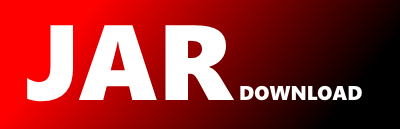
com.pulumi.gcp.monitoring.kotlin.inputs.SloWindowsBasedSliArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.monitoring.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.monitoring.inputs.SloWindowsBasedSliArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property goodBadMetricFilter A TimeSeries [monitoring filter](https://cloud.google.com/monitoring/api/v3/filters)
* with ValueType = BOOL. The window is good if any true values
* appear in the window. One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
* @property goodTotalRatioThreshold Criterion that describes a window as good if its performance is
* high enough. One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
* Structure is documented below.
* @property metricMeanInRange Criterion that describes a window as good if the metric's value
* is in a good range, *averaged* across returned streams.
* One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
* Average value X of `time_series` should satisfy
* `range.min <= X <= range.max` for a good window.
* Structure is documented below.
* @property metricSumInRange Criterion that describes a window as good if the metric's value
* is in a good range, *summed* across returned streams.
* Summed value `X` of `time_series` should satisfy
* `range.min <= X <= range.max` for a good window.
* One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
* Structure is documented below.
* @property windowPeriod Duration over which window quality is evaluated, given as a
* duration string "{X}s" representing X seconds. Must be an
* integer fraction of a day and at least 60s.
*/
public data class SloWindowsBasedSliArgs(
public val goodBadMetricFilter: Output? = null,
public val goodTotalRatioThreshold: Output? = null,
public val metricMeanInRange: Output? = null,
public val metricSumInRange: Output? = null,
public val windowPeriod: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.monitoring.inputs.SloWindowsBasedSliArgs =
com.pulumi.gcp.monitoring.inputs.SloWindowsBasedSliArgs.builder()
.goodBadMetricFilter(goodBadMetricFilter?.applyValue({ args0 -> args0 }))
.goodTotalRatioThreshold(
goodTotalRatioThreshold?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.metricMeanInRange(metricMeanInRange?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.metricSumInRange(metricSumInRange?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.windowPeriod(windowPeriod?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SloWindowsBasedSliArgs].
*/
@PulumiTagMarker
public class SloWindowsBasedSliArgsBuilder internal constructor() {
private var goodBadMetricFilter: Output? = null
private var goodTotalRatioThreshold: Output? = null
private var metricMeanInRange: Output? = null
private var metricSumInRange: Output? = null
private var windowPeriod: Output? = null
/**
* @param value A TimeSeries [monitoring filter](https://cloud.google.com/monitoring/api/v3/filters)
* with ValueType = BOOL. The window is good if any true values
* appear in the window. One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
*/
@JvmName("wdyliutlblqjctdr")
public suspend fun goodBadMetricFilter(`value`: Output) {
this.goodBadMetricFilter = value
}
/**
* @param value Criterion that describes a window as good if its performance is
* high enough. One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
* Structure is documented below.
*/
@JvmName("rphyferamorscmcx")
public suspend fun goodTotalRatioThreshold(`value`: Output) {
this.goodTotalRatioThreshold = value
}
/**
* @param value Criterion that describes a window as good if the metric's value
* is in a good range, *averaged* across returned streams.
* One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
* Average value X of `time_series` should satisfy
* `range.min <= X <= range.max` for a good window.
* Structure is documented below.
*/
@JvmName("alansnncxpfyswmc")
public suspend fun metricMeanInRange(`value`: Output) {
this.metricMeanInRange = value
}
/**
* @param value Criterion that describes a window as good if the metric's value
* is in a good range, *summed* across returned streams.
* Summed value `X` of `time_series` should satisfy
* `range.min <= X <= range.max` for a good window.
* One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
* Structure is documented below.
*/
@JvmName("veadgcyqvifyqwrb")
public suspend fun metricSumInRange(`value`: Output) {
this.metricSumInRange = value
}
/**
* @param value Duration over which window quality is evaluated, given as a
* duration string "{X}s" representing X seconds. Must be an
* integer fraction of a day and at least 60s.
*/
@JvmName("iwttwyadhdkpderw")
public suspend fun windowPeriod(`value`: Output) {
this.windowPeriod = value
}
/**
* @param value A TimeSeries [monitoring filter](https://cloud.google.com/monitoring/api/v3/filters)
* with ValueType = BOOL. The window is good if any true values
* appear in the window. One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
*/
@JvmName("dahovifqdiblavmg")
public suspend fun goodBadMetricFilter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.goodBadMetricFilter = mapped
}
/**
* @param value Criterion that describes a window as good if its performance is
* high enough. One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
* Structure is documented below.
*/
@JvmName("qypakcgdvwdxjown")
public suspend fun goodTotalRatioThreshold(`value`: SloWindowsBasedSliGoodTotalRatioThresholdArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.goodTotalRatioThreshold = mapped
}
/**
* @param argument Criterion that describes a window as good if its performance is
* high enough. One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
* Structure is documented below.
*/
@JvmName("fxkapbfroisuqclp")
public suspend fun goodTotalRatioThreshold(argument: suspend SloWindowsBasedSliGoodTotalRatioThresholdArgsBuilder.() -> Unit) {
val toBeMapped = SloWindowsBasedSliGoodTotalRatioThresholdArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.goodTotalRatioThreshold = mapped
}
/**
* @param value Criterion that describes a window as good if the metric's value
* is in a good range, *averaged* across returned streams.
* One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
* Average value X of `time_series` should satisfy
* `range.min <= X <= range.max` for a good window.
* Structure is documented below.
*/
@JvmName("pdsvkdegfpdrhsey")
public suspend fun metricMeanInRange(`value`: SloWindowsBasedSliMetricMeanInRangeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metricMeanInRange = mapped
}
/**
* @param argument Criterion that describes a window as good if the metric's value
* is in a good range, *averaged* across returned streams.
* One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
* Average value X of `time_series` should satisfy
* `range.min <= X <= range.max` for a good window.
* Structure is documented below.
*/
@JvmName("qgrpgbruixidilqn")
public suspend fun metricMeanInRange(argument: suspend SloWindowsBasedSliMetricMeanInRangeArgsBuilder.() -> Unit) {
val toBeMapped = SloWindowsBasedSliMetricMeanInRangeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.metricMeanInRange = mapped
}
/**
* @param value Criterion that describes a window as good if the metric's value
* is in a good range, *summed* across returned streams.
* Summed value `X` of `time_series` should satisfy
* `range.min <= X <= range.max` for a good window.
* One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
* Structure is documented below.
*/
@JvmName("whjgbpgsiktehmau")
public suspend fun metricSumInRange(`value`: SloWindowsBasedSliMetricSumInRangeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metricSumInRange = mapped
}
/**
* @param argument Criterion that describes a window as good if the metric's value
* is in a good range, *summed* across returned streams.
* Summed value `X` of `time_series` should satisfy
* `range.min <= X <= range.max` for a good window.
* One of `good_bad_metric_filter`,
* `good_total_ratio_threshold`, `metric_mean_in_range`,
* `metric_sum_in_range` must be set for `windows_based_sli`.
* Structure is documented below.
*/
@JvmName("fngmnguianhmcmad")
public suspend fun metricSumInRange(argument: suspend SloWindowsBasedSliMetricSumInRangeArgsBuilder.() -> Unit) {
val toBeMapped = SloWindowsBasedSliMetricSumInRangeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.metricSumInRange = mapped
}
/**
* @param value Duration over which window quality is evaluated, given as a
* duration string "{X}s" representing X seconds. Must be an
* integer fraction of a day and at least 60s.
*/
@JvmName("fgwsfxjtweewmnne")
public suspend fun windowPeriod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.windowPeriod = mapped
}
internal fun build(): SloWindowsBasedSliArgs = SloWindowsBasedSliArgs(
goodBadMetricFilter = goodBadMetricFilter,
goodTotalRatioThreshold = goodTotalRatioThreshold,
metricMeanInRange = metricMeanInRange,
metricSumInRange = metricSumInRange,
windowPeriod = windowPeriod,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy