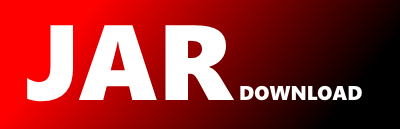
com.pulumi.gcp.netapp.kotlin.inputs.VolumeExportPolicyRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.netapp.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.netapp.inputs.VolumeExportPolicyRuleArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property accessType Defines the access type for clients matching the `allowedClients` specification.
* Possible values are: `READ_ONLY`, `READ_WRITE`, `READ_NONE`.
* @property allowedClients Defines the client ingress specification (allowed clients) as a comma seperated list with IPv4 CIDRs or IPv4 host addresses.
* @property hasRootAccess If enabled, the root user (UID = 0) of the specified clients doesn't get mapped to nobody (UID = 65534). This is also known as no_root_squash.
* @property kerberos5ReadOnly If enabled (true) the rule defines a read only access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'authentication' kerberos security mode.
* @property kerberos5ReadWrite If enabled (true) the rule defines read and write access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'authentication' kerberos security mode. The 'kerberos5ReadOnly' value is ignored if this is enabled.
* @property kerberos5iReadOnly If enabled (true) the rule defines a read only access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'integrity' kerberos security mode.
* @property kerberos5iReadWrite If enabled (true) the rule defines read and write access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'integrity' kerberos security mode. The 'kerberos5iReadOnly' value is ignored if this is enabled.
* @property kerberos5pReadOnly If enabled (true) the rule defines a read only access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'privacy' kerberos security mode.
* @property kerberos5pReadWrite If enabled (true) the rule defines read and write access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'privacy' kerberos security mode. The 'kerberos5pReadOnly' value is ignored if this is enabled.
* @property nfsv3 Enable to apply the export rule to NFSV3 clients.
* @property nfsv4 Enable to apply the export rule to NFSV4.1 clients.
*/
public data class VolumeExportPolicyRuleArgs(
public val accessType: Output? = null,
public val allowedClients: Output? = null,
public val hasRootAccess: Output? = null,
public val kerberos5ReadOnly: Output? = null,
public val kerberos5ReadWrite: Output? = null,
public val kerberos5iReadOnly: Output? = null,
public val kerberos5iReadWrite: Output? = null,
public val kerberos5pReadOnly: Output? = null,
public val kerberos5pReadWrite: Output? = null,
public val nfsv3: Output? = null,
public val nfsv4: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.netapp.inputs.VolumeExportPolicyRuleArgs =
com.pulumi.gcp.netapp.inputs.VolumeExportPolicyRuleArgs.builder()
.accessType(accessType?.applyValue({ args0 -> args0 }))
.allowedClients(allowedClients?.applyValue({ args0 -> args0 }))
.hasRootAccess(hasRootAccess?.applyValue({ args0 -> args0 }))
.kerberos5ReadOnly(kerberos5ReadOnly?.applyValue({ args0 -> args0 }))
.kerberos5ReadWrite(kerberos5ReadWrite?.applyValue({ args0 -> args0 }))
.kerberos5iReadOnly(kerberos5iReadOnly?.applyValue({ args0 -> args0 }))
.kerberos5iReadWrite(kerberos5iReadWrite?.applyValue({ args0 -> args0 }))
.kerberos5pReadOnly(kerberos5pReadOnly?.applyValue({ args0 -> args0 }))
.kerberos5pReadWrite(kerberos5pReadWrite?.applyValue({ args0 -> args0 }))
.nfsv3(nfsv3?.applyValue({ args0 -> args0 }))
.nfsv4(nfsv4?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VolumeExportPolicyRuleArgs].
*/
@PulumiTagMarker
public class VolumeExportPolicyRuleArgsBuilder internal constructor() {
private var accessType: Output? = null
private var allowedClients: Output? = null
private var hasRootAccess: Output? = null
private var kerberos5ReadOnly: Output? = null
private var kerberos5ReadWrite: Output? = null
private var kerberos5iReadOnly: Output? = null
private var kerberos5iReadWrite: Output? = null
private var kerberos5pReadOnly: Output? = null
private var kerberos5pReadWrite: Output? = null
private var nfsv3: Output? = null
private var nfsv4: Output? = null
/**
* @param value Defines the access type for clients matching the `allowedClients` specification.
* Possible values are: `READ_ONLY`, `READ_WRITE`, `READ_NONE`.
*/
@JvmName("hejpbaawgemgunbd")
public suspend fun accessType(`value`: Output) {
this.accessType = value
}
/**
* @param value Defines the client ingress specification (allowed clients) as a comma seperated list with IPv4 CIDRs or IPv4 host addresses.
*/
@JvmName("mqwmlfdkipqlutrg")
public suspend fun allowedClients(`value`: Output) {
this.allowedClients = value
}
/**
* @param value If enabled, the root user (UID = 0) of the specified clients doesn't get mapped to nobody (UID = 65534). This is also known as no_root_squash.
*/
@JvmName("hqsqnmveqtyiqokm")
public suspend fun hasRootAccess(`value`: Output) {
this.hasRootAccess = value
}
/**
* @param value If enabled (true) the rule defines a read only access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'authentication' kerberos security mode.
*/
@JvmName("mtgquqpumxkvjvfl")
public suspend fun kerberos5ReadOnly(`value`: Output) {
this.kerberos5ReadOnly = value
}
/**
* @param value If enabled (true) the rule defines read and write access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'authentication' kerberos security mode. The 'kerberos5ReadOnly' value is ignored if this is enabled.
*/
@JvmName("njrbkstutsdrlcrn")
public suspend fun kerberos5ReadWrite(`value`: Output) {
this.kerberos5ReadWrite = value
}
/**
* @param value If enabled (true) the rule defines a read only access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'integrity' kerberos security mode.
*/
@JvmName("cakkeshdcxeqxrvx")
public suspend fun kerberos5iReadOnly(`value`: Output) {
this.kerberos5iReadOnly = value
}
/**
* @param value If enabled (true) the rule defines read and write access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'integrity' kerberos security mode. The 'kerberos5iReadOnly' value is ignored if this is enabled.
*/
@JvmName("rnrsmwgvmcjdubis")
public suspend fun kerberos5iReadWrite(`value`: Output) {
this.kerberos5iReadWrite = value
}
/**
* @param value If enabled (true) the rule defines a read only access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'privacy' kerberos security mode.
*/
@JvmName("ikqpdanpnavpqltd")
public suspend fun kerberos5pReadOnly(`value`: Output) {
this.kerberos5pReadOnly = value
}
/**
* @param value If enabled (true) the rule defines read and write access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'privacy' kerberos security mode. The 'kerberos5pReadOnly' value is ignored if this is enabled.
*/
@JvmName("evppxeexmrxtypev")
public suspend fun kerberos5pReadWrite(`value`: Output) {
this.kerberos5pReadWrite = value
}
/**
* @param value Enable to apply the export rule to NFSV3 clients.
*/
@JvmName("nfjxqepfhplbchrs")
public suspend fun nfsv3(`value`: Output) {
this.nfsv3 = value
}
/**
* @param value Enable to apply the export rule to NFSV4.1 clients.
*/
@JvmName("capuwcdpqxolenyl")
public suspend fun nfsv4(`value`: Output) {
this.nfsv4 = value
}
/**
* @param value Defines the access type for clients matching the `allowedClients` specification.
* Possible values are: `READ_ONLY`, `READ_WRITE`, `READ_NONE`.
*/
@JvmName("fahbbukntdwusatf")
public suspend fun accessType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accessType = mapped
}
/**
* @param value Defines the client ingress specification (allowed clients) as a comma seperated list with IPv4 CIDRs or IPv4 host addresses.
*/
@JvmName("dwpdmbjbtqbswmjk")
public suspend fun allowedClients(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowedClients = mapped
}
/**
* @param value If enabled, the root user (UID = 0) of the specified clients doesn't get mapped to nobody (UID = 65534). This is also known as no_root_squash.
*/
@JvmName("yyidudulayesnxtq")
public suspend fun hasRootAccess(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hasRootAccess = mapped
}
/**
* @param value If enabled (true) the rule defines a read only access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'authentication' kerberos security mode.
*/
@JvmName("nodxbdktyfeuwrpl")
public suspend fun kerberos5ReadOnly(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kerberos5ReadOnly = mapped
}
/**
* @param value If enabled (true) the rule defines read and write access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'authentication' kerberos security mode. The 'kerberos5ReadOnly' value is ignored if this is enabled.
*/
@JvmName("finwyopafhitnbsr")
public suspend fun kerberos5ReadWrite(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kerberos5ReadWrite = mapped
}
/**
* @param value If enabled (true) the rule defines a read only access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'integrity' kerberos security mode.
*/
@JvmName("ojhoaiutlmnljigl")
public suspend fun kerberos5iReadOnly(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kerberos5iReadOnly = mapped
}
/**
* @param value If enabled (true) the rule defines read and write access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'integrity' kerberos security mode. The 'kerberos5iReadOnly' value is ignored if this is enabled.
*/
@JvmName("bntcueiovjsaxiwr")
public suspend fun kerberos5iReadWrite(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kerberos5iReadWrite = mapped
}
/**
* @param value If enabled (true) the rule defines a read only access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'privacy' kerberos security mode.
*/
@JvmName("xndaaohrrbtmonyn")
public suspend fun kerberos5pReadOnly(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kerberos5pReadOnly = mapped
}
/**
* @param value If enabled (true) the rule defines read and write access for clients matching the 'allowedClients' specification. It enables nfs clients to mount using 'privacy' kerberos security mode. The 'kerberos5pReadOnly' value is ignored if this is enabled.
*/
@JvmName("mxruelsfmsejenif")
public suspend fun kerberos5pReadWrite(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kerberos5pReadWrite = mapped
}
/**
* @param value Enable to apply the export rule to NFSV3 clients.
*/
@JvmName("wlvjccxdqevrvoff")
public suspend fun nfsv3(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nfsv3 = mapped
}
/**
* @param value Enable to apply the export rule to NFSV4.1 clients.
*/
@JvmName("jxckqamnykypqyqf")
public suspend fun nfsv4(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nfsv4 = mapped
}
internal fun build(): VolumeExportPolicyRuleArgs = VolumeExportPolicyRuleArgs(
accessType = accessType,
allowedClients = allowedClients,
hasRootAccess = hasRootAccess,
kerberos5ReadOnly = kerberos5ReadOnly,
kerberos5ReadWrite = kerberos5ReadWrite,
kerberos5iReadOnly = kerberos5iReadOnly,
kerberos5iReadWrite = kerberos5iReadWrite,
kerberos5pReadOnly = kerberos5pReadOnly,
kerberos5pReadWrite = kerberos5pReadWrite,
nfsv3 = nfsv3,
nfsv4 = nfsv4,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy