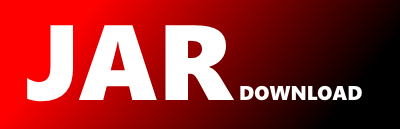
com.pulumi.gcp.monitoring.kotlin.NotificationChannel.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.monitoring.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.monitoring.kotlin.outputs.NotificationChannelSensitiveLabels
import com.pulumi.gcp.monitoring.kotlin.outputs.NotificationChannelSensitiveLabels.Companion.toKotlin
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [NotificationChannel].
*/
@PulumiTagMarker
public class NotificationChannelResourceBuilder internal constructor() {
public var name: String? = null
public var args: NotificationChannelArgs = NotificationChannelArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend NotificationChannelArgsBuilder.() -> Unit) {
val builder = NotificationChannelArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): NotificationChannel {
val builtJavaResource = com.pulumi.gcp.monitoring.NotificationChannel(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return NotificationChannel(builtJavaResource)
}
}
/**
* A NotificationChannel is a medium through which an alert is delivered
* when a policy violation is detected. Examples of channels include email, SMS,
* and third-party messaging applications. Fields containing sensitive information
* like authentication tokens or contact info are only partially populated on retrieval.
* Notification Channels are designed to be flexible and are made up of a supported `type`
* and labels to configure that channel. Each `type` has specific labels that need to be
* present for that channel to be correctly configured. The labels that are required to be
* present for one channel `type` are often different than those required for another.
* Due to these loose constraints it's often best to set up a channel through the UI
* and import it to the provider when setting up a brand new channel type to determine which
* labels are required.
* A list of supported channels per project the `list` endpoint can be
* accessed programmatically or through the api explorer at https://cloud.google.com/monitoring/api/ref_v3/rest/v3/projects.notificationChannelDescriptors/list .
* This provides the channel type and all of the required labels that must be passed.
* To get more information about NotificationChannel, see:
* * [API documentation](https://cloud.google.com/monitoring/api/ref_v3/rest/v3/projects.notificationChannels)
* * How-to Guides
* * [Notification Options](https://cloud.google.com/monitoring/support/notification-options)
* * [Monitoring API Documentation](https://cloud.google.com/monitoring/api/v3/)
* ## Example Usage
* ### Notification Channel Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const basic = new gcp.monitoring.NotificationChannel("basic", {
* displayName: "Test Notification Channel",
* type: "email",
* labels: {
* email_address: "[email protected]",
* },
* forceDelete: false,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* basic = gcp.monitoring.NotificationChannel("basic",
* display_name="Test Notification Channel",
* type="email",
* labels={
* "email_address": "[email protected]",
* },
* force_delete=False)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var basic = new Gcp.Monitoring.NotificationChannel("basic", new()
* {
* DisplayName = "Test Notification Channel",
* Type = "email",
* Labels =
* {
* { "email_address", "[email protected]" },
* },
* ForceDelete = false,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/monitoring"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := monitoring.NewNotificationChannel(ctx, "basic", &monitoring.NotificationChannelArgs{
* DisplayName: pulumi.String("Test Notification Channel"),
* Type: pulumi.String("email"),
* Labels: pulumi.StringMap{
* "email_address": pulumi.String("[email protected]"),
* },
* ForceDelete: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.monitoring.NotificationChannel;
* import com.pulumi.gcp.monitoring.NotificationChannelArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var basic = new NotificationChannel("basic", NotificationChannelArgs.builder()
* .displayName("Test Notification Channel")
* .type("email")
* .labels(Map.of("email_address", "[email protected]"))
* .forceDelete(false)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* basic:
* type: gcp:monitoring:NotificationChannel
* properties:
* displayName: Test Notification Channel
* type: email
* labels:
* email_address: [email protected]
* forceDelete: false
* ```
*
* ### Notification Channel Sensitive
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.monitoring.NotificationChannel("default", {
* displayName: "Test Slack Channel",
* type: "slack",
* labels: {
* channel_name: "#foobar",
* },
* sensitiveLabels: {
* authToken: "one",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.monitoring.NotificationChannel("default",
* display_name="Test Slack Channel",
* type="slack",
* labels={
* "channel_name": "#foobar",
* },
* sensitive_labels=gcp.monitoring.NotificationChannelSensitiveLabelsArgs(
* auth_token="one",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Monitoring.NotificationChannel("default", new()
* {
* DisplayName = "Test Slack Channel",
* Type = "slack",
* Labels =
* {
* { "channel_name", "#foobar" },
* },
* SensitiveLabels = new Gcp.Monitoring.Inputs.NotificationChannelSensitiveLabelsArgs
* {
* AuthToken = "one",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/monitoring"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := monitoring.NewNotificationChannel(ctx, "default", &monitoring.NotificationChannelArgs{
* DisplayName: pulumi.String("Test Slack Channel"),
* Type: pulumi.String("slack"),
* Labels: pulumi.StringMap{
* "channel_name": pulumi.String("#foobar"),
* },
* SensitiveLabels: &monitoring.NotificationChannelSensitiveLabelsArgs{
* AuthToken: pulumi.String("one"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.monitoring.NotificationChannel;
* import com.pulumi.gcp.monitoring.NotificationChannelArgs;
* import com.pulumi.gcp.monitoring.inputs.NotificationChannelSensitiveLabelsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new NotificationChannel("default", NotificationChannelArgs.builder()
* .displayName("Test Slack Channel")
* .type("slack")
* .labels(Map.of("channel_name", "#foobar"))
* .sensitiveLabels(NotificationChannelSensitiveLabelsArgs.builder()
* .authToken("one")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:monitoring:NotificationChannel
* properties:
* displayName: Test Slack Channel
* type: slack
* labels:
* channel_name: '#foobar'
* sensitiveLabels:
* authToken: one
* ```
*
* ## Import
* NotificationChannel can be imported using any of these accepted formats:
* * `{{name}}`
* When using the `pulumi import` command, NotificationChannel can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:monitoring/notificationChannel:NotificationChannel default {{name}}
* ```
*/
public class NotificationChannel internal constructor(
override val javaResource: com.pulumi.gcp.monitoring.NotificationChannel,
) : KotlinCustomResource(javaResource, NotificationChannelMapper) {
/**
* An optional human-readable description of this notification channel. This description may provide additional details, beyond the display name, for the channel. This may not exceed 1024 Unicode characters.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* An optional human-readable name for this notification channel. It is recommended that you specify a non-empty and unique name in order to make it easier to identify the channels in your project, though this is not enforced. The display name is limited to 512 Unicode characters.
*/
public val displayName: Output?
get() = javaResource.displayName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Whether notifications are forwarded to the described channel. This makes it possible to disable delivery of notifications to a particular channel without removing the channel from all alerting policies that reference the channel. This is a more convenient approach when the change is temporary and you want to receive notifications from the same set of alerting policies on the channel at some point in the future.
*/
public val enabled: Output?
get() = javaResource.enabled().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* If true, the notification channel will be deleted regardless
* of its use in alert policies (the policies will be updated
* to remove the channel). If false, channels that are still
* referenced by an existing alerting policy will fail to be
* deleted in a delete operation.
*/
public val forceDelete: Output?
get() = javaResource.forceDelete().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Configuration fields that define the channel and its behavior. The
* permissible and required labels are specified in the
* NotificationChannelDescriptor corresponding to the type field.
* Labels with sensitive data are obfuscated by the API and therefore the provider cannot
* determine if there are upstream changes to these fields. They can also be configured via
* the sensitive_labels block, but cannot be configured in both places.
*/
public val labels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy