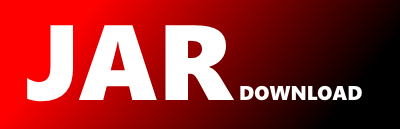
com.pulumi.gcp.monitoring.kotlin.inputs.AlertPolicyConditionConditionPrometheusQueryLanguageArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.monitoring.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.monitoring.inputs.AlertPolicyConditionConditionPrometheusQueryLanguageArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
*
* @property alertRule The alerting rule name of this alert in the corresponding Prometheus
* configuration file.
* Some external tools may require this field to be populated correctly
* in order to refer to the original Prometheus configuration file.
* The rule group name and the alert name are necessary to update the
* relevant AlertPolicies in case the definition of the rule group changes
* in the future.
* This field is optional. If this field is not empty, then it must be a
* valid Prometheus label name.
* - - -
* @property duration Alerts are considered firing once their PromQL expression evaluated
* to be "true" for this long. Alerts whose PromQL expression was not
* evaluated to be "true" for long enough are considered pending. The
* default value is zero. Must be zero or positive.
* @property evaluationInterval How often this rule should be evaluated. Must be a positive multiple
* of 30 seconds or missing. The default value is 30 seconds. If this
* PrometheusQueryLanguageCondition was generated from a Prometheus
* alerting rule, then this value should be taken from the enclosing
* rule group.
* @property labels Labels to add to or overwrite in the PromQL query result. Label names
* must be valid.
* Label values can be templatized by using variables. The only available
* variable names are the names of the labels in the PromQL result, including
* "__name__" and "value". "labels" may be empty. This field is intended to be
* used for organizing and identifying the AlertPolicy
* @property query The PromQL expression to evaluate. Every evaluation cycle this
* expression is evaluated at the current time, and all resultant time
* series become pending/firing alerts. This field must not be empty.
* @property ruleGroup The rule group name of this alert in the corresponding Prometheus
* configuration file.
* Some external tools may require this field to be populated correctly
* in order to refer to the original Prometheus configuration file.
* The rule group name and the alert name are necessary to update the
* relevant AlertPolicies in case the definition of the rule group changes
* in the future. This field is optional.
*/
public data class AlertPolicyConditionConditionPrometheusQueryLanguageArgs(
public val alertRule: Output? = null,
public val duration: Output? = null,
public val evaluationInterval: Output? = null,
public val labels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy