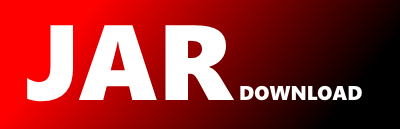
com.pulumi.gcp.monitoring.kotlin.inputs.SloRequestBasedSliArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.monitoring.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.monitoring.inputs.SloRequestBasedSliArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property distributionCut Used when good_service is defined by a count of values aggregated in a
* Distribution that fall into a good range. The total_service is the
* total count of all values aggregated in the Distribution.
* Defines a distribution TimeSeries filter and thresholds used for
* measuring good service and total service.
* Exactly one of `distribution_cut` or `good_total_ratio` can be set.
* Structure is documented below.
* @property goodTotalRatio A means to compute a ratio of `good_service` to `total_service`.
* Defines computing this ratio with two TimeSeries [monitoring filters](https://cloud.google.com/monitoring/api/v3/filters)
* Must specify exactly two of good, bad, and total service filters.
* The relationship good_service + bad_service = total_service
* will be assumed.
* Exactly one of `distribution_cut` or `good_total_ratio` can be set.
* Structure is documented below.
*/
public data class SloRequestBasedSliArgs(
public val distributionCut: Output? = null,
public val goodTotalRatio: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.monitoring.inputs.SloRequestBasedSliArgs =
com.pulumi.gcp.monitoring.inputs.SloRequestBasedSliArgs.builder()
.distributionCut(distributionCut?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.goodTotalRatio(
goodTotalRatio?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [SloRequestBasedSliArgs].
*/
@PulumiTagMarker
public class SloRequestBasedSliArgsBuilder internal constructor() {
private var distributionCut: Output? = null
private var goodTotalRatio: Output? = null
/**
* @param value Used when good_service is defined by a count of values aggregated in a
* Distribution that fall into a good range. The total_service is the
* total count of all values aggregated in the Distribution.
* Defines a distribution TimeSeries filter and thresholds used for
* measuring good service and total service.
* Exactly one of `distribution_cut` or `good_total_ratio` can be set.
* Structure is documented below.
*/
@JvmName("bssyyhknguquqfic")
public suspend fun distributionCut(`value`: Output) {
this.distributionCut = value
}
/**
* @param value A means to compute a ratio of `good_service` to `total_service`.
* Defines computing this ratio with two TimeSeries [monitoring filters](https://cloud.google.com/monitoring/api/v3/filters)
* Must specify exactly two of good, bad, and total service filters.
* The relationship good_service + bad_service = total_service
* will be assumed.
* Exactly one of `distribution_cut` or `good_total_ratio` can be set.
* Structure is documented below.
*/
@JvmName("vyxjhjicyxwtdyyh")
public suspend fun goodTotalRatio(`value`: Output) {
this.goodTotalRatio = value
}
/**
* @param value Used when good_service is defined by a count of values aggregated in a
* Distribution that fall into a good range. The total_service is the
* total count of all values aggregated in the Distribution.
* Defines a distribution TimeSeries filter and thresholds used for
* measuring good service and total service.
* Exactly one of `distribution_cut` or `good_total_ratio` can be set.
* Structure is documented below.
*/
@JvmName("hcluutvfelpvkyix")
public suspend fun distributionCut(`value`: SloRequestBasedSliDistributionCutArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.distributionCut = mapped
}
/**
* @param argument Used when good_service is defined by a count of values aggregated in a
* Distribution that fall into a good range. The total_service is the
* total count of all values aggregated in the Distribution.
* Defines a distribution TimeSeries filter and thresholds used for
* measuring good service and total service.
* Exactly one of `distribution_cut` or `good_total_ratio` can be set.
* Structure is documented below.
*/
@JvmName("mrdgyhugsjcyntmx")
public suspend fun distributionCut(argument: suspend SloRequestBasedSliDistributionCutArgsBuilder.() -> Unit) {
val toBeMapped = SloRequestBasedSliDistributionCutArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.distributionCut = mapped
}
/**
* @param value A means to compute a ratio of `good_service` to `total_service`.
* Defines computing this ratio with two TimeSeries [monitoring filters](https://cloud.google.com/monitoring/api/v3/filters)
* Must specify exactly two of good, bad, and total service filters.
* The relationship good_service + bad_service = total_service
* will be assumed.
* Exactly one of `distribution_cut` or `good_total_ratio` can be set.
* Structure is documented below.
*/
@JvmName("mkmqkllgxknjvyei")
public suspend fun goodTotalRatio(`value`: SloRequestBasedSliGoodTotalRatioArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.goodTotalRatio = mapped
}
/**
* @param argument A means to compute a ratio of `good_service` to `total_service`.
* Defines computing this ratio with two TimeSeries [monitoring filters](https://cloud.google.com/monitoring/api/v3/filters)
* Must specify exactly two of good, bad, and total service filters.
* The relationship good_service + bad_service = total_service
* will be assumed.
* Exactly one of `distribution_cut` or `good_total_ratio` can be set.
* Structure is documented below.
*/
@JvmName("bngxyncdpsrawciq")
public suspend fun goodTotalRatio(argument: suspend SloRequestBasedSliGoodTotalRatioArgsBuilder.() -> Unit) {
val toBeMapped = SloRequestBasedSliGoodTotalRatioArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.goodTotalRatio = mapped
}
internal fun build(): SloRequestBasedSliArgs = SloRequestBasedSliArgs(
distributionCut = distributionCut,
goodTotalRatio = goodTotalRatio,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy