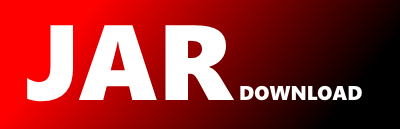
com.pulumi.gcp.logging.kotlin.FolderBucketConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.logging.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.logging.kotlin.outputs.FolderBucketConfigCmekSettings
import com.pulumi.gcp.logging.kotlin.outputs.FolderBucketConfigIndexConfig
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.gcp.logging.kotlin.outputs.FolderBucketConfigCmekSettings.Companion.toKotlin as folderBucketConfigCmekSettingsToKotlin
import com.pulumi.gcp.logging.kotlin.outputs.FolderBucketConfigIndexConfig.Companion.toKotlin as folderBucketConfigIndexConfigToKotlin
/**
* Builder for [FolderBucketConfig].
*/
@PulumiTagMarker
public class FolderBucketConfigResourceBuilder internal constructor() {
public var name: String? = null
public var args: FolderBucketConfigArgs = FolderBucketConfigArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FolderBucketConfigArgsBuilder.() -> Unit) {
val builder = FolderBucketConfigArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): FolderBucketConfig {
val builtJavaResource = com.pulumi.gcp.logging.FolderBucketConfig(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return FolderBucketConfig(builtJavaResource)
}
}
/**
* Manages a folder-level logging bucket config. For more information see
* [the official logging documentation](https://cloud.google.com/logging/docs/) and
* [Storing Logs](https://cloud.google.com/logging/docs/storage).
* > **Note:** Logging buckets are automatically created for a given folder, project, organization, billingAccount and cannot be deleted. Creating a resource of this type will acquire and update the resource that already exists at the desired location. These buckets cannot be removed so deleting this resource will remove the bucket config from your state but will leave the logging bucket unchanged. The buckets that are currently automatically created are "_Default" and "_Required".
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.organizations.Folder("default", {
* displayName: "some-folder-name",
* parent: "organizations/123456789",
* });
* const basic = new gcp.logging.FolderBucketConfig("basic", {
* folder: _default.name,
* location: "global",
* retentionDays: 30,
* bucketId: "_Default",
* indexConfigs: {
* filePath: "jsonPayload.request.status",
* type: "INDEX_TYPE_STRING",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.organizations.Folder("default",
* display_name="some-folder-name",
* parent="organizations/123456789")
* basic = gcp.logging.FolderBucketConfig("basic",
* folder=default.name,
* location="global",
* retention_days=30,
* bucket_id="_Default",
* index_configs={
* "filePath": "jsonPayload.request.status",
* "type": "INDEX_TYPE_STRING",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Organizations.Folder("default", new()
* {
* DisplayName = "some-folder-name",
* Parent = "organizations/123456789",
* });
* var basic = new Gcp.Logging.FolderBucketConfig("basic", new()
* {
* Folder = @default.Name,
* Location = "global",
* RetentionDays = 30,
* BucketId = "_Default",
* IndexConfigs =
* {
* { "filePath", "jsonPayload.request.status" },
* { "type", "INDEX_TYPE_STRING" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/logging"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := organizations.NewFolder(ctx, "default", &organizations.FolderArgs{
* DisplayName: pulumi.String("some-folder-name"),
* Parent: pulumi.String("organizations/123456789"),
* })
* if err != nil {
* return err
* }
* _, err = logging.NewFolderBucketConfig(ctx, "basic", &logging.FolderBucketConfigArgs{
* Folder: _default.Name,
* Location: pulumi.String("global"),
* RetentionDays: pulumi.Int(30),
* BucketId: pulumi.String("_Default"),
* IndexConfigs: logging.FolderBucketConfigIndexConfigArray{
* FilePath: "jsonPayload.request.status",
* Type: "INDEX_TYPE_STRING",
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.Folder;
* import com.pulumi.gcp.organizations.FolderArgs;
* import com.pulumi.gcp.logging.FolderBucketConfig;
* import com.pulumi.gcp.logging.FolderBucketConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Folder("default", FolderArgs.builder()
* .displayName("some-folder-name")
* .parent("organizations/123456789")
* .build());
* var basic = new FolderBucketConfig("basic", FolderBucketConfigArgs.builder()
* .folder(default_.name())
* .location("global")
* .retentionDays(30)
* .bucketId("_Default")
* .indexConfigs(FolderBucketConfigIndexConfigArgs.builder()
* .filePath("jsonPayload.request.status")
* .type("INDEX_TYPE_STRING")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:organizations:Folder
* properties:
* displayName: some-folder-name
* parent: organizations/123456789
* basic:
* type: gcp:logging:FolderBucketConfig
* properties:
* folder: ${default.name}
* location: global
* retentionDays: 30
* bucketId: _Default
* indexConfigs:
* filePath: jsonPayload.request.status
* type: INDEX_TYPE_STRING
* ```
*
* ## Import
* This resource can be imported using the following format:
* * `folders/{{folder}}/locations/{{location}}/buckets/{{bucket_id}}`
* When using the `pulumi import` command, this resource can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:logging/folderBucketConfig:FolderBucketConfig default folders/{{folder}}/locations/{{location}}/buckets/{{bucket_id}}
* ```
*/
public class FolderBucketConfig internal constructor(
override val javaResource: com.pulumi.gcp.logging.FolderBucketConfig,
) : KotlinCustomResource(javaResource, FolderBucketConfigMapper) {
/**
* The name of the logging bucket. Logging automatically creates two log buckets: `_Required` and `_Default`.
*/
public val bucketId: Output
get() = javaResource.bucketId().applyValue({ args0 -> args0 })
/**
* The CMEK settings of the log bucket. If present, new log entries written to this log bucket are encrypted using the CMEK
* key provided in this configuration. If a log bucket has CMEK settings, the CMEK settings cannot be disabled later by
* updating the log bucket. Changing the KMS key is allowed.
*/
public val cmekSettings: Output?
get() = javaResource.cmekSettings().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
folderBucketConfigCmekSettingsToKotlin(args0)
})
}).orElse(null)
})
/**
* Describes this bucket.
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
/**
* The parent resource that contains the logging bucket.
*/
public val folder: Output
get() = javaResource.folder().applyValue({ args0 -> args0 })
/**
* A list of indexed fields and related configuration data. Structure is documented below.
*/
public val indexConfigs: Output>?
get() = javaResource.indexConfigs().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> folderBucketConfigIndexConfigToKotlin(args0) })
})
}).orElse(null)
})
/**
* The bucket's lifecycle such as active or deleted. See [LifecycleState](https://cloud.google.com/logging/docs/reference/v2/rest/v2/billingAccounts.buckets#LogBucket.LifecycleState).
*/
public val lifecycleState: Output
get() = javaResource.lifecycleState().applyValue({ args0 -> args0 })
/**
* The location of the bucket.
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* The resource name of the bucket. For example: "folders/my-folder-id/locations/my-location/buckets/my-bucket-id"
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Logs will be retained by default for this amount of time, after which they will automatically be deleted. The minimum retention period is 1 day. If this value is set to zero at bucket creation time, the default time of 30 days will be used. Bucket retention can not be increased on buckets outside of projects.
*/
public val retentionDays: Output?
get() = javaResource.retentionDays().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object FolderBucketConfigMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.logging.FolderBucketConfig::class == javaResource::class
override fun map(javaResource: Resource): FolderBucketConfig = FolderBucketConfig(
javaResource as
com.pulumi.gcp.logging.FolderBucketConfig,
)
}
/**
* @see [FolderBucketConfig].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [FolderBucketConfig].
*/
public suspend fun folderBucketConfig(
name: String,
block: suspend FolderBucketConfigResourceBuilder.() -> Unit,
): FolderBucketConfig {
val builder = FolderBucketConfigResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [FolderBucketConfig].
* @param name The _unique_ name of the resulting resource.
*/
public fun folderBucketConfig(name: String): FolderBucketConfig {
val builder = FolderBucketConfigResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy