES_256`.
* @property keyRing The KeyRing that this import job belongs to.
* Format: `'projects/{{project}}/locations/{{location}}/keyRings/{{keyRing}}'`.
* @property protectionLevel The protection level of the ImportJob. This must match the protectionLevel of the
* versionTemplate on the CryptoKey you attempt to import into.
* Possible values are: `SOFTWARE`, `HSM`, `EXTERNAL`.
*/
public data class KeyRingImportJobArgs(
public val importJobId: Output? = null,
public val importMethod: Output? = null,
public val keyRing: Output? = null,
public val protectionLevel: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.kms.KeyRingImportJobArgs =
com.pulumi.gcp.kms.KeyRingImportJobArgs.builder()
.importJobId(importJobId?.applyValue({ args0 -> args0 }))
.importMethod(importMethod?.applyValue({ args0 -> args0 }))
.keyRing(keyRing?.applyValue({ args0 -> args0 }))
.protectionLevel(protectionLevel?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [KeyRingImportJobArgs].
*/
@PulumiTagMarker
public class KeyRingImportJobArgsBuilder internal constructor() {
private var importJobId: Output? = null
private var importMethod: Output? = null
private var keyRing: Output? = null
private var protectionLevel: Output? = null
/**
* @param value It must be unique within a KeyRing and match the regular expression [a-zA-Z0-9_-]{1,63}
* - - -
*/
@JvmName("hstbmhiwyihhfapt")
public suspend fun importJobId(`value`: Output) {
this.importJobId = value
}
/**
* @param value The wrapping method to be used for incoming key material.
* Possible values are: `RSA_OAEP_3072_SHA1_AES_256`, `RSA_OAEP_4096_SHA1_AES_256`.
*/
@JvmName("lwdvhnulbktkygxd")
public suspend fun importMethod(`value`: Output) {
this.importMethod = value
}
/**
* @param value The KeyRing that this import job belongs to.
* Format: `'projects/{{project}}/locations/{{location}}/keyRings/{{keyRing}}'`.
*/
@JvmName("xrabbsxhwcnbgldy")
public suspend fun keyRing(`value`: Output) {
this.keyRing = value
}
/**
* @param value The protection level of the ImportJob. This must match the protectionLevel of the
* versionTemplate on the CryptoKey you attempt to import into.
* Possible values are: `SOFTWARE`, `HSM`, `EXTERNAL`.
*/
@JvmName("dqrplcdqyqropujw")
public suspend fun protectionLevel(`value`: Output) {
this.protectionLevel = value
}
/**
* @param value It must be unique within a KeyRing and match the regular expression [a-zA-Z0-9_-]{1,63}
* - - -
*/
@JvmName("dbcotkmjvmahsykq")
public suspend fun importJobId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.importJobId = mapped
}
/**
* @param value The wrapping method to be used for incoming key material.
* Possible values are: `RSA_OAEP_3072_SHA1_AES_256`, `RSA_OAEP_4096_SHA1_AES_256`.
*/
@JvmName("kkakkuuobclanrfw")
public suspend fun importMethod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.importMethod = mapped
}
/**
* @param value The KeyRing that this import job belongs to.
* Format: `'projects/{{project}}/locations/{{location}}/keyRings/{{keyRing}}'`.
*/
@JvmName("elflfxvadquqpiel")
public suspend fun keyRing(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keyRing = mapped
}
/**
* @param value The protection level of the ImportJob. This must match the protectionLevel of the
* versionTemplate on the CryptoKey you attempt to import into.
* Possible values are: `SOFTWARE`, `HSM`, `EXTERNAL`.
*/
@JvmName("qpvawesfmnpdggbf")
public suspend fun protectionLevel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.protectionLevel = mapped
}
internal fun build(): KeyRingImportJobArgs = KeyRingImportJobArgs(
importJobId = importJobId,
importMethod = importMethod,
keyRing = keyRing,
protectionLevel = protectionLevel,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy