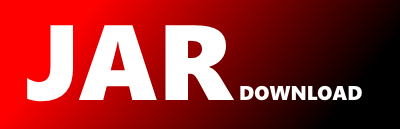
com.pulumi.gcp.monitoring.kotlin.inputs.AlertPolicyConditionConditionThresholdAggregationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.monitoring.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.monitoring.inputs.AlertPolicyConditionConditionThresholdAggregationArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property alignmentPeriod The alignment period for per-time
* series alignment. If present,
* alignmentPeriod must be at least
* 60 seconds. After per-time series
* alignment, each time series will
* contain data points only on the
* period boundaries. If
* perSeriesAligner is not specified
* or equals ALIGN_NONE, then this
* field is ignored. If
* perSeriesAligner is specified and
* does not equal ALIGN_NONE, then
* this field must be defined;
* otherwise an error is returned.
* @property crossSeriesReducer The approach to be used to combine
* time series. Not all reducer
* functions may be applied to all
* time series, depending on the
* metric type and the value type of
* the original time series.
* Reduction may change the metric
* type of value type of the time
* series.Time series data must be
* aligned in order to perform cross-
* time series reduction. If
* crossSeriesReducer is specified,
* then perSeriesAligner must be
* specified and not equal ALIGN_NONE
* and alignmentPeriod must be
* specified; otherwise, an error is
* returned.
* Possible values are: `REDUCE_NONE`, `REDUCE_MEAN`, `REDUCE_MIN`, `REDUCE_MAX`, `REDUCE_SUM`, `REDUCE_STDDEV`, `REDUCE_COUNT`, `REDUCE_COUNT_TRUE`, `REDUCE_COUNT_FALSE`, `REDUCE_FRACTION_TRUE`, `REDUCE_PERCENTILE_99`, `REDUCE_PERCENTILE_95`, `REDUCE_PERCENTILE_50`, `REDUCE_PERCENTILE_05`.
* @property groupByFields The set of fields to preserve when
* crossSeriesReducer is specified.
* The groupByFields determine how
* the time series are partitioned
* into subsets prior to applying the
* aggregation function. Each subset
* contains time series that have the
* same value for each of the
* grouping fields. Each individual
* time series is a member of exactly
* one subset. The crossSeriesReducer
* is applied to each subset of time
* series. It is not possible to
* reduce across different resource
* types, so this field implicitly
* contains resource.type. Fields not
* specified in groupByFields are
* aggregated away. If groupByFields
* is not specified and all the time
* series have the same resource
* type, then the time series are
* aggregated into a single output
* time series. If crossSeriesReducer
* is not defined, this field is
* ignored.
* @property perSeriesAligner The approach to be used to align
* individual time series. Not all
* alignment functions may be applied
* to all time series, depending on
* the metric type and value type of
* the original time series.
* Alignment may change the metric
* type or the value type of the time
* series.Time series data must be
* aligned in order to perform cross-
* time series reduction. If
* crossSeriesReducer is specified,
* then perSeriesAligner must be
* specified and not equal ALIGN_NONE
* and alignmentPeriod must be
* specified; otherwise, an error is
* returned.
* Possible values are: `ALIGN_NONE`, `ALIGN_DELTA`, `ALIGN_RATE`, `ALIGN_INTERPOLATE`, `ALIGN_NEXT_OLDER`, `ALIGN_MIN`, `ALIGN_MAX`, `ALIGN_MEAN`, `ALIGN_COUNT`, `ALIGN_SUM`, `ALIGN_STDDEV`, `ALIGN_COUNT_TRUE`, `ALIGN_COUNT_FALSE`, `ALIGN_FRACTION_TRUE`, `ALIGN_PERCENTILE_99`, `ALIGN_PERCENTILE_95`, `ALIGN_PERCENTILE_50`, `ALIGN_PERCENTILE_05`, `ALIGN_PERCENT_CHANGE`.
*/
public data class AlertPolicyConditionConditionThresholdAggregationArgs(
public val alignmentPeriod: Output? = null,
public val crossSeriesReducer: Output? = null,
public val groupByFields: Output>? = null,
public val perSeriesAligner: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.monitoring.inputs.AlertPolicyConditionConditionThresholdAggregationArgs =
com.pulumi.gcp.monitoring.inputs.AlertPolicyConditionConditionThresholdAggregationArgs.builder()
.alignmentPeriod(alignmentPeriod?.applyValue({ args0 -> args0 }))
.crossSeriesReducer(crossSeriesReducer?.applyValue({ args0 -> args0 }))
.groupByFields(groupByFields?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.perSeriesAligner(perSeriesAligner?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AlertPolicyConditionConditionThresholdAggregationArgs].
*/
@PulumiTagMarker
public class AlertPolicyConditionConditionThresholdAggregationArgsBuilder internal constructor() {
private var alignmentPeriod: Output? = null
private var crossSeriesReducer: Output? = null
private var groupByFields: Output>? = null
private var perSeriesAligner: Output? = null
/**
* @param value The alignment period for per-time
* series alignment. If present,
* alignmentPeriod must be at least
* 60 seconds. After per-time series
* alignment, each time series will
* contain data points only on the
* period boundaries. If
* perSeriesAligner is not specified
* or equals ALIGN_NONE, then this
* field is ignored. If
* perSeriesAligner is specified and
* does not equal ALIGN_NONE, then
* this field must be defined;
* otherwise an error is returned.
*/
@JvmName("lfrgdvmcumvlleqm")
public suspend fun alignmentPeriod(`value`: Output) {
this.alignmentPeriod = value
}
/**
* @param value The approach to be used to combine
* time series. Not all reducer
* functions may be applied to all
* time series, depending on the
* metric type and the value type of
* the original time series.
* Reduction may change the metric
* type of value type of the time
* series.Time series data must be
* aligned in order to perform cross-
* time series reduction. If
* crossSeriesReducer is specified,
* then perSeriesAligner must be
* specified and not equal ALIGN_NONE
* and alignmentPeriod must be
* specified; otherwise, an error is
* returned.
* Possible values are: `REDUCE_NONE`, `REDUCE_MEAN`, `REDUCE_MIN`, `REDUCE_MAX`, `REDUCE_SUM`, `REDUCE_STDDEV`, `REDUCE_COUNT`, `REDUCE_COUNT_TRUE`, `REDUCE_COUNT_FALSE`, `REDUCE_FRACTION_TRUE`, `REDUCE_PERCENTILE_99`, `REDUCE_PERCENTILE_95`, `REDUCE_PERCENTILE_50`, `REDUCE_PERCENTILE_05`.
*/
@JvmName("barsbjmsepfmkeec")
public suspend fun crossSeriesReducer(`value`: Output) {
this.crossSeriesReducer = value
}
/**
* @param value The set of fields to preserve when
* crossSeriesReducer is specified.
* The groupByFields determine how
* the time series are partitioned
* into subsets prior to applying the
* aggregation function. Each subset
* contains time series that have the
* same value for each of the
* grouping fields. Each individual
* time series is a member of exactly
* one subset. The crossSeriesReducer
* is applied to each subset of time
* series. It is not possible to
* reduce across different resource
* types, so this field implicitly
* contains resource.type. Fields not
* specified in groupByFields are
* aggregated away. If groupByFields
* is not specified and all the time
* series have the same resource
* type, then the time series are
* aggregated into a single output
* time series. If crossSeriesReducer
* is not defined, this field is
* ignored.
*/
@JvmName("ueeevoivsbqoqpey")
public suspend fun groupByFields(`value`: Output>) {
this.groupByFields = value
}
@JvmName("jhngrgyntxoupgyh")
public suspend fun groupByFields(vararg values: Output) {
this.groupByFields = Output.all(values.asList())
}
/**
* @param values The set of fields to preserve when
* crossSeriesReducer is specified.
* The groupByFields determine how
* the time series are partitioned
* into subsets prior to applying the
* aggregation function. Each subset
* contains time series that have the
* same value for each of the
* grouping fields. Each individual
* time series is a member of exactly
* one subset. The crossSeriesReducer
* is applied to each subset of time
* series. It is not possible to
* reduce across different resource
* types, so this field implicitly
* contains resource.type. Fields not
* specified in groupByFields are
* aggregated away. If groupByFields
* is not specified and all the time
* series have the same resource
* type, then the time series are
* aggregated into a single output
* time series. If crossSeriesReducer
* is not defined, this field is
* ignored.
*/
@JvmName("nrcscrmntrdxqfua")
public suspend fun groupByFields(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy