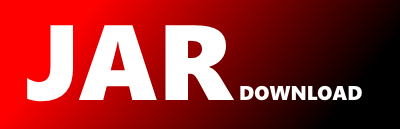
com.pulumi.gcp.monitoring.kotlin.inputs.UptimeCheckConfigContentMatcherArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.monitoring.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.monitoring.inputs.UptimeCheckConfigContentMatcherArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property content String or regex content to match (max 1024 bytes)
* @property jsonPathMatcher Information needed to perform a JSONPath content match. Used for `ContentMatcherOption::MATCHES_JSON_PATH` and `ContentMatcherOption::NOT_MATCHES_JSON_PATH`.
* Structure is documented below.
* @property matcher The type of content matcher that will be applied to the server output, compared to the content string when the check is run.
* Default value is `CONTAINS_STRING`.
* Possible values are: `CONTAINS_STRING`, `NOT_CONTAINS_STRING`, `MATCHES_REGEX`, `NOT_MATCHES_REGEX`, `MATCHES_JSON_PATH`, `NOT_MATCHES_JSON_PATH`.
*/
public data class UptimeCheckConfigContentMatcherArgs(
public val content: Output,
public val jsonPathMatcher: Output? = null,
public val matcher: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.monitoring.inputs.UptimeCheckConfigContentMatcherArgs =
com.pulumi.gcp.monitoring.inputs.UptimeCheckConfigContentMatcherArgs.builder()
.content(content.applyValue({ args0 -> args0 }))
.jsonPathMatcher(jsonPathMatcher?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.matcher(matcher?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [UptimeCheckConfigContentMatcherArgs].
*/
@PulumiTagMarker
public class UptimeCheckConfigContentMatcherArgsBuilder internal constructor() {
private var content: Output? = null
private var jsonPathMatcher: Output? = null
private var matcher: Output? = null
/**
* @param value String or regex content to match (max 1024 bytes)
*/
@JvmName("mxugcyitlkxyfftb")
public suspend fun content(`value`: Output) {
this.content = value
}
/**
* @param value Information needed to perform a JSONPath content match. Used for `ContentMatcherOption::MATCHES_JSON_PATH` and `ContentMatcherOption::NOT_MATCHES_JSON_PATH`.
* Structure is documented below.
*/
@JvmName("kjdapvhpnofbwtad")
public suspend fun jsonPathMatcher(`value`: Output) {
this.jsonPathMatcher = value
}
/**
* @param value The type of content matcher that will be applied to the server output, compared to the content string when the check is run.
* Default value is `CONTAINS_STRING`.
* Possible values are: `CONTAINS_STRING`, `NOT_CONTAINS_STRING`, `MATCHES_REGEX`, `NOT_MATCHES_REGEX`, `MATCHES_JSON_PATH`, `NOT_MATCHES_JSON_PATH`.
*/
@JvmName("hjdminowaxnotvyw")
public suspend fun matcher(`value`: Output) {
this.matcher = value
}
/**
* @param value String or regex content to match (max 1024 bytes)
*/
@JvmName("hsnswbdmfcymnmyg")
public suspend fun content(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.content = mapped
}
/**
* @param value Information needed to perform a JSONPath content match. Used for `ContentMatcherOption::MATCHES_JSON_PATH` and `ContentMatcherOption::NOT_MATCHES_JSON_PATH`.
* Structure is documented below.
*/
@JvmName("avajltslaformlcs")
public suspend fun jsonPathMatcher(`value`: UptimeCheckConfigContentMatcherJsonPathMatcherArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.jsonPathMatcher = mapped
}
/**
* @param argument Information needed to perform a JSONPath content match. Used for `ContentMatcherOption::MATCHES_JSON_PATH` and `ContentMatcherOption::NOT_MATCHES_JSON_PATH`.
* Structure is documented below.
*/
@JvmName("jtxferlhhhignelp")
public suspend fun jsonPathMatcher(argument: suspend UptimeCheckConfigContentMatcherJsonPathMatcherArgsBuilder.() -> Unit) {
val toBeMapped = UptimeCheckConfigContentMatcherJsonPathMatcherArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.jsonPathMatcher = mapped
}
/**
* @param value The type of content matcher that will be applied to the server output, compared to the content string when the check is run.
* Default value is `CONTAINS_STRING`.
* Possible values are: `CONTAINS_STRING`, `NOT_CONTAINS_STRING`, `MATCHES_REGEX`, `NOT_MATCHES_REGEX`, `MATCHES_JSON_PATH`, `NOT_MATCHES_JSON_PATH`.
*/
@JvmName("lxpyricobdtmhlxa")
public suspend fun matcher(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.matcher = mapped
}
internal fun build(): UptimeCheckConfigContentMatcherArgs = UptimeCheckConfigContentMatcherArgs(
content = content ?: throw PulumiNullFieldException("content"),
jsonPathMatcher = jsonPathMatcher,
matcher = matcher,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy