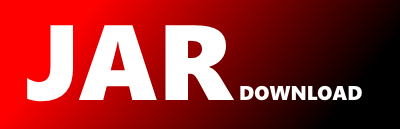
com.pulumi.gcp.networkconnectivity.kotlin.Hub.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.networkconnectivity.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.networkconnectivity.kotlin.outputs.HubRoutingVpc
import com.pulumi.gcp.networkconnectivity.kotlin.outputs.HubRoutingVpc.Companion.toKotlin
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Any
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [Hub].
*/
@PulumiTagMarker
public class HubResourceBuilder internal constructor() {
public var name: String? = null
public var args: HubArgs = HubArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend HubArgsBuilder.() -> Unit) {
val builder = HubArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Hub {
val builtJavaResource = com.pulumi.gcp.networkconnectivity.Hub(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Hub(builtJavaResource)
}
}
/**
* The NetworkConnectivity Hub resource
* ## Example Usage
* ### Basic_hub
* A basic test of a networkconnectivity hub
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const primary = new gcp.networkconnectivity.Hub("primary", {
* name: "hub",
* description: "A sample hub",
* project: "my-project-name",
* labels: {
* "label-one": "value-one",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* primary = gcp.networkconnectivity.Hub("primary",
* name="hub",
* description="A sample hub",
* project="my-project-name",
* labels={
* "label-one": "value-one",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var primary = new Gcp.NetworkConnectivity.Hub("primary", new()
* {
* Name = "hub",
* Description = "A sample hub",
* Project = "my-project-name",
* Labels =
* {
* { "label-one", "value-one" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/networkconnectivity"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := networkconnectivity.NewHub(ctx, "primary", &networkconnectivity.HubArgs{
* Name: pulumi.String("hub"),
* Description: pulumi.String("A sample hub"),
* Project: pulumi.String("my-project-name"),
* Labels: pulumi.StringMap{
* "label-one": pulumi.String("value-one"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.networkconnectivity.Hub;
* import com.pulumi.gcp.networkconnectivity.HubArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var primary = new Hub("primary", HubArgs.builder()
* .name("hub")
* .description("A sample hub")
* .project("my-project-name")
* .labels(Map.of("label-one", "value-one"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* primary:
* type: gcp:networkconnectivity:Hub
* properties:
* name: hub
* description: A sample hub
* project: my-project-name
* labels:
* label-one: value-one
* ```
*
* ## Import
* Hub can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/global/hubs/{{name}}`
* * `{{project}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, Hub can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:networkconnectivity/hub:Hub default projects/{{project}}/locations/global/hubs/{{name}}
* ```
* ```sh
* $ pulumi import gcp:networkconnectivity/hub:Hub default {{project}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:networkconnectivity/hub:Hub default {{name}}
* ```
*/
public class Hub internal constructor(
override val javaResource: com.pulumi.gcp.networkconnectivity.Hub,
) : KotlinCustomResource(javaResource, HubMapper) {
/**
* Output only. The time the hub was created.
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* An optional description of the hub.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* All of labels (key/value pairs) present on the resource in GCP, including the labels configured through Pulumi, other clients and services.
*/
public val effectiveLabels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy