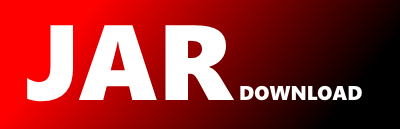
com.pulumi.gcp.monitoring.kotlin.inputs.AlertPolicyConditionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.monitoring.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.monitoring.inputs.AlertPolicyConditionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property conditionAbsent A condition that checks that a time series
* continues to receive new data points.
* Structure is documented below.
* @property conditionMatchedLog A condition that checks for log messages matching given constraints.
* If set, no other conditions can be present.
* Structure is documented below.
* @property conditionMonitoringQueryLanguage A Monitoring Query Language query that outputs a boolean stream
* Structure is documented below.
* @property conditionPrometheusQueryLanguage A condition type that allows alert policies to be defined using
* Prometheus Query Language (PromQL).
* The PrometheusQueryLanguageCondition message contains information
* from a Prometheus alerting rule and its associated rule group.
* Structure is documented below.
* @property conditionThreshold A condition that compares a time series against a
* threshold.
* Structure is documented below.
* @property displayName A short name or phrase used to identify the
* condition in dashboards, notifications, and
* incidents. To avoid confusion, don't use the same
* display name for multiple conditions in the same
* policy.
* @property name (Output)
* The unique resource name for this condition.
* Its syntax is:
* projects/[PROJECT_ID]/alertPolicies/[POLICY_ID]/conditions/[CONDITION_ID]
* [CONDITION_ID] is assigned by Stackdriver Monitoring when
* the condition is created as part of a new or updated alerting
* policy.
*/
public data class AlertPolicyConditionArgs(
public val conditionAbsent: Output? = null,
public val conditionMatchedLog: Output? = null,
public val conditionMonitoringQueryLanguage: Output? = null,
public val conditionPrometheusQueryLanguage: Output? = null,
public val conditionThreshold: Output? = null,
public val displayName: Output,
public val name: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.monitoring.inputs.AlertPolicyConditionArgs =
com.pulumi.gcp.monitoring.inputs.AlertPolicyConditionArgs.builder()
.conditionAbsent(conditionAbsent?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.conditionMatchedLog(
conditionMatchedLog?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.conditionMonitoringQueryLanguage(
conditionMonitoringQueryLanguage?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.conditionPrometheusQueryLanguage(
conditionPrometheusQueryLanguage?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.conditionThreshold(
conditionThreshold?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.displayName(displayName.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AlertPolicyConditionArgs].
*/
@PulumiTagMarker
public class AlertPolicyConditionArgsBuilder internal constructor() {
private var conditionAbsent: Output? = null
private var conditionMatchedLog: Output? = null
private var conditionMonitoringQueryLanguage:
Output? = null
private var conditionPrometheusQueryLanguage:
Output? = null
private var conditionThreshold: Output? = null
private var displayName: Output? = null
private var name: Output? = null
/**
* @param value A condition that checks that a time series
* continues to receive new data points.
* Structure is documented below.
*/
@JvmName("uqjgmothoducfixs")
public suspend fun conditionAbsent(`value`: Output) {
this.conditionAbsent = value
}
/**
* @param value A condition that checks for log messages matching given constraints.
* If set, no other conditions can be present.
* Structure is documented below.
*/
@JvmName("nwbxqaxwsferajuq")
public suspend fun conditionMatchedLog(`value`: Output) {
this.conditionMatchedLog = value
}
/**
* @param value A Monitoring Query Language query that outputs a boolean stream
* Structure is documented below.
*/
@JvmName("isxkhhdsxpkacmwv")
public suspend fun conditionMonitoringQueryLanguage(`value`: Output) {
this.conditionMonitoringQueryLanguage = value
}
/**
* @param value A condition type that allows alert policies to be defined using
* Prometheus Query Language (PromQL).
* The PrometheusQueryLanguageCondition message contains information
* from a Prometheus alerting rule and its associated rule group.
* Structure is documented below.
*/
@JvmName("qjfnrtuswhmnkvqa")
public suspend fun conditionPrometheusQueryLanguage(`value`: Output) {
this.conditionPrometheusQueryLanguage = value
}
/**
* @param value A condition that compares a time series against a
* threshold.
* Structure is documented below.
*/
@JvmName("yfkiokbdhuiuyhtc")
public suspend fun conditionThreshold(`value`: Output) {
this.conditionThreshold = value
}
/**
* @param value A short name or phrase used to identify the
* condition in dashboards, notifications, and
* incidents. To avoid confusion, don't use the same
* display name for multiple conditions in the same
* policy.
*/
@JvmName("ftocaimagewtrlwd")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value (Output)
* The unique resource name for this condition.
* Its syntax is:
* projects/[PROJECT_ID]/alertPolicies/[POLICY_ID]/conditions/[CONDITION_ID]
* [CONDITION_ID] is assigned by Stackdriver Monitoring when
* the condition is created as part of a new or updated alerting
* policy.
*/
@JvmName("kjrgotcqbqpteykf")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value A condition that checks that a time series
* continues to receive new data points.
* Structure is documented below.
*/
@JvmName("pufrfdwtnewbtucf")
public suspend fun conditionAbsent(`value`: AlertPolicyConditionConditionAbsentArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.conditionAbsent = mapped
}
/**
* @param argument A condition that checks that a time series
* continues to receive new data points.
* Structure is documented below.
*/
@JvmName("lbsbwgsyctwjirom")
public suspend fun conditionAbsent(argument: suspend AlertPolicyConditionConditionAbsentArgsBuilder.() -> Unit) {
val toBeMapped = AlertPolicyConditionConditionAbsentArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.conditionAbsent = mapped
}
/**
* @param value A condition that checks for log messages matching given constraints.
* If set, no other conditions can be present.
* Structure is documented below.
*/
@JvmName("qwyckascjiyxqaxt")
public suspend fun conditionMatchedLog(`value`: AlertPolicyConditionConditionMatchedLogArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.conditionMatchedLog = mapped
}
/**
* @param argument A condition that checks for log messages matching given constraints.
* If set, no other conditions can be present.
* Structure is documented below.
*/
@JvmName("wgjhnwanoxvoeijq")
public suspend fun conditionMatchedLog(argument: suspend AlertPolicyConditionConditionMatchedLogArgsBuilder.() -> Unit) {
val toBeMapped = AlertPolicyConditionConditionMatchedLogArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.conditionMatchedLog = mapped
}
/**
* @param value A Monitoring Query Language query that outputs a boolean stream
* Structure is documented below.
*/
@JvmName("dagynqdsmkfihlos")
public suspend fun conditionMonitoringQueryLanguage(`value`: AlertPolicyConditionConditionMonitoringQueryLanguageArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.conditionMonitoringQueryLanguage = mapped
}
/**
* @param argument A Monitoring Query Language query that outputs a boolean stream
* Structure is documented below.
*/
@JvmName("tubvkfbedurguyat")
public suspend fun conditionMonitoringQueryLanguage(argument: suspend AlertPolicyConditionConditionMonitoringQueryLanguageArgsBuilder.() -> Unit) {
val toBeMapped = AlertPolicyConditionConditionMonitoringQueryLanguageArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.conditionMonitoringQueryLanguage = mapped
}
/**
* @param value A condition type that allows alert policies to be defined using
* Prometheus Query Language (PromQL).
* The PrometheusQueryLanguageCondition message contains information
* from a Prometheus alerting rule and its associated rule group.
* Structure is documented below.
*/
@JvmName("sacgprskoqplmrmx")
public suspend fun conditionPrometheusQueryLanguage(`value`: AlertPolicyConditionConditionPrometheusQueryLanguageArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.conditionPrometheusQueryLanguage = mapped
}
/**
* @param argument A condition type that allows alert policies to be defined using
* Prometheus Query Language (PromQL).
* The PrometheusQueryLanguageCondition message contains information
* from a Prometheus alerting rule and its associated rule group.
* Structure is documented below.
*/
@JvmName("guecmaahhfgwwlra")
public suspend fun conditionPrometheusQueryLanguage(argument: suspend AlertPolicyConditionConditionPrometheusQueryLanguageArgsBuilder.() -> Unit) {
val toBeMapped = AlertPolicyConditionConditionPrometheusQueryLanguageArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.conditionPrometheusQueryLanguage = mapped
}
/**
* @param value A condition that compares a time series against a
* threshold.
* Structure is documented below.
*/
@JvmName("tuhxpllirgyjkmql")
public suspend fun conditionThreshold(`value`: AlertPolicyConditionConditionThresholdArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.conditionThreshold = mapped
}
/**
* @param argument A condition that compares a time series against a
* threshold.
* Structure is documented below.
*/
@JvmName("yyjuhusabwxkxids")
public suspend fun conditionThreshold(argument: suspend AlertPolicyConditionConditionThresholdArgsBuilder.() -> Unit) {
val toBeMapped = AlertPolicyConditionConditionThresholdArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.conditionThreshold = mapped
}
/**
* @param value A short name or phrase used to identify the
* condition in dashboards, notifications, and
* incidents. To avoid confusion, don't use the same
* display name for multiple conditions in the same
* policy.
*/
@JvmName("plvrdxbkkeurmbsi")
public suspend fun displayName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.displayName = mapped
}
/**
* @param value (Output)
* The unique resource name for this condition.
* Its syntax is:
* projects/[PROJECT_ID]/alertPolicies/[POLICY_ID]/conditions/[CONDITION_ID]
* [CONDITION_ID] is assigned by Stackdriver Monitoring when
* the condition is created as part of a new or updated alerting
* policy.
*/
@JvmName("ohutftgprubpofhh")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
internal fun build(): AlertPolicyConditionArgs = AlertPolicyConditionArgs(
conditionAbsent = conditionAbsent,
conditionMatchedLog = conditionMatchedLog,
conditionMonitoringQueryLanguage = conditionMonitoringQueryLanguage,
conditionPrometheusQueryLanguage = conditionPrometheusQueryLanguage,
conditionThreshold = conditionThreshold,
displayName = displayName ?: throw PulumiNullFieldException("displayName"),
name = name,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy