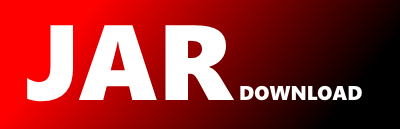
com.pulumi.gcp.migrationcenter.kotlin.inputs.PreferenceSetVirtualMachinePreferencesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.migrationcenter.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.migrationcenter.inputs.PreferenceSetVirtualMachinePreferencesArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property commitmentPlan Commitment plan to consider when calculating costs for virtual machine insights and recommendations. If you are unsure which value to set, a 3 year commitment plan is often a good value to start with.
* Possible values:
* COMMITMENT_PLAN_UNSPECIFIED
* COMMITMENT_PLAN_NONE
* COMMITMENT_PLAN_ONE_YEAR
* COMMITMENT_PLAN_THREE_YEARS
* @property computeEnginePreferences The user preferences relating to Compute Engine target platform.
* Structure is documented below.
* @property regionPreferences The user preferences relating to target regions.
* Structure is documented below.
* @property sizingOptimizationStrategy Sizing optimization strategy specifies the preferred strategy used when extrapolating usage data to calculate insights and recommendations for a virtual machine. If you are unsure which value to set, a moderate sizing optimization strategy is often a good value to start with.
* Possible values:
* SIZING_OPTIMIZATION_STRATEGY_UNSPECIFIED
* SIZING_OPTIMIZATION_STRATEGY_SAME_AS_SOURCE
* SIZING_OPTIMIZATION_STRATEGY_MODERATE
* SIZING_OPTIMIZATION_STRATEGY_AGGRESSIVE
* @property soleTenancyPreferences Preferences concerning Sole Tenancy nodes and VMs.
* Structure is documented below.
* @property targetProduct Target product for assets using this preference set. Specify either target product or business goal, but not both.
* Possible values:
* COMPUTE_MIGRATION_TARGET_PRODUCT_UNSPECIFIED
* COMPUTE_MIGRATION_TARGET_PRODUCT_COMPUTE_ENGINE
* COMPUTE_MIGRATION_TARGET_PRODUCT_VMWARE_ENGINE
* COMPUTE_MIGRATION_TARGET_PRODUCT_SOLE_TENANCY
* @property vmwareEnginePreferences The user preferences relating to Google Cloud VMware Engine target platform.
* Structure is documented below.
*/
public data class PreferenceSetVirtualMachinePreferencesArgs(
public val commitmentPlan: Output? = null,
public val computeEnginePreferences: Output? = null,
public val regionPreferences: Output? =
null,
public val sizingOptimizationStrategy: Output? = null,
public val soleTenancyPreferences: Output? = null,
public val targetProduct: Output? = null,
public val vmwareEnginePreferences: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.migrationcenter.inputs.PreferenceSetVirtualMachinePreferencesArgs =
com.pulumi.gcp.migrationcenter.inputs.PreferenceSetVirtualMachinePreferencesArgs.builder()
.commitmentPlan(commitmentPlan?.applyValue({ args0 -> args0 }))
.computeEnginePreferences(
computeEnginePreferences?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.regionPreferences(regionPreferences?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.sizingOptimizationStrategy(sizingOptimizationStrategy?.applyValue({ args0 -> args0 }))
.soleTenancyPreferences(
soleTenancyPreferences?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.targetProduct(targetProduct?.applyValue({ args0 -> args0 }))
.vmwareEnginePreferences(
vmwareEnginePreferences?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [PreferenceSetVirtualMachinePreferencesArgs].
*/
@PulumiTagMarker
public class PreferenceSetVirtualMachinePreferencesArgsBuilder internal constructor() {
private var commitmentPlan: Output? = null
private var computeEnginePreferences:
Output? = null
private var regionPreferences:
Output? = null
private var sizingOptimizationStrategy: Output? = null
private var soleTenancyPreferences:
Output? = null
private var targetProduct: Output? = null
private var vmwareEnginePreferences:
Output? = null
/**
* @param value Commitment plan to consider when calculating costs for virtual machine insights and recommendations. If you are unsure which value to set, a 3 year commitment plan is often a good value to start with.
* Possible values:
* COMMITMENT_PLAN_UNSPECIFIED
* COMMITMENT_PLAN_NONE
* COMMITMENT_PLAN_ONE_YEAR
* COMMITMENT_PLAN_THREE_YEARS
*/
@JvmName("iskxjygyjdqmldci")
public suspend fun commitmentPlan(`value`: Output) {
this.commitmentPlan = value
}
/**
* @param value The user preferences relating to Compute Engine target platform.
* Structure is documented below.
*/
@JvmName("dhhpxtxmgowhehfv")
public suspend fun computeEnginePreferences(`value`: Output) {
this.computeEnginePreferences = value
}
/**
* @param value The user preferences relating to target regions.
* Structure is documented below.
*/
@JvmName("dkmqkxtkhxrokxej")
public suspend fun regionPreferences(`value`: Output) {
this.regionPreferences = value
}
/**
* @param value Sizing optimization strategy specifies the preferred strategy used when extrapolating usage data to calculate insights and recommendations for a virtual machine. If you are unsure which value to set, a moderate sizing optimization strategy is often a good value to start with.
* Possible values:
* SIZING_OPTIMIZATION_STRATEGY_UNSPECIFIED
* SIZING_OPTIMIZATION_STRATEGY_SAME_AS_SOURCE
* SIZING_OPTIMIZATION_STRATEGY_MODERATE
* SIZING_OPTIMIZATION_STRATEGY_AGGRESSIVE
*/
@JvmName("mgdptsmiladvqmkd")
public suspend fun sizingOptimizationStrategy(`value`: Output) {
this.sizingOptimizationStrategy = value
}
/**
* @param value Preferences concerning Sole Tenancy nodes and VMs.
* Structure is documented below.
*/
@JvmName("hjgfvmdjboolijnv")
public suspend fun soleTenancyPreferences(`value`: Output) {
this.soleTenancyPreferences = value
}
/**
* @param value Target product for assets using this preference set. Specify either target product or business goal, but not both.
* Possible values:
* COMPUTE_MIGRATION_TARGET_PRODUCT_UNSPECIFIED
* COMPUTE_MIGRATION_TARGET_PRODUCT_COMPUTE_ENGINE
* COMPUTE_MIGRATION_TARGET_PRODUCT_VMWARE_ENGINE
* COMPUTE_MIGRATION_TARGET_PRODUCT_SOLE_TENANCY
*/
@JvmName("duwxxeudmjbhonud")
public suspend fun targetProduct(`value`: Output) {
this.targetProduct = value
}
/**
* @param value The user preferences relating to Google Cloud VMware Engine target platform.
* Structure is documented below.
*/
@JvmName("babnhmhehmkpqicd")
public suspend fun vmwareEnginePreferences(`value`: Output) {
this.vmwareEnginePreferences = value
}
/**
* @param value Commitment plan to consider when calculating costs for virtual machine insights and recommendations. If you are unsure which value to set, a 3 year commitment plan is often a good value to start with.
* Possible values:
* COMMITMENT_PLAN_UNSPECIFIED
* COMMITMENT_PLAN_NONE
* COMMITMENT_PLAN_ONE_YEAR
* COMMITMENT_PLAN_THREE_YEARS
*/
@JvmName("chclrgfohtxvtcau")
public suspend fun commitmentPlan(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.commitmentPlan = mapped
}
/**
* @param value The user preferences relating to Compute Engine target platform.
* Structure is documented below.
*/
@JvmName("ajirwqntglkluxaj")
public suspend fun computeEnginePreferences(`value`: PreferenceSetVirtualMachinePreferencesComputeEnginePreferencesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.computeEnginePreferences = mapped
}
/**
* @param argument The user preferences relating to Compute Engine target platform.
* Structure is documented below.
*/
@JvmName("umwckiascyqmlwxn")
public suspend fun computeEnginePreferences(argument: suspend PreferenceSetVirtualMachinePreferencesComputeEnginePreferencesArgsBuilder.() -> Unit) {
val toBeMapped =
PreferenceSetVirtualMachinePreferencesComputeEnginePreferencesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.computeEnginePreferences = mapped
}
/**
* @param value The user preferences relating to target regions.
* Structure is documented below.
*/
@JvmName("xyqcthrupqawtoqb")
public suspend fun regionPreferences(`value`: PreferenceSetVirtualMachinePreferencesRegionPreferencesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.regionPreferences = mapped
}
/**
* @param argument The user preferences relating to target regions.
* Structure is documented below.
*/
@JvmName("onydyndhjuthtyll")
public suspend fun regionPreferences(argument: suspend PreferenceSetVirtualMachinePreferencesRegionPreferencesArgsBuilder.() -> Unit) {
val toBeMapped =
PreferenceSetVirtualMachinePreferencesRegionPreferencesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.regionPreferences = mapped
}
/**
* @param value Sizing optimization strategy specifies the preferred strategy used when extrapolating usage data to calculate insights and recommendations for a virtual machine. If you are unsure which value to set, a moderate sizing optimization strategy is often a good value to start with.
* Possible values:
* SIZING_OPTIMIZATION_STRATEGY_UNSPECIFIED
* SIZING_OPTIMIZATION_STRATEGY_SAME_AS_SOURCE
* SIZING_OPTIMIZATION_STRATEGY_MODERATE
* SIZING_OPTIMIZATION_STRATEGY_AGGRESSIVE
*/
@JvmName("sflosqxsenaxjwrv")
public suspend fun sizingOptimizationStrategy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sizingOptimizationStrategy = mapped
}
/**
* @param value Preferences concerning Sole Tenancy nodes and VMs.
* Structure is documented below.
*/
@JvmName("sgrxwfkvvmnmobnf")
public suspend fun soleTenancyPreferences(`value`: PreferenceSetVirtualMachinePreferencesSoleTenancyPreferencesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.soleTenancyPreferences = mapped
}
/**
* @param argument Preferences concerning Sole Tenancy nodes and VMs.
* Structure is documented below.
*/
@JvmName("gfjckeehljqobgvr")
public suspend fun soleTenancyPreferences(argument: suspend PreferenceSetVirtualMachinePreferencesSoleTenancyPreferencesArgsBuilder.() -> Unit) {
val toBeMapped =
PreferenceSetVirtualMachinePreferencesSoleTenancyPreferencesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.soleTenancyPreferences = mapped
}
/**
* @param value Target product for assets using this preference set. Specify either target product or business goal, but not both.
* Possible values:
* COMPUTE_MIGRATION_TARGET_PRODUCT_UNSPECIFIED
* COMPUTE_MIGRATION_TARGET_PRODUCT_COMPUTE_ENGINE
* COMPUTE_MIGRATION_TARGET_PRODUCT_VMWARE_ENGINE
* COMPUTE_MIGRATION_TARGET_PRODUCT_SOLE_TENANCY
*/
@JvmName("lnpvyfpoltfyolcl")
public suspend fun targetProduct(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetProduct = mapped
}
/**
* @param value The user preferences relating to Google Cloud VMware Engine target platform.
* Structure is documented below.
*/
@JvmName("dhcgyfhmiryncmhf")
public suspend fun vmwareEnginePreferences(`value`: PreferenceSetVirtualMachinePreferencesVmwareEnginePreferencesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vmwareEnginePreferences = mapped
}
/**
* @param argument The user preferences relating to Google Cloud VMware Engine target platform.
* Structure is documented below.
*/
@JvmName("xneanavahdqktkca")
public suspend fun vmwareEnginePreferences(argument: suspend PreferenceSetVirtualMachinePreferencesVmwareEnginePreferencesArgsBuilder.() -> Unit) {
val toBeMapped =
PreferenceSetVirtualMachinePreferencesVmwareEnginePreferencesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.vmwareEnginePreferences = mapped
}
internal fun build(): PreferenceSetVirtualMachinePreferencesArgs =
PreferenceSetVirtualMachinePreferencesArgs(
commitmentPlan = commitmentPlan,
computeEnginePreferences = computeEnginePreferences,
regionPreferences = regionPreferences,
sizingOptimizationStrategy = sizingOptimizationStrategy,
soleTenancyPreferences = soleTenancyPreferences,
targetProduct = targetProduct,
vmwareEnginePreferences = vmwareEnginePreferences,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy