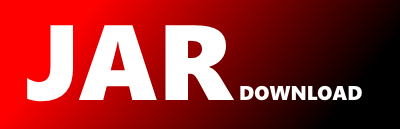
com.pulumi.gcp.networkmanagement.kotlin.inputs.ConnectivityTestSourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.networkmanagement.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.networkmanagement.inputs.ConnectivityTestSourceArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property instance A Compute Engine instance URI.
* @property ipAddress The IP address of the endpoint, which can be an external or
* internal IP. An IPv6 address is only allowed when the test's
* destination is a global load balancer VIP.
* @property network A Compute Engine network URI.
* @property networkType Type of the network where the endpoint is located.
* Possible values are: `GCP_NETWORK`, `NON_GCP_NETWORK`.
* @property port The IP protocol port of the endpoint. Only applicable when
* protocol is TCP or UDP.
* @property projectId Project ID where the endpoint is located. The Project ID can be
* derived from the URI if you provide a VM instance or network URI.
* The following are two cases where you must provide the project ID:
* 1. Only the IP address is specified, and the IP address is
* within a GCP project.
* 2. When you are using Shared VPC and the IP address
* that you provide is from the service project. In this case,
* the network that the IP address resides in is defined in the
* host project.
*/
public data class ConnectivityTestSourceArgs(
public val instance: Output? = null,
public val ipAddress: Output? = null,
public val network: Output? = null,
public val networkType: Output? = null,
public val port: Output? = null,
public val projectId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.networkmanagement.inputs.ConnectivityTestSourceArgs =
com.pulumi.gcp.networkmanagement.inputs.ConnectivityTestSourceArgs.builder()
.instance(instance?.applyValue({ args0 -> args0 }))
.ipAddress(ipAddress?.applyValue({ args0 -> args0 }))
.network(network?.applyValue({ args0 -> args0 }))
.networkType(networkType?.applyValue({ args0 -> args0 }))
.port(port?.applyValue({ args0 -> args0 }))
.projectId(projectId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConnectivityTestSourceArgs].
*/
@PulumiTagMarker
public class ConnectivityTestSourceArgsBuilder internal constructor() {
private var instance: Output? = null
private var ipAddress: Output? = null
private var network: Output? = null
private var networkType: Output? = null
private var port: Output? = null
private var projectId: Output? = null
/**
* @param value A Compute Engine instance URI.
*/
@JvmName("twjivsxvnloevgwu")
public suspend fun instance(`value`: Output) {
this.instance = value
}
/**
* @param value The IP address of the endpoint, which can be an external or
* internal IP. An IPv6 address is only allowed when the test's
* destination is a global load balancer VIP.
*/
@JvmName("ycqbpkurcdplmjdv")
public suspend fun ipAddress(`value`: Output) {
this.ipAddress = value
}
/**
* @param value A Compute Engine network URI.
*/
@JvmName("ldglibulwiivnovp")
public suspend fun network(`value`: Output) {
this.network = value
}
/**
* @param value Type of the network where the endpoint is located.
* Possible values are: `GCP_NETWORK`, `NON_GCP_NETWORK`.
*/
@JvmName("bircqbdcdybtfxrc")
public suspend fun networkType(`value`: Output) {
this.networkType = value
}
/**
* @param value The IP protocol port of the endpoint. Only applicable when
* protocol is TCP or UDP.
*/
@JvmName("jhrcmxfqpprjwbno")
public suspend fun port(`value`: Output) {
this.port = value
}
/**
* @param value Project ID where the endpoint is located. The Project ID can be
* derived from the URI if you provide a VM instance or network URI.
* The following are two cases where you must provide the project ID:
* 1. Only the IP address is specified, and the IP address is
* within a GCP project.
* 2. When you are using Shared VPC and the IP address
* that you provide is from the service project. In this case,
* the network that the IP address resides in is defined in the
* host project.
*/
@JvmName("ngftsuaquibyqgrm")
public suspend fun projectId(`value`: Output) {
this.projectId = value
}
/**
* @param value A Compute Engine instance URI.
*/
@JvmName("sftetjsduylvsxfg")
public suspend fun instance(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.instance = mapped
}
/**
* @param value The IP address of the endpoint, which can be an external or
* internal IP. An IPv6 address is only allowed when the test's
* destination is a global load balancer VIP.
*/
@JvmName("ccmmyvtheuoqtvtj")
public suspend fun ipAddress(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipAddress = mapped
}
/**
* @param value A Compute Engine network URI.
*/
@JvmName("jgeorulwltakpuhy")
public suspend fun network(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.network = mapped
}
/**
* @param value Type of the network where the endpoint is located.
* Possible values are: `GCP_NETWORK`, `NON_GCP_NETWORK`.
*/
@JvmName("kuvmslxhtrrobfea")
public suspend fun networkType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.networkType = mapped
}
/**
* @param value The IP protocol port of the endpoint. Only applicable when
* protocol is TCP or UDP.
*/
@JvmName("wcgvnpotpamdgovj")
public suspend fun port(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.port = mapped
}
/**
* @param value Project ID where the endpoint is located. The Project ID can be
* derived from the URI if you provide a VM instance or network URI.
* The following are two cases where you must provide the project ID:
* 1. Only the IP address is specified, and the IP address is
* within a GCP project.
* 2. When you are using Shared VPC and the IP address
* that you provide is from the service project. In this case,
* the network that the IP address resides in is defined in the
* host project.
*/
@JvmName("bixqikjasqypaqec")
public suspend fun projectId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.projectId = mapped
}
internal fun build(): ConnectivityTestSourceArgs = ConnectivityTestSourceArgs(
instance = instance,
ipAddress = ipAddress,
network = network,
networkType = networkType,
port = port,
projectId = projectId,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy