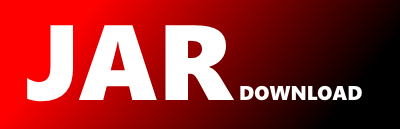
com.pulumi.gcp.networkconnectivity.kotlin.ServiceConnectionPolicy.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.networkconnectivity.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.networkconnectivity.kotlin.outputs.ServiceConnectionPolicyPscConfig
import com.pulumi.gcp.networkconnectivity.kotlin.outputs.ServiceConnectionPolicyPscConnection
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.gcp.networkconnectivity.kotlin.outputs.ServiceConnectionPolicyPscConfig.Companion.toKotlin as serviceConnectionPolicyPscConfigToKotlin
import com.pulumi.gcp.networkconnectivity.kotlin.outputs.ServiceConnectionPolicyPscConnection.Companion.toKotlin as serviceConnectionPolicyPscConnectionToKotlin
/**
* Builder for [ServiceConnectionPolicy].
*/
@PulumiTagMarker
public class ServiceConnectionPolicyResourceBuilder internal constructor() {
public var name: String? = null
public var args: ServiceConnectionPolicyArgs = ServiceConnectionPolicyArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ServiceConnectionPolicyArgsBuilder.() -> Unit) {
val builder = ServiceConnectionPolicyArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ServiceConnectionPolicy {
val builtJavaResource =
com.pulumi.gcp.networkconnectivity.ServiceConnectionPolicy(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ServiceConnectionPolicy(builtJavaResource)
}
}
/**
* Manage Service Connection Policies.
* To get more information about ServiceConnectionPolicy, see:
* * [API documentation](https://cloud.google.com/secure-web-proxy/docs/reference/networkconnectivity/rest/v1/projects.locations.networkConnectionPolicies)
* * How-to Guides
* * [About Service Connection Policies](https://cloud.google.com/vpc/docs/about-service-connection-policies#service-policies)
* ## Example Usage
* ### Network Connectivity Policy Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const producerNet = new gcp.compute.Network("producer_net", {
* name: "producer-net",
* autoCreateSubnetworks: false,
* });
* const producerSubnet = new gcp.compute.Subnetwork("producer_subnet", {
* name: "producer-subnet",
* ipCidrRange: "10.0.0.0/16",
* region: "us-central1",
* network: producerNet.id,
* });
* const _default = new gcp.networkconnectivity.ServiceConnectionPolicy("default", {
* name: "my-network-connectivity-policy",
* location: "us-central1",
* serviceClass: "my-basic-service-class",
* description: "my basic service connection policy",
* network: producerNet.id,
* pscConfig: {
* subnetworks: [producerSubnet.id],
* limit: "2",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* producer_net = gcp.compute.Network("producer_net",
* name="producer-net",
* auto_create_subnetworks=False)
* producer_subnet = gcp.compute.Subnetwork("producer_subnet",
* name="producer-subnet",
* ip_cidr_range="10.0.0.0/16",
* region="us-central1",
* network=producer_net.id)
* default = gcp.networkconnectivity.ServiceConnectionPolicy("default",
* name="my-network-connectivity-policy",
* location="us-central1",
* service_class="my-basic-service-class",
* description="my basic service connection policy",
* network=producer_net.id,
* psc_config=gcp.networkconnectivity.ServiceConnectionPolicyPscConfigArgs(
* subnetworks=[producer_subnet.id],
* limit="2",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var producerNet = new Gcp.Compute.Network("producer_net", new()
* {
* Name = "producer-net",
* AutoCreateSubnetworks = false,
* });
* var producerSubnet = new Gcp.Compute.Subnetwork("producer_subnet", new()
* {
* Name = "producer-subnet",
* IpCidrRange = "10.0.0.0/16",
* Region = "us-central1",
* Network = producerNet.Id,
* });
* var @default = new Gcp.NetworkConnectivity.ServiceConnectionPolicy("default", new()
* {
* Name = "my-network-connectivity-policy",
* Location = "us-central1",
* ServiceClass = "my-basic-service-class",
* Description = "my basic service connection policy",
* Network = producerNet.Id,
* PscConfig = new Gcp.NetworkConnectivity.Inputs.ServiceConnectionPolicyPscConfigArgs
* {
* Subnetworks = new[]
* {
* producerSubnet.Id,
* },
* Limit = "2",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/networkconnectivity"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* producerNet, err := compute.NewNetwork(ctx, "producer_net", &compute.NetworkArgs{
* Name: pulumi.String("producer-net"),
* AutoCreateSubnetworks: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* producerSubnet, err := compute.NewSubnetwork(ctx, "producer_subnet", &compute.SubnetworkArgs{
* Name: pulumi.String("producer-subnet"),
* IpCidrRange: pulumi.String("10.0.0.0/16"),
* Region: pulumi.String("us-central1"),
* Network: producerNet.ID(),
* })
* if err != nil {
* return err
* }
* _, err = networkconnectivity.NewServiceConnectionPolicy(ctx, "default", &networkconnectivity.ServiceConnectionPolicyArgs{
* Name: pulumi.String("my-network-connectivity-policy"),
* Location: pulumi.String("us-central1"),
* ServiceClass: pulumi.String("my-basic-service-class"),
* Description: pulumi.String("my basic service connection policy"),
* Network: producerNet.ID(),
* PscConfig: &networkconnectivity.ServiceConnectionPolicyPscConfigArgs{
* Subnetworks: pulumi.StringArray{
* producerSubnet.ID(),
* },
* Limit: pulumi.String("2"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.compute.Subnetwork;
* import com.pulumi.gcp.compute.SubnetworkArgs;
* import com.pulumi.gcp.networkconnectivity.ServiceConnectionPolicy;
* import com.pulumi.gcp.networkconnectivity.ServiceConnectionPolicyArgs;
* import com.pulumi.gcp.networkconnectivity.inputs.ServiceConnectionPolicyPscConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var producerNet = new Network("producerNet", NetworkArgs.builder()
* .name("producer-net")
* .autoCreateSubnetworks(false)
* .build());
* var producerSubnet = new Subnetwork("producerSubnet", SubnetworkArgs.builder()
* .name("producer-subnet")
* .ipCidrRange("10.0.0.0/16")
* .region("us-central1")
* .network(producerNet.id())
* .build());
* var default_ = new ServiceConnectionPolicy("default", ServiceConnectionPolicyArgs.builder()
* .name("my-network-connectivity-policy")
* .location("us-central1")
* .serviceClass("my-basic-service-class")
* .description("my basic service connection policy")
* .network(producerNet.id())
* .pscConfig(ServiceConnectionPolicyPscConfigArgs.builder()
* .subnetworks(producerSubnet.id())
* .limit(2)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* producerNet:
* type: gcp:compute:Network
* name: producer_net
* properties:
* name: producer-net
* autoCreateSubnetworks: false
* producerSubnet:
* type: gcp:compute:Subnetwork
* name: producer_subnet
* properties:
* name: producer-subnet
* ipCidrRange: 10.0.0.0/16
* region: us-central1
* network: ${producerNet.id}
* default:
* type: gcp:networkconnectivity:ServiceConnectionPolicy
* properties:
* name: my-network-connectivity-policy
* location: us-central1
* serviceClass: my-basic-service-class
* description: my basic service connection policy
* network: ${producerNet.id}
* pscConfig:
* subnetworks:
* - ${producerSubnet.id}
* limit: 2
* ```
*
* ## Import
* ServiceConnectionPolicy can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/serviceConnectionPolicies/{{name}}`
* * `{{project}}/{{location}}/{{name}}`
* * `{{location}}/{{name}}`
* When using the `pulumi import` command, ServiceConnectionPolicy can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:networkconnectivity/serviceConnectionPolicy:ServiceConnectionPolicy default projects/{{project}}/locations/{{location}}/serviceConnectionPolicies/{{name}}
* ```
* ```sh
* $ pulumi import gcp:networkconnectivity/serviceConnectionPolicy:ServiceConnectionPolicy default {{project}}/{{location}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:networkconnectivity/serviceConnectionPolicy:ServiceConnectionPolicy default {{location}}/{{name}}
* ```
*/
public class ServiceConnectionPolicy internal constructor(
override val javaResource: com.pulumi.gcp.networkconnectivity.ServiceConnectionPolicy,
) : KotlinCustomResource(javaResource, ServiceConnectionPolicyMapper) {
/**
* The timestamp when the resource was created.
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* Free-text description of the resource.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* All of labels (key/value pairs) present on the resource in GCP, including the labels configured through Pulumi, other clients and services.
*/
public val effectiveLabels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy