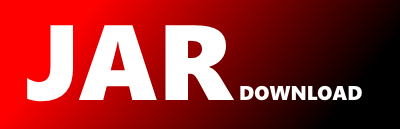
com.pulumi.gcp.kms.kotlin.inputs.EkmConnectionServiceResolverServerCertificateArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.kms.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.kms.inputs.EkmConnectionServiceResolverServerCertificateArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property issuer (Output)
* Output only. The issuer distinguished name in RFC 2253 format. Only present if parsed is true.
* @property notAfterTime (Output)
* Output only. The certificate is not valid after this time. Only present if parsed is true.
* A timestamp in RFC3339 UTC "Zulu" format, with nanosecond resolution and up to nine fractional digits. Examples: "2014-10-02T15:01:23Z" and "2014-10-02T15:01:23.045123456Z".
* @property notBeforeTime (Output)
* Output only. The certificate is not valid before this time. Only present if parsed is true.
* A timestamp in RFC3339 UTC "Zulu" format, with nanosecond resolution and up to nine fractional digits. Examples: "2014-10-02T15:01:23Z" and "2014-10-02T15:01:23.045123456Z".
* @property parsed (Output)
* Output only. True if the certificate was parsed successfully.
* @property rawDer Required. The raw certificate bytes in DER format. A base64-encoded string.
* @property serialNumber (Output)
* Output only. The certificate serial number as a hex string. Only present if parsed is true.
* @property sha256Fingerprint (Output)
* Output only. The SHA-256 certificate fingerprint as a hex string. Only present if parsed is true.
* @property subject (Output)
* Output only. The subject distinguished name in RFC 2253 format. Only present if parsed is true.
* @property subjectAlternativeDnsNames (Output)
* Output only. The subject Alternative DNS names. Only present if parsed is true.
* - - -
*/
public data class EkmConnectionServiceResolverServerCertificateArgs(
public val issuer: Output? = null,
public val notAfterTime: Output? = null,
public val notBeforeTime: Output? = null,
public val parsed: Output? = null,
public val rawDer: Output,
public val serialNumber: Output? = null,
public val sha256Fingerprint: Output? = null,
public val subject: Output? = null,
public val subjectAlternativeDnsNames: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.kms.inputs.EkmConnectionServiceResolverServerCertificateArgs = com.pulumi.gcp.kms.inputs.EkmConnectionServiceResolverServerCertificateArgs.builder()
.issuer(issuer?.applyValue({ args0 -> args0 }))
.notAfterTime(notAfterTime?.applyValue({ args0 -> args0 }))
.notBeforeTime(notBeforeTime?.applyValue({ args0 -> args0 }))
.parsed(parsed?.applyValue({ args0 -> args0 }))
.rawDer(rawDer.applyValue({ args0 -> args0 }))
.serialNumber(serialNumber?.applyValue({ args0 -> args0 }))
.sha256Fingerprint(sha256Fingerprint?.applyValue({ args0 -> args0 }))
.subject(subject?.applyValue({ args0 -> args0 }))
.subjectAlternativeDnsNames(
subjectAlternativeDnsNames?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
).build()
}
/**
* Builder for [EkmConnectionServiceResolverServerCertificateArgs].
*/
@PulumiTagMarker
public class EkmConnectionServiceResolverServerCertificateArgsBuilder internal constructor() {
private var issuer: Output? = null
private var notAfterTime: Output? = null
private var notBeforeTime: Output? = null
private var parsed: Output? = null
private var rawDer: Output? = null
private var serialNumber: Output? = null
private var sha256Fingerprint: Output? = null
private var subject: Output? = null
private var subjectAlternativeDnsNames: Output>? = null
/**
* @param value (Output)
* Output only. The issuer distinguished name in RFC 2253 format. Only present if parsed is true.
*/
@JvmName("wuhshrqrjujsihhm")
public suspend fun issuer(`value`: Output) {
this.issuer = value
}
/**
* @param value (Output)
* Output only. The certificate is not valid after this time. Only present if parsed is true.
* A timestamp in RFC3339 UTC "Zulu" format, with nanosecond resolution and up to nine fractional digits. Examples: "2014-10-02T15:01:23Z" and "2014-10-02T15:01:23.045123456Z".
*/
@JvmName("nbigmwrlebdokvuu")
public suspend fun notAfterTime(`value`: Output) {
this.notAfterTime = value
}
/**
* @param value (Output)
* Output only. The certificate is not valid before this time. Only present if parsed is true.
* A timestamp in RFC3339 UTC "Zulu" format, with nanosecond resolution and up to nine fractional digits. Examples: "2014-10-02T15:01:23Z" and "2014-10-02T15:01:23.045123456Z".
*/
@JvmName("ttodetwquaxfwbqi")
public suspend fun notBeforeTime(`value`: Output) {
this.notBeforeTime = value
}
/**
* @param value (Output)
* Output only. True if the certificate was parsed successfully.
*/
@JvmName("haonmmhflxarnasn")
public suspend fun parsed(`value`: Output) {
this.parsed = value
}
/**
* @param value Required. The raw certificate bytes in DER format. A base64-encoded string.
*/
@JvmName("mwxsqcpwhosfyyei")
public suspend fun rawDer(`value`: Output) {
this.rawDer = value
}
/**
* @param value (Output)
* Output only. The certificate serial number as a hex string. Only present if parsed is true.
*/
@JvmName("pwqbdtlocavibyjv")
public suspend fun serialNumber(`value`: Output) {
this.serialNumber = value
}
/**
* @param value (Output)
* Output only. The SHA-256 certificate fingerprint as a hex string. Only present if parsed is true.
*/
@JvmName("utoldmjoplrcmxmg")
public suspend fun sha256Fingerprint(`value`: Output) {
this.sha256Fingerprint = value
}
/**
* @param value (Output)
* Output only. The subject distinguished name in RFC 2253 format. Only present if parsed is true.
*/
@JvmName("tqhinnqkkelycwpp")
public suspend fun subject(`value`: Output) {
this.subject = value
}
/**
* @param value (Output)
* Output only. The subject Alternative DNS names. Only present if parsed is true.
* - - -
*/
@JvmName("xjjwwcmkiwtlqbom")
public suspend fun subjectAlternativeDnsNames(`value`: Output>) {
this.subjectAlternativeDnsNames = value
}
@JvmName("dwumtjeytksugsqb")
public suspend fun subjectAlternativeDnsNames(vararg values: Output) {
this.subjectAlternativeDnsNames = Output.all(values.asList())
}
/**
* @param values (Output)
* Output only. The subject Alternative DNS names. Only present if parsed is true.
* - - -
*/
@JvmName("saswftgsfsdtgohr")
public suspend fun subjectAlternativeDnsNames(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy