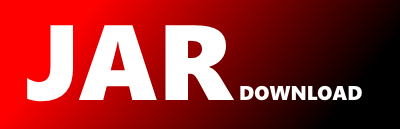
com.pulumi.gcp.netapp.kotlin.inputs.VolumeSnapshotPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.netapp.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.netapp.inputs.VolumeSnapshotPolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property dailySchedule Daily schedule policy.
* Structure is documented below.
* @property enabled Enables automated snapshot creation according to defined schedule. Default is false.
* To disable automatic snapshot creation you have to remove the whole snapshot_policy block.
* @property hourlySchedule Hourly schedule policy.
* Structure is documented below.
* @property monthlySchedule Monthly schedule policy.
* Structure is documented below.
* @property weeklySchedule Weekly schedule policy.
* Structure is documented below.
*/
public data class VolumeSnapshotPolicyArgs(
public val dailySchedule: Output? = null,
public val enabled: Output? = null,
public val hourlySchedule: Output? = null,
public val monthlySchedule: Output? = null,
public val weeklySchedule: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.netapp.inputs.VolumeSnapshotPolicyArgs =
com.pulumi.gcp.netapp.inputs.VolumeSnapshotPolicyArgs.builder()
.dailySchedule(dailySchedule?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.enabled(enabled?.applyValue({ args0 -> args0 }))
.hourlySchedule(hourlySchedule?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.monthlySchedule(monthlySchedule?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.weeklySchedule(
weeklySchedule?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [VolumeSnapshotPolicyArgs].
*/
@PulumiTagMarker
public class VolumeSnapshotPolicyArgsBuilder internal constructor() {
private var dailySchedule: Output? = null
private var enabled: Output? = null
private var hourlySchedule: Output? = null
private var monthlySchedule: Output? = null
private var weeklySchedule: Output? = null
/**
* @param value Daily schedule policy.
* Structure is documented below.
*/
@JvmName("evedejwetttnffjq")
public suspend fun dailySchedule(`value`: Output) {
this.dailySchedule = value
}
/**
* @param value Enables automated snapshot creation according to defined schedule. Default is false.
* To disable automatic snapshot creation you have to remove the whole snapshot_policy block.
*/
@JvmName("glkpbeyyaihshvop")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value Hourly schedule policy.
* Structure is documented below.
*/
@JvmName("hggckxgveicignvw")
public suspend fun hourlySchedule(`value`: Output) {
this.hourlySchedule = value
}
/**
* @param value Monthly schedule policy.
* Structure is documented below.
*/
@JvmName("mjyskxmrxtgdypkt")
public suspend fun monthlySchedule(`value`: Output) {
this.monthlySchedule = value
}
/**
* @param value Weekly schedule policy.
* Structure is documented below.
*/
@JvmName("sxrwfxvuwfrbfovg")
public suspend fun weeklySchedule(`value`: Output) {
this.weeklySchedule = value
}
/**
* @param value Daily schedule policy.
* Structure is documented below.
*/
@JvmName("fhhsumsjopgmycoh")
public suspend fun dailySchedule(`value`: VolumeSnapshotPolicyDailyScheduleArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dailySchedule = mapped
}
/**
* @param argument Daily schedule policy.
* Structure is documented below.
*/
@JvmName("cevqlgjkqjwgvevh")
public suspend fun dailySchedule(argument: suspend VolumeSnapshotPolicyDailyScheduleArgsBuilder.() -> Unit) {
val toBeMapped = VolumeSnapshotPolicyDailyScheduleArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dailySchedule = mapped
}
/**
* @param value Enables automated snapshot creation according to defined schedule. Default is false.
* To disable automatic snapshot creation you have to remove the whole snapshot_policy block.
*/
@JvmName("fbmnfegyjleajanp")
public suspend fun enabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enabled = mapped
}
/**
* @param value Hourly schedule policy.
* Structure is documented below.
*/
@JvmName("wtqaqnqvpmgbfmrc")
public suspend fun hourlySchedule(`value`: VolumeSnapshotPolicyHourlyScheduleArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hourlySchedule = mapped
}
/**
* @param argument Hourly schedule policy.
* Structure is documented below.
*/
@JvmName("hwccihbyyjwbvpbw")
public suspend fun hourlySchedule(argument: suspend VolumeSnapshotPolicyHourlyScheduleArgsBuilder.() -> Unit) {
val toBeMapped = VolumeSnapshotPolicyHourlyScheduleArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.hourlySchedule = mapped
}
/**
* @param value Monthly schedule policy.
* Structure is documented below.
*/
@JvmName("rmpubspxcrriwefr")
public suspend fun monthlySchedule(`value`: VolumeSnapshotPolicyMonthlyScheduleArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.monthlySchedule = mapped
}
/**
* @param argument Monthly schedule policy.
* Structure is documented below.
*/
@JvmName("laykkuboritkwsub")
public suspend fun monthlySchedule(argument: suspend VolumeSnapshotPolicyMonthlyScheduleArgsBuilder.() -> Unit) {
val toBeMapped = VolumeSnapshotPolicyMonthlyScheduleArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.monthlySchedule = mapped
}
/**
* @param value Weekly schedule policy.
* Structure is documented below.
*/
@JvmName("glxdmsmbqkeihvqe")
public suspend fun weeklySchedule(`value`: VolumeSnapshotPolicyWeeklyScheduleArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.weeklySchedule = mapped
}
/**
* @param argument Weekly schedule policy.
* Structure is documented below.
*/
@JvmName("lerfdxwsupwrdacf")
public suspend fun weeklySchedule(argument: suspend VolumeSnapshotPolicyWeeklyScheduleArgsBuilder.() -> Unit) {
val toBeMapped = VolumeSnapshotPolicyWeeklyScheduleArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.weeklySchedule = mapped
}
internal fun build(): VolumeSnapshotPolicyArgs = VolumeSnapshotPolicyArgs(
dailySchedule = dailySchedule,
enabled = enabled,
hourlySchedule = hourlySchedule,
monthlySchedule = monthlySchedule,
weeklySchedule = weeklySchedule,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy