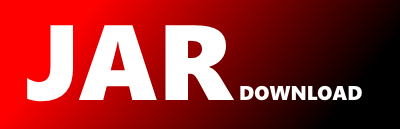
com.pulumi.gcp.netapp.kotlin.inputs.VolumeReplicationDestinationVolumeParametersArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.netapp.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.netapp.inputs.VolumeReplicationDestinationVolumeParametersArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property description Description for the destination volume.
* @property shareName Share name for destination volume. If not specified, name of source volume's share name will be used.
* @property storagePool Name of an existing storage pool for the destination volume with format: `projects/{{project}}/locations/{{location}}/storagePools/{{poolId}}`
* @property volumeId Name for the destination volume to be created. If not specified, the name of the source volume will be used.
*/
public data class VolumeReplicationDestinationVolumeParametersArgs(
public val description: Output? = null,
public val shareName: Output? = null,
public val storagePool: Output,
public val volumeId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.netapp.inputs.VolumeReplicationDestinationVolumeParametersArgs =
com.pulumi.gcp.netapp.inputs.VolumeReplicationDestinationVolumeParametersArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.shareName(shareName?.applyValue({ args0 -> args0 }))
.storagePool(storagePool.applyValue({ args0 -> args0 }))
.volumeId(volumeId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VolumeReplicationDestinationVolumeParametersArgs].
*/
@PulumiTagMarker
public class VolumeReplicationDestinationVolumeParametersArgsBuilder internal constructor() {
private var description: Output? = null
private var shareName: Output? = null
private var storagePool: Output? = null
private var volumeId: Output? = null
/**
* @param value Description for the destination volume.
*/
@JvmName("mspndeclabwodruy")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Share name for destination volume. If not specified, name of source volume's share name will be used.
*/
@JvmName("aeekovntbxntdjqq")
public suspend fun shareName(`value`: Output) {
this.shareName = value
}
/**
* @param value Name of an existing storage pool for the destination volume with format: `projects/{{project}}/locations/{{location}}/storagePools/{{poolId}}`
*/
@JvmName("kphxrgtqrgybxnlk")
public suspend fun storagePool(`value`: Output) {
this.storagePool = value
}
/**
* @param value Name for the destination volume to be created. If not specified, the name of the source volume will be used.
*/
@JvmName("xnpioirnpfiqbdbb")
public suspend fun volumeId(`value`: Output) {
this.volumeId = value
}
/**
* @param value Description for the destination volume.
*/
@JvmName("glimpsrevjwaofdb")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Share name for destination volume. If not specified, name of source volume's share name will be used.
*/
@JvmName("evcroybhohavgovm")
public suspend fun shareName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.shareName = mapped
}
/**
* @param value Name of an existing storage pool for the destination volume with format: `projects/{{project}}/locations/{{location}}/storagePools/{{poolId}}`
*/
@JvmName("wybspmacxcgdgcrn")
public suspend fun storagePool(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.storagePool = mapped
}
/**
* @param value Name for the destination volume to be created. If not specified, the name of the source volume will be used.
*/
@JvmName("tpoqivipeumkjocd")
public suspend fun volumeId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.volumeId = mapped
}
internal fun build(): VolumeReplicationDestinationVolumeParametersArgs =
VolumeReplicationDestinationVolumeParametersArgs(
description = description,
shareName = shareName,
storagePool = storagePool ?: throw PulumiNullFieldException("storagePool"),
volumeId = volumeId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy